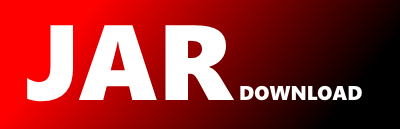
com.reprezen.genflow.openapi3.doc.AttrDetails Maven / Gradle / Ivy
package com.reprezen.genflow.openapi3.doc;
import com.google.common.base.Strings;
import com.reprezen.genflow.openapi3.doc.HelperHelper;
import com.reprezen.genflow.openapi3.doc.HtmlHelper;
import com.reprezen.genflow.openapi3.doc.KaiZenParserHelper;
import com.reprezen.kaizen.oasparser.model3.Schema;
import java.util.List;
import org.eclipse.xtend2.lib.StringConcatenation;
import org.eclipse.xtext.xbase.lib.Extension;
import org.eclipse.xtext.xbase.lib.Functions.Function1;
import org.eclipse.xtext.xbase.lib.IterableExtensions;
import org.eclipse.xtext.xbase.lib.ListExtensions;
@SuppressWarnings("all")
public class AttrDetails {
@Extension
private HtmlHelper _htmlHelper = HelperHelper.getHtmlHelper();
@Extension
private KaiZenParserHelper _kaiZenParserHelper = new KaiZenParserHelper();
private final Schema obj;
private final String id;
public AttrDetails(final Schema obj) {
this.obj = obj;
this.id = this._htmlHelper.getHtmlId(this);
}
public CharSequence details(final boolean topLevel) {
CharSequence _xblockexpression = null;
{
final String details = this.getDetailRows();
CharSequence _xifexpression = null;
boolean _isEmpty = details.isEmpty();
boolean _not = (!_isEmpty);
if (_not) {
CharSequence _xblockexpression_1 = null;
{
final CharSequence detailsTable = this.detailsTable(details);
CharSequence _xifexpression_1 = null;
if (topLevel) {
StringConcatenation _builder = new StringConcatenation();
_builder.append("");
_builder.append(detailsTable);
_builder.append("
");
_xifexpression_1 = _builder;
} else {
_xifexpression_1 = detailsTable;
}
_xblockexpression_1 = _xifexpression_1;
}
_xifexpression = _xblockexpression_1;
}
_xblockexpression = _xifexpression;
}
return _xblockexpression;
}
private CharSequence detailsTable(final String rows) {
StringConcatenation _builder = new StringConcatenation();
_builder.append("");
_builder.newLineIfNotEmpty();
_builder.append(" ");
_builder.append("");
_builder.append(rows, " ");
_builder.append("
");
_builder.newLineIfNotEmpty();
_builder.append("");
_builder.newLine();
return _builder;
}
public CharSequence getInfoButton() {
CharSequence _xifexpression = null;
boolean _isEmpty = this.getDetailRows().isEmpty();
boolean _not = (!_isEmpty);
if (_not) {
_xifexpression = this.getInfoButton(this.id);
}
return _xifexpression;
}
private CharSequence getInfoButton(final String id) {
StringConcatenation _builder = new StringConcatenation();
_builder.append("");
_builder.newLineIfNotEmpty();
_builder.append(" ");
_builder.append("");
_builder.newLine();
_builder.append(" ");
_builder.append("");
_builder.newLine();
_builder.append("");
_builder.newLine();
return _builder;
}
private String getDetailRows() {
StringConcatenation _builder = new StringConcatenation();
CharSequence _detailRow = this.detailRow("Format");
_builder.append(_detailRow);
_builder.newLineIfNotEmpty();
CharSequence _detailRow_1 = this.detailRow("Default");
_builder.append(_detailRow_1);
_builder.newLineIfNotEmpty();
CharSequence _detailRow_2 = this.detailRow("Bounds");
_builder.append(_detailRow_2);
_builder.newLineIfNotEmpty();
CharSequence _detailRow_3 = this.detailRow("Multiple Of");
_builder.append(_detailRow_3);
_builder.newLineIfNotEmpty();
CharSequence _detailRow_4 = this.detailRow("Length");
_builder.append(_detailRow_4);
_builder.newLineIfNotEmpty();
CharSequence _detailRow_5 = this.detailRow("Pattern");
_builder.append(_detailRow_5);
_builder.newLineIfNotEmpty();
CharSequence _detailRow_6 = this.detailRow("Allowed Values");
_builder.append(_detailRow_6);
_builder.newLineIfNotEmpty();
CharSequence _detailRow_7 = this.detailRow("Unique Values");
_builder.append(_detailRow_7);
_builder.newLineIfNotEmpty();
CharSequence _detailRow_8 = this.detailRow("Example");
_builder.append(_detailRow_8);
_builder.newLineIfNotEmpty();
return _builder.toString();
}
private CharSequence detailRow(final String detailName) {
CharSequence _xblockexpression = null;
{
String _switchResult = null;
if (detailName != null) {
switch (detailName) {
case "Format":
_switchResult = this.obj.getFormat();
break;
case "Default":
Object _default = this.obj.getDefault();
String _string = null;
if (_default!=null) {
_string=_default.toString();
}
_switchResult = _string;
break;
case "Bounds":
_switchResult = this.getBounds();
break;
case "Multiple Of":
Number _multipleOf = this.obj.getMultipleOf();
String _string_1 = null;
if (_multipleOf!=null) {
_string_1=_multipleOf.toString();
}
_switchResult = _string_1;
break;
case "Length":
_switchResult = this.getLengthBounds();
break;
case "Pattern":
String _pattern = this.obj.getPattern();
String _htmlEscape = null;
if (_pattern!=null) {
_htmlEscape=this._htmlHelper.htmlEscape(_pattern);
}
_switchResult = _htmlEscape;
break;
case "Allowed Values":
_switchResult = this.getEnumList();
break;
case "Unique Values":
Boolean _uniqueItems = this.obj.getUniqueItems();
String _string_2 = null;
if (_uniqueItems!=null) {
_string_2=_uniqueItems.toString();
}
_switchResult = _string_2;
break;
case "Example":
Object _example = this.obj.getExample();
String _string_3 = null;
if (_example!=null) {
_string_3=_example.toString();
}
_switchResult = _string_3;
break;
default:
throw new IllegalArgumentException(("Internal error - unexpected detail label: " + detailName));
}
} else {
throw new IllegalArgumentException(("Internal error - unexpected detail label: " + detailName));
}
final String value = _switchResult;
CharSequence _xifexpression = null;
boolean _isNullOrEmpty = Strings.isNullOrEmpty(value);
boolean _not = (!_isNullOrEmpty);
if (_not) {
StringConcatenation _builder = new StringConcatenation();
_builder.append("");
_builder.append(detailName);
_builder.append(" ");
_builder.append(value);
_builder.append(" ");
_xifexpression = _builder;
}
_xblockexpression = _xifexpression;
}
return _xblockexpression;
}
private String getBounds() {
String _xblockexpression = null;
{
String _xifexpression = null;
if (((this.obj.getExclusiveMinimum() != null) && (!(this.obj.getExclusiveMinimum()).booleanValue()))) {
_xifexpression = "=";
}
final String minEq = _xifexpression;
String _xifexpression_1 = null;
if (((this.obj.getExclusiveMaximum() != null) && (!(this.obj.getExclusiveMaximum()).booleanValue()))) {
_xifexpression_1 = "=";
}
final String maxEq = _xifexpression_1;
String _xifexpression_2 = null;
Number _minimum = this.obj.getMinimum();
boolean _tripleNotEquals = (_minimum != null);
if (_tripleNotEquals) {
String _xifexpression_3 = null;
Number _maximum = this.obj.getMaximum();
boolean _tripleNotEquals_1 = (_maximum != null);
if (_tripleNotEquals_1) {
StringConcatenation _builder = new StringConcatenation();
Number _minimum_1 = this.obj.getMinimum();
_builder.append(_minimum_1);
_builder.append(" <");
_builder.append(minEq);
_builder.append(" ");
String _title = this.obj.getTitle();
_builder.append(_title);
_builder.append(" <");
_builder.append(maxEq);
_builder.append(" ");
Number _maximum_1 = this.obj.getMaximum();
_builder.append(_maximum_1);
_xifexpression_3 = _builder.toString();
}
_xifexpression_2 = _xifexpression_3;
} else {
String _xifexpression_4 = null;
Number _minimum_2 = this.obj.getMinimum();
boolean _tripleNotEquals_2 = (_minimum_2 != null);
if (_tripleNotEquals_2) {
StringConcatenation _builder_1 = new StringConcatenation();
String _title_1 = this.obj.getTitle();
_builder_1.append(_title_1);
_builder_1.append(" >");
_builder_1.append(minEq);
_builder_1.append(" ");
Number _minimum_3 = this.obj.getMinimum();
_builder_1.append(_minimum_3);
_xifexpression_4 = _builder_1.toString();
} else {
String _xifexpression_5 = null;
Number _maximum_2 = this.obj.getMaximum();
boolean _tripleNotEquals_3 = (_maximum_2 != null);
if (_tripleNotEquals_3) {
StringConcatenation _builder_2 = new StringConcatenation();
String _title_2 = this.obj.getTitle();
_builder_2.append(_title_2);
_builder_2.append(" <");
_builder_2.append(maxEq);
_builder_2.append(" ");
Number _maximum_3 = this.obj.getMaximum();
_builder_2.append(_maximum_3);
_xifexpression_5 = _builder_2.toString();
}
_xifexpression_4 = _xifexpression_5;
}
_xifexpression_2 = _xifexpression_4;
}
final String bounds = _xifexpression_2;
String _htmlEscape = null;
if (bounds!=null) {
_htmlEscape=this._htmlHelper.htmlEscape(bounds);
}
_xblockexpression = _htmlEscape;
}
return _xblockexpression;
}
private String getLengthBounds() {
String _xifexpression = null;
if ((((this.obj.getMinLength() != null) && ((this.obj.getMinLength()).intValue() > 0)) && ((this.obj.getMaxLength() != null) && ((this.obj.getMaxLength()).intValue() < Integer.MAX_VALUE)))) {
StringConcatenation _builder = new StringConcatenation();
Integer _minLength = this.obj.getMinLength();
_builder.append(_minLength);
_builder.append(" <= length <= ");
Integer _maxLength = this.obj.getMaxLength();
_builder.append(_maxLength);
_xifexpression = _builder.toString();
} else {
String _xifexpression_1 = null;
if (((this.obj.getMinLength() != null) && ((this.obj.getMinLength()).intValue() > 0))) {
StringConcatenation _builder_1 = new StringConcatenation();
_builder_1.append("length >= ");
Integer _minLength_1 = this.obj.getMinLength();
_builder_1.append(_minLength_1);
_xifexpression_1 = _builder_1.toString();
} else {
String _xifexpression_2 = null;
if (((this.obj.getMaxLength() != null) && ((this.obj.getMaxLength()).intValue() < Integer.MAX_VALUE))) {
StringConcatenation _builder_2 = new StringConcatenation();
_builder_2.append("length <= ");
Integer _maxLength_1 = this.obj.getMaxLength();
_builder_2.append(_maxLength_1);
_xifexpression_2 = _builder_2.toString();
}
_xifexpression_1 = _xifexpression_2;
}
_xifexpression = _xifexpression_1;
}
return _xifexpression;
}
private String getEnumList() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy