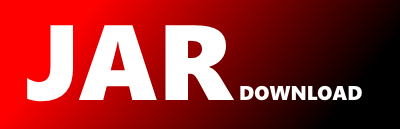
com.reprezen.genflow.openapi3.doc.ExamplesHelper Maven / Gradle / Ivy
package com.reprezen.genflow.openapi3.doc;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.dataformat.yaml.YAMLFactory;
import com.reprezen.genflow.openapi3.doc.DocHelper;
import com.reprezen.genflow.openapi3.doc.Helper;
import com.reprezen.genflow.openapi3.doc.HelperHelper;
import com.reprezen.genflow.openapi3.doc.HtmlHelper;
import com.reprezen.kaizen.oasparser.model3.Example;
import java.util.Map;
import java.util.Set;
import org.eclipse.xtend2.lib.StringConcatenation;
import org.eclipse.xtext.xbase.lib.Exceptions;
import org.eclipse.xtext.xbase.lib.Extension;
import org.eclipse.xtext.xbase.lib.Functions.Function1;
import org.eclipse.xtext.xbase.lib.IterableExtensions;
@SuppressWarnings("all")
public class ExamplesHelper implements Helper {
@Extension
private HtmlHelper htmlHelper;
@Extension
private DocHelper docHelper;
private final static ObjectMapper yamlMapper = new ObjectMapper(new YAMLFactory());
@Override
public void init() {
this.htmlHelper = HelperHelper.getHtmlHelper();
this.docHelper = HelperHelper.getDocHelper();
}
public String renderExamples(final Map examples) {
Set _keySet = null;
if (examples!=null) {
_keySet=examples.keySet();
}
Iterable _map = null;
if (_keySet!=null) {
final Function1 _function = (String name) -> {
return this.render(examples.get(name), name);
};
_map=IterableExtensions.map(_keySet, _function);
}
String _examplesSection = null;
if (_map!=null) {
_examplesSection=this.examplesSection(_map);
}
return _examplesSection;
}
public String renderExample(final Object example) {
String _exampleText = null;
if (example!=null) {
_exampleText=this.exampleText(example);
}
String _exampleSection = null;
if (_exampleText!=null) {
_exampleSection=this.exampleSection(_exampleText);
}
return _exampleSection;
}
private String render(final Example example, final String name) {
StringConcatenation _builder = new StringConcatenation();
_builder.append("");
_builder.append(name);
_builder.append(" ");
_builder.newLineIfNotEmpty();
_builder.append("");
_builder.newLine();
_builder.append("\t");
{
String _summary = example.getSummary();
boolean _tripleNotEquals = (_summary != null);
if (_tripleNotEquals) {
_builder.append("");
String _htmlEscape = this.htmlHelper.htmlEscape(example.getSummary());
_builder.append(_htmlEscape, "\t");
_builder.append("
");
}
}
_builder.newLineIfNotEmpty();
_builder.append("\t");
{
String _description = example.getDescription();
boolean _tripleNotEquals_1 = (_description != null);
if (_tripleNotEquals_1) {
String _docHtml = this.docHelper.getDocHtml(example.getDescription());
_builder.append(_docHtml, "\t");
}
}
_builder.newLineIfNotEmpty();
_builder.append("\t");
{
String _externalValue = example.getExternalValue();
boolean _tripleNotEquals_2 = (_externalValue != null);
if (_tripleNotEquals_2) {
_builder.append("External Value: ");
String _htmlEscape_1 = this.htmlHelper.htmlEscape(example.getExternalValue());
_builder.append(_htmlEscape_1, "\t");
_builder.append("
");
}
}
_builder.newLineIfNotEmpty();
_builder.append("\t");
Object _value = null;
if (example!=null) {
_value=example.getValue();
}
String _exampleText = null;
if (_value!=null) {
_exampleText=this.exampleText(_value);
}
_builder.append(_exampleText, "\t");
_builder.newLineIfNotEmpty();
_builder.append(" \t\t\t\t\t\t\t");
_builder.newLine();
return _builder.toString();
}
private String exampleText(final Object value) {
try {
String _xblockexpression = null;
{
String _switchResult = null;
boolean _matched = false;
if (value instanceof String) {
_matched=true;
_switchResult = ((String)value);
}
if (!_matched) {
_switchResult = ExamplesHelper.yamlMapper.writerWithDefaultPrettyPrinter().writeValueAsString(ExamplesHelper.yamlMapper.convertValue(value, JsonNode.class));
}
final String content = _switchResult;
StringConcatenation _builder = new StringConcatenation();
_builder.append("");
_builder.newLine();
String _htmlEscape = this.htmlHelper.htmlEscape(content);
_builder.append(_htmlEscape);
_builder.newLineIfNotEmpty();
_builder.append("
");
_xblockexpression = _builder.toString();
}
return _xblockexpression;
} catch (Throwable _e) {
throw Exceptions.sneakyThrow(_e);
}
}
private String exampleSection(final String html) {
StringConcatenation _builder = new StringConcatenation();
_builder.append("Example
");
_builder.newLine();
_builder.append(html);
_builder.newLineIfNotEmpty();
return _builder.toString();
}
private String examplesSection(final Iterable examples) {
StringConcatenation _builder = new StringConcatenation();
_builder.append("Examples
");
_builder.newLine();
_builder.append("");
_builder.newLine();
{
for(final String example : examples) {
_builder.append(example);
_builder.newLineIfNotEmpty();
}
}
_builder.append(" ");
_builder.newLine();
return _builder.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy