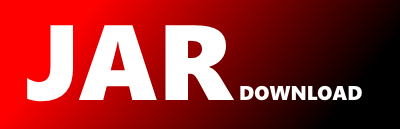
com.reprezen.genflow.common.jsonschema.JsonSchemaHelper Maven / Gradle / Ivy
/**
* Copyright © 2013, 2016 Modelsolv, Inc.
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property
* of ModelSolv, Inc. See the file license.html in the root directory of
* this project for further information.
*/
package com.reprezen.genflow.common.jsonschema;
import com.google.common.base.Objects;
import com.reprezen.rapidml.Feature;
import com.reprezen.rapidml.PrimitiveType;
import com.reprezen.rapidml.PropertyRealization;
import com.reprezen.rapidml.ReferenceElement;
import com.reprezen.rapidml.ReferenceTreatment;
import com.reprezen.rapidml.SingleValueType;
import java.util.Arrays;
import java.util.Collections;
import org.eclipse.emf.ecore.EObject;
import org.eclipse.xtext.xbase.lib.CollectionLiterals;
@SuppressWarnings("all")
public class JsonSchemaHelper {
protected boolean _isArrayProperty(final ReferenceTreatment refEmbedOrLink) {
final ReferenceElement referencedProperty = this.getReferencedProperty(refEmbedOrLink);
return ((referencedProperty.getMaxOccurs() == (-1)) || (referencedProperty.getMaxOccurs() > 1));
}
protected boolean _isArrayProperty(final PropertyRealization prop) {
return ((1 < prop.getMaxOccurs()) || ((-1) == prop.getMaxOccurs()));
}
protected boolean _isArrayProperty(final Feature prop) {
return ((1 < prop.getMaxOccurs()) || ((-1) == prop.getMaxOccurs()));
}
protected boolean _isArrayProperty(final EObject prop) {
return false;
}
public ReferenceElement getReferencedProperty(final ReferenceTreatment refEmbed) {
return refEmbed.getReferenceElement();
}
public String getJSONSchemaTypeName(final PrimitiveType type) {
String _xblockexpression = null;
{
final String typeName = type.getName();
String _switchResult = null;
boolean _matched = false;
boolean _contains = Collections.unmodifiableList(CollectionLiterals.newArrayList("anyURI", "duration", "gMonth", "gMonthDay", "gDay", "gYearMonth", "gYear", "QName", "time", "string", "NCName")).contains(typeName);
if (_contains) {
_matched=true;
_switchResult = "string";
}
if (!_matched) {
if (Objects.equal(typeName, "boolean")) {
_matched=true;
_switchResult = "boolean";
}
}
if (!_matched) {
if (Objects.equal(typeName, "base64Binary")) {
_matched=true;
_switchResult = "string";
}
}
if (!_matched) {
if (Objects.equal(typeName, "date")) {
_matched=true;
_switchResult = "string";
}
}
if (!_matched) {
if (Objects.equal(typeName, "dateTime")) {
_matched=true;
_switchResult = "string";
}
}
if (!_matched) {
if (Objects.equal(typeName, "decimal")) {
_matched=true;
_switchResult = "number";
}
}
if (!_matched) {
if (Objects.equal(typeName, "double")) {
_matched=true;
_switchResult = "number";
}
}
if (!_matched) {
if (Objects.equal(typeName, "float")) {
_matched=true;
_switchResult = "number";
}
}
if (!_matched) {
if (Objects.equal(typeName, "integer")) {
_matched=true;
_switchResult = "integer";
}
}
if (!_matched) {
if (Objects.equal(typeName, "int")) {
_matched=true;
_switchResult = "integer";
}
}
if (!_matched) {
if (Objects.equal(typeName, "long")) {
_matched=true;
_switchResult = "integer";
}
}
if (!_matched) {
_switchResult = "string";
}
_xblockexpression = _switchResult;
}
return _xblockexpression;
}
public String getJSONSchemaTypeName(final SingleValueType type) {
return this.getJSONSchemaTypeName(type.getPrimitiveType());
}
public String getJSONSchemaTypeFormat(final PrimitiveType type) {
String _switchResult = null;
String _name = type.getName();
if (_name != null) {
switch (_name) {
case "date":
_switchResult = "date";
break;
case "dateTime":
_switchResult = "date-time";
break;
case "double":
_switchResult = "double";
break;
case "float":
_switchResult = "float";
break;
case "long":
_switchResult = "int64";
break;
}
}
return _switchResult;
}
public String getJSONSchemaTypeFormat(final SingleValueType type) {
return this.getJSONSchemaTypeFormat(type.getPrimitiveType());
}
public boolean isZenNumericType(final String typeName) {
return Collections.unmodifiableList(CollectionLiterals.newArrayList("decimal", "double", "float", "integer", "int", "long")).contains(typeName);
}
public boolean isArrayProperty(final EObject prop) {
if (prop instanceof PropertyRealization) {
return _isArrayProperty((PropertyRealization)prop);
} else if (prop instanceof Feature) {
return _isArrayProperty((Feature)prop);
} else if (prop instanceof ReferenceTreatment) {
return _isArrayProperty((ReferenceTreatment)prop);
} else if (prop != null) {
return _isArrayProperty(prop);
} else {
throw new IllegalArgumentException("Unhandled parameter types: " +
Arrays.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy