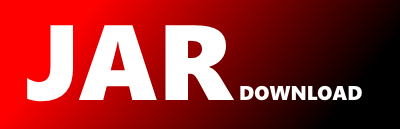
com.reprezen.genflow.common.xtend.XParameterHelper Maven / Gradle / Ivy
/**
* Copyright © 2013, 2016 Modelsolv, Inc.
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property
* of ModelSolv, Inc. See the file license.html in the root directory of
* this project for further information.
*/
package com.reprezen.genflow.common.xtend;
import com.reprezen.genflow.common.xtend.XImportHelper;
import com.reprezen.rapidml.Feature;
import com.reprezen.rapidml.MessageParameter;
import com.reprezen.rapidml.Parameter;
import com.reprezen.rapidml.PrimitiveProperty;
import com.reprezen.rapidml.PropertyReference;
import com.reprezen.rapidml.ReferenceProperty;
import com.reprezen.rapidml.ReferenceTreatment;
import com.reprezen.rapidml.SourceReference;
import com.reprezen.rapidml.TypedMessage;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import org.eclipse.xtext.xbase.lib.Functions.Function1;
import org.eclipse.xtext.xbase.lib.IterableExtensions;
/**
* Helper methods for parameters.
*/
@SuppressWarnings("all")
public class XParameterHelper {
public Iterable getPrimitiveParameters(final TypedMessage aMessage) {
final Function1 _function = (MessageParameter p) -> {
return Boolean.valueOf(this.isPrimitivePropertyParameter(p.getSourceReference()));
};
return IterableExtensions.filter(aMessage.getParameters(), _function);
}
public PrimitiveProperty getPrimitiveProperty(final Parameter aParameter) {
SourceReference _sourceReference = aParameter.getSourceReference();
return ((PropertyReference) _sourceReference).getConceptualFeature();
}
public Iterable extends Parameter> getReferenceParameters(final TypedMessage aMessage) {
final Function1 _function = (MessageParameter p) -> {
return Boolean.valueOf(this.isReferencePropertyParameter(p.getSourceReference()));
};
return IterableExtensions.filter(aMessage.getParameters(), _function);
}
/**
* @return parameter name depend on reference class
*/
protected String _paramName(final PropertyReference propertyReference) {
return propertyReference.getConceptualFeature().getName();
}
protected String _paramName(final SourceReference sourceReference) {
return "";
}
/**
* @return parameter type depend on reference class
*/
public String paramType(final SourceReference propertyReference, final XImportHelper importHelper) {
return importHelper.getQualifiedName(propertyReference.getType());
}
/**
* @return is source reference a primitive property
*/
protected boolean _isPrimitivePropertyParameter(final SourceReference sourceReference) {
return false;
}
protected boolean _isPrimitivePropertyParameter(final PropertyReference propertyReference) {
PrimitiveProperty _conceptualFeature = propertyReference.getConceptualFeature();
return (_conceptualFeature instanceof PrimitiveProperty);
}
/**
* @return is source reference a property reference
*/
protected boolean _isReferencePropertyParameter(final SourceReference sourceReference) {
return false;
}
protected boolean _isReferencePropertyParameter(final PropertyReference sourceReference) {
PrimitiveProperty _conceptualFeature = sourceReference.getConceptualFeature();
return (_conceptualFeature instanceof ReferenceProperty);
}
/**
* @return feature type depend on property type
*/
protected String _featureType(final ReferenceProperty feature, final XImportHelper importHelper) {
return feature.getType().getName();
}
protected String _featureType(final PrimitiveProperty feature, final XImportHelper importHelper) {
return feature.getType().getName();
}
public List getContainmentReferences(final ReferenceTreatment aReferenceLink) {
return Collections.emptyList();
}
public String paramName(final SourceReference propertyReference) {
if (propertyReference instanceof PropertyReference) {
return _paramName((PropertyReference)propertyReference);
} else if (propertyReference != null) {
return _paramName(propertyReference);
} else {
throw new IllegalArgumentException("Unhandled parameter types: " +
Arrays.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy