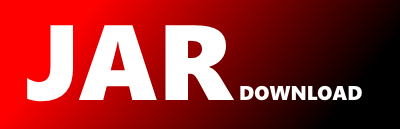
com.reprezen.genflow.common.jsonschema.builder.EnumerationNode Maven / Gradle / Ivy
package com.reprezen.genflow.common.jsonschema.builder;
import com.fasterxml.jackson.databind.node.ArrayNode;
import com.fasterxml.jackson.databind.node.ObjectNode;
import com.reprezen.genflow.common.jsonschema.JsonSchemaHelper;
import com.reprezen.genflow.common.jsonschema.builder.JsonSchemaNodeFactory;
import com.reprezen.genflow.common.jsonschema.builder.TypedNode;
import com.reprezen.genflow.common.xtend.XImportHelper;
import com.reprezen.rapidml.EnumConstant;
import com.reprezen.rapidml.Enumeration;
import java.util.List;
import java.util.function.Consumer;
import org.eclipse.xtext.xbase.lib.Extension;
import org.eclipse.xtext.xbase.lib.Functions.Function1;
import org.eclipse.xtext.xbase.lib.ListExtensions;
@SuppressWarnings("all")
public class EnumerationNode extends TypedNode {
@Extension
protected JsonSchemaHelper _jsonSchemaHelper = new JsonSchemaHelper();
private final XImportHelper importHelper;
public EnumerationNode(final JsonSchemaNodeFactory director, final Enumeration element, final XImportHelper importHelper) {
super(director, element);
this.importHelper = importHelper;
}
@Override
public String getName() {
return this.importHelper.getQualifiedName(this.element);
}
@Override
public void writeBody(final ObjectNode body) {
this.writePropertyType(body, this.element.getBaseType());
this.putDescription(body, this._zenModelHelper.getDocumentation(this.element));
final boolean isNumeric = this._jsonSchemaHelper.isZenNumericType(this.element.getBaseType().getName());
final Function1 _function = (EnumConstant it) -> {
return this.getEnumConstant(it, isNumeric);
};
final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy