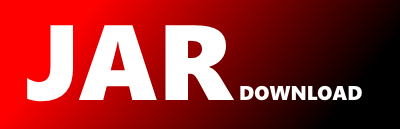
com.reprezen.genflow.common.graph.GraphSplitter Maven / Gradle / Ivy
/*******************************************************************************
* Copyright © 2013, 2016 Modelsolv, Inc.
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property
* of ModelSolv, Inc. See the file license.html in the root directory of
* this project for further information.
*******************************************************************************/
package com.reprezen.genflow.common.graph;
import java.util.ArrayList;
import java.util.Collection;
import java.util.LinkedHashSet;
import java.util.List;
/**
* Finds disconnected subgraphs.
*
* @author Tatiana Fesenko
*
*/
public class GraphSplitter {
/**
* Finds disconnected subgraphs inside the graph
*
* @param directed graph
* @return a list of disconnected subgraphs
*/
public List> splitToDisconnectedSubGraphs(DirectedGraph graph) {
List>> subgraphs = new ArrayList<>();
for (DirectedGraphNode next : graph) {
LinkedHashSet> subgraph = findOrCreateEntry(subgraphs, next);
Collection> edges = next.edgesFrom();
for (DirectedGraphNode edge : edges) {
LinkedHashSet> subgraph1 = findOrCreateEntry(subgraphs, edge);
if (subgraph != subgraph1) {
subgraph.addAll(subgraph1);
subgraphs.remove(subgraph1);
}
}
}
List> result = new ArrayList<>();
for (LinkedHashSet> subgraph : subgraphs) {
result.add(new DirectedGraph<>(subgraph));
}
return result;
}
private LinkedHashSet> findOrCreateEntry(List>> subgraphs,
DirectedGraphNode element) {
for (LinkedHashSet> subgraph : subgraphs) {
if (subgraph.contains(element)) {
return subgraph;
}
}
LinkedHashSet> newSubgraph = new LinkedHashSet<>();
newSubgraph.add(element);
subgraphs.add(newSubgraph);
return newSubgraph;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy