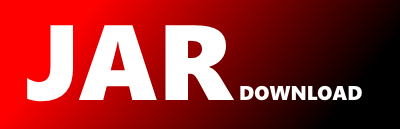
com.reprezen.genflow.common.xtend.ZenModelHelper.xtend Maven / Gradle / Ivy
/*******************************************************************************
* Copyright © 2013, 2016 Modelsolv, Inc.
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property
* of ModelSolv, Inc. See the file license.html in the root directory of
* this project for further information.
*******************************************************************************/
package com.reprezen.genflow.common.xtend
import com.reprezen.rapidml.Documentable
import com.reprezen.rapidml.Enumeration
import com.reprezen.rapidml.Feature
import com.reprezen.rapidml.PrimitiveProperty
import com.reprezen.rapidml.RealizationContainer
import com.reprezen.rapidml.ReferenceEmbed
import com.reprezen.rapidml.ReferenceLink
import com.reprezen.rapidml.ReferenceProperty
import com.reprezen.rapidml.ReferenceTreatment
import com.reprezen.rapidml.ResourceAPI
import com.reprezen.rapidml.ServiceDataResource
import com.reprezen.rapidml.TypedMessage
import com.reprezen.rapidml.UserDefinedType
import com.reprezen.rapidml.ZenModel
import java.util.ArrayList
import java.util.Arrays
import java.util.Collection
import java.util.Collections
import java.util.List
import org.eclipse.emf.ecore.EObject
class ZenModelHelper {
protected def dispatch List extends Object> getReferenceTreatmentIncludedProperties(ReferenceEmbed refEmbed) {
// contains Features and ReferenceTreatments
val includedProps = new ArrayList();
includedProps.addAll(getIncludedPrimitiveProperties(refEmbed))
includedProps.addAll(refEmbed.nestedReferenceTreatments)
return includedProps
}
protected def dispatch getReferenceTreatmentIncludedProperties(ReferenceLink refEmbed) {
return getIncludedPrimitiveProperties(refEmbed)
}
def List getIncludedPrimitiveProperties(ReferenceTreatment refTreatment) {
return getIncludedProperties(refTreatment).filter[e|e instanceof PrimitiveProperty].toList
}
def List getIncludedProperties(ReferenceTreatment refLink) {
refLink.linkDescriptor?.allIncludedProperties?.map[baseProperty] ?: Collections::emptyList
}
def boolean hasReferenceTreatment(RealizationContainer resource, Feature feature) {
resource.referenceTreatments.map[referenceElement].exists[f|f === feature]
}
def boolean hasReferenceTreatment(TypedMessage resource, Feature feature) {
// TODO remove this method when TypedMessage and ServiceDataResource have a shared ObjectRealization supertype
resource.referenceTreatments.map[referenceElement].exists[f|f === feature]
}
def dispatch Collection getUsedEnums(Object o) {
// For Xtend compilation. Otherwise it tries to cast to Extensible
throw new IllegalArgumentException("Unhandled parameter types: " + Arrays.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy