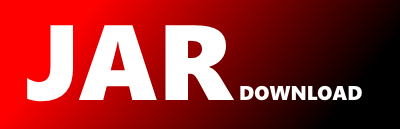
org.eclipse.swt.graphics.Image Maven / Gradle / Ivy
Show all versions of org.eclipse.swt.macosx.x86_64 Show documentation
/*******************************************************************************
* Copyright (c) 2000, 2016 IBM Corporation and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* IBM Corporation - initial API and implementation
*******************************************************************************/
package org.eclipse.swt.graphics;
import java.io.*;
import org.eclipse.swt.*;
import org.eclipse.swt.internal.*;
import org.eclipse.swt.internal.cocoa.*;
/**
* Instances of this class are graphics which have been prepared
* for display on a specific device. That is, they are ready
* to paint using methods such as GC.drawImage()
* and display on widgets with, for example, Button.setImage()
.
*
* If loaded from a file format that supports it, an
* Image
may have transparency, meaning that certain
* pixels are specified as being transparent when drawn. Examples
* of file formats that support transparency are GIF and PNG.
*
* There are two primary ways to use Images
.
* The first is to load a graphic file from disk and create an
* Image
from it. This is done using an Image
* constructor, for example:
*
* Image i = new Image(device, "C:\\graphic.bmp");
*
* A graphic file may contain a color table specifying which
* colors the image was intended to possess. In the above example,
* these colors will be mapped to the closest available color in
* SWT. It is possible to get more control over the mapping of
* colors as the image is being created, using code of the form:
*
* ImageData data = new ImageData("C:\\graphic.bmp");
* RGB[] rgbs = data.getRGBs();
* // At this point, rgbs contains specifications of all
* // the colors contained within this image. You may
* // allocate as many of these colors as you wish by
* // using the Color constructor Color(RGB), then
* // create the image:
* Image i = new Image(device, data);
*
*
* Applications which require even greater control over the image
* loading process should use the support provided in class
* ImageLoader
.
*
* Application code must explicitly invoke the Image.dispose()
* method to release the operating system resources managed by each instance
* when those instances are no longer required.
*
*
* @see Color
* @see ImageData
* @see ImageLoader
* @see Image snippets
* @see SWT Examples: GraphicsExample, ImageAnalyzer
* @see Sample code and further information
*/
public final class Image extends Resource implements Drawable {
/**
* specifies whether the receiver is a bitmap or an icon
* (one of SWT.BITMAP
, SWT.ICON
)
*
* IMPORTANT: This field is not part of the SWT
* public API. It is marked public only so that it can be shared
* within the packages provided by SWT. It is not available on all
* platforms and should never be accessed from application code.
*
*
* @noreference This field is not intended to be referenced by clients.
*/
public int type;
/**
* the handle to the OS image resource
* (Warning: This field is platform dependent)
*
* IMPORTANT: This field is not part of the SWT
* public API. It is marked public only so that it can be shared
* within the packages provided by SWT. It is not available on all
* platforms and should never be accessed from application code.
*
*
* @noreference This field is not intended to be referenced by clients.
*/
public NSImage handle;
/**
* specifies the transparent pixel
*/
int transparentPixel = -1;
/**
* The GC the image is currently selected in.
*/
GC memGC;
/**
* The alpha data of the image.
*/
byte[] alphaData;
/**
* The global alpha value to be used for every pixel.
*/
int alpha = -1;
/**
* The width of the image.
*/
int width = -1;
/**
* The height of the image.
*/
int height = -1;
/**
* Specifies the default scanline padding.
*/
static final int DEFAULT_SCANLINE_PAD = 4;
/**
* ImageFileNameProvider to provide file names at various Zoom levels
*/
ImageFileNameProvider imageFileNameProvider;
/**
* ImageDataProvider to provide ImageData at various Zoom levels
*/
ImageDataProvider imageDataProvider;
Image(Device device) {
super(device);
}
/**
* Constructs an empty instance of this class with the
* specified width and height. The result may be drawn upon
* by creating a GC and using any of its drawing operations,
* as shown in the following example:
*
* Image i = new Image(device, width, height);
* GC gc = new GC(i);
* gc.drawRectangle(0, 0, 50, 50);
* gc.dispose();
*
*
* Note: Some platforms may have a limitation on the size
* of image that can be created (size depends on width, height,
* and depth). For example, Windows 95, 98, and ME do not allow
* images larger than 16M.
*
*
* You must dispose the image when it is no longer required.
*
*
* @param device the device on which to create the image
* @param width the width of the new image
* @param height the height of the new image
*
* @exception IllegalArgumentException
* - ERROR_NULL_ARGUMENT - if device is null and there is no current device
* - ERROR_INVALID_ARGUMENT - if either the width or height is negative or zero
*
* @exception SWTError
* - ERROR_NO_HANDLES if a handle could not be obtained for image creation
*
*
* @see #dispose()
*/
public Image(Device device, int width, int height) {
super(device);
NSAutoreleasePool pool = null;
if (!NSThread.isMainThread()) pool = (NSAutoreleasePool) new NSAutoreleasePool().alloc().init();
try {
init(width, height);
init();
} finally {
if (pool != null) pool.release();
}
}
/**
* Constructs a new instance of this class based on the
* provided image, with an appearance that varies depending
* on the value of the flag. The possible flag values are:
*
* - {@link SWT#IMAGE_COPY}
* - the result is an identical copy of srcImage
* - {@link SWT#IMAGE_DISABLE}
* - the result is a copy of srcImage which has a disabled look
* - {@link SWT#IMAGE_GRAY}
* - the result is a copy of srcImage which has a gray scale look
*
*
* You must dispose the image when it is no longer required.
*
*
* @param device the device on which to create the image
* @param srcImage the image to use as the source
* @param flag the style, either IMAGE_COPY
, IMAGE_DISABLE
or IMAGE_GRAY
*
* @exception IllegalArgumentException
* - ERROR_NULL_ARGUMENT - if device is null and there is no current device
* - ERROR_NULL_ARGUMENT - if srcImage is null
* - ERROR_INVALID_ARGUMENT - if the flag is not one of
IMAGE_COPY
, IMAGE_DISABLE
or IMAGE_GRAY
* - ERROR_INVALID_ARGUMENT - if the image has been disposed
*
* @exception SWTException
* - ERROR_INVALID_IMAGE - if the image is not a bitmap or an icon, or is otherwise in an invalid state
* - ERROR_UNSUPPORTED_DEPTH - if the depth of the image is not supported
*
* @exception SWTError
* - ERROR_NO_HANDLES if a handle could not be obtained for image creation
*
*
* @see #dispose()
*/
public Image(Device device, Image srcImage, int flag) {
super(device);
if (srcImage == null) SWT.error(SWT.ERROR_NULL_ARGUMENT);
if (srcImage.isDisposed()) SWT.error(SWT.ERROR_INVALID_ARGUMENT);
switch (flag) {
case SWT.IMAGE_COPY:
case SWT.IMAGE_DISABLE:
case SWT.IMAGE_GRAY:
break;
default:
SWT.error(SWT.ERROR_INVALID_ARGUMENT);
}
NSAutoreleasePool pool = null;
if (!NSThread.isMainThread()) pool = (NSAutoreleasePool) new NSAutoreleasePool().alloc().init();
try {
device = this.device;
this.type = srcImage.type;
/* Get source image size */
NSSize size = srcImage.handle.size();
int width = (int)size.width;
int height = (int)size.height;
NSBitmapImageRep srcRep = srcImage.getRepresentation();
long /*int*/ bpr = srcRep.bytesPerRow();
/* Copy transparent pixel and alpha data when necessary */
transparentPixel = srcImage.transparentPixel;
alpha = srcImage.alpha;
if (srcImage.alphaData != null) {
alphaData = new byte[srcImage.alphaData.length];
System.arraycopy(srcImage.alphaData, 0, alphaData, 0, alphaData.length);
}
long /*int*/ srcData = srcRep.bitmapData();
long /*int*/ format = srcRep.bitmapFormat();
long /*int*/ bpp = srcRep.bitsPerPixel();
/* Create the image */
handle = (NSImage)new NSImage().alloc();
handle = handle.initWithSize(size);
NSBitmapImageRep rep = (NSBitmapImageRep)new NSBitmapImageRep().alloc();
rep = rep.initWithBitmapDataPlanes(0, width, height, srcRep.bitsPerSample(), srcRep.samplesPerPixel(), srcRep.hasAlpha(), srcRep.isPlanar(), OS.NSDeviceRGBColorSpace, format, srcRep.bytesPerRow(), bpp);
handle.addRepresentation(rep);
rep.release();
handle.setCacheMode(OS.NSImageCacheNever);
long /*int*/ data = rep.bitmapData();
OS.memmove(data, srcData, width * height * 4);
if (flag != SWT.IMAGE_COPY) {
final int redOffset, greenOffset, blueOffset;
if (bpp == 32 && (format & OS.NSAlphaFirstBitmapFormat) == 0) {
redOffset = 0;
greenOffset = 1;
blueOffset = 2;
} else {
redOffset = 1;
greenOffset = 2;
blueOffset = 3;
}
/* Apply transformation */
switch (flag) {
case SWT.IMAGE_DISABLE: {
Color zeroColor = device.getSystemColor(SWT.COLOR_WIDGET_NORMAL_SHADOW);
RGB zeroRGB = zeroColor.getRGB();
byte zeroRed = (byte)zeroRGB.red;
byte zeroGreen = (byte)zeroRGB.green;
byte zeroBlue = (byte)zeroRGB.blue;
Color oneColor = device.getSystemColor(SWT.COLOR_WIDGET_BACKGROUND);
RGB oneRGB = oneColor.getRGB();
byte oneRed = (byte)oneRGB.red;
byte oneGreen = (byte)oneRGB.green;
byte oneBlue = (byte)oneRGB.blue;
byte[] line = new byte[(int)/*64*/bpr];
for (int y=0; y> 3);
line[offset+redOffset] = line[offset+greenOffset] = line[offset+blueOffset] = intensity;
offset += 4;
}
OS.memmove(data + (y * bpr), line, bpr);
}
break;
}
}
}
init();
} finally {
if (pool != null) pool.release();
}
}
/**
* Constructs an empty instance of this class with the
* width and height of the specified rectangle. The result
* may be drawn upon by creating a GC and using any of its
* drawing operations, as shown in the following example:
*
* Image i = new Image(device, boundsRectangle);
* GC gc = new GC(i);
* gc.drawRectangle(0, 0, 50, 50);
* gc.dispose();
*
*
* Note: Some platforms may have a limitation on the size
* of image that can be created (size depends on width, height,
* and depth). For example, Windows 95, 98, and ME do not allow
* images larger than 16M.
*
*
* You must dispose the image when it is no longer required.
*
*
* @param device the device on which to create the image
* @param bounds a rectangle specifying the image's width and height (must not be null)
*
* @exception IllegalArgumentException
* - ERROR_NULL_ARGUMENT - if device is null and there is no current device
* - ERROR_NULL_ARGUMENT - if the bounds rectangle is null
* - ERROR_INVALID_ARGUMENT - if either the rectangle's width or height is negative
*
* @exception SWTError
* - ERROR_NO_HANDLES if a handle could not be obtained for image creation
*
*
* @see #dispose()
*/
public Image(Device device, Rectangle bounds) {
super(device);
if (bounds == null) SWT.error(SWT.ERROR_NULL_ARGUMENT);
NSAutoreleasePool pool = null;
if (!NSThread.isMainThread()) pool = (NSAutoreleasePool) new NSAutoreleasePool().alloc().init();
try {
init(bounds.width, bounds.height);
init();
} finally {
if (pool != null) pool.release();
}
}
/**
* Constructs an instance of this class from the given
* ImageData
.
*
* You must dispose the image when it is no longer required.
*
*
* @param device the device on which to create the image
* @param data the image data to create the image from (must not be null)
*
* @exception IllegalArgumentException
* - ERROR_NULL_ARGUMENT - if device is null and there is no current device
* - ERROR_NULL_ARGUMENT - if the image data is null
*
* @exception SWTException
* - ERROR_UNSUPPORTED_DEPTH - if the depth of the ImageData is not supported
*
* @exception SWTError
* - ERROR_NO_HANDLES if a handle could not be obtained for image creation
*
*
* @see #dispose()
*/
public Image(Device device, ImageData data) {
super(device);
NSAutoreleasePool pool = null;
if (!NSThread.isMainThread()) pool = (NSAutoreleasePool) new NSAutoreleasePool().alloc().init();
try {
init(data);
init();
} finally {
if (pool != null) pool.release();
}
}
/**
* Constructs an instance of this class, whose type is
* SWT.ICON
, from the two given ImageData
* objects. The two images must be the same size. Pixel transparency
* in either image will be ignored.
*
* The mask image should contain white wherever the icon is to be visible,
* and black wherever the icon is to be transparent. In addition,
* the source image should contain black wherever the icon is to be
* transparent.
*
*
* You must dispose the image when it is no longer required.
*
*
* @param device the device on which to create the icon
* @param source the color data for the icon
* @param mask the mask data for the icon
*
* @exception IllegalArgumentException
* - ERROR_NULL_ARGUMENT - if device is null and there is no current device
* - ERROR_NULL_ARGUMENT - if either the source or mask is null
* - ERROR_INVALID_ARGUMENT - if source and mask are different sizes
*
* @exception SWTError
* - ERROR_NO_HANDLES if a handle could not be obtained for image creation
*
*
* @see #dispose()
*/
public Image(Device device, ImageData source, ImageData mask) {
super(device);
if (source == null) SWT.error(SWT.ERROR_NULL_ARGUMENT);
if (mask == null) SWT.error(SWT.ERROR_NULL_ARGUMENT);
if (source.width != mask.width || source.height != mask.height) {
SWT.error(SWT.ERROR_INVALID_ARGUMENT);
}
NSAutoreleasePool pool = null;
if (!NSThread.isMainThread()) pool = (NSAutoreleasePool) new NSAutoreleasePool().alloc().init();
try {
mask = ImageData.convertMask(mask);
ImageData image = new ImageData(source.width, source.height, source.depth, source.palette, source.scanlinePad, source.data);
image.maskPad = mask.scanlinePad;
image.maskData = mask.data;
init(image);
} finally {
if (pool != null) pool.release();
}
}
/**
* Constructs an instance of this class by loading its representation
* from the specified input stream. Throws an error if an error
* occurs while loading the image, or if the result is an image
* of an unsupported type. Application code is still responsible
* for closing the input stream.
*
* This constructor is provided for convenience when loading a single
* image only. If the stream contains multiple images, only the first
* one will be loaded. To load multiple images, use
* ImageLoader.load()
.
*
* This constructor may be used to load a resource as follows:
*
*
* static Image loadImage (Display display, Class clazz, String string) {
* InputStream stream = clazz.getResourceAsStream (string);
* if (stream == null) return null;
* Image image = null;
* try {
* image = new Image (display, stream);
* } catch (SWTException ex) {
* } finally {
* try {
* stream.close ();
* } catch (IOException ex) {}
* }
* return image;
* }
*
*
* You must dispose the image when it is no longer required.
*
*
* @param device the device on which to create the image
* @param stream the input stream to load the image from
*
* @exception IllegalArgumentException
* - ERROR_NULL_ARGUMENT - if device is null and there is no current device
* - ERROR_NULL_ARGUMENT - if the stream is null
*
* @exception SWTException
* - ERROR_IO - if an IO error occurs while reading from the stream
* - ERROR_INVALID_IMAGE - if the image stream contains invalid data
* - ERROR_UNSUPPORTED_DEPTH - if the image stream describes an image with an unsupported depth
* - ERROR_UNSUPPORTED_FORMAT - if the image stream contains an unrecognized format
*
* @exception SWTError
* - ERROR_NO_HANDLES if a handle could not be obtained for image creation
*
*
* @see #dispose()
*/
public Image(Device device, InputStream stream) {
super(device);
NSAutoreleasePool pool = null;
if (!NSThread.isMainThread()) pool = (NSAutoreleasePool) new NSAutoreleasePool().alloc().init();
try {
init(new ImageData(stream));
init();
} finally {
if (pool != null) pool.release();
}
}
/**
* Constructs an instance of this class by loading its representation
* from the file with the specified name. Throws an error if an error
* occurs while loading the image, or if the result is an image
* of an unsupported type.
*
* This constructor is provided for convenience when loading
* a single image only. If the specified file contains
* multiple images, only the first one will be used.
*
* You must dispose the image when it is no longer required.
*
*
* @param device the device on which to create the image
* @param filename the name of the file to load the image from
*
* @exception IllegalArgumentException
* - ERROR_NULL_ARGUMENT - if device is null and there is no current device
* - ERROR_NULL_ARGUMENT - if the file name is null
*
* @exception SWTException
* - ERROR_IO - if an IO error occurs while reading from the file
* - ERROR_INVALID_IMAGE - if the image file contains invalid data
* - ERROR_UNSUPPORTED_DEPTH - if the image file describes an image with an unsupported depth
* - ERROR_UNSUPPORTED_FORMAT - if the image file contains an unrecognized format
*
* @exception SWTError
* - ERROR_NO_HANDLES if a handle could not be obtained for image creation
*
*
* @see #dispose()
*/
public Image(Device device, String filename) {
super(device);
NSAutoreleasePool pool = null;
if (!NSThread.isMainThread()) pool = (NSAutoreleasePool) new NSAutoreleasePool().alloc().init();
try {
if (filename == null) SWT.error(SWT.ERROR_NULL_ARGUMENT);
initNative(filename);
if (this.handle == null) init(new ImageData(filename));
init();
} finally {
if (pool != null) pool.release();
}
}
/**
* Constructs an instance of this class by loading its representation
* from the file retrieved from the ImageFileNameProvider. Throws an
* error if an error occurs while loading the image, or if the result
* is an image of an unsupported type.
*
* This constructor is provided for convenience for loading image as
* per DPI level.
*
* @param device the device on which to create the image
* @param imageFileNameProvider the ImageFileNameProvider object that is
* to be used to get the file name
*
* @exception IllegalArgumentException
* - ERROR_NULL_ARGUMENT - if device is null and there is no current device
* - ERROR_NULL_ARGUMENT - if the ImageFileNameProvider is null
* - ERROR_INVALID_ARGUMENT - if the fileName provided by ImageFileNameProvider is null at 100% zoom
*
* @exception SWTException
* - ERROR_IO - if an IO error occurs while reading from the file
* - ERROR_INVALID_IMAGE - if the image file contains invalid data
* - ERROR_UNSUPPORTED_DEPTH - if the image file describes an image with an unsupported depth
* - ERROR_UNSUPPORTED_FORMAT - if the image file contains an unrecognized format
*
* @exception SWTError
* - ERROR_NO_HANDLES if a handle could not be obtained for image creation
*
* @since 3.104
*/
public Image(Device device, ImageFileNameProvider imageFileNameProvider) {
super(device);
if (imageFileNameProvider == null) SWT.error(SWT.ERROR_NULL_ARGUMENT);
this.imageFileNameProvider = imageFileNameProvider;
String filename = imageFileNameProvider.getImagePath(100);
if (filename == null) SWT.error(SWT.ERROR_INVALID_ARGUMENT);
NSAutoreleasePool pool = null;
if (!NSThread.isMainThread()) pool = (NSAutoreleasePool) new NSAutoreleasePool().alloc().init();
try {
initNative(filename);
if (this.handle == null) init(new ImageData(filename));
init();
String filename2x = imageFileNameProvider.getImagePath(200);
if (filename2x != null) {
id id = NSImageRep.imageRepWithContentsOfFile(NSString.stringWith(filename2x));
NSImageRep rep = new NSImageRep(id);
handle.addRepresentation(rep);
}
} finally {
if (pool != null) pool.release();
}
}
/**
* Constructs an instance of this class by loading its representation
* from the ImageData retrieved from the ImageDataProvider. Throws an
* error if an error occurs while loading the image, or if the result
* is an image of an unsupported type.
*
* This constructor is provided for convenience for loading image as
* per DPI level.
*
* @param device the device on which to create the image
* @param imageDataProvider the ImageDataProvider object that is
* to be used to get the ImageData
*
* @exception IllegalArgumentException
* - ERROR_NULL_ARGUMENT - if device is null and there is no current device
* - ERROR_NULL_ARGUMENT - if the ImageDataProvider is null
* - ERROR_INVALID_ARGUMENT - if the ImageData provided by ImageDataProvider is null at 100% zoom
*
* @exception SWTException
* - ERROR_IO - if an IO error occurs while reading from the file
* - ERROR_INVALID_IMAGE - if the image file contains invalid data
* - ERROR_UNSUPPORTED_DEPTH - if the image file describes an image with an unsupported depth
* - ERROR_UNSUPPORTED_FORMAT - if the image file contains an unrecognized format
*
* @exception SWTError
* - ERROR_NO_HANDLES if a handle could not be obtained for image creation
*
* @since 3.104
*/
public Image(Device device, ImageDataProvider imageDataProvider) {
super(device);
if (imageDataProvider == null) SWT.error(SWT.ERROR_NULL_ARGUMENT);
this.imageDataProvider = imageDataProvider;
ImageData data = imageDataProvider.getImageData (100);
if (data == null) SWT.error(SWT.ERROR_INVALID_ARGUMENT);
NSAutoreleasePool pool = null;
if (!NSThread.isMainThread()) pool = (NSAutoreleasePool) new NSAutoreleasePool().alloc().init();
try {
init (data);
init ();
ImageData data2x = imageDataProvider.getImageData (200);
if (data2x != null) {
NSBitmapImageRep rep = createRepresentaion (data2x);
handle.addRepresentation(rep);
rep.release();
}
} finally {
if (pool != null) pool.release();
}
}
void createAlpha () {
if (transparentPixel == -1 && alpha == -1 && alphaData == null) return;
NSAutoreleasePool pool = null;
if (!NSThread.isMainThread()) pool = (NSAutoreleasePool) new NSAutoreleasePool().alloc().init();
try {
NSBitmapImageRep imageRep = getRepresentation();
long /*int*/ height = imageRep.pixelsHigh();
long /*int*/ bpr = imageRep.bytesPerRow();
long /*int*/ bitmapData = imageRep.bitmapData();
long /*int*/ format = imageRep.bitmapFormat();
long /*int*/ dataSize = height * bpr;
byte[] srcData = new byte[(int)/*64*/dataSize];
OS.memmove(srcData, bitmapData, dataSize);
if (transparentPixel != -1) {
if ((format & OS.NSAlphaFirstBitmapFormat) != 0) {
for (int i=0; isame object using a class
* specific comparison.
*
* @param object the object to compare with this object
* @return true
if the object is the same as this object and false
otherwise
*
* @see #hashCode
*/
@Override
public boolean equals (Object object) {
if (object == this) return true;
if (!(object instanceof Image)) return false;
Image image = (Image)object;
if (device != image.device || transparentPixel != image.transparentPixel) return false;
if (imageDataProvider != null && image.imageDataProvider != null) {
return imageDataProvider.equals (image.imageDataProvider);
} else if (imageFileNameProvider != null && image.imageFileNameProvider != null) {
return imageFileNameProvider.equals (image.imageFileNameProvider);
} else {
return handle == image.handle;
}
}
/**
* Returns the color to which to map the transparent pixel, or null if
* the receiver has no transparent pixel.
*
* There are certain uses of Images that do not support transparency
* (for example, setting an image into a button or label). In these cases,
* it may be desired to simulate transparency by using the background
* color of the widget to paint the transparent pixels of the image.
* Use this method to check which color will be used in these cases
* in place of transparency. This value may be set with setBackground().
*
*
* @return the background color of the image, or null if there is no transparency in the image
*
* @exception SWTException
* - ERROR_GRAPHIC_DISPOSED - if the receiver has been disposed
*
*/
public Color getBackground() {
if (isDisposed()) SWT.error(SWT.ERROR_GRAPHIC_DISPOSED);
if (transparentPixel == -1) return null;
int red = (transparentPixel >> 16) & 0xFF;
int green = (transparentPixel >> 8) & 0xFF;
int blue = (transparentPixel >> 0) & 0xFF;
return Color.cocoa_new(device, new double /*float*/ []{red / 255f, green / 255f, blue / 255f, 1});
}
/**
* Returns the bounds of the receiver. The rectangle will always
* have x and y values of 0, and the width and height of the
* image.
*
* @return a rectangle specifying the image's bounds in points.
*
* @exception SWTException
* - ERROR_GRAPHIC_DISPOSED - if the receiver has been disposed
* - ERROR_INVALID_IMAGE - if the image is not a bitmap or an icon
*
*/
public Rectangle getBounds() {
if (isDisposed()) SWT.error(SWT.ERROR_GRAPHIC_DISPOSED);
NSAutoreleasePool pool = null;
if (!NSThread.isMainThread()) pool = (NSAutoreleasePool) new NSAutoreleasePool().alloc().init();
try {
if (width != -1 && height != -1) {
return new Rectangle(0, 0, width, height);
}
NSSize size = handle.size();
return new Rectangle(0, 0, width = (int)size.width, height = (int)size.height);
} finally {
if (pool != null) pool.release();
}
}
/**
* Returns the bounds of the receiver. The rectangle will always
* have x and y values of 0, and the width and height of the
* image in pixels.
*
* @return a rectangle specifying the image's bounds in pixels.
*
* @exception SWTException
* - ERROR_GRAPHIC_DISPOSED - if the receiver has been disposed
* - ERROR_INVALID_IMAGE - if the image is not a bitmap or an icon
*
* @since 3.105
*/
public Rectangle getBoundsInPixels() {
Rectangle bounds = getBounds();
int scaleFactor = (int) NSScreen.mainScreen().backingScaleFactor();
bounds.width *= scaleFactor;
bounds.height *= scaleFactor;
return bounds;
}
ImageData _getImageData(NSBitmapImageRep imageRep) {
long /*int*/ width = imageRep.pixelsWide();
long /*int*/ height = imageRep.pixelsHigh();
long /*int*/ bpr = imageRep.bytesPerRow();
long /*int*/ bpp = imageRep.bitsPerPixel();
long /*int*/ bitmapData = imageRep.bitmapData();
long /*int*/ bitmapFormat = imageRep.bitmapFormat();
long /*int*/ dataSize = height * bpr;
byte[] srcData = new byte[(int)/*64*/dataSize];
OS.memmove(srcData, bitmapData, dataSize);
PaletteData palette;
if (bpp == 32 && (bitmapFormat & OS.NSAlphaFirstBitmapFormat) == 0) {
palette = new PaletteData(0xFF000000, 0xFF0000, 0xFF00);
} else {
palette = new PaletteData(0xFF0000, 0xFF00, 0xFF);
}
ImageData data = new ImageData((int)/*64*/width, (int)/*64*/height, (int)/*64*/bpp, palette, 1, srcData);
data.bytesPerLine = (int)/*64*/bpr;
if (imageRep.hasAlpha() && transparentPixel == -1 && alpha == -1 && alphaData == null) {
byte[] alphaData = new byte[(int)/*64*/(width * height)];
int offset = (bitmapFormat & OS.NSAlphaFirstBitmapFormat) != 0 ? 0 : 3, a = 0;
for (int i = offset; i < srcData.length; i+= 4) {
alphaData[a++] = srcData[i];
}
data.alphaData = alphaData;
} else {
data.transparentPixel = transparentPixel;
if (transparentPixel == -1 && type == SWT.ICON) {
/* Get the icon mask data */
int maskPad = 2;
long /*int*/ maskBpl = (((width + 7) / 8) + (maskPad - 1)) / maskPad * maskPad;
byte[] maskData = new byte[(int)/*64*/(height * maskBpl)];
int offset = 0, maskOffset = 0;
for (int y = 0; y> 3)] |= (1 << (7 - (x & 0x7)));
} else {
maskData[maskOffset + (x >> 3)] &= ~(1 << (7 - (x & 0x7)));
}
offset += 4;
}
maskOffset += maskBpl;
}
data.maskData = maskData;
data.maskPad = maskPad;
}
data.alpha = alpha;
if (alpha == -1 && alphaData != null) {
data.alphaData = new byte[alphaData.length];
System.arraycopy(alphaData, 0, data.alphaData, 0, alphaData.length);
}
}
if (bpp == 32) {
int offset = (bitmapFormat & OS.NSAlphaFirstBitmapFormat) != 0 ? 0 : 3;
for (int i = offset; i < srcData.length; i+= 4) {
srcData[i] = 0;
}
}
return data;
}
/**
* Returns an ImageData
based on the receiver.
* Modifications made to this ImageData
will not
* affect the Image.
*
* @return an ImageData
containing the image's data and
* attributes at 100% zoom level.
*
* @exception SWTException
* - ERROR_GRAPHIC_DISPOSED - if the receiver has been disposed
* - ERROR_INVALID_IMAGE - if the image is not a bitmap or an icon
*
*
* @see ImageData
*/
public ImageData getImageData() {
if (isDisposed()) SWT.error(SWT.ERROR_GRAPHIC_DISPOSED);
NSAutoreleasePool pool = null;
if (!NSThread.isMainThread()) pool = (NSAutoreleasePool) new NSAutoreleasePool().alloc().init();
try {
NSBitmapImageRep imageRep = getActualRepresentation();
return _getImageData(imageRep);
} finally {
if (pool != null) pool.release();
}
}
/**
* Returns an ImageData
based on the receiver.
* Modifications made to this ImageData
will not
* affect the Image.
*
* @return an ImageData
containing the image's data
* and attributes at the current zoom level.
*
* @exception SWTException
* - ERROR_GRAPHIC_DISPOSED - if the receiver has been disposed
* - ERROR_INVALID_IMAGE - if the image is not a bitmap or an icon
*
*
* @see ImageData
* @since 3.105
*/
public ImageData getImageDataAtCurrentZoom() {
if (isDisposed()) SWT.error(SWT.ERROR_GRAPHIC_DISPOSED);
NSAutoreleasePool pool = null;
if (!NSThread.isMainThread()) pool = (NSAutoreleasePool) new NSAutoreleasePool().alloc().init();
try {
if (imageFileNameProvider != null || imageDataProvider != null) {
NSBitmapImageRep imageRep = getRepresentation();
return _getImageData(imageRep);
}
return DPIUtil.autoScaleImageData(device, getImageData(), DPIUtil.getDeviceZoom(), 100);
} finally {
if (pool != null) pool.release();
}
}
/**
* Invokes platform specific functionality to allocate a new image.
*
* IMPORTANT: This method is not part of the public
* API for Image
. It is marked public only so that it
* can be shared within the packages provided by SWT. It is not
* available on all platforms, and should never be called from
* application code.
*
*
* @param device the device on which to allocate the color
* @param type the type of the image (SWT.BITMAP
or SWT.ICON
)
* @param nsImage the OS data for the image
*
* @noreference This method is not intended to be referenced by clients.
*/
public static Image cocoa_new(Device device, int type, NSImage nsImage) {
Image image = new Image(device);
image.type = type;
image.handle = nsImage;
return image;
}
// Method to get the Image representation for the actual image without any scaling.
NSBitmapImageRep getActualRepresentation () {
NSArray reps = handle.representations();
NSSize size = handle.size();
long /*int*/ count = reps.count();
for (int i = 0; i < count; i++) {
NSBitmapImageRep rep = new NSBitmapImageRep(reps.objectAtIndex(i));
if (((int)size.width == rep.pixelsWide() && (int)size.height == rep.pixelsHigh())) {
if (rep.isKindOfClass(OS.class_NSBitmapImageRep)) {
return rep;
}
}
}
NSBitmapImageRep newRep = (NSBitmapImageRep)new NSBitmapImageRep().alloc();
newRep = newRep.initWithData(handle.TIFFRepresentation());
handle.addRepresentation(newRep);
newRep.release();
return newRep;
}
NSBitmapImageRep getRepresentation () {
NSBitmapImageRep rep = new NSBitmapImageRep(handle.bestRepresentationForDevice(null));
if (rep.isKindOfClass(OS.class_NSBitmapImageRep)) {
return rep;
}
NSArray reps = handle.representations();
NSSize size = handle.size();
long /*int*/ count = reps.count();
NSBitmapImageRep bestRep = null;
for (int i = 0; i < count; i++) {
rep = new NSBitmapImageRep(reps.objectAtIndex(i));
if (rep.isKindOfClass(OS.class_NSBitmapImageRep)) return rep;
if (bestRep == null || ((int)size.width == rep.pixelsWide() && (int)size.height == rep.pixelsHigh())) {
bestRep = rep;
}
}
bestRep.retain();
for (int i = 0; i < count; i++) {
handle.removeRepresentation(new NSImageRep(handle.representations().objectAtIndex(0)));
}
handle.addRepresentation(bestRep);
NSBitmapImageRep newRep = (NSBitmapImageRep)new NSBitmapImageRep().alloc();
newRep = newRep.initWithData(handle.TIFFRepresentation());
handle.addRepresentation(newRep);
handle.removeRepresentation(bestRep);
bestRep.release();
newRep.release();
return newRep;
}
/**
* Returns an integer hash code for the receiver. Any two
* objects that return true
when passed to
* equals
must return the same value for this
* method.
*
* @return the receiver's hash
*
* @see #equals
*/
@Override
public int hashCode () {
if (imageDataProvider != null) {
return imageDataProvider.hashCode();
} else if (imageFileNameProvider != null) {
return imageFileNameProvider.hashCode();
} else {
return handle != null ? (int)/*64*/handle.id : 0;
}
}
void init(int width, int height) {
if (width <= 0 || height <= 0) {
SWT.error (SWT.ERROR_INVALID_ARGUMENT);
}
this.type = SWT.BITMAP;
this.width = width;
this.height = height;
handle = (NSImage)new NSImage().alloc();
NSSize size = new NSSize();
size.width = width;
size.height = height;
handle = handle.initWithSize(size);
NSBitmapImageRep rep = (NSBitmapImageRep)new NSBitmapImageRep().alloc();
rep = rep.initWithBitmapDataPlanes(0, width, height, 8, 3, false, false, OS.NSDeviceRGBColorSpace, OS.NSAlphaFirstBitmapFormat | OS.NSAlphaNonpremultipliedBitmapFormat, width * 4, 32);
OS.memset(rep.bitmapData(), 0xFF, width * height * 4);
handle.addRepresentation(rep);
rep.release();
handle.setCacheMode(OS.NSImageCacheNever);
}
void init(ImageData image) {
if (image == null) SWT.error(SWT.ERROR_NULL_ARGUMENT);
if (handle != null) handle.release();
handle = (NSImage)new NSImage().alloc();
NSSize size = new NSSize();
size.width = width;
size.height = height;
handle = handle.initWithSize(size);
this.width = image.width;
this.height = image.height;
NSBitmapImageRep rep = createRepresentaion(image);
handle.addRepresentation(rep);
rep.release();
handle.setCacheMode(OS.NSImageCacheNever);
}
private NSBitmapImageRep createRepresentaion(ImageData imageData) {
NSBitmapImageRep rep = (NSBitmapImageRep)new NSBitmapImageRep().alloc();
PaletteData palette = imageData.palette;
if (!(((imageData.depth == 1 || imageData.depth == 2 || imageData.depth == 4 || imageData.depth == 8) && !palette.isDirect) ||
((imageData.depth == 8) || (imageData.depth == 16 || imageData.depth == 24 || imageData.depth == 32) && palette.isDirect)))
SWT.error(SWT.ERROR_UNSUPPORTED_DEPTH);
/* Create the image */
int dataSize = imageData.width * imageData.height * 4;
/* Initialize data */
int bpr = imageData.width * 4;
byte[] buffer = new byte[dataSize];
if (palette.isDirect) {
ImageData.blit(ImageData.BLIT_SRC,
imageData.data, imageData.depth, imageData.bytesPerLine, imageData.getByteOrder(), 0, 0, imageData.width, imageData.height, palette.redMask, palette.greenMask, palette.blueMask,
ImageData.ALPHA_OPAQUE, null, 0, 0, 0,
buffer, 32, bpr, ImageData.MSB_FIRST, 0, 0, imageData.width, imageData.height, 0xFF0000, 0xFF00, 0xFF,
false, false);
} else {
RGB[] rgbs = palette.getRGBs();
int length = rgbs.length;
byte[] srcReds = new byte[length];
byte[] srcGreens = new byte[length];
byte[] srcBlues = new byte[length];
for (int i = 0; i < rgbs.length; i++) {
RGB rgb = rgbs[i];
if (rgb == null) continue;
srcReds[i] = (byte)rgb.red;
srcGreens[i] = (byte)rgb.green;
srcBlues[i] = (byte)rgb.blue;
}
ImageData.blit(ImageData.BLIT_SRC,
imageData.data, imageData.depth, imageData.bytesPerLine, imageData.getByteOrder(), 0, 0, imageData.width, imageData.height, srcReds, srcGreens, srcBlues,
ImageData.ALPHA_OPAQUE, null, 0, 0, 0,
buffer, 32, bpr, ImageData.MSB_FIRST, 0, 0, imageData.width, imageData.height, 0xFF0000, 0xFF00, 0xFF,
false, false);
}
/* Initialize transparency */
int transparency = imageData.getTransparencyType();
boolean hasAlpha = transparency != SWT.TRANSPARENCY_NONE;
if (transparency == SWT.TRANSPARENCY_MASK || imageData.transparentPixel != -1) {
this.type = imageData.transparentPixel != -1 ? SWT.BITMAP : SWT.ICON;
if (imageData.transparentPixel != -1) {
int transRed = 0, transGreen = 0, transBlue = 0;
if (palette.isDirect) {
RGB rgb = palette.getRGB(imageData.transparentPixel);
transRed = rgb.red;
transGreen = rgb.green;
transBlue = rgb.blue;
} else {
RGB[] rgbs = palette.getRGBs();
if (imageData.transparentPixel < rgbs.length) {
RGB rgb = rgbs[imageData.transparentPixel];
transRed = rgb.red;
transGreen = rgb.green;
transBlue = rgb.blue;
}
}
transparentPixel = transRed << 16 | transGreen << 8 | transBlue;
}
ImageData maskImage = imageData.getTransparencyMask();
byte[] maskData = maskImage.data;
int maskBpl = maskImage.bytesPerLine;
int offset = 0, maskOffset = 0;
for (int y = 0; y> 3)]) & (1 << (7 - (x & 0x7)))) != 0 ? (byte)0xff : 0;
offset += 4;
}
maskOffset += maskBpl;
}
} else {
this.type = SWT.BITMAP;
if (imageData.alpha != -1) {
hasAlpha = true;
this.alpha = imageData.alpha;
byte a = (byte)this.alpha;
for (int dataIndex=0; dataIndex
* IMPORTANT: This method is not part of the public
* API for Image
. It is marked public only so that it
* can be shared within the packages provided by SWT. It is not
* available on all platforms, and should never be called from
* application code.
*
*
* @param data the platform specific GC data
* @return the platform specific GC handle
*
* @noreference This method is not intended to be referenced by clients.
*/
@Override
public long /*int*/ internal_new_GC (GCData data) {
if (handle == null) SWT.error(SWT.ERROR_GRAPHIC_DISPOSED);
if (type != SWT.BITMAP || memGC != null) {
SWT.error(SWT.ERROR_INVALID_ARGUMENT);
}
NSAutoreleasePool pool = null;
if (!NSThread.isMainThread()) pool = (NSAutoreleasePool) new NSAutoreleasePool().alloc().init();
try {
NSBitmapImageRep imageRep = getRepresentation();
// Can't perform transforms on image reps with alpha.
imageRep.setAlpha(false);
NSGraphicsContext context = NSGraphicsContext.graphicsContextWithBitmapImageRep(imageRep);
NSGraphicsContext flippedContext = NSGraphicsContext.graphicsContextWithGraphicsPort(context.graphicsPort(), true);
context = flippedContext;
context.retain();
if (data != null) data.flippedContext = flippedContext;
NSGraphicsContext.static_saveGraphicsState();
NSGraphicsContext.setCurrentContext(context);
NSAffineTransform transform = NSAffineTransform.transform();
NSSize size = handle.size();
transform.translateXBy(0, size.height);
transform.scaleXBy(1, -1);
transform.set();
NSGraphicsContext.static_restoreGraphicsState();
if (data != null) {
int mask = SWT.LEFT_TO_RIGHT | SWT.RIGHT_TO_LEFT;
if ((data.style & mask) == 0) {
data.style |= SWT.LEFT_TO_RIGHT;
}
data.device = device;
data.background = device.COLOR_WHITE.handle;
data.foreground = device.COLOR_BLACK.handle;
data.font = device.systemFont;
data.image = this;
}
return context.id;
} finally {
if (pool != null) pool.release();
}
}
/**
* Invokes platform specific functionality to dispose a GC handle.
*
* IMPORTANT: This method is not part of the public
* API for Image
. It is marked public only so that it
* can be shared within the packages provided by SWT. It is not
* available on all platforms, and should never be called from
* application code.
*
*
* @param hDC the platform specific GC handle
* @param data the platform specific GC data
*
* @noreference This method is not intended to be referenced by clients.
*/
@Override
public void internal_dispose_GC (long /*int*/ hDC, GCData data) {
long /*int*/ context = hDC;
NSAutoreleasePool pool = null;
if (!NSThread.isMainThread()) pool = (NSAutoreleasePool) new NSAutoreleasePool().alloc().init();
try {
if (context != 0) {
/*
* Bug in cocoa. For some reason, there cases when the image pixels
* are not transfered from the underlining CGImage into the bitmap
* representation. This only happens when bitmapData() is called.
*/
NSBitmapImageRep imageRep = getRepresentation();
imageRep.bitmapData();
NSGraphicsContext contextObj = new NSGraphicsContext(context);
contextObj.release();
}
// handle.setCacheMode(OS.NSImageCacheDefault);
} finally {
if (pool != null) pool.release();
}
}
/**
* Returns true
if the image has been disposed,
* and false
otherwise.
*
* This method gets the dispose state for the image.
* When an image has been disposed, it is an error to
* invoke any other method (except {@link #dispose()}) using the image.
*
* @return true
when the image is disposed and false
otherwise
*/
@Override
public boolean isDisposed() {
return handle == null;
}
/**
* Sets the color to which to map the transparent pixel.
*
* There are certain uses of Images
that do not support
* transparency (for example, setting an image into a button or label).
* In these cases, it may be desired to simulate transparency by using
* the background color of the widget to paint the transparent pixels
* of the image. This method specifies the color that will be used in
* these cases. For example:
*
* Button b = new Button();
* image.setBackground(b.getBackground());
* b.setImage(image);
*
*
* The image may be modified by this operation (in effect, the
* transparent regions may be filled with the supplied color). Hence
* this operation is not reversible and it is not legal to call
* this function twice or with a null argument.
*
* This method has no effect if the receiver does not have a transparent
* pixel value.
*
*
* @param color the color to use when a transparent pixel is specified
*
* @exception IllegalArgumentException
* - ERROR_NULL_ARGUMENT - if the color is null
* - ERROR_INVALID_ARGUMENT - if the color has been disposed
*
* @exception SWTException
* - ERROR_GRAPHIC_DISPOSED - if the receiver has been disposed
*
*/
public void setBackground(Color color) {
if (isDisposed()) SWT.error(SWT.ERROR_GRAPHIC_DISPOSED);
if (color == null) SWT.error(SWT.ERROR_NULL_ARGUMENT);
if (color.isDisposed()) SWT.error(SWT.ERROR_INVALID_ARGUMENT);
if (transparentPixel == -1) return;
NSAutoreleasePool pool = null;
if (!NSThread.isMainThread()) pool = (NSAutoreleasePool) new NSAutoreleasePool().alloc().init();
try {
byte red = (byte)((transparentPixel >> 16) & 0xFF);
byte green = (byte)((transparentPixel >> 8) & 0xFF);
byte blue = (byte)((transparentPixel >> 0) & 0xFF);
byte newRed = (byte)((int)(color.handle[0] * 255) & 0xFF);
byte newGreen = (byte)((int)(color.handle[1] * 255) & 0xFF);
byte newBlue = (byte)((int)(color.handle[2] * 255) & 0xFF);
NSBitmapImageRep imageRep = getRepresentation();
long /*int*/ bpr = imageRep.bytesPerRow();
long /*int*/ data = imageRep.bitmapData();
long /*int*/ format = imageRep.bitmapFormat();
long /*int*/ bpp = imageRep.bitsPerPixel();
final int redOffset, greenOffset, blueOffset;
if (bpp == 32 && (format & OS.NSAlphaFirstBitmapFormat) == 0) {
redOffset = 0;
greenOffset = 1;
blueOffset = 2;
} else {
redOffset = 1;
greenOffset = 2;
blueOffset = 3;
}
byte[] line = new byte[(int)bpr];
for (int i = 0, offset = 0; i < height; i++, offset += bpr) {
OS.memmove(line, data + offset, bpr);
for (int j = 0; j < line.length; j += 4) {
if (line[j + redOffset] == red && line[j + greenOffset] == green && line[j + blueOffset] == blue) {
line[j + redOffset] = newRed;
line[j + greenOffset] = newGreen;
line[j + blueOffset] = newBlue;
}
}
OS.memmove(data + offset, line, bpr);
}
transparentPixel = (newRed & 0xFF) << 16 | (newGreen & 0xFF) << 8 | (newBlue & 0xFF);
} finally {
if (pool != null) pool.release();
}
}
/**
* Returns a string containing a concise, human-readable
* description of the receiver.
*
* @return a string representation of the receiver
*/
@Override
public String toString () {
if (isDisposed()) return "Image {*DISPOSED*}";
return "Image {" + handle + "}";
}
}