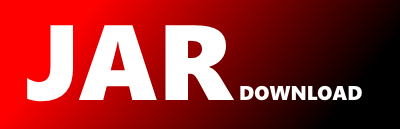
com.eclipsesource.restfuse.Destination Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of com.eclipsesource.restfuse Show documentation
Show all versions of com.eclipsesource.restfuse Show documentation
An open-source JUnit extension for automated HTTP/REST Tests
/*******************************************************************************
* Copyright (c) 2011 EclipseSource and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* Holger Staudacher - initial API and implementation
******************************************************************************/
package com.eclipsesource.restfuse;
import java.net.MalformedURLException;
import java.net.URL;
import org.junit.rules.MethodRule;
import org.junit.runners.model.FrameworkMethod;
import org.junit.runners.model.Statement;
import com.eclipsesource.restfuse.annotation.Callback;
import com.eclipsesource.restfuse.annotation.HttpTest;
import com.eclipsesource.restfuse.internal.HttpTestStatement;
/**
* A Destination
marks a requirement for http tests. Before you can use the
* {@link HttpTest}
annotation you need to instantiate a Destination
in
* your TestCase. This rule does manage the {@link HttpTest}
and the
* {@link Callback}
annotations of your test methods.
*
* A Destination
needs an url during instantiation. This url will be used within a
* TestCase to send requests to. If the url is not valid an IllegalArgumentException
* will be thrown.
*
* A simple test looks like this:
*
* @RunWith( HttpJUnitRunner.class )
* public class Example {
*
* @Rule
* public Destination destination = new Destination( "http://localhost" );
*
* @Context
* private Response response;
*
* @HttpTest( method = Method.GET, path = "/test" )
* public void testMethod() {
* com.eclipsesource.restfuse.Assert.assertAccepted( response );
* }
* }
*
*
*
* @see HttpTest
* @see Callback
*/
public class Destination implements MethodRule {
private HttpTestStatement requestStatement;
private final String baseUrl;
/**
* Constructs a new Destination
object. An url is needed as parameter which will
* be used in the whole test to send requests to.
*
* @param baseUrl The url to send requests to.
*
* @throws IllegalArgumentException Will be thrown when the baseUrl
is null or
* not a valid url.
*/
public Destination( String baseUrl ) {
checkBaseUrl( baseUrl );
this.baseUrl = baseUrl;
}
private void checkBaseUrl( String baseUrl ) {
if( baseUrl == null ) {
throw new IllegalArgumentException( "baseUrl must not be null" );
}
try {
new URL( baseUrl );
} catch( MalformedURLException mue ) {
throw new IllegalArgumentException( "baseUrl has to be an URL" );
}
}
@Override
/**
* Not meant for public use. This method will be invoked by the JUnit framework.
*/
public Statement apply( Statement base, FrameworkMethod method, Object target )
{
Statement result;
if( hasAnnotation( method ) ) {
requestStatement = new HttpTestStatement( base, method, target, baseUrl );
result = requestStatement;
} else {
result = base;
}
return result;
}
private boolean hasAnnotation( FrameworkMethod method ) {
return method.getAnnotation( HttpTest.class ) != null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy