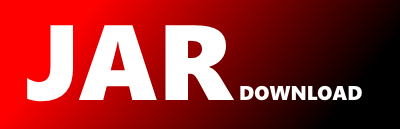
com.reprezen.genflow.rapidml.jaxrs.JavaXtendTemplate Maven / Gradle / Ivy
/**
* Copyright © 2013, 2016 Modelsolv, Inc.
* All Rights Reserved.
*
* NOTICE: All information contained herein is, and remains the property
* of ModelSolv, Inc. See the file license.html in the root directory of
* this project for further information.
*/
package com.reprezen.genflow.rapidml.jaxrs;
import com.reprezen.genflow.api.util.TypeUtils;
import com.reprezen.genflow.api.zenmodel.ZenModelExtractOutputItem;
import java.util.Collections;
import java.util.List;
import java.util.Set;
import org.eclipse.emf.ecore.EObject;
import org.eclipse.xtext.xbase.lib.CollectionLiterals;
import org.eclipse.xtext.xbase.lib.Exceptions;
import org.eclipse.xtext.xbase.lib.Functions.Function1;
import org.eclipse.xtext.xbase.lib.IterableExtensions;
import org.eclipse.xtext.xbase.lib.ListExtensions;
@SuppressWarnings("all")
public abstract class JavaXtendTemplate extends ZenModelExtractOutputItem {
private final static Set JAVA_KEYWORDS = Collections.unmodifiableSet(CollectionLiterals.newHashSet("abstract", "assert", "boolean", "break", "byte", "case", "catch", "char", "class", "const", "continue", "default", "do", "double", "else", "enum", "extends", "false", "final", "finally", "float", "for", "goto", "if", "implements", "import", "instanceof", "int", "interface", "long", "native", "new", "null", "package", "private", "protected", "public", "return", "short", "static", "strictfp", "super", "switch", "synchronized", "this", "throw", "throws", "transient", "true", "try", "void", "volatile", "while"));
private final Set imports = CollectionLiterals.newHashSet();
@Override
public Class> getItemType() {
try {
Class extends JavaXtendTemplate> _class = this.getClass();
return TypeUtils.getTypeParamClass(_class, JavaXtendTemplate.class, 0);
} catch (Throwable _e) {
throw Exceptions.sneakyThrow(_e);
}
}
protected String escapeJavaKeywords(final String name) {
boolean _contains = JavaXtendTemplate.JAVA_KEYWORDS.contains(name);
if (_contains) {
return name.concat("_arg");
} else {
return name.replaceAll("[:\\.\\-]", "_");
}
}
protected void addImport(final Class> clazz) {
boolean _isPrimitive = clazz.isPrimitive();
boolean _not = (!_isPrimitive);
if (_not) {
String _canonicalName = clazz.getCanonicalName();
this.addImport(_canonicalName);
}
}
protected void addImport(final String fqClassName) {
this.imports.add(fqClassName);
}
protected String generateImports() {
List _sort = IterableExtensions.sort(this.imports);
final Function1 _function = (String it) -> {
return (("import " + it) + ";\n");
};
List _map = ListExtensions.map(_sort, _function);
return IterableExtensions.join(_map, "");
}
protected String getTypeName(final Class> value) {
if ((value == null)) {
return null;
}
this.addImport(value);
return value.getSimpleName();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy