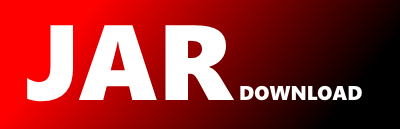
com.reprezen.genflow.common.jsonschema.builder.ConstraintNode Maven / Gradle / Ivy
package com.reprezen.genflow.common.jsonschema.builder;
import com.fasterxml.jackson.databind.node.ObjectNode;
import com.reprezen.genflow.common.jsonschema.JacksonUtils;
import com.reprezen.genflow.common.jsonschema.builder.JsonSchemaNode;
import com.reprezen.genflow.common.jsonschema.builder.JsonSchemaNodeFactory;
import com.reprezen.rapidml.Constraint;
import com.reprezen.rapidml.LengthConstraint;
import com.reprezen.rapidml.RegExConstraint;
import com.reprezen.rapidml.ValueRangeConstraint;
import java.text.NumberFormat;
import org.eclipse.xtext.xbase.lib.Exceptions;
import org.eclipse.xtext.xbase.lib.Extension;
@SuppressWarnings("all")
public class ConstraintNode extends JsonSchemaNode {
@Extension
private JacksonUtils _jacksonUtils = new JacksonUtils();
public ConstraintNode(final JsonSchemaNodeFactory factory, final Constraint element) {
super(factory, element);
}
@Override
public ObjectNode write(final ObjectNode node) {
ObjectNode _switchResult = null;
final Constraint element = this.element;
boolean _matched = false;
if (element instanceof LengthConstraint) {
_matched=true;
ObjectNode _xblockexpression = null;
{
boolean _isSetMinLength = ((LengthConstraint)this.element).isSetMinLength();
if (_isSetMinLength) {
node.put("minLength", ((LengthConstraint)this.element).getMinLength());
}
ObjectNode _xifexpression = null;
boolean _isSetMaxLength = ((LengthConstraint)this.element).isSetMaxLength();
if (_isSetMaxLength) {
_xifexpression = node.put("maxLength", ((LengthConstraint)this.element).getMaxLength());
}
_xblockexpression = _xifexpression;
}
_switchResult = _xblockexpression;
}
if (!_matched) {
if (element instanceof RegExConstraint) {
_matched=true;
ObjectNode _xifexpression = null;
String _pattern = ((RegExConstraint)this.element).getPattern();
boolean _tripleNotEquals = (_pattern != null);
if (_tripleNotEquals) {
String _pattern_1 = ((RegExConstraint)this.element).getPattern();
String _plus = ("^" + _pattern_1);
String _plus_1 = (_plus + "$");
_xifexpression = node.put("pattern", _plus_1);
}
_switchResult = _xifexpression;
}
}
if (!_matched) {
if (element instanceof ValueRangeConstraint) {
_matched=true;
ObjectNode _xblockexpression = null;
{
String _minValue = ((ValueRangeConstraint)this.element).getMinValue();
boolean _tripleNotEquals = (_minValue != null);
if (_tripleNotEquals) {
String value = ((ValueRangeConstraint)this.element).getMinValue();
try {
final Number number = NumberFormat.getInstance().parse(value);
this._jacksonUtils.putNumber(node, "minimum", number);
} catch (final Throwable _t) {
if (_t instanceof Exception) {
node.put("minimum", value);
} else {
throw Exceptions.sneakyThrow(_t);
}
}
}
boolean _isMinValueExclusive = ((ValueRangeConstraint)this.element).isMinValueExclusive();
if (_isMinValueExclusive) {
node.put("exclusiveMinimum", ((ValueRangeConstraint)this.element).isMinValueExclusive());
}
String _maxValue = ((ValueRangeConstraint)this.element).getMaxValue();
boolean _tripleNotEquals_1 = (_maxValue != null);
if (_tripleNotEquals_1) {
node.put("maximum", ((ValueRangeConstraint)this.element).getMaxValue());
String value_1 = ((ValueRangeConstraint)this.element).getMaxValue();
try {
final Number number_1 = NumberFormat.getInstance().parse(value_1);
this._jacksonUtils.putNumber(node, "maximum", number_1);
} catch (final Throwable _t_1) {
if (_t_1 instanceof Exception) {
node.put("maximum", value_1);
} else {
throw Exceptions.sneakyThrow(_t_1);
}
}
}
ObjectNode _xifexpression = null;
boolean _isMaxValueExclusive = ((ValueRangeConstraint)this.element).isMaxValueExclusive();
if (_isMaxValueExclusive) {
_xifexpression = node.put("exclusiveMaximum", ((ValueRangeConstraint)this.element).isMaxValueExclusive());
}
_xblockexpression = _xifexpression;
}
_switchResult = _xblockexpression;
}
}
return _switchResult;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy