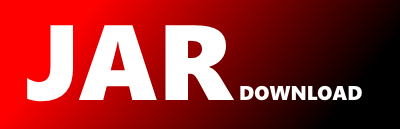
com.reprezen.genflow.common.jsonschema.builder.FeatureNode Maven / Gradle / Ivy
package com.reprezen.genflow.common.jsonschema.builder;
import com.fasterxml.jackson.databind.node.ObjectNode;
import com.reprezen.genflow.common.jsonschema.builder.JsonSchemaNodeFactory;
import com.reprezen.genflow.common.jsonschema.builder.NamedSchemaNode;
import com.reprezen.genflow.common.jsonschema.builder.PropertyNode;
import com.reprezen.genflow.common.jsonschema.builder.ResourceLinkNode;
import com.reprezen.rapidml.Feature;
import com.reprezen.rapidml.PrimitiveProperty;
import com.reprezen.rapidml.ReferenceProperty;
import com.reprezen.rapidml.ServiceDataResource;
import com.reprezen.rapidml.Structure;
@SuppressWarnings("all")
public class FeatureNode extends PropertyNode {
public FeatureNode(final JsonSchemaNodeFactory director, final Feature element) {
super(director, element);
}
@Override
public String getName() {
return this.element.getName();
}
@Override
public void writeType(final ObjectNode node) {
final Feature element = this.element;
boolean _matched = false;
if (element instanceof ReferenceProperty) {
_matched=true;
final Structure type = ((ReferenceProperty)this.element).getType();
this.factory.getDefinitionsNode().addReferenceToDefinition(node,
this.createReferenceToStructureNode(type, this.isMultiValued()));
}
if (!_matched) {
if (element instanceof PrimitiveProperty) {
_matched=true;
this.writeConstrainableType(node, ((PrimitiveProperty)this.element), ((PrimitiveProperty)this.element).getType());
}
}
}
public NamedSchemaNode> createReferenceToStructureNode(final Structure structure, final boolean isMultiValued) {
final ServiceDataResource defaultResource = this.factory.getDefaultResource(structure, isMultiValued);
if ((defaultResource != null)) {
return new ResourceLinkNode(this.factory, defaultResource);
}
return this.factory.createStructureNode(structure);
}
@Override
public boolean isArrayProperty() {
return (this.isMultiValued() && (!this.isMultiValuedReferenceWithCollectionResource()));
}
public boolean isMultiValuedReferenceWithCollectionResource() {
return ((this.isMultiValued() && (this.element instanceof ReferenceProperty)) && (this.factory.getDefaultResource(((ReferenceProperty) this.element).getType(), true) != null));
}
@Override
public int getMinOccurs() {
return this.element.getMinOccurs();
}
@Override
public int getMaxOccurs() {
return this.element.getMaxOccurs();
}
@Override
public Feature getBaseFeature() {
return this.element;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy