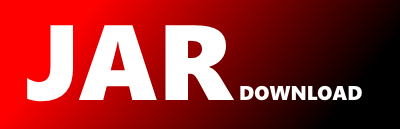
com.reprezen.genflow.openapi3.doc.StructureTable Maven / Gradle / Ivy
package com.reprezen.genflow.openapi3.doc;
import com.google.common.base.Objects;
import com.reprezen.genflow.openapi3.doc.Activation;
import com.reprezen.genflow.openapi3.doc.ArrayHelper;
import com.reprezen.genflow.openapi3.doc.AttrDetails;
import com.reprezen.genflow.openapi3.doc.AttributeHelper;
import com.reprezen.genflow.openapi3.doc.BadArrayException;
import com.reprezen.genflow.openapi3.doc.BadReferenceException;
import com.reprezen.genflow.openapi3.doc.DocHelper;
import com.reprezen.genflow.openapi3.doc.HelperHelper;
import com.reprezen.genflow.openapi3.doc.HtmlHelper;
import com.reprezen.genflow.openapi3.doc.Indentation;
import com.reprezen.genflow.openapi3.doc.KaiZenParserHelper;
import com.reprezen.genflow.openapi3.doc.OptionHelper;
import com.reprezen.genflow.openapi3.doc.RecursionHelper;
import com.reprezen.genflow.openapi3.doc.RecursiveRenderException;
import com.reprezen.genflow.openapi3.doc.RefHelper;
import com.reprezen.kaizen.oasparser.model3.Parameter;
import com.reprezen.kaizen.oasparser.model3.Schema;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.apache.commons.lang3.StringUtils;
import org.eclipse.xtend2.lib.StringConcatenation;
import org.eclipse.xtext.xbase.lib.CollectionLiterals;
import org.eclipse.xtext.xbase.lib.Conversions;
import org.eclipse.xtext.xbase.lib.Exceptions;
import org.eclipse.xtext.xbase.lib.Extension;
import org.eclipse.xtext.xbase.lib.Functions.Function1;
import org.eclipse.xtext.xbase.lib.IterableExtensions;
import org.eclipse.xtext.xbase.lib.ListExtensions;
@SuppressWarnings("all")
public abstract class StructureTable {
public static class SchemaStructureTable extends StructureTable {
public SchemaStructureTable(final Schema obj, final String[]... cols) {
super(obj, cols);
}
@Override
protected String render(final String name, final Object referrer, final Indentation ind) {
String _xblockexpression = null;
{
String _type = this.obj.getType();
boolean _equals = Objects.equal(_type, "array");
if (_equals) {
Schema _elementType = this._arrayHelper.getElementType(this.obj);
final AttrDetails det = new AttrDetails(_elementType);
Schema _elementType_1 = this._arrayHelper.getElementType(this.obj);
final StructureTable.SchemaStructureTable itemsTable = new StructureTable.SchemaStructureTable(_elementType_1, this.cols);
StringConcatenation _builder = new StringConcatenation();
String _defaultRender = this.defaultRender(name, referrer, ind, det);
_builder.append(_defaultRender);
_builder.newLineIfNotEmpty();
String _renderObject = itemsTable.renderObject(null, null, ind.advance2());
_builder.append(_renderObject);
_builder.newLineIfNotEmpty();
return _builder.toString();
}
final AttrDetails det_1 = new AttrDetails(this.obj);
Schema _xtrycatchfinallyexpression = null;
try {
Schema _additionalPropertiesSchema = this.obj.getAdditionalPropertiesSchema();
Schema _asNullIfMissing = null;
if (_additionalPropertiesSchema!=null) {
_asNullIfMissing=this._kaiZenParserHelper.asNullIfMissing(_additionalPropertiesSchema);
}
_xtrycatchfinallyexpression = _asNullIfMissing;
} catch (final Throwable _t) {
if (_t instanceof NullPointerException) {
_xtrycatchfinallyexpression = null;
} else {
throw Exceptions.sneakyThrow(_t);
}
}
final Schema additionalPropertiesSchema = _xtrycatchfinallyexpression;
final List allOfSchemas = this.obj.getAllOfSchemas();
final List oneOfSchemas = this.obj.getOneOfSchemas();
final List anyOfSchemas = this.obj.getAnyOfSchemas();
ind.use2();
ind.advance2();
String _xifexpression = null;
if ((name != null)) {
_xifexpression = this.defaultRender(name, referrer, ind, det_1);
}
final String modelRow = _xifexpression;
StringConcatenation _builder_1 = new StringConcatenation();
_builder_1.append(modelRow);
{
Set> _entrySet = this.obj.getProperties().entrySet();
for(final Map.Entry prop : _entrySet) {
Schema _value = prop.getValue();
StructureTable.SchemaStructureTable _schemaStructureTable = new StructureTable.SchemaStructureTable(_value, this.cols);
String _key = prop.getKey();
String _plus = ("!" + _key);
String _renderObject_1 = _schemaStructureTable.renderObject(_plus, this.obj, ind.advance2());
_builder_1.append(_renderObject_1);
}
}
_builder_1.newLineIfNotEmpty();
{
if ((additionalPropertiesSchema != null)) {
String _renderObject_2 = new StructureTable.SchemaStructureTable(additionalPropertiesSchema, this.cols).renderObject("![additional properties]", this.obj, ind.advance2());
_builder_1.append(_renderObject_2);
_builder_1.newLineIfNotEmpty();
}
}
{
boolean _isEmpty = allOfSchemas.isEmpty();
boolean _not = (!_isEmpty);
if (_not) {
{
for(final Schema schema : allOfSchemas) {
String _renderMemberModel = this.renderMemberModel(schema, "allOf", ind.advance2(), det_1);
_builder_1.append(_renderMemberModel);
_builder_1.newLineIfNotEmpty();
}
}
}
}
{
boolean _isEmpty_1 = oneOfSchemas.isEmpty();
boolean _not_1 = (!_isEmpty_1);
if (_not_1) {
{
for(final Schema schema_1 : oneOfSchemas) {
String _renderMemberModel_1 = this.renderMemberModel(schema_1, "oneOf", ind.advance2(), det_1);
_builder_1.append(_renderMemberModel_1);
_builder_1.newLineIfNotEmpty();
}
}
}
}
{
boolean _isEmpty_2 = anyOfSchemas.isEmpty();
boolean _not_2 = (!_isEmpty_2);
if (_not_2) {
{
for(final Schema schema_2 : anyOfSchemas) {
String _renderMemberModel_2 = this.renderMemberModel(schema_2, "anyOf", ind.advance2(), det_1);
_builder_1.append(_renderMemberModel_2);
_builder_1.newLineIfNotEmpty();
}
}
}
}
_xblockexpression = _builder_1.toString();
}
return _xblockexpression;
}
@Override
protected String getTypeSpec(final AttrDetails det) {
String _xblockexpression = null;
{
String _type = this.obj.getType();
boolean _equals = Objects.equal(_type, "array");
if (_equals) {
StringConcatenation _builder = new StringConcatenation();
String _arrayTypeSpec = this._arrayHelper.getArrayTypeSpec(this.obj);
_builder.append(_arrayTypeSpec);
CharSequence _infoButton = det.getInfoButton();
_builder.append(_infoButton);
return _builder.toString();
}
_xblockexpression = this.getTypeSpec(this.obj, det);
}
return _xblockexpression;
}
protected String getTypeSpec(final Schema model, final AttrDetails det) {
final String modelType = this.getDefaultTypeSpec(model);
StringConcatenation _builder = new StringConcatenation();
_builder.append(modelType);
CharSequence _infoButton = det.getInfoButton();
_builder.append(_infoButton);
return _builder.toString();
}
protected String renderMemberModel(final Schema memberModel, final String label, final Indentation ind, final AttrDetails det) {
StringConcatenation _builder = new StringConcatenation();
String _renderMemberRow = this.renderMemberRow(memberModel, label, ind.copy(), det);
_builder.append(_renderMemberRow);
_builder.newLineIfNotEmpty();
String _renderObject = new StructureTable.SchemaStructureTable(memberModel, this.cols).renderObject(null, null, ind.copy());
_builder.append(_renderObject);
_builder.newLineIfNotEmpty();
return _builder.toString();
}
private String renderMemberRow(final Schema member, final String label, final Indentation ind, final AttrDetails det) {
String _xifexpression = null;
Boolean _isShowComponentModels = this._optionHelper.isShowComponentModels();
if ((_isShowComponentModels).booleanValue()) {
String _xblockexpression = null;
{
StringConcatenation _builder = new StringConcatenation();
_builder.append("");
_builder.append(label);
_builder.append(": ");
String _typeSpec = this.getTypeSpec(member, det);
String _htmlEscape = null;
if (_typeSpec!=null) {
_htmlEscape=this._htmlHelper.htmlEscape(_typeSpec);
}
String _samp = null;
if (_htmlEscape!=null) {
_samp=this._htmlHelper.getSamp(_htmlEscape);
}
_builder.append(_samp);
_builder.append("");
final String text = _builder.toString();
String _indentTextToCode = this.indentTextToCode(text.toString(), ind);
_xblockexpression = this.wrapHeaderRow(Collections.unmodifiableList(CollectionLiterals.newArrayList(_indentTextToCode)));
}
_xifexpression = _xblockexpression;
}
return _xifexpression;
}
@Override
protected String getDoc() {
String _description = this.obj.getDescription();
String _docHtml = null;
if (_description!=null) {
_docHtml=this._docHelper.getDocHtml(_description);
}
return _docHtml;
}
}
public static class ParameterStructureTable extends StructureTable {
public ParameterStructureTable(final Parameter obj, final String[]... cols) {
super(obj, cols);
}
@Override
protected String render(final String name, final Object referrer, final Indentation ind) {
String _xblockexpression = null;
{
Schema _xifexpression = null;
String _type = this.obj.getSchema().getType();
boolean _equals = Objects.equal(_type, "array");
if (_equals) {
_xifexpression = this._arrayHelper.getElementType(this.obj.getSchema());
} else {
_xifexpression = this.obj.getSchema();
}
final Schema detailType = _xifexpression;
final AttrDetails det = new AttrDetails(detailType);
StringConcatenation _builder = new StringConcatenation();
String _defaultRender = this.defaultRender(name, referrer, ind, det);
_builder.append(_defaultRender);
_builder.newLineIfNotEmpty();
_xblockexpression = _builder.toString();
}
return _xblockexpression;
}
@Override
protected String getTypeSpec(final AttrDetails det) {
String _xblockexpression = null;
{
final Parameter param = this.obj;
String _xifexpression = null;
String _type = param.getSchema().getType();
boolean _equals = Objects.equal(_type, "array");
if (_equals) {
StringConcatenation _builder = new StringConcatenation();
String _arrayTypeSpec = this._arrayHelper.getArrayTypeSpec(param.getSchema());
_builder.append(_arrayTypeSpec);
CharSequence _infoButton = det.getInfoButton();
_builder.append(_infoButton);
_xifexpression = _builder.toString();
} else {
StringConcatenation _builder_1 = new StringConcatenation();
String _defaultTypeSpec = this.getDefaultTypeSpec(param.getSchema());
_builder_1.append(_defaultTypeSpec);
CharSequence _infoButton_1 = det.getInfoButton();
_builder_1.append(_infoButton_1);
_xifexpression = _builder_1.toString();
}
_xblockexpression = _xifexpression;
}
return _xblockexpression;
}
@Override
protected String getDoc() {
String _description = this.obj.getDescription();
String _docHtml = null;
if (_description!=null) {
_docHtml=this._docHelper.getDocHtml(_description);
}
return _docHtml;
}
}
public static class ParametersStructureTable extends StructureTable> {
public ParametersStructureTable(final List obj, final String[]... cols) {
super(obj, cols);
}
@Override
protected String render(final String name, final Object referrer, final Indentation ind) {
String _xifexpression = null;
boolean _isEmpty = this.obj.isEmpty();
boolean _not = (!_isEmpty);
if (_not) {
StringConcatenation _builder = new StringConcatenation();
{
for(final Parameter param : this.obj) {
String _renderObject = new StructureTable.ParameterStructureTable(param, this.cols).renderObject(null, referrer, ind.advance2());
_builder.append(_renderObject);
}
}
_xifexpression = _builder.toString();
}
return _xifexpression;
}
@Override
protected String getTypeSpec(final AttrDetails det) {
throw new UnsupportedOperationException("TODO: auto-generated method stub");
}
@Override
protected String getDoc() {
throw new UnsupportedOperationException("TODO: auto-generated method stub");
}
}
public static class ExceptionStructureTable extends StructureTable {
protected ExceptionStructureTable(final Exception obj, final String[]... cols) {
super(obj, cols);
}
@Override
protected String render(final String name, final Object referrer, final Indentation ind) {
return this.defaultRender(name, referrer, ind, null);
}
@Override
protected String getTypeSpec(final AttrDetails det) {
return this.getTypeSpec(this.obj, det);
}
/**
* Attempt to render nonsensical array
*/
private String _getTypeSpec(final BadArrayException e, final AttrDetails det) {
return "???[]";
}
private String _getTypeSpec(final BadReferenceException e, final AttrDetails det) {
return "ref";
}
private String _getTypeSpec(final RecursiveRenderException e, final AttrDetails det) {
return "(recursive)";
}
@Override
protected String renderColumn(final String colAttr, final String name, final Object referrer, final Indentation ind, final AttrDetails det) {
String _xifexpression = null;
if ((this.obj instanceof RecursiveRenderException)) {
_xifexpression = this.renderColumn(((RecursiveRenderException)this.obj), colAttr, name, referrer, ind, det);
} else {
_xifexpression = super.renderColumn(colAttr, name, referrer, ind, det);
}
return _xifexpression;
}
/**
* Recursive rendering attempt
*/
protected String renderColumn(final RecursiveRenderException e, final String colAttr, final String name, final Object referrer, final Indentation ind, final AttrDetails det) {
String _xblockexpression = null;
{
final Object obj = this._refHelper.safeResolve(e.getObject());
String _switchResult = null;
if (colAttr != null) {
switch (colAttr) {
case "name":
String _xblockexpression_1 = null;
{
final String tooltip = " …";
String _htmlEscape = this._htmlHelper.htmlEscape(this.chooseName(obj, name));
_xblockexpression_1 = this.indentCode((_htmlEscape + tooltip), ind);
}
_switchResult = _xblockexpression_1;
break;
case "type":
String _typeSpec = this.getTypeSpec(det);
String _code = null;
if (_typeSpec!=null) {
_code=this._htmlHelper.getCode(_typeSpec);
}
_switchResult = _code;
break;
default:
_switchResult = this.getValueForDisplay(this._attributeHelper.getAttribute(obj, colAttr));
break;
}
} else {
_switchResult = this.getValueForDisplay(this._attributeHelper.getAttribute(obj, colAttr));
}
_xblockexpression = _switchResult;
}
return _xblockexpression;
}
@Override
protected String getDoc() {
return this.getDoc(this.obj);
}
private String _getDoc(final BadReferenceException e) {
String _xblockexpression = null;
{
final String refString = e.getRefString().replaceAll("#/_UNRESOLVABLE/", "");
StringConcatenation _builder = new StringConcatenation();
_builder.append("Invalid Reference: ");
String _htmlEscape = this._htmlHelper.htmlEscape(refString);
_builder.append(_htmlEscape);
_builder.append("
");
_xblockexpression = _builder.toString();
}
return _xblockexpression;
}
private String _getDoc(final BadArrayException e) {
return e.getMessage();
}
private String getTypeSpec(final Exception e, final AttrDetails det) {
if (e instanceof BadArrayException) {
return _getTypeSpec((BadArrayException)e, det);
} else if (e instanceof BadReferenceException) {
return _getTypeSpec((BadReferenceException)e, det);
} else if (e instanceof RecursiveRenderException) {
return _getTypeSpec((RecursiveRenderException)e, det);
} else {
throw new IllegalArgumentException("Unhandled parameter types: " +
Arrays.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy