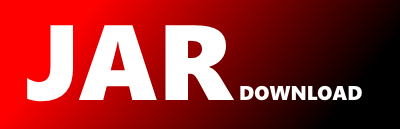
com.reprezen.genflow.common.jsonschema.builder.NamedSchemaNode Maven / Gradle / Ivy
package com.reprezen.genflow.common.jsonschema.builder;
import com.fasterxml.jackson.databind.node.ArrayNode;
import com.fasterxml.jackson.databind.node.ObjectNode;
import com.google.common.base.Strings;
import com.reprezen.genflow.common.jsonschema.builder.JsonSchemaNode;
import com.reprezen.genflow.common.jsonschema.builder.JsonSchemaNodeFactory;
import com.reprezen.genflow.common.xtend.ExtensionsHelper;
import com.reprezen.rapidml.Feature;
import com.reprezen.rapidml.ObjectRealization;
import com.reprezen.rapidml.PropertyRealization;
import com.reprezen.rapidml.RealizationContainer;
import com.reprezen.rapidml.Structure;
import com.reprezen.rapidml.xtext.util.ZenModelHelper;
import java.util.function.Consumer;
import org.eclipse.emf.common.util.EList;
import org.eclipse.xtext.xbase.lib.Extension;
import org.eclipse.xtext.xbase.lib.Functions.Function1;
import org.eclipse.xtext.xbase.lib.IterableExtensions;
@SuppressWarnings("all")
public abstract class NamedSchemaNode extends JsonSchemaNode {
@Extension
protected ZenModelHelper _zenModelHelper = new ZenModelHelper();
@Extension
protected ExtensionsHelper _extensionsHelper = new ExtensionsHelper();
public NamedSchemaNode(final JsonSchemaNodeFactory factory, final T element) {
super(factory, element);
}
@Override
public ObjectNode write(final ObjectNode parentNode) {
final ObjectNode body = parentNode.putObject(this.getName());
this.writeBody(body);
return body;
}
public abstract void writeBody(final ObjectNode bodyNode);
public abstract String getName();
protected Iterable getRequiredPropertyNames(final RealizationContainer realization) {
ObjectRealization _properties = realization.getProperties();
EList _allIncludedProperties = null;
if (_properties!=null) {
_allIncludedProperties=_properties.getAllIncludedProperties();
}
final Function1 _function = (PropertyRealization e) -> {
int _minOccurs = e.getMinOccurs();
return Boolean.valueOf((_minOccurs > 0));
};
final Function1 _function_1 = (PropertyRealization it) -> {
return it.getBaseProperty().getName();
};
return IterableExtensions.map(IterableExtensions.filter(_allIncludedProperties, _function), _function_1);
}
protected Iterable getRequiredPropertyNames(final Structure datatype) {
final Function1 _function = (Feature e) -> {
int _minOccurs = e.getMinOccurs();
return Boolean.valueOf((_minOccurs > 0));
};
final Function1 _function_1 = (Feature it) -> {
return it.getName();
};
return IterableExtensions.map(IterableExtensions.filter(datatype.getOwnedFeatures(), _function), _function_1);
}
protected ObjectNode writeRequiredProperties(final ObjectNode node, final Iterable requiredPropNames) {
if (((!this.factory.schemaFormat.defineRequiredElementsInJsonSchemaV3Style) && (!IterableExtensions.isEmpty(requiredPropNames)))) {
final ArrayNode requiredNode = node.putArray("required");
final Consumer _function = (String it) -> {
requiredNode.add(it);
};
requiredPropNames.forEach(_function);
}
return node;
}
protected ObjectNode writePropertyAsRequired(final ObjectNode node) {
if (this.factory.schemaFormat.defineRequiredElementsInJsonSchemaV3Style) {
node.put("required", true);
}
return node;
}
protected ObjectNode putDescription(final ObjectNode body, final String doc) {
ObjectNode _xifexpression = null;
boolean _isNullOrEmpty = Strings.isNullOrEmpty(doc);
boolean _not = (!_isNullOrEmpty);
if (_not) {
_xifexpression = body.put("description", doc);
}
return _xifexpression;
}
protected void addVendorExtensions(final ObjectNode body, final Iterable extensions) {
if (extensions!=null) {
final Consumer _function = (com.reprezen.rapidml.Extension it) -> {
this.addVendorExtension(body, it.getName(), it.getValue());
};
extensions.forEach(_function);
}
}
protected ObjectNode addVendorExtension(final ObjectNode body, final String tag, final String value) {
return body.put(tag, value);
}
protected ObjectNode Description(final ObjectNode body, final String doc) {
ObjectNode _xifexpression = null;
boolean _isNullOrEmpty = Strings.isNullOrEmpty(doc);
boolean _not = (!_isNullOrEmpty);
if (_not) {
_xifexpression = body.put("description", doc);
}
return _xifexpression;
}
protected ObjectNode setObjectAsType(final ObjectNode body) {
body.put("type", "object");
boolean _isAllowEmptyObject = this.factory.getOptions().isAllowEmptyObject();
boolean _not = (!_isAllowEmptyObject);
if (_not) {
body.put("minProperties", 1);
}
return body;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy