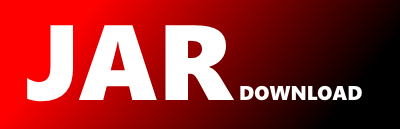
com.reprezen.genflow.openapi3.doc.OpDoc Maven / Gradle / Ivy
/**
* Copyright © 2013, 2016 Modelsolv, Inc.
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property
* of ModelSolv, Inc. See the file license.html in the root directory of
* this project for further information.
*/
package com.reprezen.genflow.openapi3.doc;
import com.google.common.base.Objects;
import com.reprezen.genflow.openapi3.doc.DocHelper;
import com.reprezen.genflow.openapi3.doc.ExamplesHelper;
import com.reprezen.genflow.openapi3.doc.HelperHelper;
import com.reprezen.genflow.openapi3.doc.HtmlHelper;
import com.reprezen.genflow.openapi3.doc.KaiZenParserHelper;
import com.reprezen.genflow.openapi3.doc.MiscHelper;
import com.reprezen.genflow.openapi3.doc.OptionHelper;
import com.reprezen.genflow.openapi3.doc.ParamsDoc;
import com.reprezen.genflow.openapi3.doc.PathDoc;
import com.reprezen.genflow.openapi3.doc.ResponseHelper;
import com.reprezen.genflow.openapi3.doc.SchemaHelper;
import com.reprezen.genflow.openapi3.doc.TagHelper;
import com.reprezen.kaizen.oasparser.model3.Callback;
import com.reprezen.kaizen.oasparser.model3.Example;
import com.reprezen.kaizen.oasparser.model3.Link;
import com.reprezen.kaizen.oasparser.model3.MediaType;
import com.reprezen.kaizen.oasparser.model3.OpenApi3;
import com.reprezen.kaizen.oasparser.model3.Operation;
import com.reprezen.kaizen.oasparser.model3.Parameter;
import com.reprezen.kaizen.oasparser.model3.Path;
import com.reprezen.kaizen.oasparser.model3.RequestBody;
import com.reprezen.kaizen.oasparser.model3.Response;
import com.reprezen.kaizen.oasparser.model3.Schema;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.eclipse.xtend2.lib.StringConcatenation;
import org.eclipse.xtext.xbase.lib.Exceptions;
import org.eclipse.xtext.xbase.lib.Extension;
import org.eclipse.xtext.xbase.lib.Functions.Function1;
import org.eclipse.xtext.xbase.lib.IterableExtensions;
@SuppressWarnings("all")
public class OpDoc {
private final Operation op;
@Extension
private KaiZenParserHelper _kaiZenParserHelper = new KaiZenParserHelper();
@Extension
private DocHelper _docHelper = HelperHelper.getDocHelper();
@Extension
private TagHelper _tagHelper = HelperHelper.getTagHelper();
@Extension
private SchemaHelper _schemaHelper = HelperHelper.getSchemaHelper();
@Extension
private ResponseHelper _responseHelper = HelperHelper.getResponseHelper();
@Extension
private HtmlHelper _htmlHelper = HelperHelper.getHtmlHelper();
@Extension
private MiscHelper _miscHelper = HelperHelper.getMiscHelper();
@Extension
private OptionHelper _optionHelper = HelperHelper.getOptionHelper();
@Extension
private ExamplesHelper _examplesHelper = HelperHelper.getExamplesHelper();
public OpDoc(final Operation op, final OpenApi3 model, final Path path) {
this.op = op;
}
public String getHtml(final String method) {
StringConcatenation _builder = new StringConcatenation();
_builder.append("");
_builder.newLineIfNotEmpty();
_builder.append("");
_builder.append(method);
_builder.append("");
_builder.newLineIfNotEmpty();
_builder.append("");
CharSequence _deprecate = this.deprecate(this.op.getOperationId(), Boolean.valueOf(this.op.isDeprecated()));
_builder.append(_deprecate);
_builder.append("
");
_builder.newLineIfNotEmpty();
CharSequence _tagBadges = this._tagHelper.getTagBadges(this.op);
_builder.append(_tagBadges);
_builder.newLineIfNotEmpty();
{
Boolean _isPreview = this._optionHelper.isPreview();
if ((_isPreview).booleanValue()) {
_builder.append(" ");
_builder.newLine();
_builder.append("");
_builder.newLine();
_builder.append("\t\t");
_builder.append("");
_builder.newLineIfNotEmpty();
_builder.append("");
_builder.newLine();
}
}
_builder.append("");
_builder.newLine();
_builder.append(" ");
String _docHtml = this._docHelper.getDocHtml(this.op.getSummary(), this.op.getDescription());
_builder.append(_docHtml, " ");
_builder.newLineIfNotEmpty();
_builder.append(" ");
CharSequence _paramsHtml = new ParamsDoc().paramsHtml(this.getNonBodyParameters(this.op));
_builder.append(_paramsHtml, " ");
_builder.newLineIfNotEmpty();
_builder.append(" ");
CharSequence _messageBodyHtml = this.getMessageBodyHtml(this.op);
_builder.append(_messageBodyHtml, " ");
_builder.newLineIfNotEmpty();
{
Set _keySet = this.op.getCallbacks().keySet();
for(final String callback : _keySet) {
_builder.append(" ");
CharSequence _callbackHtml = this.getCallbackHtml(this.op.getCallbacks().get(callback), callback);
_builder.append(_callbackHtml, " ");
_builder.newLineIfNotEmpty();
}
}
_builder.append("
");
_builder.newLine();
return _builder.toString();
}
public List getNonBodyParameters(final Operation op) {
List _parameters = op.getParameters();
Iterable _filter = null;
if (_parameters!=null) {
final Function1 _function = (Parameter it) -> {
String _in = it.getIn();
return Boolean.valueOf((!Objects.equal(_in, "body")));
};
_filter=IterableExtensions.filter(_parameters, _function);
}
return IterableExtensions.toList(_filter);
}
public CharSequence getMessageBodyHtml(final Operation op) {
StringConcatenation _builder = new StringConcatenation();
RequestBody _asNullIfMissing = this._kaiZenParserHelper.asNullIfMissing(op.getRequestBody());
CharSequence _requestBodyHtml = null;
if (_asNullIfMissing!=null) {
_requestBodyHtml=this.getRequestBodyHtml(_asNullIfMissing);
}
_builder.append(_requestBodyHtml);
_builder.newLineIfNotEmpty();
{
List _sortByPosition = this._miscHelper.sortByPosition(op.getResponses().keySet(), op.getResponses());
for(final String code : _sortByPosition) {
CharSequence _responseHtml = this.getResponseHtml(op.getResponses().get(code), code);
_builder.append(_responseHtml);
}
}
_builder.newLineIfNotEmpty();
return _builder;
}
public CharSequence getRequestBodyHtml(final RequestBody bodyParam) {
StringConcatenation _builder = new StringConcatenation();
_builder.append("");
_builder.newLine();
{
Set _keySet = bodyParam.getContentMediaTypes().keySet();
for(final String contentType : _keySet) {
_builder.append(" ");
_builder.append("Request ");
_builder.append(contentType, " ");
_builder.append("");
String _chevron = this.chevron();
_builder.append(_chevron, " ");
Schema _schema = bodyParam.getContentMediaTypes().get(contentType).getSchema();
String _schemaTitle = null;
if (_schema!=null) {
_schemaTitle=this._schemaHelper.getSchemaTitle(_schema);
}
_builder.append(_schemaTitle, " ");
_builder.newLineIfNotEmpty();
_builder.append(" ");
CharSequence _mediaTypeHtml = this.getMediaTypeHtml(contentType, bodyParam.getContentMediaTypes().get(contentType));
_builder.append(_mediaTypeHtml, " ");
_builder.newLineIfNotEmpty();
}
}
_builder.append(" ");
_builder.newLine();
return _builder;
}
public CharSequence getMediaTypeHtml(final String name, final MediaType mediaType) {
StringConcatenation _builder = new StringConcatenation();
Schema _schema = mediaType.getSchema();
String _description = null;
if (_schema!=null) {
_description=_schema.getDescription();
}
String _docHtml = null;
if (_description!=null) {
_docHtml=this._docHelper.getDocHtml(_description);
}
_builder.append(_docHtml);
_builder.newLineIfNotEmpty();
_builder.append(" ");
Schema _schema_1 = mediaType.getSchema();
CharSequence _renderSchema = null;
if (_schema_1!=null) {
_renderSchema=this._schemaHelper.renderSchema(_schema_1);
}
_builder.append(_renderSchema, " ");
_builder.newLineIfNotEmpty();
_builder.append(" ");
String _renderExamples = this._examplesHelper.renderExamples(mediaType.getExamples());
_builder.append(_renderExamples, " ");
_builder.newLineIfNotEmpty();
return _builder;
}
public CharSequence getResponseHtml(final Response response, final String status) {
StringConcatenation _builder = new StringConcatenation();
_builder.append("");
_builder.newLine();
_builder.append("\t");
_builder.append("Response ");
CharSequence _statusLabel = this.statusLabel(status);
_builder.append(_statusLabel, "\t");
_builder.append("
");
_builder.newLineIfNotEmpty();
_builder.append("\t");
String _description = response.getDescription();
String _docHtml = null;
if (_description!=null) {
_docHtml=this._docHelper.getDocHtml(_description);
}
_builder.append(_docHtml, "\t");
_builder.newLineIfNotEmpty();
{
Set _keySet = response.getContentMediaTypes().keySet();
for(final String contentType : _keySet) {
_builder.append("\t");
CharSequence _responseContentHtml = this.getResponseContentHtml(response, contentType, response.getContentMediaTypes().get(contentType), status);
_builder.append(_responseContentHtml, "\t");
_builder.newLineIfNotEmpty();
_builder.append("\t");
_builder.append("
");
_builder.newLine();
}
}
_builder.append("\t");
CharSequence _responseHeaders = this.getResponseHeaders(response);
_builder.append(_responseHeaders, "\t");
_builder.newLineIfNotEmpty();
_builder.append(" ");
_builder.newLine();
return _builder;
}
public CharSequence getResponseContentHtml(final Response response, final String contentType, final MediaType mediaType, final String status) {
CharSequence _xblockexpression = null;
{
Schema _schema = null;
if (mediaType!=null) {
_schema=mediaType.getSchema();
}
final Schema schema = _schema;
StringConcatenation _builder = new StringConcatenation();
String _chevron = this.chevron();
_builder.append(_chevron);
_builder.append(" ");
_builder.append(contentType);
_builder.append(" ");
_builder.newLineIfNotEmpty();
String _schemaTitle = null;
if (schema!=null) {
_schemaTitle=this._schemaHelper.getSchemaTitle(schema);
}
_builder.append(_schemaTitle);
_builder.newLineIfNotEmpty();
CharSequence _renderSchema = null;
if (schema!=null) {
_renderSchema=this._schemaHelper.renderSchema(schema);
}
_builder.append(_renderSchema);
_builder.append("\t\t\t");
_builder.newLineIfNotEmpty();
Object _example = null;
if (mediaType!=null) {
_example=mediaType.getExample();
}
String _renderExample = this._examplesHelper.renderExample(_example);
_builder.append(_renderExample);
_builder.newLineIfNotEmpty();
Map _examples = null;
if (mediaType!=null) {
_examples=mediaType.getExamples();
}
String _renderExamples = this._examplesHelper.renderExamples(_examples);
_builder.append(_renderExamples);
_builder.newLineIfNotEmpty();
CharSequence _responseLinks = this.getResponseLinks(response);
_builder.append(_responseLinks);
_builder.newLineIfNotEmpty();
_xblockexpression = _builder;
}
return _xblockexpression;
}
private CharSequence getResponseHeaders(final Response response) {
CharSequence _xblockexpression = null;
{
final CharSequence html = this._responseHelper.getHeadersHtml(response);
CharSequence _xifexpression = null;
if ((html != null)) {
StringConcatenation _builder = new StringConcatenation();
_builder.append("Headers
");
_builder.newLine();
_builder.append(html);
_builder.newLineIfNotEmpty();
_xifexpression = _builder;
}
_xblockexpression = _xifexpression;
}
return _xblockexpression;
}
public CharSequence getCallbackHtml(final Callback callback, final String name) {
StringConcatenation _builder = new StringConcatenation();
_builder.append("");
_builder.newLine();
_builder.append("\t");
_builder.append("Callback ");
_builder.append(name, "\t");
_builder.append("
");
_builder.newLineIfNotEmpty();
{
Set _keySet = callback.getCallbackPaths().keySet();
for(final String path : _keySet) {
_builder.append("\t");
Path _get = callback.getCallbackPaths().get(path);
CharSequence _html = new PathDoc(path, _get).getHtml();
_builder.append(_html, "\t");
_builder.newLineIfNotEmpty();
}
}
_builder.append(" ");
_builder.newLine();
return _builder;
}
public CharSequence getResponseLinks(final Response response) {
CharSequence _xifexpression = null;
boolean _isEmpty = response.getLinks().isEmpty();
if (_isEmpty) {
StringConcatenation _builder = new StringConcatenation();
_xifexpression = _builder;
} else {
StringConcatenation _builder_1 = new StringConcatenation();
_builder_1.append("");
_builder_1.newLine();
_builder_1.append("\t");
_builder_1.append("Links
");
_builder_1.newLine();
{
Set _keySet = response.getLinks().keySet();
for(final String link : _keySet) {
_builder_1.append("\t");
CharSequence _linkHtml = this.getLinkHtml(link, response.getLinks().get(link));
_builder_1.append(_linkHtml, "\t");
_builder_1.newLineIfNotEmpty();
}
}
_builder_1.append(" ");
_builder_1.newLine();
_xifexpression = _builder_1;
}
return _xifexpression;
}
public CharSequence getLinkHtml(final String name, final Link link) {
CharSequence _xblockexpression = null;
{
final Set parameters = link.getParameters().keySet();
StringConcatenation _builder = new StringConcatenation();
String _chevron = this.chevron();
_builder.append(_chevron);
_builder.append(" ");
_builder.append(name);
_builder.append(" ");
String _operationId = link.getOperationId();
_builder.append(_operationId);
_builder.append("
");
_builder.newLineIfNotEmpty();
_builder.append(" ");
_builder.newLine();
_builder.append("");
String _description = link.getDescription();
_builder.append(_description);
_builder.append("
");
_builder.newLineIfNotEmpty();
_builder.append("Parameters
");
_builder.newLine();
_builder.append("\t\t\t\t\t");
_builder.newLine();
_builder.append("\t");
_builder.append("Name Value ");
_builder.newLine();
{
for(final String parameter : parameters) {
_builder.append("\t");
_builder.append("");
_builder.append(parameter, "\t");
_builder.append(" ");
String _get = link.getParameters().get(parameter);
_builder.append(_get, "\t");
_builder.append(" ");
_builder.newLineIfNotEmpty();
}
}
_builder.append("
");
_builder.newLine();
_xblockexpression = _builder;
}
return _xblockexpression;
}
public String chevron() {
return "";
}
public CharSequence statusLabel(final String status) {
CharSequence _xblockexpression = null;
{
String _xtrycatchfinallyexpression = null;
try {
String _switchResult = null;
int _parseInt = Integer.parseInt(status);
final int s = _parseInt;
boolean _matched = false;
if (((s >= 100) && (s < 200))) {
_matched=true;
_switchResult = "info";
}
if (!_matched) {
if (((s >= 200) && (s < 300))) {
_matched=true;
_switchResult = "success";
}
}
if (!_matched) {
if (((s >= 300) && (s < 400))) {
_matched=true;
_switchResult = "info";
}
}
if (!_matched) {
if (((s >= 400) && (s < 500))) {
_matched=true;
_switchResult = "danger";
}
}
if (!_matched) {
if (((s >= 500) && (s < 600))) {
_matched=true;
_switchResult = "danger";
}
}
if (!_matched) {
_switchResult = "default";
}
_xtrycatchfinallyexpression = _switchResult;
} catch (final Throwable _t) {
if (_t instanceof NumberFormatException) {
_xtrycatchfinallyexpression = status;
} else {
throw Exceptions.sneakyThrow(_t);
}
}
final String context = _xtrycatchfinallyexpression;
StringConcatenation _builder = new StringConcatenation();
_builder.append("");
_builder.append(status);
_builder.append("");
_xblockexpression = _builder;
}
return _xblockexpression;
}
public CharSequence deprecate(final String text, final Boolean deprecated) {
CharSequence _xifexpression = null;
if (((deprecated != null) && (deprecated).booleanValue())) {
StringConcatenation _builder = new StringConcatenation();
_builder.append("");
_builder.append(text);
_builder.append("");
_xifexpression = _builder;
} else {
_xifexpression = text;
}
return _xifexpression;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy