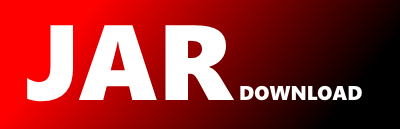
com.reprezen.genflow.openapi3.doc.SchemaHelper Maven / Gradle / Ivy
/**
* Copyright © 2013, 2016 Modelsolv, Inc.
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property
* of ModelSolv, Inc. See the file license.html in the root directory of
* this project for further information.
*/
package com.reprezen.genflow.openapi3.doc;
import com.google.common.base.Objects;
import com.reprezen.genflow.openapi3.doc.ArrayHelper;
import com.reprezen.genflow.openapi3.doc.AttrDetails;
import com.reprezen.genflow.openapi3.doc.AttributeHelper;
import com.reprezen.genflow.openapi3.doc.Helper;
import com.reprezen.genflow.openapi3.doc.HelperHelper;
import com.reprezen.genflow.openapi3.doc.KaiZenParserHelper;
import com.reprezen.genflow.openapi3.doc.StructureTable;
import com.reprezen.jsonoverlay.Overlay;
import com.reprezen.kaizen.oasparser.model3.Schema;
import java.util.Collections;
import org.eclipse.xtend2.lib.StringConcatenation;
import org.eclipse.xtext.xbase.lib.CollectionLiterals;
import org.eclipse.xtext.xbase.lib.Extension;
import org.eclipse.xtext.xbase.lib.Functions.Function1;
import org.eclipse.xtext.xbase.lib.IterableExtensions;
@SuppressWarnings("all")
public class SchemaHelper implements Helper {
@Extension
private AttributeHelper attributeHelper;
@Extension
private ArrayHelper arrayHelper;
@Extension
private KaiZenParserHelper _kaiZenParserHelper = new KaiZenParserHelper();
@Override
public void init() {
this.attributeHelper = HelperHelper.getAttributeHelper();
this.arrayHelper = HelperHelper.getArrayHelper();
}
public CharSequence renderSchema(final Schema schema) {
CharSequence _switchResult = null;
boolean _matched = false;
boolean _isPrimitive = this.isPrimitive(schema);
if (_isPrimitive) {
_matched=true;
_switchResult = this.renderPrimitiveSchema(schema);
}
if (!_matched) {
String _type = schema.getType();
boolean _equals = Objects.equal(_type, "array");
if (_equals) {
_matched=true;
_switchResult = this.renderArraySchema(schema);
}
}
if (!_matched) {
_switchResult = this.renderSchemaTable(schema);
}
return _switchResult;
}
private String renderSchemaTable(final Schema schema) {
String _xifexpression = null;
if (((schema != null) && (!IterableExtensions.isEmpty(Overlay.of(schema).toJson())))) {
String _xblockexpression = null;
{
final StructureTable.SchemaStructureTable table = new StructureTable.SchemaStructureTable(schema, new String[] { "name", "Name" }, new String[] { "type", "Type" }, new String[] { "doc", "Description" });
_xblockexpression = table.render(null);
}
_xifexpression = _xblockexpression;
}
return _xifexpression;
}
public CharSequence renderArraySchema(final Schema schema) {
CharSequence _xblockexpression = null;
{
final String typeSpec = this.arrayHelper.getArrayTypeSpec(schema);
final Schema eltType = this.arrayHelper.getElementType(schema);
final AttrDetails details = new AttrDetails(eltType);
StringConcatenation _builder = new StringConcatenation();
_builder.append("");
_builder.append(typeSpec);
_builder.append("
");
_builder.newLineIfNotEmpty();
{
boolean _isPrimitive = this.isPrimitive(eltType);
if (_isPrimitive) {
CharSequence _infoButton = details.getInfoButton();
_builder.append(_infoButton);
CharSequence _details = details.details(true);
_builder.append(_details);
} else {
String _renderSchemaTable = this.renderSchemaTable(eltType);
_builder.append(_renderSchemaTable);
}
}
_builder.newLineIfNotEmpty();
_xblockexpression = _builder;
}
return _xblockexpression;
}
public boolean isPrimitive(final Schema obj) {
return Collections.unmodifiableSet(CollectionLiterals.newHashSet("boolean", "integer", "null", "number", "string")).contains(obj.getType());
}
public CharSequence renderPrimitiveSchema(final Schema schema) {
CharSequence _xblockexpression = null;
{
final AttrDetails details = new AttrDetails(schema);
StringConcatenation _builder = new StringConcatenation();
_builder.append("");
String _type = schema.getType();
_builder.append(_type);
_builder.append("
");
CharSequence _infoButton = details.getInfoButton();
_builder.append(_infoButton);
CharSequence _details = details.details(true);
_builder.append(_details);
_xblockexpression = _builder;
}
return _xblockexpression;
}
public String getSchemaTitle(final Schema schema) {
String _kaiZenSchemaName = this._kaiZenParserHelper.getKaiZenSchemaName(schema);
String _title = schema.getTitle();
String _rzveTypeName = this.attributeHelper.getRzveTypeName(schema);
final Function1 _function = (String it) -> {
return Boolean.valueOf((it != null));
};
return IterableExtensions.last(IterableExtensions.filter(Collections.unmodifiableList(CollectionLiterals.newArrayList(_kaiZenSchemaName, _title, _rzveTypeName)), _function));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy