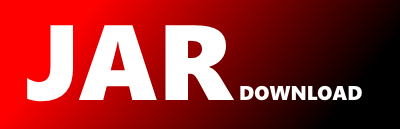
org.eclipse.swt.widgets.Menu Maven / Gradle / Ivy
Show all versions of org.eclipse.swt.macosx.x86_64 Show documentation
/******************************************************************************* * Copyright (c) 2000, 2012 IBM Corporation and others. * All rights reserved. This program and the accompanying materials * are made available under the terms of the Eclipse Public License v1.0 * which accompanies this distribution, and is available at * http://www.eclipse.org/legal/epl-v10.html * * Contributors: * IBM Corporation - initial API and implementation *******************************************************************************/ package org.eclipse.swt.widgets; import org.eclipse.swt.*; import org.eclipse.swt.events.*; import org.eclipse.swt.graphics.*; import org.eclipse.swt.internal.cocoa.*; /** * Instances of this class are user interface objects that contain * menu items. *
or*
*- Styles:
*- BAR, DROP_DOWN, POP_UP, NO_RADIO_GROUP
*- LEFT_TO_RIGHT, RIGHT_TO_LEFT
*- Events:
*- Help, Hide, Show
** Note: Only one of BAR, DROP_DOWN and POP_UP may be specified. * Only one of LEFT_TO_RIGHT or RIGHT_TO_LEFT may be specified. *
* IMPORTANT: This class is not intended to be subclassed. *
* * @see Menu snippets * @see SWT Example: ControlExample * @see Sample code and further information * @noextend This class is not intended to be subclassed by clients. */ public class Menu extends Widget { NSMenu nsMenu; int x, y, itemCount; boolean hasLocation, visible; MenuItem [] items; MenuItem cascade, defaultItem; Decorations parent; static final int GAP = 4; /** * Constructs a new instance of this class given its parent, * and sets the style for the instance so that the instance * will be a popup menu on the given parent's shell. ** After constructing a menu, it can be set into its parent * using
* * @param parent a control which will be the parent of the new instance (cannot be null) * * @exception IllegalArgumentExceptionparent.setMenu(menu)
. In this case, the parent may * be any control in the same widget tree as the parent. **
* @exception SWTException- ERROR_NULL_ARGUMENT - if the parent is null
**
* * @see SWT#POP_UP * @see Widget#checkSubclass * @see Widget#getStyle */ public Menu (Control parent) { this (checkNull (parent).menuShell (), SWT.POP_UP); } /** * Constructs a new instance of this class given its parent * (which must be a- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the parent
*- ERROR_INVALID_SUBCLASS - if this class is not an allowed subclass
*Decorations
) and a style value * describing its behavior and appearance. ** The style value is either one of the style constants defined in * class
SWT
which is applicable to instances of this * class, or must be built by bitwise OR'ing together * (that is, using theint
"|" operator) two or more * of thoseSWT
style constants. The class description * lists the style constants that are applicable to the class. * Style bits are also inherited from superclasses. ** After constructing a menu or menuBar, it can be set into its parent * using
* * @param parent a decorations control which will be the parent of the new instance (cannot be null) * @param style the style of menu to construct * * @exception IllegalArgumentExceptionparent.setMenu(menu)
orparent.setMenuBar(menuBar)
. **
* @exception SWTException- ERROR_NULL_ARGUMENT - if the parent is null
**
* * @see SWT#BAR * @see SWT#DROP_DOWN * @see SWT#POP_UP * @see SWT#NO_RADIO_GROUP * @see SWT#LEFT_TO_RIGHT * @see SWT#RIGHT_TO_LEFT * @see Widget#checkSubclass * @see Widget#getStyle */ public Menu (Decorations parent, int style) { checkSubclass (); checkParent (parent); this.style = checkStyle(style); if (parent != null) { display = parent.display; } else { display = Display.getCurrent (); if (display == null) display = Display.getDefault (); if (!display.isValidThread ()) { error (SWT.ERROR_THREAD_INVALID_ACCESS); } } this.parent = parent; reskinWidget(); createWidget(); } /** * Constructs a new instance of this class given its parent * (which must be a- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the parent
*- ERROR_INVALID_SUBCLASS - if this class is not an allowed subclass
*Menu
) and sets the style * for the instance so that the instance will be a drop-down * menu on the given parent's parent. ** After constructing a drop-down menu, it can be set into its parentMenu * using
* * @param parentMenu a menu which will be the parent of the new instance (cannot be null) * * @exception IllegalArgumentExceptionparentMenu.setMenu(menu)
. **
* @exception SWTException- ERROR_NULL_ARGUMENT - if the parent is null
**
* * @see SWT#DROP_DOWN * @see Widget#checkSubclass * @see Widget#getStyle */ public Menu (Menu parentMenu) { this (checkNull (parentMenu).parent, SWT.DROP_DOWN); } /** * Constructs a new instance of this class given its parent * (which must be a- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the parent
*- ERROR_INVALID_SUBCLASS - if this class is not an allowed subclass
*MenuItem
) and sets the style * for the instance so that the instance will be a drop-down * menu on the given parent's parent menu. ** After constructing a drop-down menu, it can be set into its parentItem * using
* * @param parentItem a menu item which will be the parent of the new instance (cannot be null) * * @exception IllegalArgumentExceptionparentItem.setMenu(menu)
. **
* @exception SWTException- ERROR_NULL_ARGUMENT - if the parent is null
**
* * @see SWT#DROP_DOWN * @see Widget#checkSubclass * @see Widget#getStyle */ public Menu (MenuItem parentItem) { this (checkNull (parentItem).parent); } Menu (Display display) { if (display == null) display = Display.getCurrent (); if (display == null) display = Display.getDefault (); if (!display.isValidThread ()) { error (SWT.ERROR_THREAD_INVALID_ACCESS); } this.display = display; this.style = SWT.BAR; reskinWidget(); createWidget(); } Menu (Display display, NSMenu nativeMenu) { this.display = display; this.style = SWT.DROP_DOWN; this.nsMenu = nativeMenu; reskinWidget(); createWidget(); } static Control checkNull (Control control) { if (control == null) SWT.error (SWT.ERROR_NULL_ARGUMENT); return control; } static Menu checkNull (Menu menu) { if (menu == null) SWT.error (SWT.ERROR_NULL_ARGUMENT); return menu; } static MenuItem checkNull (MenuItem item) { if (item == null) SWT.error (SWT.ERROR_NULL_ARGUMENT); return item; } @Override void checkParent (Widget parent) { // A null parent is okay when the app menu bar is in use. if (parent == null && Display.getDefault().appMenuBar == null) error (SWT.ERROR_NULL_ARGUMENT); if (parent != null) { if (parent.isDisposed ()) error (SWT.ERROR_INVALID_ARGUMENT); parent.checkWidget (); parent.checkOpen (); } } static int checkStyle (int style) { return checkBits (style, SWT.POP_UP, SWT.BAR, SWT.DROP_DOWN, 0, 0, 0); } void _setVisible (boolean visible) { if ((style & (SWT.BAR | SWT.DROP_DOWN)) != 0) return; TrayItem trayItem = display.currentTrayItem; if (trayItem != null && visible) { trayItem.showMenu (this); return; } if (visible) { Shell shell = getShell (); NSWindow window = shell.view.window (); NSPoint location = null; if (hasLocation) { NSView topView = window.contentView(); Point shellCoord = display.map(null, shell, new Point(x,y)); location = new NSPoint (); location.x = shellCoord.x; location.y = topView.frame().height - shellCoord.y; } else { location = window.mouseLocationOutsideOfEventStream(); } hasLocation = false; // Hold on to window in case it is disposed while the popup is open. window.retain(); // NSMenu processes events on its own while the popup is open. display.sendPreExternalEventDispatchEvent (); NSEvent nsEvent = NSEvent.otherEventWithType(OS.NSApplicationDefined, location, 0, 0.0, window.windowNumber(), window.graphicsContext(), (short)0, 0, 0); NSMenu.popUpContextMenu(nsMenu, nsEvent, shell.view); display.sendPostExternalEventDispatchEvent (); window.release(); } else { nsMenu.cancelTracking (); } } /** * Adds the listener to the collection of listeners who will * be notified when help events are generated for the control, * by sending it one of the messages defined in the *- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the parent
*- ERROR_INVALID_SUBCLASS - if this class is not an allowed subclass
*HelpListener
interface. * * @param listener the listener which should be notified * * @exception IllegalArgumentException*
* @exception SWTException- ERROR_NULL_ARGUMENT - if the listener is null
**
* * @see HelpListener * @see #removeHelpListener */ public void addHelpListener (HelpListener listener) { checkWidget (); if (listener == null) error (SWT.ERROR_NULL_ARGUMENT); TypedListener typedListener = new TypedListener (listener); addListener (SWT.Help, typedListener); } /** * Adds the listener to the collection of listeners who will * be notified when menus are hidden or shown, by sending it * one of the messages defined in the- ERROR_WIDGET_DISPOSED - if the receiver has been disposed
*- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver
*MenuListener
* interface. * * @param listener the listener which should be notified * * @exception IllegalArgumentException*
* @exception SWTException- ERROR_NULL_ARGUMENT - if the listener is null
**
* * @see MenuListener * @see #removeMenuListener */ public void addMenuListener (MenuListener listener) { checkWidget (); if (listener == null) error (SWT.ERROR_NULL_ARGUMENT); TypedListener typedListener = new TypedListener (listener); addListener (SWT.Hide,typedListener); addListener (SWT.Show,typedListener); } @Override void createHandle () { display.addMenu (this); if (nsMenu == null) { NSMenu widget = (NSMenu)new SWTMenu().alloc(); widget = widget.initWithTitle(NSString.string()); widget.setAutoenablesItems(false); widget.setDelegate(widget); nsMenu = widget; } else { nsMenu.retain(); long /*int*/ cls = OS.object_getClass(nsMenu.id); long /*int*/ dynNSMenu_class = display.createMenuSubclass(cls, "SWTSystemMenu", true); if (cls != dynNSMenu_class) { OS.object_setClass(nsMenu.id, dynNSMenu_class); } nsMenu.setDelegate(nsMenu); } } void createItem (MenuItem item, int index) { if (!(0 <= index && index <= itemCount)) error (SWT.ERROR_INVALID_RANGE); boolean add = true; NSMenuItem nsItem = item.nsItem; if (nsItem == null) { if ((item.style & SWT.SEPARATOR) != 0) { nsItem = NSMenuItem.separatorItem(); nsItem.retain(); } else { nsItem = (NSMenuItem)new SWTMenuItem().alloc(); NSString empty = NSString.string(); nsItem.initWithTitle(empty, 0, empty); nsItem.setTarget(nsItem); nsItem.setAction(OS.sel_sendSelection); } item.nsItem = nsItem; } else { long /*int*/ cls = OS.object_getClass(nsItem.id); long /*int*/ dynNSMenuItem_class = display.createMenuItemSubclass(cls, "SWTSystemMenuItem", true); if (cls != dynNSMenuItem_class) { OS.object_setClass(nsItem.id, dynNSMenuItem_class); } nsItem.retain(); item.nsItemAction = nsItem.action(); item.nsItemTarget = nsItem.target(); nsItem.setTarget(nsItem); nsItem.setAction(OS.sel_sendSelection); // Sync native item type to Item's style. int type = SWT.PUSH; if (nsItem.isSeparatorItem()) type = SWT.SEPARATOR; if (nsItem.submenu() != null) type = SWT.CASCADE; item.style |= type; // Sync native item text to Item's text. item.text = nsItem.title().getString(); // Sync native key equivalent to MenuItem's accelerator. // The system menu on OS X only uses command and option, so it's // safe to just check for those two key masks. long /*int*/ keyMask = nsItem.keyEquivalentModifierMask(); NSString keyEquivString = nsItem.keyEquivalent(); long /*int*/ keyEquiv = 0; if (keyEquivString != null) { keyEquiv = keyEquivString.characterAtIndex(0); if ((keyMask & OS.NSCommandKeyMask) != 0) keyEquiv |= SWT.COMMAND; if ((keyMask & OS.NSAlternateKeyMask) != 0) keyEquiv |= SWT.ALT; item.accelerator = (int) keyEquiv; } add = false; } item.createJNIRef(); item.register(); if (add) { nsMenu.insertItem(nsItem, index); } if (itemCount == items.length) { MenuItem [] newItems = new MenuItem [items.length + 4]; System.arraycopy (items, 0, newItems, 0, items.length); items = newItems; } System.arraycopy (items, index, items, index + 1, itemCount++ - index); items [index] = item; if (add) { NSMenu emptyMenu = item.createEmptyMenu (); if (emptyMenu != null) { nsItem.setSubmenu (emptyMenu); emptyMenu.release(); } if (display.menuBar == this) { NSApplication application = display.application; NSMenu menubar = application.mainMenu(); if (menubar != null) { nsItem.setMenu(null); menubar.insertItem(nsItem, index + 1); } } } //TODO - find a way to disable the menu instead of each item if (!getEnabled ()) nsItem.setEnabled (false); } @Override void createWidget () { checkOrientation (parent); super.createWidget (); items = new MenuItem [4]; } @Override void deregister () { super.deregister (); display.removeWidget (nsMenu); } void destroyItem (MenuItem item) { int index = 0; while (index < itemCount) { if (items [index] == item) break; index++; } if (index == itemCount) return; System.arraycopy (items, index + 1, items, index, --itemCount - index); items [itemCount] = null; if (itemCount == 0) items = new MenuItem [4]; nsMenu.removeItem (item.nsItem); if (display.menuBar == this) { NSApplication application = display.application; NSMenu menubar = application.mainMenu(); if (menubar != null) { NSMenuItem nsItem = item.nsItem; menubar.removeItem(nsItem); } } } void fixMenus (Decorations newParent) { this.parent = newParent; } /** * Returns the default menu item or null if none has * been previously set. * * @return the default menu item. * * * @exception SWTException- ERROR_WIDGET_DISPOSED - if the receiver has been disposed
*- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver
**
*/ public MenuItem getDefaultItem () { checkWidget(); return defaultItem; } /** * Returns- ERROR_WIDGET_DISPOSED - if the receiver has been disposed
*- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver
*true
if the receiver is enabled, and *false
otherwise. A disabled menu is typically * not selectable from the user interface and draws with an * inactive or "grayed" look. * * @return the receiver's enabled state * * @exception SWTException*
* * @see #isEnabled */ public boolean getEnabled () { checkWidget(); return (state & DISABLED) == 0; } /** * Returns the item at the given, zero-relative index in the * receiver. Throws an exception if the index is out of range. * * @param index the index of the item to return * @return the item at the given index * * @exception IllegalArgumentException- ERROR_WIDGET_DISPOSED - if the receiver has been disposed
*- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver
**
* @exception SWTException- ERROR_INVALID_RANGE - if the index is not between 0 and the number of elements in the list minus 1 (inclusive)
**
*/ public MenuItem getItem (int index) { checkWidget (); if (!(0 <= index && index < itemCount)) error (SWT.ERROR_INVALID_RANGE); return items [index]; } /** * Returns the number of items contained in the receiver. * * @return the number of items * * @exception SWTException- ERROR_WIDGET_DISPOSED - if the receiver has been disposed
*- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver
**
*/ public int getItemCount () { checkWidget (); return itemCount; } /** * Returns a (possibly empty) array of- ERROR_WIDGET_DISPOSED - if the receiver has been disposed
*- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver
*MenuItem
s which * are the items in the receiver. ** Note: This is not the actual structure used by the receiver * to maintain its list of items, so modifying the array will * not affect the receiver. *
* * @return the items in the receiver * * @exception SWTException*
*/ public MenuItem [] getItems () { checkWidget (); MenuItem [] result = new MenuItem [itemCount]; int index = 0; if (items != null) { for (int i = 0; i < itemCount; i++) { MenuItem item = items [i]; if (item != null && !item.isDisposed ()) { result [index++] = item; } } } if (index != result.length) { MenuItem [] newItems = new MenuItem[index]; System.arraycopy(result, 0, newItems, 0, index); result = newItems; } return result; } @Override String getNameText () { String result = ""; MenuItem [] items = getItems (); int length = items.length; if (length > 0) { for (int i=0; i- ERROR_WIDGET_DISPOSED - if the receiver has been disposed
*- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver
*SWT.LEFT_TO_RIGHT SWT.RIGHT_TO_LEFT
. * * @return the orientation style * * @exception SWTException
-
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
Decorations
.
*
* @return the receiver's parent
*
* @exception SWTException -
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
MenuItem
or null when the receiver is a
* root.
*
* @return the receiver's parent item
*
* @exception SWTException -
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
Menu
or null when the receiver is a
* root.
*
* @return the receiver's parent item
*
* @exception SWTException -
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
-
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
true
if the receiver is visible, and
* false
otherwise.
* * If one of the receiver's ancestors is not visible or some * other condition makes the receiver not visible, this method * may still indicate that it is considered visible even though * it may not actually be showing. *
* * @return the receiver's visibility state * * @exception SWTException-
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
-
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
false
* otherwise. A disabled menu is typically not selectable from the
* user interface and draws with an inactive or "grayed" look.
*
* @return the receiver's enabled state
*
* @exception SWTException -
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
true
if the receiver is visible and all
* of the receiver's ancestors are visible and false
* otherwise.
*
* @return the receiver's visibility state
*
* @exception SWTException -
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
-
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
-
*
- ERROR_NULL_ARGUMENT - if the listener is null *
-
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
-
*
- ERROR_INVALID_ARGUMENT - if the menu item has been disposed *
-
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
true
,
* and disables it otherwise. A disabled menu is typically
* not selectable from the user interface and draws with an
* inactive or "grayed" look.
*
* @param enabled the new enabled state
*
* @exception SWTException -
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
* Note that the platform window manager ultimately has control * over the location of popup menus. *
* * @param x the new x coordinate for the receiver * @param y the new y coordinate for the receiver * * @exception SWTException-
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
* Note that this is different from most widgets where the * location of the widget is relative to the parent. *
* Note that the platform window manager ultimately has control * over the location of popup menus. *
* * @param location the new location for the receiver * * @exception IllegalArgumentException-
*
- ERROR_NULL_ARGUMENT - if the point is null *
-
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
SWT.LEFT_TO_RIGHT
or SWT.RIGHT_TO_LEFT
.
* * * @param orientation new orientation style * * @exception SWTException
-
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *
true
,
* and marks it invisible otherwise.
* * If one of the receiver's ancestors is not visible or some * other condition makes the receiver not visible, marking * it visible may not actually cause it to be displayed. *
* * @param visible the new visibility state * * @exception SWTException-
*
- ERROR_WIDGET_DISPOSED - if the receiver has been disposed *
- ERROR_THREAD_INVALID_ACCESS - if not called from the thread that created the receiver *