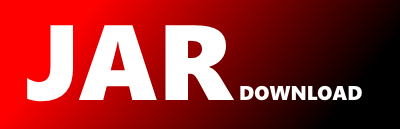
com.reprezen.genflow.common.xtend.ZenModelHelper Maven / Gradle / Ivy
/**
* Copyright © 2013, 2016 Modelsolv, Inc.
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property
* of ModelSolv, Inc. See the file license.html in the root directory of
* this project for further information.
*/
package com.reprezen.genflow.common.xtend;
import com.google.common.collect.Iterables;
import com.reprezen.rapidml.Documentable;
import com.reprezen.rapidml.Documentation;
import com.reprezen.rapidml.Enumeration;
import com.reprezen.rapidml.Feature;
import com.reprezen.rapidml.ObjectRealization;
import com.reprezen.rapidml.PrimitiveProperty;
import com.reprezen.rapidml.PropertyRealization;
import com.reprezen.rapidml.RealizationContainer;
import com.reprezen.rapidml.ReferenceElement;
import com.reprezen.rapidml.ReferenceEmbed;
import com.reprezen.rapidml.ReferenceLink;
import com.reprezen.rapidml.ReferenceProperty;
import com.reprezen.rapidml.ReferenceTreatment;
import com.reprezen.rapidml.ResourceAPI;
import com.reprezen.rapidml.ServiceDataResource;
import com.reprezen.rapidml.SimpleType;
import com.reprezen.rapidml.SingleValueType;
import com.reprezen.rapidml.TypedMessage;
import com.reprezen.rapidml.UserDefinedType;
import com.reprezen.rapidml.ZenModel;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import org.eclipse.emf.common.util.EList;
import org.eclipse.emf.ecore.EObject;
import org.eclipse.xtext.xbase.lib.Functions.Function1;
import org.eclipse.xtext.xbase.lib.IterableExtensions;
import org.eclipse.xtext.xbase.lib.ListExtensions;
@SuppressWarnings("all")
public class ZenModelHelper {
protected List> _getReferenceTreatmentIncludedProperties(final ReferenceEmbed refEmbed) {
final ArrayList includedProps = new ArrayList();
includedProps.addAll(this.getIncludedPrimitiveProperties(refEmbed));
includedProps.addAll(refEmbed.getNestedReferenceTreatments());
return includedProps;
}
protected List> _getReferenceTreatmentIncludedProperties(final ReferenceLink refEmbed) {
return this.getIncludedPrimitiveProperties(refEmbed);
}
public List getIncludedPrimitiveProperties(final ReferenceTreatment refTreatment) {
final Function1 _function = (Feature e) -> {
return Boolean.valueOf((e instanceof PrimitiveProperty));
};
return IterableExtensions.toList(IterableExtensions.filter(this.getIncludedProperties(refTreatment), _function));
}
public List getIncludedProperties(final ReferenceTreatment refLink) {
List _elvis = null;
ObjectRealization _linkDescriptor = refLink.getLinkDescriptor();
EList _allIncludedProperties = null;
if (_linkDescriptor!=null) {
_allIncludedProperties=_linkDescriptor.getAllIncludedProperties();
}
List _map = null;
if (_allIncludedProperties!=null) {
final Function1 _function = (PropertyRealization it) -> {
return it.getBaseProperty();
};
_map=ListExtensions.map(_allIncludedProperties, _function);
}
if (_map != null) {
_elvis = _map;
} else {
List _emptyList = Collections.emptyList();
_elvis = _emptyList;
}
return _elvis;
}
public boolean hasReferenceTreatment(final RealizationContainer resource, final Feature feature) {
final Function1 _function = (ReferenceTreatment it) -> {
return it.getReferenceElement();
};
final Function1 _function_1 = (ReferenceElement f) -> {
return Boolean.valueOf((f == feature));
};
return IterableExtensions.exists(ListExtensions.map(resource.getReferenceTreatments(), _function), _function_1);
}
public boolean hasReferenceTreatment(final TypedMessage resource, final Feature feature) {
final Function1 _function = (ReferenceTreatment it) -> {
return it.getReferenceElement();
};
final Function1 _function_1 = (ReferenceElement f) -> {
return Boolean.valueOf((f == feature));
};
return IterableExtensions.exists(ListExtensions.map(resource.getReferenceTreatments(), _function), _function_1);
}
protected Collection _getUsedEnums(final Object o) {
String _string = Arrays.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy