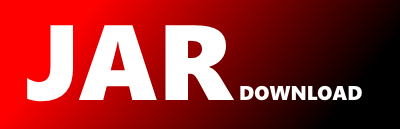
com.reprezen.genflow.openapi3.doc.AttributeHelper Maven / Gradle / Ivy
package com.reprezen.genflow.openapi3.doc;
import com.reprezen.genflow.api.normal.openapi.RepreZenVendorExtension;
import com.reprezen.genflow.openapi3.doc.Helper;
import com.reprezen.genflow.openapi3.doc.HelperHelper;
import com.reprezen.genflow.openapi3.doc.ModelHelper;
import com.reprezen.genflow.openapi3.doc.ParameterHelper;
import com.reprezen.jsonoverlay.JsonOverlay;
import com.reprezen.jsonoverlay.Overlay;
import com.reprezen.kaizen.oasparser.model3.Parameter;
import com.reprezen.kaizen.oasparser.model3.Schema;
import java.util.Arrays;
import java.util.List;
@SuppressWarnings("all")
public class AttributeHelper implements Helper {
private ParameterHelper parameterHelper;
private ModelHelper modelHelper;
@Override
public void init() {
this.parameterHelper = HelperHelper.getParameterHelper();
this.modelHelper = HelperHelper.getModelHelper();
}
protected Object _getAttribute(final Object obj, final String attr) {
return null;
}
protected Object _getAttribute(final Parameter param, final String attr) {
return this.parameterHelper.getAttribute(param, attr);
}
protected Object _getAttribute(final Schema model, final String attr) {
return this.modelHelper.getAttribute(model, attr);
}
/**
* Convenience method for specific attributes
*/
public String getName(final Object obj) {
Object _attribute = this.getAttribute(obj, "name");
return ((String) _attribute);
}
public Object getDefaultValue(final Object obj) {
return this.getAttribute(obj, "defaultValue");
}
public String getTitle(final Object obj) {
Object _attribute = this.getAttribute(obj, "title");
return ((String) _attribute);
}
public RepreZenVendorExtension getRZVE(final Object obj) {
RepreZenVendorExtension _xifexpression = null;
if ((obj instanceof JsonOverlay>)) {
_xifexpression = RepreZenVendorExtension.get(Overlay.of(((JsonOverlay>)obj)).toJson());
} else {
_xifexpression = RepreZenVendorExtension.get(obj);
}
return _xifexpression;
}
public String getRzveTypeName(final Object obj) {
RepreZenVendorExtension _rZVE = this.getRZVE(obj);
String _typeName = null;
if (_rZVE!=null) {
_typeName=_rZVE.getTypeName();
}
return _typeName;
}
public String getMinimum(final Object obj) {
Object _attribute = this.getAttribute(obj, "minimum");
String _string = null;
if (_attribute!=null) {
_string=_attribute.toString();
}
return _string;
}
public String getMaximum(final Object obj) {
Object _attribute = this.getAttribute(obj, "maximum");
String _string = null;
if (_attribute!=null) {
_string=_attribute.toString();
}
return _string;
}
public Boolean getExclusiveMinimum(final Object obj) {
Object _attribute = this.getAttribute(obj, "exclusiveMinimum");
return Boolean.valueOf(((String) _attribute));
}
public Boolean getExclusiveMaximum(final Object obj) {
Object _attribute = this.getAttribute(obj, "exclusiveMaximum");
return Boolean.valueOf(((String) _attribute));
}
public Double getMultipleOf(final Object obj) {
return ((Double) null);
}
public Integer getMinLength(final Object obj) {
Integer _elvis = null;
Object _attribute = this.getAttribute(obj, "minLength");
if (((Integer) _attribute) != null) {
_elvis = ((Integer) _attribute);
} else {
_elvis = Integer.valueOf(0);
}
return _elvis;
}
public Integer getMaxLength(final Object obj) {
Integer _elvis = null;
Object _attribute = this.getAttribute(obj, "maxLength");
if (((Integer) _attribute) != null) {
_elvis = ((Integer) _attribute);
} else {
_elvis = Integer.valueOf(Integer.MAX_VALUE);
}
return _elvis;
}
public String getPattern(final Object obj) {
Object _attribute = this.getAttribute(obj, "pattern");
return ((String) _attribute);
}
public List> getEnums(final Object obj) {
Object _attribute = this.getAttribute(obj, "enum");
return ((List>) _attribute);
}
public Boolean getUniqueItems(final Object obj) {
return ((Boolean) null);
}
public String getExample(final Object obj) {
Object _attribute = this.getAttribute(obj, "x-example");
String _string = null;
if (_attribute!=null) {
_string=_attribute.toString();
}
return _string;
}
public Object getAttribute(final Object param, final String attr) {
if (param instanceof Parameter) {
return _getAttribute((Parameter)param, attr);
} else if (param instanceof Schema) {
return _getAttribute((Schema)param, attr);
} else if (param != null) {
return _getAttribute(param, attr);
} else {
throw new IllegalArgumentException("Unhandled parameter types: " +
Arrays.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy