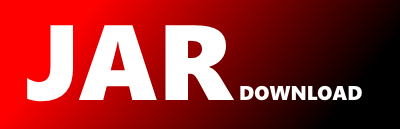
com.reprezen.genflow.api.target.GenTarget Maven / Gradle / Ivy
/*******************************************************************************
* Copyright © 2013, 2016 Modelsolv, Inc.
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property
* of ModelSolv, Inc. See the file license.html in the root directory of
* this project for further information.
*******************************************************************************/
package com.reprezen.genflow.api.target;
import java.io.File;
import java.io.IOException;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.logging.Logger;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.google.common.collect.Maps;
import com.reprezen.genflow.api.GenerationException;
import com.reprezen.genflow.api.template.GenTemplateRegistry;
import com.reprezen.genflow.api.template.IGenTemplate;
import com.reprezen.genflow.api.template.IGenTemplate.Generator;
import com.reprezen.genflow.api.trace.GenTemplateTrace;
import com.reprezen.genflow.api.trace.GenTemplateTraces;
/**
* @author Konstantin Zaitsev
* @date May 19, 2015
*/
public class GenTarget {
/**
* Name of this GenTarget (used in naming of .gen file.
*
* This is typically copied from the name of the GenTemplate executed by this
* GenTarget, but it need not be. For example, two GenTargets in the same
* directory that execute the same GenTemplate must have different names.
*/
private String name;
/** ID of GenTemplate executed by this GenTarget */
private String genTemplateId;
/**
* Instance of GenTemplate type to be executed.
*
* If this is null when execution is requested, it will be looked up in the
* headless registry using the id.
*/
@JsonIgnore
private IGenTemplate genTemplate = null;
/**
* Base directory for resolving file paths.
*
* This is not saved in the serialized form, and it is set during
* deserialization to the directory containing the GenTarget, to achieve a level
* of portability.
*/
@JsonIgnore
private File baseDir;
/** Generation output directory relative to {@link #baseDir}. */
private File relativeOutputDir;
private GenTargetPrimarySource primarySource;
/** Paths to prerequisite .gen files for prerequisite GenTargets. */
Map prerequisites = Maps.newHashMap();
private final Map namedSources = Maps.newHashMap();
private Map parameters = Maps.newHashMap();
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getGenTemplateId() {
return genTemplateId;
}
public void setGenTemplateId(String genTemplateId) {
this.genTemplateId = genTemplateId;
}
public void setGenTemplate(IGenTemplate genTemplate) {
this.genTemplate = genTemplate;
setGenTemplateId(genTemplate.getId());
}
public File getBaseDir() {
return baseDir;
}
public void setBaseDir(File baseDir) {
this.baseDir = baseDir;
}
public File getRelativeOutputDir() {
return relativeOutputDir;
}
public void setRelativeOutputDir(File relativeOutputDir) {
this.relativeOutputDir = relativeOutputDir;
}
public GenTargetPrimarySource getPrimarySource() {
return primarySource;
}
public void setPrimarySource(GenTargetPrimarySource primarySource) {
this.primarySource = primarySource;
}
public Map getPrerequisites() {
return prerequisites;
}
@JsonProperty("prerequisites")
public Collection getPrerequisiteList() {
return prerequisites.values();
}
@JsonProperty("prerequisites")
public void setPrerequisiteList(List prerequisiteList) {
prerequisites.clear();
if (prerequisiteList != null) {
for (GenTargetPrerequisite prerequisite : prerequisiteList) {
this.prerequisites.put(prerequisite.getName(), prerequisite);
}
}
}
public Map getNamedSources() {
return namedSources;
}
@JsonProperty("namedSources")
public Collection getNamedSourceList() {
return namedSources.values();
}
@JsonProperty("namedSources")
public void SetNamedSourceList(List namedSourceList) {
namedSources.clear();
if (namedSourceList != null) {
for (GenTargetNamedSource namedSource : namedSourceList) {
namedSources.put(namedSource.getName(), namedSource);
}
}
}
public Map getParameters() {
return parameters;
}
@JsonProperty("parameters")
public void setParameters(Object parameters) throws GenerationException {
if (parameters == null) {
this.parameters = Maps.newHashMap();
} else if (parameters instanceof Map) {
@SuppressWarnings("unchecked")
Map parameterMap = (Map) parameters;
this.parameters = parameterMap;
} else if (parameters instanceof Collection) {
@SuppressWarnings("unchecked")
Collection