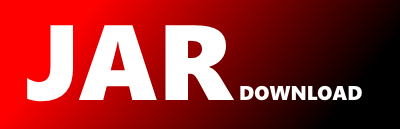
com.reprezen.genflow.openapi3.doc.PropertyHelper Maven / Gradle / Ivy
package com.reprezen.genflow.openapi3.doc;
import com.reprezen.genflow.openapi3.doc.Helper;
import io.swagger.models.properties.AbstractNumericProperty;
import io.swagger.models.properties.AbstractProperty;
import io.swagger.models.properties.ArrayProperty;
import io.swagger.models.properties.BaseIntegerProperty;
import io.swagger.models.properties.BinaryProperty;
import io.swagger.models.properties.BooleanProperty;
import io.swagger.models.properties.ByteArrayProperty;
import io.swagger.models.properties.DateProperty;
import io.swagger.models.properties.DateTimeProperty;
import io.swagger.models.properties.EmailProperty;
import io.swagger.models.properties.FileProperty;
import io.swagger.models.properties.IntegerProperty;
import io.swagger.models.properties.LongProperty;
import io.swagger.models.properties.ObjectProperty;
import io.swagger.models.properties.PasswordProperty;
import io.swagger.models.properties.Property;
import io.swagger.models.properties.StringProperty;
import io.swagger.models.properties.UUIDProperty;
import java.util.List;
@SuppressWarnings("all")
public class PropertyHelper implements Helper {
@Override
public void init() {
}
/**
* getAttribtue attempts to obtain a named attribute from a Property object.
*
* These attributes are scattered through the Property type hierarchy and so are not accessible without casting to the
* appropriate type. Here we provide a Property-level method that returns a requested property from wherever in the type
* hierarchy it is defined for the given property, or null if it is not available (or actually has the value null).
*
* getAttribute is defined as an overloaded method, and there is an implementation for every class in the Property type hierarchy.
* The overload for Property type implements dynamic dispatch based on the actual type of the supplied property and is the only
* non-private overload. Dynamic dispatch is not suitable for other overloads because they use the same overloads to delegate up the
* supertype chain to find inherited attributes.
*
* Each overload directly supports all values for which its dispatch class defines a getter; for other values, each method delegates
* to its supertype overload.
*
* Xtend's native dynamic dispatch is unsuitable for this class, though it might appear to be exactly what's needed. The problem
* is that we need, at the end of most of the overloads, to call up through the overloads for supertypes, looking for one that can
* supply the requested attribute. Were these all "dispatch" methods, the supertype calls would be impossible - all attempts to do
* so would remain stuck at the actual type of the property being interrogated, and infinite recursion would result.
*/
public Object getAttribute(final Property prop, final String attr) {
Object _switchResult = null;
boolean _matched = false;
if (prop instanceof BinaryProperty) {
_matched=true;
_switchResult = this.getAttribute(((BinaryProperty)prop), attr);
}
if (!_matched) {
if (prop instanceof BooleanProperty) {
_matched=true;
_switchResult = this.getAttribute(((BooleanProperty)prop), attr);
}
}
if (!_matched) {
if (prop instanceof ByteArrayProperty) {
_matched=true;
_switchResult = this.getAttribute(((ByteArrayProperty)prop), attr);
}
}
if (!_matched) {
if (prop instanceof DateProperty) {
_matched=true;
_switchResult = this.getAttribute(((DateProperty)prop), attr);
}
}
if (!_matched) {
if (prop instanceof DateTimeProperty) {
_matched=true;
_switchResult = this.getAttribute(((DateTimeProperty)prop), attr);
}
}
if (!_matched) {
if (prop instanceof EmailProperty) {
_matched=true;
_switchResult = this.getAttribute(((EmailProperty)prop), attr);
}
}
if (!_matched) {
if (prop instanceof FileProperty) {
_matched=true;
_switchResult = this.getAttribute(((FileProperty)prop), attr);
}
}
if (!_matched) {
if (prop instanceof IntegerProperty) {
_matched=true;
_switchResult = this.getAttribute(((IntegerProperty)prop), attr);
}
}
if (!_matched) {
if (prop instanceof LongProperty) {
_matched=true;
_switchResult = this.getAttribute(((LongProperty)prop), attr);
}
}
if (!_matched) {
if (prop instanceof PasswordProperty) {
_matched=true;
_switchResult = this.getAttribute(((PasswordProperty)prop), attr);
}
}
if (!_matched) {
if (prop instanceof UUIDProperty) {
_matched=true;
_switchResult = this.getAttribute(((UUIDProperty)prop), attr);
}
}
if (!_matched) {
if (prop instanceof BaseIntegerProperty) {
_matched=true;
_switchResult = this.getAttribute(((BaseIntegerProperty)prop), attr);
}
}
if (!_matched) {
if (prop instanceof StringProperty) {
_matched=true;
_switchResult = this.getAttribute(((StringProperty)prop), attr);
}
}
if (!_matched) {
if (prop instanceof AbstractNumericProperty) {
_matched=true;
_switchResult = this.getAttribute(((AbstractNumericProperty)prop), attr);
}
}
if (!_matched) {
if (prop instanceof ArrayProperty) {
_matched=true;
_switchResult = this.getAttribute(((ArrayProperty)prop), attr);
}
}
if (!_matched) {
if (prop instanceof ObjectProperty) {
_matched=true;
_switchResult = this.getAttribute(((ObjectProperty)prop), attr);
}
}
if (!_matched) {
if (prop instanceof AbstractProperty) {
_matched=true;
_switchResult = this.getAttribute(((AbstractProperty)prop), attr);
}
}
if (!_matched) {
throw new IllegalArgumentException("Unhandled Property type");
}
return _switchResult;
}
private Object getAttribute(final AbstractProperty prop, final String attr) {
Object _xblockexpression = null;
{
Object _switchResult = null;
if (attr != null) {
switch (attr) {
case "description":
_switchResult = prop.getDescription();
break;
case "example":
_switchResult = prop.getExample();
break;
case "format":
_switchResult = prop.getFormat();
break;
case "name":
_switchResult = prop.getName();
break;
case "position":
_switchResult = prop.getPosition();
break;
case "readOnly":
_switchResult = prop.getReadOnly();
break;
case "required":
_switchResult = Boolean.valueOf(prop.getRequired());
break;
case "title":
_switchResult = prop.getTitle();
break;
case "type":
_switchResult = prop.getType();
break;
case "xml":
_switchResult = prop.getXml();
break;
}
}
final Object value = _switchResult;
_xblockexpression = value;
}
return _xblockexpression;
}
private Object getAttribute(final ArrayProperty prop, final String attr) {
Object _xblockexpression = null;
{
Object _switchResult = null;
if (attr != null) {
switch (attr) {
case "items":
_switchResult = prop.getItems();
break;
case "minItems":
_switchResult = prop.getMinItems();
break;
case "maxItems":
_switchResult = prop.getMaxItems();
break;
case "uniqueItems":
_switchResult = prop.getUniqueItems();
break;
}
}
final Object value = _switchResult;
Object _elvis = null;
if (value != null) {
_elvis = value;
} else {
Object _attribute = this.getAttribute(((AbstractProperty) prop), attr);
_elvis = _attribute;
}
_xblockexpression = _elvis;
}
return _xblockexpression;
}
private Object getAttribute(final ObjectProperty prop, final String attr) {
Object _xblockexpression = null;
{
Object _switchResult = null;
if (attr != null) {
switch (attr) {
case "properties":
_switchResult = prop.getProperties();
break;
case "requiredProperties":
_switchResult = prop.getRequiredProperties();
break;
}
}
final Object value = _switchResult;
Object _elvis = null;
if (value != null) {
_elvis = value;
} else {
Object _attribute = this.getAttribute(((AbstractProperty) prop), attr);
_elvis = _attribute;
}
_xblockexpression = _elvis;
}
return _xblockexpression;
}
private Object getAttribute(final AbstractNumericProperty prop, final String attr) {
Object _xblockexpression = null;
{
Object _switchResult = null;
if (attr != null) {
switch (attr) {
case "minimum":
_switchResult = prop.getMinimum();
break;
case "maximum":
_switchResult = prop.getMaximum();
break;
case "exclusiveMinimum":
_switchResult = prop.getExclusiveMinimum();
break;
case "exclusiveMaximum":
_switchResult = prop.getExclusiveMaximum();
break;
}
}
final Object value = ((Object)_switchResult);
Object _elvis = null;
if (value != null) {
_elvis = value;
} else {
Object _attribute = this.getAttribute(((AbstractProperty) prop), attr);
_elvis = _attribute;
}
_xblockexpression = _elvis;
}
return _xblockexpression;
}
private Object getAttribute(final BaseIntegerProperty prop, final String attr) {
return this.getAttribute(((AbstractNumericProperty) prop), attr);
}
private Object getAttribute(final IntegerProperty prop, final String attr) {
Object _xblockexpression = null;
{
Object _switchResult = null;
if (attr != null) {
switch (attr) {
case "default":
_switchResult = prop.getDefault();
break;
case "enum":
_switchResult = prop.getEnum();
break;
}
}
final Object value = _switchResult;
Object _elvis = null;
if (value != null) {
_elvis = value;
} else {
Object _attribute = this.getAttribute(((BaseIntegerProperty) prop), attr);
_elvis = _attribute;
}
_xblockexpression = _elvis;
}
return _xblockexpression;
}
private Object getAttribute(final LongProperty prop, final String attr) {
Object _xblockexpression = null;
{
Object _switchResult = null;
if (attr != null) {
switch (attr) {
case "default":
_switchResult = prop.getDefault();
break;
case "enum":
_switchResult = prop.getEnum();
break;
}
}
final Object value = _switchResult;
Object _elvis = null;
if (value != null) {
_elvis = value;
} else {
Object _attribute = this.getAttribute(((BaseIntegerProperty) prop), attr);
_elvis = _attribute;
}
_xblockexpression = _elvis;
}
return _xblockexpression;
}
private Object getAttribute(final BinaryProperty prop, final String attr) {
Object _xblockexpression = null;
{
Object _switchResult = null;
if (attr != null) {
switch (attr) {
case "default":
_switchResult = prop.getDefault();
break;
case "enum":
_switchResult = prop.getEnum();
break;
case "minLength":
_switchResult = prop.getMinLength();
break;
case "maxLength":
_switchResult = prop.getMaxLength();
break;
case "pattern":
_switchResult = prop.getPattern();
break;
}
}
final Object value = _switchResult;
Object _elvis = null;
if (value != null) {
_elvis = value;
} else {
Object _attribute = this.getAttribute(((AbstractProperty) prop), attr);
_elvis = _attribute;
}
_xblockexpression = _elvis;
}
return _xblockexpression;
}
private Object getAttribute(final BooleanProperty prop, final String attr) {
Object _xblockexpression = null;
{
Boolean _switchResult = null;
if (attr != null) {
switch (attr) {
case "default":
_switchResult = prop.getDefault();
break;
}
}
final Boolean value = _switchResult;
Object _elvis = null;
if (value != null) {
_elvis = value;
} else {
Object _attribute = this.getAttribute(((AbstractProperty) prop), attr);
_elvis = _attribute;
}
_xblockexpression = _elvis;
}
return _xblockexpression;
}
private Object getAttribute(final ByteArrayProperty prop, final String attr) {
return this.getAttribute(((AbstractProperty) prop), attr);
}
private Object getAttribute(final DateProperty prop, final String attr) {
Object _xblockexpression = null;
{
List _switchResult = null;
if (attr != null) {
switch (attr) {
case "enum":
_switchResult = prop.getEnum();
break;
}
}
final List value = _switchResult;
Object _elvis = null;
if (value != null) {
_elvis = value;
} else {
Object _attribute = this.getAttribute(((AbstractProperty) prop), attr);
_elvis = _attribute;
}
_xblockexpression = _elvis;
}
return _xblockexpression;
}
private Object getAttribute(final DateTimeProperty prop, final String attr) {
Object _xblockexpression = null;
{
List _switchResult = null;
if (attr != null) {
switch (attr) {
case "enum":
_switchResult = prop.getEnum();
break;
}
}
final List value = _switchResult;
Object _elvis = null;
if (value != null) {
_elvis = value;
} else {
Object _attribute = this.getAttribute(((AbstractProperty) prop), attr);
_elvis = _attribute;
}
_xblockexpression = _elvis;
}
return _xblockexpression;
}
private Object getAttribute(final FileProperty prop, final String attr) {
return this.getAttribute(((AbstractProperty) prop), attr);
}
private Object getAttribute(final PasswordProperty prop, final String attr) {
Object _xblockexpression = null;
{
Object _switchResult = null;
if (attr != null) {
switch (attr) {
case "default":
_switchResult = prop.getDefault();
break;
case "enum":
_switchResult = prop.getEnum();
break;
case "minLength":
_switchResult = prop.getMinLength();
break;
case "maxLength":
_switchResult = prop.getMaxLength();
break;
case "pattern":
_switchResult = prop.getPattern();
break;
}
}
final Object value = _switchResult;
Object _elvis = null;
if (value != null) {
_elvis = value;
} else {
Object _attribute = this.getAttribute(((AbstractProperty) prop), attr);
_elvis = _attribute;
}
_xblockexpression = _elvis;
}
return _xblockexpression;
}
private Object getAttribute(final StringProperty prop, final String attr) {
Object _xblockexpression = null;
{
Object _switchResult = null;
if (attr != null) {
switch (attr) {
case "default":
_switchResult = prop.getDefault();
break;
case "enum":
_switchResult = prop.getEnum();
break;
case "minLength":
_switchResult = prop.getMinLength();
break;
case "maxLength":
_switchResult = prop.getMaxLength();
break;
case "pattern":
_switchResult = prop.getPattern();
break;
}
}
final Object value = _switchResult;
Object _elvis = null;
if (value != null) {
_elvis = value;
} else {
Object _attribute = this.getAttribute(((AbstractProperty) prop), attr);
_elvis = _attribute;
}
_xblockexpression = _elvis;
}
return _xblockexpression;
}
private Object getAttribute(final EmailProperty prop, final String attr) {
return this.getAttribute(((StringProperty) prop), attr);
}
private Object getAttribute(final UUIDProperty prop, final String attr) {
Object _xblockexpression = null;
{
Object _switchResult = null;
if (attr != null) {
switch (attr) {
case "default":
_switchResult = prop.getDefault();
break;
case "enum":
_switchResult = prop.getEnum();
break;
case "minLength":
_switchResult = prop.getMinLength();
break;
case "maxLength":
_switchResult = prop.getMaxLength();
break;
case "pattern":
_switchResult = prop.getPattern();
break;
}
}
final Object value = _switchResult;
Object _elvis = null;
if (value != null) {
_elvis = value;
} else {
Object _attribute = this.getAttribute(((AbstractProperty) prop), attr);
_elvis = _attribute;
}
_xblockexpression = _elvis;
}
return _xblockexpression;
}
}