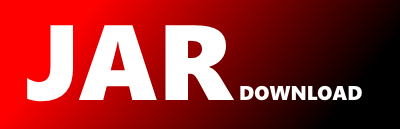
com.reprezen.genflow.openapi3.doc.ArrayHelper Maven / Gradle / Ivy
package com.reprezen.genflow.openapi3.doc;
import com.google.common.base.Objects;
import com.google.common.collect.Lists;
import com.reprezen.genflow.openapi3.doc.AttributeHelper;
import com.reprezen.genflow.openapi3.doc.BadArrayException;
import com.reprezen.genflow.openapi3.doc.Helper;
import com.reprezen.genflow.openapi3.doc.HelperHelper;
import com.reprezen.genflow.openapi3.doc.KaiZenParserHelper;
import com.reprezen.kaizen.oasparser.model3.Schema;
import java.util.ArrayList;
import java.util.Collections;
import org.eclipse.xtend2.lib.StringConcatenation;
import org.eclipse.xtext.xbase.lib.CollectionLiterals;
import org.eclipse.xtext.xbase.lib.Exceptions;
import org.eclipse.xtext.xbase.lib.Extension;
import org.eclipse.xtext.xbase.lib.Functions.Function1;
import org.eclipse.xtext.xbase.lib.IterableExtensions;
import org.eclipse.xtext.xbase.lib.ListExtensions;
@SuppressWarnings("all")
public class ArrayHelper implements Helper {
@Extension
private AttributeHelper attributeHelper;
@Extension
private KaiZenParserHelper _kaiZenParserHelper = new KaiZenParserHelper();
@Override
public void init() {
this.attributeHelper = HelperHelper.getAttributeHelper();
}
public Schema getElementType(final Schema obj) {
return IterableExtensions.last(this.collectItemTypes(obj, true));
}
public String getArrayTypeSpec(final Schema obj) {
StringConcatenation _builder = new StringConcatenation();
String _elementTypeName = this.getElementTypeName(obj);
_builder.append(_elementTypeName);
String _arrayShape = this.getArrayShape(obj);
_builder.append(_arrayShape);
final String result = _builder.toString();
return result;
}
public String getElementTypeName(final Schema obj) {
String _xblockexpression = null;
{
final Schema elementType = this.getElementType(obj);
String _kaiZenSchemaName = null;
if (elementType!=null) {
_kaiZenSchemaName=this._kaiZenParserHelper.getKaiZenSchemaName(elementType);
}
String _type = null;
if (elementType!=null) {
_type=elementType.getType();
}
String _rzveTypeName = this.attributeHelper.getRzveTypeName(elementType);
final Function1 _function = (String it) -> {
return Boolean.valueOf((it != null));
};
_xblockexpression = IterableExtensions.head(IterableExtensions.filter(Collections.unmodifiableList(CollectionLiterals.newArrayList(_kaiZenSchemaName, _type, _rzveTypeName)), _function));
}
return _xblockexpression;
}
public ArrayList collectItemTypes(final Schema obj, final boolean includeFinal) {
try {
ArrayList _xblockexpression = null;
{
final ArrayList result = Lists.newArrayList();
Schema current = obj;
while (Objects.equal(current.getType(), "array")) {
{
result.add(current);
final Schema item = current.getItemsSchema();
if ((item == null)) {
throw new BadArrayException("Array has no items type");
} else {
boolean _contains = result.contains(item);
if (_contains) {
throw new BadArrayException("Array is (or is nested within) its own element type");
} else {
current = item;
}
}
}
}
if (includeFinal) {
result.add(current);
}
_xblockexpression = result;
}
return _xblockexpression;
} catch (Throwable _e) {
throw Exceptions.sneakyThrow(_e);
}
}
private String getArrayShape(final Schema obj) {
final Function1 _function = (Schema it) -> {
return this.getArrayBounds(it);
};
return IterableExtensions.join(ListExtensions.map(this.collectItemTypes(obj, false), _function));
}
private String getArrayBounds(final Schema obj) {
String _xblockexpression = null;
{
final Integer max = obj.getMaxItems();
final Integer min = obj.getMinItems();
String _switchResult = null;
final Object _switchValue = null;
boolean _matched = false;
if (((min == null) && (max == null))) {
_matched=true;
_switchResult = "[*]";
}
if (!_matched) {
if ((((min).intValue() == 0) && ((max).intValue() == 1))) {
_matched=true;
_switchResult = "[?]";
}
}
if (!_matched) {
if ((((min).intValue() == 1) && (max == null))) {
_matched=true;
_switchResult = "[+]";
}
}
if (!_matched) {
if (((min != null) && (max == null))) {
_matched=true;
StringConcatenation _builder = new StringConcatenation();
_builder.append("[");
_builder.append(min);
_builder.append("+]");
_switchResult = _builder.toString();
}
}
if (!_matched) {
if (((min == null) && (max != null))) {
_matched=true;
StringConcatenation _builder_1 = new StringConcatenation();
_builder_1.append("[..");
_builder_1.append(max);
_builder_1.append("]");
_switchResult = _builder_1.toString();
}
}
if (!_matched) {
boolean _equals = Objects.equal(min, max);
if (_equals) {
_matched=true;
StringConcatenation _builder_2 = new StringConcatenation();
_builder_2.append("[");
_builder_2.append(min);
_builder_2.append("]");
_switchResult = _builder_2.toString();
}
}
if (!_matched) {
StringConcatenation _builder_3 = new StringConcatenation();
_builder_3.append(min);
_builder_3.append("..");
_builder_3.append(max);
_switchResult = _builder_3.toString();
}
_xblockexpression = _switchResult;
}
return _xblockexpression;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy