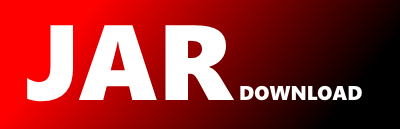
com.reprezen.genflow.openapi.diagram.swagger.XtendHelper Maven / Gradle / Ivy
/**
* Copyright © 2013, 2016 Modelsolv, Inc.
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property
* of ModelSolv, Inc. See the file license.html in the root directory of
* this project for further information.
*/
package com.reprezen.genflow.openapi.diagram.swagger;
import com.google.common.collect.Iterables;
import com.google.common.collect.Maps;
import java.util.Collections;
import java.util.Comparator;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.SortedMap;
import java.util.TreeMap;
import org.eclipse.xtext.xbase.lib.Conversions;
import org.eclipse.xtext.xbase.lib.Functions.Function1;
import org.eclipse.xtext.xbase.lib.Functions.Function2;
import org.eclipse.xtext.xbase.lib.IterableExtensions;
import org.eclipse.xtext.xbase.lib.StringExtensions;
@SuppressWarnings("all")
public class XtendHelper {
public Map safe(final Map map) {
Map _elvis = null;
if (map != null) {
_elvis = map;
} else {
Map _emptyMap = Collections.emptyMap();
_elvis = _emptyMap;
}
return _elvis;
}
public Iterable safe(final Iterable list) {
Iterable _elvis = null;
if (list != null) {
_elvis = list;
} else {
List _emptyList = Collections.emptyList();
_elvis = _emptyList;
}
return _elvis;
}
public , V extends Object> SortedMap sorted(final Map map) {
if ((map instanceof SortedMap, ?>)) {
return ((SortedMap) map);
}
final TreeMap result = Maps.newTreeMap();
if ((map != null)) {
result.putAll(map);
}
return result;
}
public SortedMap sortedWith(final Map map, final Comparator comparator) {
if ((map instanceof SortedMap, ?>)) {
return ((SortedMap) map);
}
final TreeMap result = Maps.newTreeMap(comparator);
if ((map != null)) {
result.putAll(map);
}
return result;
}
public Iterable andAlso(final Iterable list, final Iterable another) {
if (((list == null) || IterableExtensions.isEmpty(list))) {
return this.safe(another);
}
if (((another == null) || IterableExtensions.isEmpty(another))) {
return list;
}
final LinkedList result = new LinkedList();
Iterables.addAll(result, list);
Iterables.addAll(result, another);
return result;
}
public String joinedMap(final Iterable extends T> list, final String separator, final Function2 op) {
String _xblockexpression = null;
{
if (((list == null) || IterableExtensions.isEmpty(list))) {
return "";
}
final int[] idxRef = { 0 };
final Function1 _function = (T it) -> {
String _xblockexpression_1 = null;
{
final int idx = idxRef[0];
idxRef[0] = (idx + 1);
_xblockexpression_1 = op.apply(it, Integer.valueOf(idx));
}
return _xblockexpression_1;
};
_xblockexpression = IterableExtensions.join(IterableExtensions.map(list, _function), separator);
}
return _xblockexpression;
}
public Iterable excludeType(final Iterable list, final Class> excluded) {
final Function1 _function = (T it) -> {
boolean _isInstance = excluded.isInstance(it);
return Boolean.valueOf((!_isInstance));
};
return IterableExtensions.filter(list, _function);
}
public Iterable excludeTypes(final Iterable list, final Class>... excluded) {
final Function2, Class>, Iterable> _function = (Iterable l, Class> c) -> {
return this.excludeType(l, c);
};
return IterableExtensions., Iterable>fold(((Iterable>)Conversions.doWrapArray(excluded)), list, _function);
}
public String spaceIfNeeded(final String prefix, final String text) {
String _xifexpression = null;
boolean _isNullOrEmpty = StringExtensions.isNullOrEmpty(prefix);
if (_isNullOrEmpty) {
_xifexpression = text;
} else {
_xifexpression = ((prefix + " ") + text);
}
return _xifexpression;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy