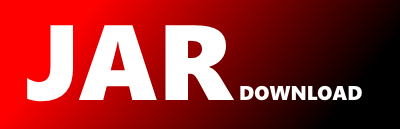
com.reprezen.genflow.common.jsonschema.builder.PropertyNode Maven / Gradle / Ivy
package com.reprezen.genflow.common.jsonschema.builder;
import com.fasterxml.jackson.databind.node.ObjectNode;
import com.google.common.base.Strings;
import com.reprezen.genflow.common.jsonschema.builder.JsonSchemaNodeFactory;
import com.reprezen.genflow.common.jsonschema.builder.NamedSchemaNode;
import com.reprezen.genflow.common.jsonschema.builder.TypedNode;
import com.reprezen.rapidml.ConstrainableType;
import com.reprezen.rapidml.Constraint;
import com.reprezen.rapidml.Enumeration;
import com.reprezen.rapidml.Extension;
import com.reprezen.rapidml.Feature;
import com.reprezen.rapidml.PrimitiveProperty;
import com.reprezen.rapidml.PropertyRealization;
import com.reprezen.rapidml.SingleValueType;
import java.util.Arrays;
import org.eclipse.emf.common.util.EList;
@SuppressWarnings("all")
public abstract class PropertyNode extends TypedNode {
public PropertyNode(final JsonSchemaNodeFactory director, final T element) {
super(director, element);
}
@Override
public void writeBody(final ObjectNode bodyNode) {
boolean _isArrayProperty = this.isArrayProperty();
if (_isArrayProperty) {
this.writeArray(bodyNode);
} else {
this.writeObject(bodyNode);
}
Feature _baseFeature = this.getBaseFeature();
Iterable _rapidExtensions = null;
if (_baseFeature!=null) {
_rapidExtensions=this._extensionsHelper.getRapidExtensions(_baseFeature);
}
this.addVendorExtensions(bodyNode, _rapidExtensions);
}
public void writeArray(final ObjectNode body) {
this.putDescription(body, this.getPropertyDocumentation());
body.put("type", "array");
int minOccursValue = this.getMinOccurs();
boolean _isAllowEmptyArray = this.factory.getOptions().isAllowEmptyArray();
boolean _not = (!_isAllowEmptyArray);
if (_not) {
minOccursValue = Math.max(1, minOccursValue);
}
if ((minOccursValue > 0)) {
body.put("minItems", minOccursValue);
}
int _maxOccurs = this.getMaxOccurs();
boolean _greaterThan = (_maxOccurs > 0);
if (_greaterThan) {
body.put("maxItems", this.getMaxOccurs());
}
final ObjectNode items = body.putObject("items");
this.writeType(items);
}
public void writeObject(final ObjectNode body) {
final String doc = this.getPropertyDocumentation();
boolean _isNullOrEmpty = Strings.isNullOrEmpty(doc);
boolean _not = (!_isNullOrEmpty);
if (_not) {
body.put("description", doc);
}
this.writeType(body);
}
protected ObjectNode writeConstrainableType(final ObjectNode node, final ConstrainableType property, final SingleValueType type) {
if ((type instanceof Enumeration)) {
return this.writeEnum(node, ((Enumeration) type));
}
this.writePropertyType(node, type);
boolean _isReadOnly = this.isReadOnly(property);
if (_isReadOnly) {
node.put("readOnly", true);
}
EList _allConstraints = property.getAllConstraints();
for (final Constraint constraint : _allConstraints) {
this.factory.createConstraintNode(constraint).write(node);
}
return node;
}
private ObjectNode writeEnum(final ObjectNode node, final Enumeration enumType) {
ObjectNode _xblockexpression = null;
{
final NamedSchemaNode enumerationBuilder = this.factory.createEnumerationNode(enumType);
ObjectNode _xifexpression = null;
if (this.factory.schemaFormat.inlineSimpleTypes) {
_xifexpression = enumerationBuilder.write(node);
} else {
this.factory.getDefinitionsNode().addReferenceToDefinition(node, enumerationBuilder);
return node;
}
_xblockexpression = _xifexpression;
}
return _xblockexpression;
}
public String getPropertyDocumentation() {
return this._zenModelHelper.getDocumentation(this.getBaseFeature());
}
public abstract void writeType(final ObjectNode body);
public boolean isArrayProperty() {
return this.isMultiValued();
}
public boolean isMultiValued() {
return ((1 < this.getMaxOccurs()) || ((-1) == this.getMaxOccurs()));
}
public abstract int getMinOccurs();
public abstract int getMaxOccurs();
public abstract Feature getBaseFeature();
private boolean _isReadOnly(final ConstrainableType property) {
return false;
}
private boolean _isReadOnly(final PropertyRealization property) {
return property.getBaseProperty().isReadOnly();
}
private boolean _isReadOnly(final PrimitiveProperty property) {
return property.isReadOnly();
}
private boolean isReadOnly(final ConstrainableType property) {
if (property instanceof PrimitiveProperty) {
return _isReadOnly((PrimitiveProperty)property);
} else if (property instanceof PropertyRealization) {
return _isReadOnly((PropertyRealization)property);
} else if (property != null) {
return _isReadOnly(property);
} else {
throw new IllegalArgumentException("Unhandled parameter types: " +
Arrays.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy