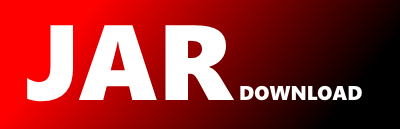
com.rhythm.pb.RequestProtos Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: louie/request.proto
package com.rhythm.pb;
public final class RequestProtos {
private RequestProtos() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
}
public interface RequestHeaderPBOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional string user = 1 [deprecated = true];
/**
* optional string user = 1 [deprecated = true];
*/
@java.lang.Deprecated boolean hasUser();
/**
* optional string user = 1 [deprecated = true];
*/
@java.lang.Deprecated java.lang.String getUser();
/**
* optional string user = 1 [deprecated = true];
*/
@java.lang.Deprecated com.google.protobuf.ByteString
getUserBytes();
// optional string agent = 2;
/**
* optional string agent = 2;
*/
boolean hasAgent();
/**
* optional string agent = 2;
*/
java.lang.String getAgent();
/**
* optional string agent = 2;
*/
com.google.protobuf.ByteString
getAgentBytes();
// optional uint32 count = 3;
/**
* optional uint32 count = 3;
*/
boolean hasCount();
/**
* optional uint32 count = 3;
*/
int getCount();
// optional .louie.SessionKey key = 10;
/**
* optional .louie.SessionKey key = 10;
*/
boolean hasKey();
/**
* optional .louie.SessionKey key = 10;
*/
com.rhythm.pb.RequestProtos.SessionKey getKey();
/**
* optional .louie.SessionKey key = 10;
*/
com.rhythm.pb.RequestProtos.SessionKeyOrBuilder getKeyOrBuilder();
// optional .louie.IdentityPB identity = 11;
/**
* optional .louie.IdentityPB identity = 11;
*
*
* set on initial request, thereafter requests should use the key that would have come back in the initial ResponsePB
*
*/
boolean hasIdentity();
/**
* optional .louie.IdentityPB identity = 11;
*
*
* set on initial request, thereafter requests should use the key that would have come back in the initial ResponsePB
*
*/
com.rhythm.pb.RequestProtos.IdentityPB getIdentity();
/**
* optional .louie.IdentityPB identity = 11;
*
*
* set on initial request, thereafter requests should use the key that would have come back in the initial ResponsePB
*
*/
com.rhythm.pb.RequestProtos.IdentityPBOrBuilder getIdentityOrBuilder();
// repeated .louie.RoutePB route = 20 [deprecated = true];
/**
* repeated .louie.RoutePB route = 20 [deprecated = true];
*/
@java.lang.Deprecated java.util.List
getRouteList();
/**
* repeated .louie.RoutePB route = 20 [deprecated = true];
*/
@java.lang.Deprecated com.rhythm.pb.RequestProtos.RoutePB getRoute(int index);
/**
* repeated .louie.RoutePB route = 20 [deprecated = true];
*/
@java.lang.Deprecated int getRouteCount();
/**
* repeated .louie.RoutePB route = 20 [deprecated = true];
*/
@java.lang.Deprecated java.util.List extends com.rhythm.pb.RequestProtos.RoutePBOrBuilder>
getRouteOrBuilderList();
/**
* repeated .louie.RoutePB route = 20 [deprecated = true];
*/
@java.lang.Deprecated com.rhythm.pb.RequestProtos.RoutePBOrBuilder getRouteOrBuilder(
int index);
// optional string routeUser = 30 [deprecated = true];
/**
* optional string routeUser = 30 [deprecated = true];
*/
@java.lang.Deprecated boolean hasRouteUser();
/**
* optional string routeUser = 30 [deprecated = true];
*/
@java.lang.Deprecated java.lang.String getRouteUser();
/**
* optional string routeUser = 30 [deprecated = true];
*/
@java.lang.Deprecated com.google.protobuf.ByteString
getRouteUserBytes();
}
/**
* Protobuf type {@code louie.RequestHeaderPB}
*/
public static final class RequestHeaderPB extends
com.google.protobuf.GeneratedMessage
implements RequestHeaderPBOrBuilder {
// Use RequestHeaderPB.newBuilder() to construct.
private RequestHeaderPB(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private RequestHeaderPB(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final RequestHeaderPB defaultInstance;
public static RequestHeaderPB getDefaultInstance() {
return defaultInstance;
}
public RequestHeaderPB getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RequestHeaderPB(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
user_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
agent_ = input.readBytes();
break;
}
case 24: {
bitField0_ |= 0x00000004;
count_ = input.readUInt32();
break;
}
case 82: {
com.rhythm.pb.RequestProtos.SessionKey.Builder subBuilder = null;
if (((bitField0_ & 0x00000008) == 0x00000008)) {
subBuilder = key_.toBuilder();
}
key_ = input.readMessage(com.rhythm.pb.RequestProtos.SessionKey.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(key_);
key_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000008;
break;
}
case 90: {
com.rhythm.pb.RequestProtos.IdentityPB.Builder subBuilder = null;
if (((bitField0_ & 0x00000010) == 0x00000010)) {
subBuilder = identity_.toBuilder();
}
identity_ = input.readMessage(com.rhythm.pb.RequestProtos.IdentityPB.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(identity_);
identity_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000010;
break;
}
case 162: {
if (!((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
route_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000020;
}
route_.add(input.readMessage(com.rhythm.pb.RequestProtos.RoutePB.PARSER, extensionRegistry));
break;
}
case 242: {
bitField0_ |= 0x00000020;
routeUser_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
route_ = java.util.Collections.unmodifiableList(route_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.rhythm.pb.RequestProtos.internal_static_louie_RequestHeaderPB_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.rhythm.pb.RequestProtos.internal_static_louie_RequestHeaderPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.rhythm.pb.RequestProtos.RequestHeaderPB.class, com.rhythm.pb.RequestProtos.RequestHeaderPB.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public RequestHeaderPB parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RequestHeaderPB(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional string user = 1 [deprecated = true];
public static final int USER_FIELD_NUMBER = 1;
private java.lang.Object user_;
/**
* optional string user = 1 [deprecated = true];
*/
@java.lang.Deprecated public boolean hasUser() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string user = 1 [deprecated = true];
*/
@java.lang.Deprecated public java.lang.String getUser() {
java.lang.Object ref = user_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
user_ = s;
}
return s;
}
}
/**
* optional string user = 1 [deprecated = true];
*/
@java.lang.Deprecated public com.google.protobuf.ByteString
getUserBytes() {
java.lang.Object ref = user_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
user_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string agent = 2;
public static final int AGENT_FIELD_NUMBER = 2;
private java.lang.Object agent_;
/**
* optional string agent = 2;
*/
public boolean hasAgent() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string agent = 2;
*/
public java.lang.String getAgent() {
java.lang.Object ref = agent_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
agent_ = s;
}
return s;
}
}
/**
* optional string agent = 2;
*/
public com.google.protobuf.ByteString
getAgentBytes() {
java.lang.Object ref = agent_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
agent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional uint32 count = 3;
public static final int COUNT_FIELD_NUMBER = 3;
private int count_;
/**
* optional uint32 count = 3;
*/
public boolean hasCount() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional uint32 count = 3;
*/
public int getCount() {
return count_;
}
// optional .louie.SessionKey key = 10;
public static final int KEY_FIELD_NUMBER = 10;
private com.rhythm.pb.RequestProtos.SessionKey key_;
/**
* optional .louie.SessionKey key = 10;
*/
public boolean hasKey() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .louie.SessionKey key = 10;
*/
public com.rhythm.pb.RequestProtos.SessionKey getKey() {
return key_;
}
/**
* optional .louie.SessionKey key = 10;
*/
public com.rhythm.pb.RequestProtos.SessionKeyOrBuilder getKeyOrBuilder() {
return key_;
}
// optional .louie.IdentityPB identity = 11;
public static final int IDENTITY_FIELD_NUMBER = 11;
private com.rhythm.pb.RequestProtos.IdentityPB identity_;
/**
* optional .louie.IdentityPB identity = 11;
*
*
* set on initial request, thereafter requests should use the key that would have come back in the initial ResponsePB
*
*/
public boolean hasIdentity() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional .louie.IdentityPB identity = 11;
*
*
* set on initial request, thereafter requests should use the key that would have come back in the initial ResponsePB
*
*/
public com.rhythm.pb.RequestProtos.IdentityPB getIdentity() {
return identity_;
}
/**
* optional .louie.IdentityPB identity = 11;
*
*
* set on initial request, thereafter requests should use the key that would have come back in the initial ResponsePB
*
*/
public com.rhythm.pb.RequestProtos.IdentityPBOrBuilder getIdentityOrBuilder() {
return identity_;
}
// repeated .louie.RoutePB route = 20 [deprecated = true];
public static final int ROUTE_FIELD_NUMBER = 20;
private java.util.List route_;
/**
* repeated .louie.RoutePB route = 20 [deprecated = true];
*/
@java.lang.Deprecated public java.util.List getRouteList() {
return route_;
}
/**
* repeated .louie.RoutePB route = 20 [deprecated = true];
*/
@java.lang.Deprecated public java.util.List extends com.rhythm.pb.RequestProtos.RoutePBOrBuilder>
getRouteOrBuilderList() {
return route_;
}
/**
* repeated .louie.RoutePB route = 20 [deprecated = true];
*/
@java.lang.Deprecated public int getRouteCount() {
return route_.size();
}
/**
* repeated .louie.RoutePB route = 20 [deprecated = true];
*/
@java.lang.Deprecated public com.rhythm.pb.RequestProtos.RoutePB getRoute(int index) {
return route_.get(index);
}
/**
* repeated .louie.RoutePB route = 20 [deprecated = true];
*/
@java.lang.Deprecated public com.rhythm.pb.RequestProtos.RoutePBOrBuilder getRouteOrBuilder(
int index) {
return route_.get(index);
}
// optional string routeUser = 30 [deprecated = true];
public static final int ROUTEUSER_FIELD_NUMBER = 30;
private java.lang.Object routeUser_;
/**
* optional string routeUser = 30 [deprecated = true];
*/
@java.lang.Deprecated public boolean hasRouteUser() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string routeUser = 30 [deprecated = true];
*/
@java.lang.Deprecated public java.lang.String getRouteUser() {
java.lang.Object ref = routeUser_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
routeUser_ = s;
}
return s;
}
}
/**
* optional string routeUser = 30 [deprecated = true];
*/
@java.lang.Deprecated public com.google.protobuf.ByteString
getRouteUserBytes() {
java.lang.Object ref = routeUser_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
routeUser_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
user_ = "";
agent_ = "";
count_ = 0;
key_ = com.rhythm.pb.RequestProtos.SessionKey.getDefaultInstance();
identity_ = com.rhythm.pb.RequestProtos.IdentityPB.getDefaultInstance();
route_ = java.util.Collections.emptyList();
routeUser_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getUserBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getAgentBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeUInt32(3, count_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeMessage(10, key_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeMessage(11, identity_);
}
for (int i = 0; i < route_.size(); i++) {
output.writeMessage(20, route_.get(i));
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeBytes(30, getRouteUserBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getUserBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getAgentBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(3, count_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, key_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, identity_);
}
for (int i = 0; i < route_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(20, route_.get(i));
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(30, getRouteUserBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.rhythm.pb.RequestProtos.RequestHeaderPB)) {
return super.equals(obj);
}
com.rhythm.pb.RequestProtos.RequestHeaderPB other = (com.rhythm.pb.RequestProtos.RequestHeaderPB) obj;
boolean result = true;
result = result && (hasUser() == other.hasUser());
if (hasUser()) {
result = result && getUser()
.equals(other.getUser());
}
result = result && (hasAgent() == other.hasAgent());
if (hasAgent()) {
result = result && getAgent()
.equals(other.getAgent());
}
result = result && (hasCount() == other.hasCount());
if (hasCount()) {
result = result && (getCount()
== other.getCount());
}
result = result && (hasKey() == other.hasKey());
if (hasKey()) {
result = result && getKey()
.equals(other.getKey());
}
result = result && (hasIdentity() == other.hasIdentity());
if (hasIdentity()) {
result = result && getIdentity()
.equals(other.getIdentity());
}
result = result && getRouteList()
.equals(other.getRouteList());
result = result && (hasRouteUser() == other.hasRouteUser());
if (hasRouteUser()) {
result = result && getRouteUser()
.equals(other.getRouteUser());
}
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasUser()) {
hash = (37 * hash) + USER_FIELD_NUMBER;
hash = (53 * hash) + getUser().hashCode();
}
if (hasAgent()) {
hash = (37 * hash) + AGENT_FIELD_NUMBER;
hash = (53 * hash) + getAgent().hashCode();
}
if (hasCount()) {
hash = (37 * hash) + COUNT_FIELD_NUMBER;
hash = (53 * hash) + getCount();
}
if (hasKey()) {
hash = (37 * hash) + KEY_FIELD_NUMBER;
hash = (53 * hash) + getKey().hashCode();
}
if (hasIdentity()) {
hash = (37 * hash) + IDENTITY_FIELD_NUMBER;
hash = (53 * hash) + getIdentity().hashCode();
}
if (getRouteCount() > 0) {
hash = (37 * hash) + ROUTE_FIELD_NUMBER;
hash = (53 * hash) + getRouteList().hashCode();
}
if (hasRouteUser()) {
hash = (37 * hash) + ROUTEUSER_FIELD_NUMBER;
hash = (53 * hash) + getRouteUser().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.rhythm.pb.RequestProtos.RequestHeaderPB parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.rhythm.pb.RequestProtos.RequestHeaderPB parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.RequestHeaderPB parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.rhythm.pb.RequestProtos.RequestHeaderPB parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.RequestHeaderPB parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.rhythm.pb.RequestProtos.RequestHeaderPB parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.RequestHeaderPB parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.rhythm.pb.RequestProtos.RequestHeaderPB parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.RequestHeaderPB parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.rhythm.pb.RequestProtos.RequestHeaderPB parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.rhythm.pb.RequestProtos.RequestHeaderPB prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code louie.RequestHeaderPB}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.rhythm.pb.RequestProtos.RequestHeaderPBOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.rhythm.pb.RequestProtos.internal_static_louie_RequestHeaderPB_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.rhythm.pb.RequestProtos.internal_static_louie_RequestHeaderPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.rhythm.pb.RequestProtos.RequestHeaderPB.class, com.rhythm.pb.RequestProtos.RequestHeaderPB.Builder.class);
}
// Construct using com.rhythm.pb.RequestProtos.RequestHeaderPB.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getKeyFieldBuilder();
getIdentityFieldBuilder();
getRouteFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
user_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
agent_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
count_ = 0;
bitField0_ = (bitField0_ & ~0x00000004);
if (keyBuilder_ == null) {
key_ = com.rhythm.pb.RequestProtos.SessionKey.getDefaultInstance();
} else {
keyBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
if (identityBuilder_ == null) {
identity_ = com.rhythm.pb.RequestProtos.IdentityPB.getDefaultInstance();
} else {
identityBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
if (routeBuilder_ == null) {
route_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
} else {
routeBuilder_.clear();
}
routeUser_ = "";
bitField0_ = (bitField0_ & ~0x00000040);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.rhythm.pb.RequestProtos.internal_static_louie_RequestHeaderPB_descriptor;
}
public com.rhythm.pb.RequestProtos.RequestHeaderPB getDefaultInstanceForType() {
return com.rhythm.pb.RequestProtos.RequestHeaderPB.getDefaultInstance();
}
public com.rhythm.pb.RequestProtos.RequestHeaderPB build() {
com.rhythm.pb.RequestProtos.RequestHeaderPB result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.rhythm.pb.RequestProtos.RequestHeaderPB buildPartial() {
com.rhythm.pb.RequestProtos.RequestHeaderPB result = new com.rhythm.pb.RequestProtos.RequestHeaderPB(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.user_ = user_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.agent_ = agent_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.count_ = count_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
if (keyBuilder_ == null) {
result.key_ = key_;
} else {
result.key_ = keyBuilder_.build();
}
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
if (identityBuilder_ == null) {
result.identity_ = identity_;
} else {
result.identity_ = identityBuilder_.build();
}
if (routeBuilder_ == null) {
if (((bitField0_ & 0x00000020) == 0x00000020)) {
route_ = java.util.Collections.unmodifiableList(route_);
bitField0_ = (bitField0_ & ~0x00000020);
}
result.route_ = route_;
} else {
result.route_ = routeBuilder_.build();
}
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000020;
}
result.routeUser_ = routeUser_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.rhythm.pb.RequestProtos.RequestHeaderPB) {
return mergeFrom((com.rhythm.pb.RequestProtos.RequestHeaderPB)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.rhythm.pb.RequestProtos.RequestHeaderPB other) {
if (other == com.rhythm.pb.RequestProtos.RequestHeaderPB.getDefaultInstance()) return this;
if (other.hasUser()) {
bitField0_ |= 0x00000001;
user_ = other.user_;
onChanged();
}
if (other.hasAgent()) {
bitField0_ |= 0x00000002;
agent_ = other.agent_;
onChanged();
}
if (other.hasCount()) {
setCount(other.getCount());
}
if (other.hasKey()) {
mergeKey(other.getKey());
}
if (other.hasIdentity()) {
mergeIdentity(other.getIdentity());
}
if (routeBuilder_ == null) {
if (!other.route_.isEmpty()) {
if (route_.isEmpty()) {
route_ = other.route_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensureRouteIsMutable();
route_.addAll(other.route_);
}
onChanged();
}
} else {
if (!other.route_.isEmpty()) {
if (routeBuilder_.isEmpty()) {
routeBuilder_.dispose();
routeBuilder_ = null;
route_ = other.route_;
bitField0_ = (bitField0_ & ~0x00000020);
routeBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getRouteFieldBuilder() : null;
} else {
routeBuilder_.addAllMessages(other.route_);
}
}
}
if (other.hasRouteUser()) {
bitField0_ |= 0x00000040;
routeUser_ = other.routeUser_;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.rhythm.pb.RequestProtos.RequestHeaderPB parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.rhythm.pb.RequestProtos.RequestHeaderPB) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional string user = 1 [deprecated = true];
private java.lang.Object user_ = "";
/**
* optional string user = 1 [deprecated = true];
*/
@java.lang.Deprecated public boolean hasUser() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string user = 1 [deprecated = true];
*/
@java.lang.Deprecated public java.lang.String getUser() {
java.lang.Object ref = user_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
user_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string user = 1 [deprecated = true];
*/
@java.lang.Deprecated public com.google.protobuf.ByteString
getUserBytes() {
java.lang.Object ref = user_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
user_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string user = 1 [deprecated = true];
*/
@java.lang.Deprecated public Builder setUser(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
user_ = value;
onChanged();
return this;
}
/**
* optional string user = 1 [deprecated = true];
*/
@java.lang.Deprecated public Builder clearUser() {
bitField0_ = (bitField0_ & ~0x00000001);
user_ = getDefaultInstance().getUser();
onChanged();
return this;
}
/**
* optional string user = 1 [deprecated = true];
*/
@java.lang.Deprecated public Builder setUserBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
user_ = value;
onChanged();
return this;
}
// optional string agent = 2;
private java.lang.Object agent_ = "";
/**
* optional string agent = 2;
*/
public boolean hasAgent() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string agent = 2;
*/
public java.lang.String getAgent() {
java.lang.Object ref = agent_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
agent_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string agent = 2;
*/
public com.google.protobuf.ByteString
getAgentBytes() {
java.lang.Object ref = agent_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
agent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string agent = 2;
*/
public Builder setAgent(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
agent_ = value;
onChanged();
return this;
}
/**
* optional string agent = 2;
*/
public Builder clearAgent() {
bitField0_ = (bitField0_ & ~0x00000002);
agent_ = getDefaultInstance().getAgent();
onChanged();
return this;
}
/**
* optional string agent = 2;
*/
public Builder setAgentBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
agent_ = value;
onChanged();
return this;
}
// optional uint32 count = 3;
private int count_ ;
/**
* optional uint32 count = 3;
*/
public boolean hasCount() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional uint32 count = 3;
*/
public int getCount() {
return count_;
}
/**
* optional uint32 count = 3;
*/
public Builder setCount(int value) {
bitField0_ |= 0x00000004;
count_ = value;
onChanged();
return this;
}
/**
* optional uint32 count = 3;
*/
public Builder clearCount() {
bitField0_ = (bitField0_ & ~0x00000004);
count_ = 0;
onChanged();
return this;
}
// optional .louie.SessionKey key = 10;
private com.rhythm.pb.RequestProtos.SessionKey key_ = com.rhythm.pb.RequestProtos.SessionKey.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.rhythm.pb.RequestProtos.SessionKey, com.rhythm.pb.RequestProtos.SessionKey.Builder, com.rhythm.pb.RequestProtos.SessionKeyOrBuilder> keyBuilder_;
/**
* optional .louie.SessionKey key = 10;
*/
public boolean hasKey() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .louie.SessionKey key = 10;
*/
public com.rhythm.pb.RequestProtos.SessionKey getKey() {
if (keyBuilder_ == null) {
return key_;
} else {
return keyBuilder_.getMessage();
}
}
/**
* optional .louie.SessionKey key = 10;
*/
public Builder setKey(com.rhythm.pb.RequestProtos.SessionKey value) {
if (keyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
key_ = value;
onChanged();
} else {
keyBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .louie.SessionKey key = 10;
*/
public Builder setKey(
com.rhythm.pb.RequestProtos.SessionKey.Builder builderForValue) {
if (keyBuilder_ == null) {
key_ = builderForValue.build();
onChanged();
} else {
keyBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .louie.SessionKey key = 10;
*/
public Builder mergeKey(com.rhythm.pb.RequestProtos.SessionKey value) {
if (keyBuilder_ == null) {
if (((bitField0_ & 0x00000008) == 0x00000008) &&
key_ != com.rhythm.pb.RequestProtos.SessionKey.getDefaultInstance()) {
key_ =
com.rhythm.pb.RequestProtos.SessionKey.newBuilder(key_).mergeFrom(value).buildPartial();
} else {
key_ = value;
}
onChanged();
} else {
keyBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .louie.SessionKey key = 10;
*/
public Builder clearKey() {
if (keyBuilder_ == null) {
key_ = com.rhythm.pb.RequestProtos.SessionKey.getDefaultInstance();
onChanged();
} else {
keyBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
/**
* optional .louie.SessionKey key = 10;
*/
public com.rhythm.pb.RequestProtos.SessionKey.Builder getKeyBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getKeyFieldBuilder().getBuilder();
}
/**
* optional .louie.SessionKey key = 10;
*/
public com.rhythm.pb.RequestProtos.SessionKeyOrBuilder getKeyOrBuilder() {
if (keyBuilder_ != null) {
return keyBuilder_.getMessageOrBuilder();
} else {
return key_;
}
}
/**
* optional .louie.SessionKey key = 10;
*/
private com.google.protobuf.SingleFieldBuilder<
com.rhythm.pb.RequestProtos.SessionKey, com.rhythm.pb.RequestProtos.SessionKey.Builder, com.rhythm.pb.RequestProtos.SessionKeyOrBuilder>
getKeyFieldBuilder() {
if (keyBuilder_ == null) {
keyBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.rhythm.pb.RequestProtos.SessionKey, com.rhythm.pb.RequestProtos.SessionKey.Builder, com.rhythm.pb.RequestProtos.SessionKeyOrBuilder>(
key_,
getParentForChildren(),
isClean());
key_ = null;
}
return keyBuilder_;
}
// optional .louie.IdentityPB identity = 11;
private com.rhythm.pb.RequestProtos.IdentityPB identity_ = com.rhythm.pb.RequestProtos.IdentityPB.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.rhythm.pb.RequestProtos.IdentityPB, com.rhythm.pb.RequestProtos.IdentityPB.Builder, com.rhythm.pb.RequestProtos.IdentityPBOrBuilder> identityBuilder_;
/**
* optional .louie.IdentityPB identity = 11;
*
*
* set on initial request, thereafter requests should use the key that would have come back in the initial ResponsePB
*
*/
public boolean hasIdentity() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional .louie.IdentityPB identity = 11;
*
*
* set on initial request, thereafter requests should use the key that would have come back in the initial ResponsePB
*
*/
public com.rhythm.pb.RequestProtos.IdentityPB getIdentity() {
if (identityBuilder_ == null) {
return identity_;
} else {
return identityBuilder_.getMessage();
}
}
/**
* optional .louie.IdentityPB identity = 11;
*
*
* set on initial request, thereafter requests should use the key that would have come back in the initial ResponsePB
*
*/
public Builder setIdentity(com.rhythm.pb.RequestProtos.IdentityPB value) {
if (identityBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
identity_ = value;
onChanged();
} else {
identityBuilder_.setMessage(value);
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .louie.IdentityPB identity = 11;
*
*
* set on initial request, thereafter requests should use the key that would have come back in the initial ResponsePB
*
*/
public Builder setIdentity(
com.rhythm.pb.RequestProtos.IdentityPB.Builder builderForValue) {
if (identityBuilder_ == null) {
identity_ = builderForValue.build();
onChanged();
} else {
identityBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .louie.IdentityPB identity = 11;
*
*
* set on initial request, thereafter requests should use the key that would have come back in the initial ResponsePB
*
*/
public Builder mergeIdentity(com.rhythm.pb.RequestProtos.IdentityPB value) {
if (identityBuilder_ == null) {
if (((bitField0_ & 0x00000010) == 0x00000010) &&
identity_ != com.rhythm.pb.RequestProtos.IdentityPB.getDefaultInstance()) {
identity_ =
com.rhythm.pb.RequestProtos.IdentityPB.newBuilder(identity_).mergeFrom(value).buildPartial();
} else {
identity_ = value;
}
onChanged();
} else {
identityBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .louie.IdentityPB identity = 11;
*
*
* set on initial request, thereafter requests should use the key that would have come back in the initial ResponsePB
*
*/
public Builder clearIdentity() {
if (identityBuilder_ == null) {
identity_ = com.rhythm.pb.RequestProtos.IdentityPB.getDefaultInstance();
onChanged();
} else {
identityBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
/**
* optional .louie.IdentityPB identity = 11;
*
*
* set on initial request, thereafter requests should use the key that would have come back in the initial ResponsePB
*
*/
public com.rhythm.pb.RequestProtos.IdentityPB.Builder getIdentityBuilder() {
bitField0_ |= 0x00000010;
onChanged();
return getIdentityFieldBuilder().getBuilder();
}
/**
* optional .louie.IdentityPB identity = 11;
*
*
* set on initial request, thereafter requests should use the key that would have come back in the initial ResponsePB
*
*/
public com.rhythm.pb.RequestProtos.IdentityPBOrBuilder getIdentityOrBuilder() {
if (identityBuilder_ != null) {
return identityBuilder_.getMessageOrBuilder();
} else {
return identity_;
}
}
/**
* optional .louie.IdentityPB identity = 11;
*
*
* set on initial request, thereafter requests should use the key that would have come back in the initial ResponsePB
*
*/
private com.google.protobuf.SingleFieldBuilder<
com.rhythm.pb.RequestProtos.IdentityPB, com.rhythm.pb.RequestProtos.IdentityPB.Builder, com.rhythm.pb.RequestProtos.IdentityPBOrBuilder>
getIdentityFieldBuilder() {
if (identityBuilder_ == null) {
identityBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.rhythm.pb.RequestProtos.IdentityPB, com.rhythm.pb.RequestProtos.IdentityPB.Builder, com.rhythm.pb.RequestProtos.IdentityPBOrBuilder>(
identity_,
getParentForChildren(),
isClean());
identity_ = null;
}
return identityBuilder_;
}
// repeated .louie.RoutePB route = 20 [deprecated = true];
private java.util.List route_ =
java.util.Collections.emptyList();
private void ensureRouteIsMutable() {
if (!((bitField0_ & 0x00000020) == 0x00000020)) {
route_ = new java.util.ArrayList(route_);
bitField0_ |= 0x00000020;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.rhythm.pb.RequestProtos.RoutePB, com.rhythm.pb.RequestProtos.RoutePB.Builder, com.rhythm.pb.RequestProtos.RoutePBOrBuilder> routeBuilder_;
/**
* repeated .louie.RoutePB route = 20 [deprecated = true];
*/
@java.lang.Deprecated public java.util.List getRouteList() {
if (routeBuilder_ == null) {
return java.util.Collections.unmodifiableList(route_);
} else {
return routeBuilder_.getMessageList();
}
}
/**
* repeated .louie.RoutePB route = 20 [deprecated = true];
*/
@java.lang.Deprecated public int getRouteCount() {
if (routeBuilder_ == null) {
return route_.size();
} else {
return routeBuilder_.getCount();
}
}
/**
* repeated .louie.RoutePB route = 20 [deprecated = true];
*/
@java.lang.Deprecated public com.rhythm.pb.RequestProtos.RoutePB getRoute(int index) {
if (routeBuilder_ == null) {
return route_.get(index);
} else {
return routeBuilder_.getMessage(index);
}
}
/**
* repeated .louie.RoutePB route = 20 [deprecated = true];
*/
@java.lang.Deprecated public Builder setRoute(
int index, com.rhythm.pb.RequestProtos.RoutePB value) {
if (routeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRouteIsMutable();
route_.set(index, value);
onChanged();
} else {
routeBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .louie.RoutePB route = 20 [deprecated = true];
*/
@java.lang.Deprecated public Builder setRoute(
int index, com.rhythm.pb.RequestProtos.RoutePB.Builder builderForValue) {
if (routeBuilder_ == null) {
ensureRouteIsMutable();
route_.set(index, builderForValue.build());
onChanged();
} else {
routeBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .louie.RoutePB route = 20 [deprecated = true];
*/
@java.lang.Deprecated public Builder addRoute(com.rhythm.pb.RequestProtos.RoutePB value) {
if (routeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRouteIsMutable();
route_.add(value);
onChanged();
} else {
routeBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .louie.RoutePB route = 20 [deprecated = true];
*/
@java.lang.Deprecated public Builder addRoute(
int index, com.rhythm.pb.RequestProtos.RoutePB value) {
if (routeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRouteIsMutable();
route_.add(index, value);
onChanged();
} else {
routeBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .louie.RoutePB route = 20 [deprecated = true];
*/
@java.lang.Deprecated public Builder addRoute(
com.rhythm.pb.RequestProtos.RoutePB.Builder builderForValue) {
if (routeBuilder_ == null) {
ensureRouteIsMutable();
route_.add(builderForValue.build());
onChanged();
} else {
routeBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .louie.RoutePB route = 20 [deprecated = true];
*/
@java.lang.Deprecated public Builder addRoute(
int index, com.rhythm.pb.RequestProtos.RoutePB.Builder builderForValue) {
if (routeBuilder_ == null) {
ensureRouteIsMutable();
route_.add(index, builderForValue.build());
onChanged();
} else {
routeBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .louie.RoutePB route = 20 [deprecated = true];
*/
@java.lang.Deprecated public Builder addAllRoute(
java.lang.Iterable extends com.rhythm.pb.RequestProtos.RoutePB> values) {
if (routeBuilder_ == null) {
ensureRouteIsMutable();
super.addAll(values, route_);
onChanged();
} else {
routeBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .louie.RoutePB route = 20 [deprecated = true];
*/
@java.lang.Deprecated public Builder clearRoute() {
if (routeBuilder_ == null) {
route_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
} else {
routeBuilder_.clear();
}
return this;
}
/**
* repeated .louie.RoutePB route = 20 [deprecated = true];
*/
@java.lang.Deprecated public Builder removeRoute(int index) {
if (routeBuilder_ == null) {
ensureRouteIsMutable();
route_.remove(index);
onChanged();
} else {
routeBuilder_.remove(index);
}
return this;
}
/**
* repeated .louie.RoutePB route = 20 [deprecated = true];
*/
@java.lang.Deprecated public com.rhythm.pb.RequestProtos.RoutePB.Builder getRouteBuilder(
int index) {
return getRouteFieldBuilder().getBuilder(index);
}
/**
* repeated .louie.RoutePB route = 20 [deprecated = true];
*/
@java.lang.Deprecated public com.rhythm.pb.RequestProtos.RoutePBOrBuilder getRouteOrBuilder(
int index) {
if (routeBuilder_ == null) {
return route_.get(index); } else {
return routeBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .louie.RoutePB route = 20 [deprecated = true];
*/
@java.lang.Deprecated public java.util.List extends com.rhythm.pb.RequestProtos.RoutePBOrBuilder>
getRouteOrBuilderList() {
if (routeBuilder_ != null) {
return routeBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(route_);
}
}
/**
* repeated .louie.RoutePB route = 20 [deprecated = true];
*/
@java.lang.Deprecated public com.rhythm.pb.RequestProtos.RoutePB.Builder addRouteBuilder() {
return getRouteFieldBuilder().addBuilder(
com.rhythm.pb.RequestProtos.RoutePB.getDefaultInstance());
}
/**
* repeated .louie.RoutePB route = 20 [deprecated = true];
*/
@java.lang.Deprecated public com.rhythm.pb.RequestProtos.RoutePB.Builder addRouteBuilder(
int index) {
return getRouteFieldBuilder().addBuilder(
index, com.rhythm.pb.RequestProtos.RoutePB.getDefaultInstance());
}
/**
* repeated .louie.RoutePB route = 20 [deprecated = true];
*/
@java.lang.Deprecated public java.util.List
getRouteBuilderList() {
return getRouteFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.rhythm.pb.RequestProtos.RoutePB, com.rhythm.pb.RequestProtos.RoutePB.Builder, com.rhythm.pb.RequestProtos.RoutePBOrBuilder>
getRouteFieldBuilder() {
if (routeBuilder_ == null) {
routeBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.rhythm.pb.RequestProtos.RoutePB, com.rhythm.pb.RequestProtos.RoutePB.Builder, com.rhythm.pb.RequestProtos.RoutePBOrBuilder>(
route_,
((bitField0_ & 0x00000020) == 0x00000020),
getParentForChildren(),
isClean());
route_ = null;
}
return routeBuilder_;
}
// optional string routeUser = 30 [deprecated = true];
private java.lang.Object routeUser_ = "";
/**
* optional string routeUser = 30 [deprecated = true];
*/
@java.lang.Deprecated public boolean hasRouteUser() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional string routeUser = 30 [deprecated = true];
*/
@java.lang.Deprecated public java.lang.String getRouteUser() {
java.lang.Object ref = routeUser_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
routeUser_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string routeUser = 30 [deprecated = true];
*/
@java.lang.Deprecated public com.google.protobuf.ByteString
getRouteUserBytes() {
java.lang.Object ref = routeUser_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
routeUser_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string routeUser = 30 [deprecated = true];
*/
@java.lang.Deprecated public Builder setRouteUser(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
routeUser_ = value;
onChanged();
return this;
}
/**
* optional string routeUser = 30 [deprecated = true];
*/
@java.lang.Deprecated public Builder clearRouteUser() {
bitField0_ = (bitField0_ & ~0x00000040);
routeUser_ = getDefaultInstance().getRouteUser();
onChanged();
return this;
}
/**
* optional string routeUser = 30 [deprecated = true];
*/
@java.lang.Deprecated public Builder setRouteUserBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
routeUser_ = value;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:louie.RequestHeaderPB)
}
static {
defaultInstance = new RequestHeaderPB(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:louie.RequestHeaderPB)
}
public interface RoutePBOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional string hostIp = 1;
/**
* optional string hostIp = 1;
*/
boolean hasHostIp();
/**
* optional string hostIp = 1;
*/
java.lang.String getHostIp();
/**
* optional string hostIp = 1;
*/
com.google.protobuf.ByteString
getHostIpBytes();
// optional string gateway = 2;
/**
* optional string gateway = 2;
*/
boolean hasGateway();
/**
* optional string gateway = 2;
*/
java.lang.String getGateway();
/**
* optional string gateway = 2;
*/
com.google.protobuf.ByteString
getGatewayBytes();
// optional string service = 3;
/**
* optional string service = 3;
*/
boolean hasService();
/**
* optional string service = 3;
*/
java.lang.String getService();
/**
* optional string service = 3;
*/
com.google.protobuf.ByteString
getServiceBytes();
// optional string variant = 4;
/**
* optional string variant = 4;
*/
boolean hasVariant();
/**
* optional string variant = 4;
*/
java.lang.String getVariant();
/**
* optional string variant = 4;
*/
com.google.protobuf.ByteString
getVariantBytes();
}
/**
* Protobuf type {@code louie.RoutePB}
*/
public static final class RoutePB extends
com.google.protobuf.GeneratedMessage
implements RoutePBOrBuilder {
// Use RoutePB.newBuilder() to construct.
private RoutePB(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private RoutePB(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final RoutePB defaultInstance;
public static RoutePB getDefaultInstance() {
return defaultInstance;
}
public RoutePB getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RoutePB(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
hostIp_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
gateway_ = input.readBytes();
break;
}
case 26: {
bitField0_ |= 0x00000004;
service_ = input.readBytes();
break;
}
case 34: {
bitField0_ |= 0x00000008;
variant_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.rhythm.pb.RequestProtos.internal_static_louie_RoutePB_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.rhythm.pb.RequestProtos.internal_static_louie_RoutePB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.rhythm.pb.RequestProtos.RoutePB.class, com.rhythm.pb.RequestProtos.RoutePB.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public RoutePB parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RoutePB(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional string hostIp = 1;
public static final int HOSTIP_FIELD_NUMBER = 1;
private java.lang.Object hostIp_;
/**
* optional string hostIp = 1;
*/
public boolean hasHostIp() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string hostIp = 1;
*/
public java.lang.String getHostIp() {
java.lang.Object ref = hostIp_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
hostIp_ = s;
}
return s;
}
}
/**
* optional string hostIp = 1;
*/
public com.google.protobuf.ByteString
getHostIpBytes() {
java.lang.Object ref = hostIp_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
hostIp_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string gateway = 2;
public static final int GATEWAY_FIELD_NUMBER = 2;
private java.lang.Object gateway_;
/**
* optional string gateway = 2;
*/
public boolean hasGateway() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string gateway = 2;
*/
public java.lang.String getGateway() {
java.lang.Object ref = gateway_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
gateway_ = s;
}
return s;
}
}
/**
* optional string gateway = 2;
*/
public com.google.protobuf.ByteString
getGatewayBytes() {
java.lang.Object ref = gateway_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
gateway_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string service = 3;
public static final int SERVICE_FIELD_NUMBER = 3;
private java.lang.Object service_;
/**
* optional string service = 3;
*/
public boolean hasService() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string service = 3;
*/
public java.lang.String getService() {
java.lang.Object ref = service_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
service_ = s;
}
return s;
}
}
/**
* optional string service = 3;
*/
public com.google.protobuf.ByteString
getServiceBytes() {
java.lang.Object ref = service_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
service_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string variant = 4;
public static final int VARIANT_FIELD_NUMBER = 4;
private java.lang.Object variant_;
/**
* optional string variant = 4;
*/
public boolean hasVariant() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string variant = 4;
*/
public java.lang.String getVariant() {
java.lang.Object ref = variant_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
variant_ = s;
}
return s;
}
}
/**
* optional string variant = 4;
*/
public com.google.protobuf.ByteString
getVariantBytes() {
java.lang.Object ref = variant_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
variant_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
hostIp_ = "";
gateway_ = "";
service_ = "";
variant_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getHostIpBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getGatewayBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, getServiceBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBytes(4, getVariantBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getHostIpBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getGatewayBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, getServiceBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, getVariantBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.rhythm.pb.RequestProtos.RoutePB)) {
return super.equals(obj);
}
com.rhythm.pb.RequestProtos.RoutePB other = (com.rhythm.pb.RequestProtos.RoutePB) obj;
boolean result = true;
result = result && (hasHostIp() == other.hasHostIp());
if (hasHostIp()) {
result = result && getHostIp()
.equals(other.getHostIp());
}
result = result && (hasGateway() == other.hasGateway());
if (hasGateway()) {
result = result && getGateway()
.equals(other.getGateway());
}
result = result && (hasService() == other.hasService());
if (hasService()) {
result = result && getService()
.equals(other.getService());
}
result = result && (hasVariant() == other.hasVariant());
if (hasVariant()) {
result = result && getVariant()
.equals(other.getVariant());
}
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasHostIp()) {
hash = (37 * hash) + HOSTIP_FIELD_NUMBER;
hash = (53 * hash) + getHostIp().hashCode();
}
if (hasGateway()) {
hash = (37 * hash) + GATEWAY_FIELD_NUMBER;
hash = (53 * hash) + getGateway().hashCode();
}
if (hasService()) {
hash = (37 * hash) + SERVICE_FIELD_NUMBER;
hash = (53 * hash) + getService().hashCode();
}
if (hasVariant()) {
hash = (37 * hash) + VARIANT_FIELD_NUMBER;
hash = (53 * hash) + getVariant().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.rhythm.pb.RequestProtos.RoutePB parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.rhythm.pb.RequestProtos.RoutePB parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.RoutePB parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.rhythm.pb.RequestProtos.RoutePB parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.RoutePB parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.rhythm.pb.RequestProtos.RoutePB parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.RoutePB parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.rhythm.pb.RequestProtos.RoutePB parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.RoutePB parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.rhythm.pb.RequestProtos.RoutePB parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.rhythm.pb.RequestProtos.RoutePB prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code louie.RoutePB}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.rhythm.pb.RequestProtos.RoutePBOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.rhythm.pb.RequestProtos.internal_static_louie_RoutePB_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.rhythm.pb.RequestProtos.internal_static_louie_RoutePB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.rhythm.pb.RequestProtos.RoutePB.class, com.rhythm.pb.RequestProtos.RoutePB.Builder.class);
}
// Construct using com.rhythm.pb.RequestProtos.RoutePB.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
hostIp_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
gateway_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
service_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
variant_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.rhythm.pb.RequestProtos.internal_static_louie_RoutePB_descriptor;
}
public com.rhythm.pb.RequestProtos.RoutePB getDefaultInstanceForType() {
return com.rhythm.pb.RequestProtos.RoutePB.getDefaultInstance();
}
public com.rhythm.pb.RequestProtos.RoutePB build() {
com.rhythm.pb.RequestProtos.RoutePB result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.rhythm.pb.RequestProtos.RoutePB buildPartial() {
com.rhythm.pb.RequestProtos.RoutePB result = new com.rhythm.pb.RequestProtos.RoutePB(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.hostIp_ = hostIp_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.gateway_ = gateway_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.service_ = service_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.variant_ = variant_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.rhythm.pb.RequestProtos.RoutePB) {
return mergeFrom((com.rhythm.pb.RequestProtos.RoutePB)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.rhythm.pb.RequestProtos.RoutePB other) {
if (other == com.rhythm.pb.RequestProtos.RoutePB.getDefaultInstance()) return this;
if (other.hasHostIp()) {
bitField0_ |= 0x00000001;
hostIp_ = other.hostIp_;
onChanged();
}
if (other.hasGateway()) {
bitField0_ |= 0x00000002;
gateway_ = other.gateway_;
onChanged();
}
if (other.hasService()) {
bitField0_ |= 0x00000004;
service_ = other.service_;
onChanged();
}
if (other.hasVariant()) {
bitField0_ |= 0x00000008;
variant_ = other.variant_;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.rhythm.pb.RequestProtos.RoutePB parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.rhythm.pb.RequestProtos.RoutePB) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional string hostIp = 1;
private java.lang.Object hostIp_ = "";
/**
* optional string hostIp = 1;
*/
public boolean hasHostIp() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string hostIp = 1;
*/
public java.lang.String getHostIp() {
java.lang.Object ref = hostIp_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
hostIp_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string hostIp = 1;
*/
public com.google.protobuf.ByteString
getHostIpBytes() {
java.lang.Object ref = hostIp_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
hostIp_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string hostIp = 1;
*/
public Builder setHostIp(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
hostIp_ = value;
onChanged();
return this;
}
/**
* optional string hostIp = 1;
*/
public Builder clearHostIp() {
bitField0_ = (bitField0_ & ~0x00000001);
hostIp_ = getDefaultInstance().getHostIp();
onChanged();
return this;
}
/**
* optional string hostIp = 1;
*/
public Builder setHostIpBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
hostIp_ = value;
onChanged();
return this;
}
// optional string gateway = 2;
private java.lang.Object gateway_ = "";
/**
* optional string gateway = 2;
*/
public boolean hasGateway() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string gateway = 2;
*/
public java.lang.String getGateway() {
java.lang.Object ref = gateway_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
gateway_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string gateway = 2;
*/
public com.google.protobuf.ByteString
getGatewayBytes() {
java.lang.Object ref = gateway_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
gateway_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string gateway = 2;
*/
public Builder setGateway(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
gateway_ = value;
onChanged();
return this;
}
/**
* optional string gateway = 2;
*/
public Builder clearGateway() {
bitField0_ = (bitField0_ & ~0x00000002);
gateway_ = getDefaultInstance().getGateway();
onChanged();
return this;
}
/**
* optional string gateway = 2;
*/
public Builder setGatewayBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
gateway_ = value;
onChanged();
return this;
}
// optional string service = 3;
private java.lang.Object service_ = "";
/**
* optional string service = 3;
*/
public boolean hasService() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string service = 3;
*/
public java.lang.String getService() {
java.lang.Object ref = service_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
service_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string service = 3;
*/
public com.google.protobuf.ByteString
getServiceBytes() {
java.lang.Object ref = service_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
service_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string service = 3;
*/
public Builder setService(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
service_ = value;
onChanged();
return this;
}
/**
* optional string service = 3;
*/
public Builder clearService() {
bitField0_ = (bitField0_ & ~0x00000004);
service_ = getDefaultInstance().getService();
onChanged();
return this;
}
/**
* optional string service = 3;
*/
public Builder setServiceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
service_ = value;
onChanged();
return this;
}
// optional string variant = 4;
private java.lang.Object variant_ = "";
/**
* optional string variant = 4;
*/
public boolean hasVariant() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string variant = 4;
*/
public java.lang.String getVariant() {
java.lang.Object ref = variant_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
variant_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string variant = 4;
*/
public com.google.protobuf.ByteString
getVariantBytes() {
java.lang.Object ref = variant_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
variant_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string variant = 4;
*/
public Builder setVariant(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
variant_ = value;
onChanged();
return this;
}
/**
* optional string variant = 4;
*/
public Builder clearVariant() {
bitField0_ = (bitField0_ & ~0x00000008);
variant_ = getDefaultInstance().getVariant();
onChanged();
return this;
}
/**
* optional string variant = 4;
*/
public Builder setVariantBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
variant_ = value;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:louie.RoutePB)
}
static {
defaultInstance = new RoutePB(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:louie.RoutePB)
}
public interface RoutePathPBOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional .louie.RoutePB route = 1;
/**
* optional .louie.RoutePB route = 1;
*/
boolean hasRoute();
/**
* optional .louie.RoutePB route = 1;
*/
com.rhythm.pb.RequestProtos.RoutePB getRoute();
/**
* optional .louie.RoutePB route = 1;
*/
com.rhythm.pb.RequestProtos.RoutePBOrBuilder getRouteOrBuilder();
// repeated .louie.RoutePathPB path = 2;
/**
* repeated .louie.RoutePathPB path = 2;
*/
java.util.List
getPathList();
/**
* repeated .louie.RoutePathPB path = 2;
*/
com.rhythm.pb.RequestProtos.RoutePathPB getPath(int index);
/**
* repeated .louie.RoutePathPB path = 2;
*/
int getPathCount();
/**
* repeated .louie.RoutePathPB path = 2;
*/
java.util.List extends com.rhythm.pb.RequestProtos.RoutePathPBOrBuilder>
getPathOrBuilderList();
/**
* repeated .louie.RoutePathPB path = 2;
*/
com.rhythm.pb.RequestProtos.RoutePathPBOrBuilder getPathOrBuilder(
int index);
}
/**
* Protobuf type {@code louie.RoutePathPB}
*/
public static final class RoutePathPB extends
com.google.protobuf.GeneratedMessage
implements RoutePathPBOrBuilder {
// Use RoutePathPB.newBuilder() to construct.
private RoutePathPB(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private RoutePathPB(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final RoutePathPB defaultInstance;
public static RoutePathPB getDefaultInstance() {
return defaultInstance;
}
public RoutePathPB getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RoutePathPB(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.rhythm.pb.RequestProtos.RoutePB.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = route_.toBuilder();
}
route_ = input.readMessage(com.rhythm.pb.RequestProtos.RoutePB.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(route_);
route_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
path_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
path_.add(input.readMessage(com.rhythm.pb.RequestProtos.RoutePathPB.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
path_ = java.util.Collections.unmodifiableList(path_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.rhythm.pb.RequestProtos.internal_static_louie_RoutePathPB_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.rhythm.pb.RequestProtos.internal_static_louie_RoutePathPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.rhythm.pb.RequestProtos.RoutePathPB.class, com.rhythm.pb.RequestProtos.RoutePathPB.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public RoutePathPB parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RoutePathPB(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional .louie.RoutePB route = 1;
public static final int ROUTE_FIELD_NUMBER = 1;
private com.rhythm.pb.RequestProtos.RoutePB route_;
/**
* optional .louie.RoutePB route = 1;
*/
public boolean hasRoute() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .louie.RoutePB route = 1;
*/
public com.rhythm.pb.RequestProtos.RoutePB getRoute() {
return route_;
}
/**
* optional .louie.RoutePB route = 1;
*/
public com.rhythm.pb.RequestProtos.RoutePBOrBuilder getRouteOrBuilder() {
return route_;
}
// repeated .louie.RoutePathPB path = 2;
public static final int PATH_FIELD_NUMBER = 2;
private java.util.List path_;
/**
* repeated .louie.RoutePathPB path = 2;
*/
public java.util.List getPathList() {
return path_;
}
/**
* repeated .louie.RoutePathPB path = 2;
*/
public java.util.List extends com.rhythm.pb.RequestProtos.RoutePathPBOrBuilder>
getPathOrBuilderList() {
return path_;
}
/**
* repeated .louie.RoutePathPB path = 2;
*/
public int getPathCount() {
return path_.size();
}
/**
* repeated .louie.RoutePathPB path = 2;
*/
public com.rhythm.pb.RequestProtos.RoutePathPB getPath(int index) {
return path_.get(index);
}
/**
* repeated .louie.RoutePathPB path = 2;
*/
public com.rhythm.pb.RequestProtos.RoutePathPBOrBuilder getPathOrBuilder(
int index) {
return path_.get(index);
}
private void initFields() {
route_ = com.rhythm.pb.RequestProtos.RoutePB.getDefaultInstance();
path_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, route_);
}
for (int i = 0; i < path_.size(); i++) {
output.writeMessage(2, path_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, route_);
}
for (int i = 0; i < path_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, path_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.rhythm.pb.RequestProtos.RoutePathPB)) {
return super.equals(obj);
}
com.rhythm.pb.RequestProtos.RoutePathPB other = (com.rhythm.pb.RequestProtos.RoutePathPB) obj;
boolean result = true;
result = result && (hasRoute() == other.hasRoute());
if (hasRoute()) {
result = result && getRoute()
.equals(other.getRoute());
}
result = result && getPathList()
.equals(other.getPathList());
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasRoute()) {
hash = (37 * hash) + ROUTE_FIELD_NUMBER;
hash = (53 * hash) + getRoute().hashCode();
}
if (getPathCount() > 0) {
hash = (37 * hash) + PATH_FIELD_NUMBER;
hash = (53 * hash) + getPathList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.rhythm.pb.RequestProtos.RoutePathPB parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.rhythm.pb.RequestProtos.RoutePathPB parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.RoutePathPB parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.rhythm.pb.RequestProtos.RoutePathPB parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.RoutePathPB parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.rhythm.pb.RequestProtos.RoutePathPB parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.RoutePathPB parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.rhythm.pb.RequestProtos.RoutePathPB parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.RoutePathPB parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.rhythm.pb.RequestProtos.RoutePathPB parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.rhythm.pb.RequestProtos.RoutePathPB prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code louie.RoutePathPB}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.rhythm.pb.RequestProtos.RoutePathPBOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.rhythm.pb.RequestProtos.internal_static_louie_RoutePathPB_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.rhythm.pb.RequestProtos.internal_static_louie_RoutePathPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.rhythm.pb.RequestProtos.RoutePathPB.class, com.rhythm.pb.RequestProtos.RoutePathPB.Builder.class);
}
// Construct using com.rhythm.pb.RequestProtos.RoutePathPB.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getRouteFieldBuilder();
getPathFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (routeBuilder_ == null) {
route_ = com.rhythm.pb.RequestProtos.RoutePB.getDefaultInstance();
} else {
routeBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
if (pathBuilder_ == null) {
path_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
pathBuilder_.clear();
}
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.rhythm.pb.RequestProtos.internal_static_louie_RoutePathPB_descriptor;
}
public com.rhythm.pb.RequestProtos.RoutePathPB getDefaultInstanceForType() {
return com.rhythm.pb.RequestProtos.RoutePathPB.getDefaultInstance();
}
public com.rhythm.pb.RequestProtos.RoutePathPB build() {
com.rhythm.pb.RequestProtos.RoutePathPB result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.rhythm.pb.RequestProtos.RoutePathPB buildPartial() {
com.rhythm.pb.RequestProtos.RoutePathPB result = new com.rhythm.pb.RequestProtos.RoutePathPB(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
if (routeBuilder_ == null) {
result.route_ = route_;
} else {
result.route_ = routeBuilder_.build();
}
if (pathBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
path_ = java.util.Collections.unmodifiableList(path_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.path_ = path_;
} else {
result.path_ = pathBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.rhythm.pb.RequestProtos.RoutePathPB) {
return mergeFrom((com.rhythm.pb.RequestProtos.RoutePathPB)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.rhythm.pb.RequestProtos.RoutePathPB other) {
if (other == com.rhythm.pb.RequestProtos.RoutePathPB.getDefaultInstance()) return this;
if (other.hasRoute()) {
mergeRoute(other.getRoute());
}
if (pathBuilder_ == null) {
if (!other.path_.isEmpty()) {
if (path_.isEmpty()) {
path_ = other.path_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensurePathIsMutable();
path_.addAll(other.path_);
}
onChanged();
}
} else {
if (!other.path_.isEmpty()) {
if (pathBuilder_.isEmpty()) {
pathBuilder_.dispose();
pathBuilder_ = null;
path_ = other.path_;
bitField0_ = (bitField0_ & ~0x00000002);
pathBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getPathFieldBuilder() : null;
} else {
pathBuilder_.addAllMessages(other.path_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.rhythm.pb.RequestProtos.RoutePathPB parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.rhythm.pb.RequestProtos.RoutePathPB) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional .louie.RoutePB route = 1;
private com.rhythm.pb.RequestProtos.RoutePB route_ = com.rhythm.pb.RequestProtos.RoutePB.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.rhythm.pb.RequestProtos.RoutePB, com.rhythm.pb.RequestProtos.RoutePB.Builder, com.rhythm.pb.RequestProtos.RoutePBOrBuilder> routeBuilder_;
/**
* optional .louie.RoutePB route = 1;
*/
public boolean hasRoute() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .louie.RoutePB route = 1;
*/
public com.rhythm.pb.RequestProtos.RoutePB getRoute() {
if (routeBuilder_ == null) {
return route_;
} else {
return routeBuilder_.getMessage();
}
}
/**
* optional .louie.RoutePB route = 1;
*/
public Builder setRoute(com.rhythm.pb.RequestProtos.RoutePB value) {
if (routeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
route_ = value;
onChanged();
} else {
routeBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .louie.RoutePB route = 1;
*/
public Builder setRoute(
com.rhythm.pb.RequestProtos.RoutePB.Builder builderForValue) {
if (routeBuilder_ == null) {
route_ = builderForValue.build();
onChanged();
} else {
routeBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .louie.RoutePB route = 1;
*/
public Builder mergeRoute(com.rhythm.pb.RequestProtos.RoutePB value) {
if (routeBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
route_ != com.rhythm.pb.RequestProtos.RoutePB.getDefaultInstance()) {
route_ =
com.rhythm.pb.RequestProtos.RoutePB.newBuilder(route_).mergeFrom(value).buildPartial();
} else {
route_ = value;
}
onChanged();
} else {
routeBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .louie.RoutePB route = 1;
*/
public Builder clearRoute() {
if (routeBuilder_ == null) {
route_ = com.rhythm.pb.RequestProtos.RoutePB.getDefaultInstance();
onChanged();
} else {
routeBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* optional .louie.RoutePB route = 1;
*/
public com.rhythm.pb.RequestProtos.RoutePB.Builder getRouteBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getRouteFieldBuilder().getBuilder();
}
/**
* optional .louie.RoutePB route = 1;
*/
public com.rhythm.pb.RequestProtos.RoutePBOrBuilder getRouteOrBuilder() {
if (routeBuilder_ != null) {
return routeBuilder_.getMessageOrBuilder();
} else {
return route_;
}
}
/**
* optional .louie.RoutePB route = 1;
*/
private com.google.protobuf.SingleFieldBuilder<
com.rhythm.pb.RequestProtos.RoutePB, com.rhythm.pb.RequestProtos.RoutePB.Builder, com.rhythm.pb.RequestProtos.RoutePBOrBuilder>
getRouteFieldBuilder() {
if (routeBuilder_ == null) {
routeBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.rhythm.pb.RequestProtos.RoutePB, com.rhythm.pb.RequestProtos.RoutePB.Builder, com.rhythm.pb.RequestProtos.RoutePBOrBuilder>(
route_,
getParentForChildren(),
isClean());
route_ = null;
}
return routeBuilder_;
}
// repeated .louie.RoutePathPB path = 2;
private java.util.List path_ =
java.util.Collections.emptyList();
private void ensurePathIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
path_ = new java.util.ArrayList(path_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.rhythm.pb.RequestProtos.RoutePathPB, com.rhythm.pb.RequestProtos.RoutePathPB.Builder, com.rhythm.pb.RequestProtos.RoutePathPBOrBuilder> pathBuilder_;
/**
* repeated .louie.RoutePathPB path = 2;
*/
public java.util.List getPathList() {
if (pathBuilder_ == null) {
return java.util.Collections.unmodifiableList(path_);
} else {
return pathBuilder_.getMessageList();
}
}
/**
* repeated .louie.RoutePathPB path = 2;
*/
public int getPathCount() {
if (pathBuilder_ == null) {
return path_.size();
} else {
return pathBuilder_.getCount();
}
}
/**
* repeated .louie.RoutePathPB path = 2;
*/
public com.rhythm.pb.RequestProtos.RoutePathPB getPath(int index) {
if (pathBuilder_ == null) {
return path_.get(index);
} else {
return pathBuilder_.getMessage(index);
}
}
/**
* repeated .louie.RoutePathPB path = 2;
*/
public Builder setPath(
int index, com.rhythm.pb.RequestProtos.RoutePathPB value) {
if (pathBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePathIsMutable();
path_.set(index, value);
onChanged();
} else {
pathBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .louie.RoutePathPB path = 2;
*/
public Builder setPath(
int index, com.rhythm.pb.RequestProtos.RoutePathPB.Builder builderForValue) {
if (pathBuilder_ == null) {
ensurePathIsMutable();
path_.set(index, builderForValue.build());
onChanged();
} else {
pathBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .louie.RoutePathPB path = 2;
*/
public Builder addPath(com.rhythm.pb.RequestProtos.RoutePathPB value) {
if (pathBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePathIsMutable();
path_.add(value);
onChanged();
} else {
pathBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .louie.RoutePathPB path = 2;
*/
public Builder addPath(
int index, com.rhythm.pb.RequestProtos.RoutePathPB value) {
if (pathBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePathIsMutable();
path_.add(index, value);
onChanged();
} else {
pathBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .louie.RoutePathPB path = 2;
*/
public Builder addPath(
com.rhythm.pb.RequestProtos.RoutePathPB.Builder builderForValue) {
if (pathBuilder_ == null) {
ensurePathIsMutable();
path_.add(builderForValue.build());
onChanged();
} else {
pathBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .louie.RoutePathPB path = 2;
*/
public Builder addPath(
int index, com.rhythm.pb.RequestProtos.RoutePathPB.Builder builderForValue) {
if (pathBuilder_ == null) {
ensurePathIsMutable();
path_.add(index, builderForValue.build());
onChanged();
} else {
pathBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .louie.RoutePathPB path = 2;
*/
public Builder addAllPath(
java.lang.Iterable extends com.rhythm.pb.RequestProtos.RoutePathPB> values) {
if (pathBuilder_ == null) {
ensurePathIsMutable();
super.addAll(values, path_);
onChanged();
} else {
pathBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .louie.RoutePathPB path = 2;
*/
public Builder clearPath() {
if (pathBuilder_ == null) {
path_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
pathBuilder_.clear();
}
return this;
}
/**
* repeated .louie.RoutePathPB path = 2;
*/
public Builder removePath(int index) {
if (pathBuilder_ == null) {
ensurePathIsMutable();
path_.remove(index);
onChanged();
} else {
pathBuilder_.remove(index);
}
return this;
}
/**
* repeated .louie.RoutePathPB path = 2;
*/
public com.rhythm.pb.RequestProtos.RoutePathPB.Builder getPathBuilder(
int index) {
return getPathFieldBuilder().getBuilder(index);
}
/**
* repeated .louie.RoutePathPB path = 2;
*/
public com.rhythm.pb.RequestProtos.RoutePathPBOrBuilder getPathOrBuilder(
int index) {
if (pathBuilder_ == null) {
return path_.get(index); } else {
return pathBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .louie.RoutePathPB path = 2;
*/
public java.util.List extends com.rhythm.pb.RequestProtos.RoutePathPBOrBuilder>
getPathOrBuilderList() {
if (pathBuilder_ != null) {
return pathBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(path_);
}
}
/**
* repeated .louie.RoutePathPB path = 2;
*/
public com.rhythm.pb.RequestProtos.RoutePathPB.Builder addPathBuilder() {
return getPathFieldBuilder().addBuilder(
com.rhythm.pb.RequestProtos.RoutePathPB.getDefaultInstance());
}
/**
* repeated .louie.RoutePathPB path = 2;
*/
public com.rhythm.pb.RequestProtos.RoutePathPB.Builder addPathBuilder(
int index) {
return getPathFieldBuilder().addBuilder(
index, com.rhythm.pb.RequestProtos.RoutePathPB.getDefaultInstance());
}
/**
* repeated .louie.RoutePathPB path = 2;
*/
public java.util.List
getPathBuilderList() {
return getPathFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.rhythm.pb.RequestProtos.RoutePathPB, com.rhythm.pb.RequestProtos.RoutePathPB.Builder, com.rhythm.pb.RequestProtos.RoutePathPBOrBuilder>
getPathFieldBuilder() {
if (pathBuilder_ == null) {
pathBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.rhythm.pb.RequestProtos.RoutePathPB, com.rhythm.pb.RequestProtos.RoutePathPB.Builder, com.rhythm.pb.RequestProtos.RoutePathPBOrBuilder>(
path_,
((bitField0_ & 0x00000002) == 0x00000002),
getParentForChildren(),
isClean());
path_ = null;
}
return pathBuilder_;
}
// @@protoc_insertion_point(builder_scope:louie.RoutePathPB)
}
static {
defaultInstance = new RoutePathPB(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:louie.RoutePathPB)
}
public interface IdentityPBOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional string user = 1;
/**
* optional string user = 1;
*/
boolean hasUser();
/**
* optional string user = 1;
*/
java.lang.String getUser();
/**
* optional string user = 1;
*/
com.google.protobuf.ByteString
getUserBytes();
// optional string language = 2;
/**
* optional string language = 2;
*/
boolean hasLanguage();
/**
* optional string language = 2;
*/
java.lang.String getLanguage();
/**
* optional string language = 2;
*/
com.google.protobuf.ByteString
getLanguageBytes();
// optional string program = 3;
/**
* optional string program = 3;
*/
boolean hasProgram();
/**
* optional string program = 3;
*/
java.lang.String getProgram();
/**
* optional string program = 3;
*/
com.google.protobuf.ByteString
getProgramBytes();
// optional string path = 4;
/**
* optional string path = 4;
*/
boolean hasPath();
/**
* optional string path = 4;
*/
java.lang.String getPath();
/**
* optional string path = 4;
*/
com.google.protobuf.ByteString
getPathBytes();
// optional string location = 5;
/**
* optional string location = 5;
*/
boolean hasLocation();
/**
* optional string location = 5;
*/
java.lang.String getLocation();
/**
* optional string location = 5;
*/
com.google.protobuf.ByteString
getLocationBytes();
// optional string machine = 6;
/**
* optional string machine = 6;
*/
boolean hasMachine();
/**
* optional string machine = 6;
*/
java.lang.String getMachine();
/**
* optional string machine = 6;
*/
com.google.protobuf.ByteString
getMachineBytes();
// optional string env = 7;
/**
* optional string env = 7;
*/
boolean hasEnv();
/**
* optional string env = 7;
*/
java.lang.String getEnv();
/**
* optional string env = 7;
*/
com.google.protobuf.ByteString
getEnvBytes();
// optional string ip = 8;
/**
* optional string ip = 8;
*/
boolean hasIp();
/**
* optional string ip = 8;
*/
java.lang.String getIp();
/**
* optional string ip = 8;
*/
com.google.protobuf.ByteString
getIpBytes();
// optional string languageVersion = 9;
/**
* optional string languageVersion = 9;
*/
boolean hasLanguageVersion();
/**
* optional string languageVersion = 9;
*/
java.lang.String getLanguageVersion();
/**
* optional string languageVersion = 9;
*/
com.google.protobuf.ByteString
getLanguageVersionBytes();
// optional string programVersion = 10;
/**
* optional string programVersion = 10;
*/
boolean hasProgramVersion();
/**
* optional string programVersion = 10;
*/
java.lang.String getProgramVersion();
/**
* optional string programVersion = 10;
*/
com.google.protobuf.ByteString
getProgramVersionBytes();
// optional string os = 11;
/**
* optional string os = 11;
*/
boolean hasOs();
/**
* optional string os = 11;
*/
java.lang.String getOs();
/**
* optional string os = 11;
*/
com.google.protobuf.ByteString
getOsBytes();
// optional string osVersion = 12;
/**
* optional string osVersion = 12;
*/
boolean hasOsVersion();
/**
* optional string osVersion = 12;
*/
java.lang.String getOsVersion();
/**
* optional string osVersion = 12;
*/
com.google.protobuf.ByteString
getOsVersionBytes();
// optional string processId = 13;
/**
* optional string processId = 13;
*/
boolean hasProcessId();
/**
* optional string processId = 13;
*/
java.lang.String getProcessId();
/**
* optional string processId = 13;
*/
com.google.protobuf.ByteString
getProcessIdBytes();
}
/**
* Protobuf type {@code louie.IdentityPB}
*/
public static final class IdentityPB extends
com.google.protobuf.GeneratedMessage
implements IdentityPBOrBuilder {
// Use IdentityPB.newBuilder() to construct.
private IdentityPB(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private IdentityPB(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final IdentityPB defaultInstance;
public static IdentityPB getDefaultInstance() {
return defaultInstance;
}
public IdentityPB getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private IdentityPB(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
user_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
language_ = input.readBytes();
break;
}
case 26: {
bitField0_ |= 0x00000004;
program_ = input.readBytes();
break;
}
case 34: {
bitField0_ |= 0x00000008;
path_ = input.readBytes();
break;
}
case 42: {
bitField0_ |= 0x00000010;
location_ = input.readBytes();
break;
}
case 50: {
bitField0_ |= 0x00000020;
machine_ = input.readBytes();
break;
}
case 58: {
bitField0_ |= 0x00000040;
env_ = input.readBytes();
break;
}
case 66: {
bitField0_ |= 0x00000080;
ip_ = input.readBytes();
break;
}
case 74: {
bitField0_ |= 0x00000100;
languageVersion_ = input.readBytes();
break;
}
case 82: {
bitField0_ |= 0x00000200;
programVersion_ = input.readBytes();
break;
}
case 90: {
bitField0_ |= 0x00000400;
os_ = input.readBytes();
break;
}
case 98: {
bitField0_ |= 0x00000800;
osVersion_ = input.readBytes();
break;
}
case 106: {
bitField0_ |= 0x00001000;
processId_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.rhythm.pb.RequestProtos.internal_static_louie_IdentityPB_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.rhythm.pb.RequestProtos.internal_static_louie_IdentityPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.rhythm.pb.RequestProtos.IdentityPB.class, com.rhythm.pb.RequestProtos.IdentityPB.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public IdentityPB parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new IdentityPB(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional string user = 1;
public static final int USER_FIELD_NUMBER = 1;
private java.lang.Object user_;
/**
* optional string user = 1;
*/
public boolean hasUser() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string user = 1;
*/
public java.lang.String getUser() {
java.lang.Object ref = user_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
user_ = s;
}
return s;
}
}
/**
* optional string user = 1;
*/
public com.google.protobuf.ByteString
getUserBytes() {
java.lang.Object ref = user_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
user_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string language = 2;
public static final int LANGUAGE_FIELD_NUMBER = 2;
private java.lang.Object language_;
/**
* optional string language = 2;
*/
public boolean hasLanguage() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string language = 2;
*/
public java.lang.String getLanguage() {
java.lang.Object ref = language_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
language_ = s;
}
return s;
}
}
/**
* optional string language = 2;
*/
public com.google.protobuf.ByteString
getLanguageBytes() {
java.lang.Object ref = language_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
language_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string program = 3;
public static final int PROGRAM_FIELD_NUMBER = 3;
private java.lang.Object program_;
/**
* optional string program = 3;
*/
public boolean hasProgram() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string program = 3;
*/
public java.lang.String getProgram() {
java.lang.Object ref = program_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
program_ = s;
}
return s;
}
}
/**
* optional string program = 3;
*/
public com.google.protobuf.ByteString
getProgramBytes() {
java.lang.Object ref = program_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
program_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string path = 4;
public static final int PATH_FIELD_NUMBER = 4;
private java.lang.Object path_;
/**
* optional string path = 4;
*/
public boolean hasPath() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string path = 4;
*/
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
path_ = s;
}
return s;
}
}
/**
* optional string path = 4;
*/
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string location = 5;
public static final int LOCATION_FIELD_NUMBER = 5;
private java.lang.Object location_;
/**
* optional string location = 5;
*/
public boolean hasLocation() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string location = 5;
*/
public java.lang.String getLocation() {
java.lang.Object ref = location_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
location_ = s;
}
return s;
}
}
/**
* optional string location = 5;
*/
public com.google.protobuf.ByteString
getLocationBytes() {
java.lang.Object ref = location_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
location_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string machine = 6;
public static final int MACHINE_FIELD_NUMBER = 6;
private java.lang.Object machine_;
/**
* optional string machine = 6;
*/
public boolean hasMachine() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string machine = 6;
*/
public java.lang.String getMachine() {
java.lang.Object ref = machine_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
machine_ = s;
}
return s;
}
}
/**
* optional string machine = 6;
*/
public com.google.protobuf.ByteString
getMachineBytes() {
java.lang.Object ref = machine_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
machine_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string env = 7;
public static final int ENV_FIELD_NUMBER = 7;
private java.lang.Object env_;
/**
* optional string env = 7;
*/
public boolean hasEnv() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional string env = 7;
*/
public java.lang.String getEnv() {
java.lang.Object ref = env_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
env_ = s;
}
return s;
}
}
/**
* optional string env = 7;
*/
public com.google.protobuf.ByteString
getEnvBytes() {
java.lang.Object ref = env_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
env_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string ip = 8;
public static final int IP_FIELD_NUMBER = 8;
private java.lang.Object ip_;
/**
* optional string ip = 8;
*/
public boolean hasIp() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional string ip = 8;
*/
public java.lang.String getIp() {
java.lang.Object ref = ip_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
ip_ = s;
}
return s;
}
}
/**
* optional string ip = 8;
*/
public com.google.protobuf.ByteString
getIpBytes() {
java.lang.Object ref = ip_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
ip_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string languageVersion = 9;
public static final int LANGUAGEVERSION_FIELD_NUMBER = 9;
private java.lang.Object languageVersion_;
/**
* optional string languageVersion = 9;
*/
public boolean hasLanguageVersion() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional string languageVersion = 9;
*/
public java.lang.String getLanguageVersion() {
java.lang.Object ref = languageVersion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
languageVersion_ = s;
}
return s;
}
}
/**
* optional string languageVersion = 9;
*/
public com.google.protobuf.ByteString
getLanguageVersionBytes() {
java.lang.Object ref = languageVersion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
languageVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string programVersion = 10;
public static final int PROGRAMVERSION_FIELD_NUMBER = 10;
private java.lang.Object programVersion_;
/**
* optional string programVersion = 10;
*/
public boolean hasProgramVersion() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional string programVersion = 10;
*/
public java.lang.String getProgramVersion() {
java.lang.Object ref = programVersion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
programVersion_ = s;
}
return s;
}
}
/**
* optional string programVersion = 10;
*/
public com.google.protobuf.ByteString
getProgramVersionBytes() {
java.lang.Object ref = programVersion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
programVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string os = 11;
public static final int OS_FIELD_NUMBER = 11;
private java.lang.Object os_;
/**
* optional string os = 11;
*/
public boolean hasOs() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional string os = 11;
*/
public java.lang.String getOs() {
java.lang.Object ref = os_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
os_ = s;
}
return s;
}
}
/**
* optional string os = 11;
*/
public com.google.protobuf.ByteString
getOsBytes() {
java.lang.Object ref = os_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
os_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string osVersion = 12;
public static final int OSVERSION_FIELD_NUMBER = 12;
private java.lang.Object osVersion_;
/**
* optional string osVersion = 12;
*/
public boolean hasOsVersion() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional string osVersion = 12;
*/
public java.lang.String getOsVersion() {
java.lang.Object ref = osVersion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
osVersion_ = s;
}
return s;
}
}
/**
* optional string osVersion = 12;
*/
public com.google.protobuf.ByteString
getOsVersionBytes() {
java.lang.Object ref = osVersion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
osVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string processId = 13;
public static final int PROCESSID_FIELD_NUMBER = 13;
private java.lang.Object processId_;
/**
* optional string processId = 13;
*/
public boolean hasProcessId() {
return ((bitField0_ & 0x00001000) == 0x00001000);
}
/**
* optional string processId = 13;
*/
public java.lang.String getProcessId() {
java.lang.Object ref = processId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
processId_ = s;
}
return s;
}
}
/**
* optional string processId = 13;
*/
public com.google.protobuf.ByteString
getProcessIdBytes() {
java.lang.Object ref = processId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
processId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
user_ = "";
language_ = "";
program_ = "";
path_ = "";
location_ = "";
machine_ = "";
env_ = "";
ip_ = "";
languageVersion_ = "";
programVersion_ = "";
os_ = "";
osVersion_ = "";
processId_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getUserBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getLanguageBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, getProgramBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBytes(4, getPathBytes());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeBytes(5, getLocationBytes());
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeBytes(6, getMachineBytes());
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeBytes(7, getEnvBytes());
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeBytes(8, getIpBytes());
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
output.writeBytes(9, getLanguageVersionBytes());
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
output.writeBytes(10, getProgramVersionBytes());
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
output.writeBytes(11, getOsBytes());
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
output.writeBytes(12, getOsVersionBytes());
}
if (((bitField0_ & 0x00001000) == 0x00001000)) {
output.writeBytes(13, getProcessIdBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getUserBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getLanguageBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, getProgramBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(4, getPathBytes());
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(5, getLocationBytes());
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(6, getMachineBytes());
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(7, getEnvBytes());
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(8, getIpBytes());
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(9, getLanguageVersionBytes());
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(10, getProgramVersionBytes());
}
if (((bitField0_ & 0x00000400) == 0x00000400)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(11, getOsBytes());
}
if (((bitField0_ & 0x00000800) == 0x00000800)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(12, getOsVersionBytes());
}
if (((bitField0_ & 0x00001000) == 0x00001000)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(13, getProcessIdBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.rhythm.pb.RequestProtos.IdentityPB)) {
return super.equals(obj);
}
com.rhythm.pb.RequestProtos.IdentityPB other = (com.rhythm.pb.RequestProtos.IdentityPB) obj;
boolean result = true;
result = result && (hasUser() == other.hasUser());
if (hasUser()) {
result = result && getUser()
.equals(other.getUser());
}
result = result && (hasLanguage() == other.hasLanguage());
if (hasLanguage()) {
result = result && getLanguage()
.equals(other.getLanguage());
}
result = result && (hasProgram() == other.hasProgram());
if (hasProgram()) {
result = result && getProgram()
.equals(other.getProgram());
}
result = result && (hasPath() == other.hasPath());
if (hasPath()) {
result = result && getPath()
.equals(other.getPath());
}
result = result && (hasLocation() == other.hasLocation());
if (hasLocation()) {
result = result && getLocation()
.equals(other.getLocation());
}
result = result && (hasMachine() == other.hasMachine());
if (hasMachine()) {
result = result && getMachine()
.equals(other.getMachine());
}
result = result && (hasEnv() == other.hasEnv());
if (hasEnv()) {
result = result && getEnv()
.equals(other.getEnv());
}
result = result && (hasIp() == other.hasIp());
if (hasIp()) {
result = result && getIp()
.equals(other.getIp());
}
result = result && (hasLanguageVersion() == other.hasLanguageVersion());
if (hasLanguageVersion()) {
result = result && getLanguageVersion()
.equals(other.getLanguageVersion());
}
result = result && (hasProgramVersion() == other.hasProgramVersion());
if (hasProgramVersion()) {
result = result && getProgramVersion()
.equals(other.getProgramVersion());
}
result = result && (hasOs() == other.hasOs());
if (hasOs()) {
result = result && getOs()
.equals(other.getOs());
}
result = result && (hasOsVersion() == other.hasOsVersion());
if (hasOsVersion()) {
result = result && getOsVersion()
.equals(other.getOsVersion());
}
result = result && (hasProcessId() == other.hasProcessId());
if (hasProcessId()) {
result = result && getProcessId()
.equals(other.getProcessId());
}
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasUser()) {
hash = (37 * hash) + USER_FIELD_NUMBER;
hash = (53 * hash) + getUser().hashCode();
}
if (hasLanguage()) {
hash = (37 * hash) + LANGUAGE_FIELD_NUMBER;
hash = (53 * hash) + getLanguage().hashCode();
}
if (hasProgram()) {
hash = (37 * hash) + PROGRAM_FIELD_NUMBER;
hash = (53 * hash) + getProgram().hashCode();
}
if (hasPath()) {
hash = (37 * hash) + PATH_FIELD_NUMBER;
hash = (53 * hash) + getPath().hashCode();
}
if (hasLocation()) {
hash = (37 * hash) + LOCATION_FIELD_NUMBER;
hash = (53 * hash) + getLocation().hashCode();
}
if (hasMachine()) {
hash = (37 * hash) + MACHINE_FIELD_NUMBER;
hash = (53 * hash) + getMachine().hashCode();
}
if (hasEnv()) {
hash = (37 * hash) + ENV_FIELD_NUMBER;
hash = (53 * hash) + getEnv().hashCode();
}
if (hasIp()) {
hash = (37 * hash) + IP_FIELD_NUMBER;
hash = (53 * hash) + getIp().hashCode();
}
if (hasLanguageVersion()) {
hash = (37 * hash) + LANGUAGEVERSION_FIELD_NUMBER;
hash = (53 * hash) + getLanguageVersion().hashCode();
}
if (hasProgramVersion()) {
hash = (37 * hash) + PROGRAMVERSION_FIELD_NUMBER;
hash = (53 * hash) + getProgramVersion().hashCode();
}
if (hasOs()) {
hash = (37 * hash) + OS_FIELD_NUMBER;
hash = (53 * hash) + getOs().hashCode();
}
if (hasOsVersion()) {
hash = (37 * hash) + OSVERSION_FIELD_NUMBER;
hash = (53 * hash) + getOsVersion().hashCode();
}
if (hasProcessId()) {
hash = (37 * hash) + PROCESSID_FIELD_NUMBER;
hash = (53 * hash) + getProcessId().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.rhythm.pb.RequestProtos.IdentityPB parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.rhythm.pb.RequestProtos.IdentityPB parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.IdentityPB parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.rhythm.pb.RequestProtos.IdentityPB parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.IdentityPB parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.rhythm.pb.RequestProtos.IdentityPB parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.IdentityPB parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.rhythm.pb.RequestProtos.IdentityPB parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.IdentityPB parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.rhythm.pb.RequestProtos.IdentityPB parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.rhythm.pb.RequestProtos.IdentityPB prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code louie.IdentityPB}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.rhythm.pb.RequestProtos.IdentityPBOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.rhythm.pb.RequestProtos.internal_static_louie_IdentityPB_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.rhythm.pb.RequestProtos.internal_static_louie_IdentityPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.rhythm.pb.RequestProtos.IdentityPB.class, com.rhythm.pb.RequestProtos.IdentityPB.Builder.class);
}
// Construct using com.rhythm.pb.RequestProtos.IdentityPB.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
user_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
language_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
program_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
path_ = "";
bitField0_ = (bitField0_ & ~0x00000008);
location_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
machine_ = "";
bitField0_ = (bitField0_ & ~0x00000020);
env_ = "";
bitField0_ = (bitField0_ & ~0x00000040);
ip_ = "";
bitField0_ = (bitField0_ & ~0x00000080);
languageVersion_ = "";
bitField0_ = (bitField0_ & ~0x00000100);
programVersion_ = "";
bitField0_ = (bitField0_ & ~0x00000200);
os_ = "";
bitField0_ = (bitField0_ & ~0x00000400);
osVersion_ = "";
bitField0_ = (bitField0_ & ~0x00000800);
processId_ = "";
bitField0_ = (bitField0_ & ~0x00001000);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.rhythm.pb.RequestProtos.internal_static_louie_IdentityPB_descriptor;
}
public com.rhythm.pb.RequestProtos.IdentityPB getDefaultInstanceForType() {
return com.rhythm.pb.RequestProtos.IdentityPB.getDefaultInstance();
}
public com.rhythm.pb.RequestProtos.IdentityPB build() {
com.rhythm.pb.RequestProtos.IdentityPB result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.rhythm.pb.RequestProtos.IdentityPB buildPartial() {
com.rhythm.pb.RequestProtos.IdentityPB result = new com.rhythm.pb.RequestProtos.IdentityPB(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.user_ = user_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.language_ = language_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.program_ = program_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.path_ = path_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.location_ = location_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.machine_ = machine_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
result.env_ = env_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000080;
}
result.ip_ = ip_;
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000100;
}
result.languageVersion_ = languageVersion_;
if (((from_bitField0_ & 0x00000200) == 0x00000200)) {
to_bitField0_ |= 0x00000200;
}
result.programVersion_ = programVersion_;
if (((from_bitField0_ & 0x00000400) == 0x00000400)) {
to_bitField0_ |= 0x00000400;
}
result.os_ = os_;
if (((from_bitField0_ & 0x00000800) == 0x00000800)) {
to_bitField0_ |= 0x00000800;
}
result.osVersion_ = osVersion_;
if (((from_bitField0_ & 0x00001000) == 0x00001000)) {
to_bitField0_ |= 0x00001000;
}
result.processId_ = processId_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.rhythm.pb.RequestProtos.IdentityPB) {
return mergeFrom((com.rhythm.pb.RequestProtos.IdentityPB)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.rhythm.pb.RequestProtos.IdentityPB other) {
if (other == com.rhythm.pb.RequestProtos.IdentityPB.getDefaultInstance()) return this;
if (other.hasUser()) {
bitField0_ |= 0x00000001;
user_ = other.user_;
onChanged();
}
if (other.hasLanguage()) {
bitField0_ |= 0x00000002;
language_ = other.language_;
onChanged();
}
if (other.hasProgram()) {
bitField0_ |= 0x00000004;
program_ = other.program_;
onChanged();
}
if (other.hasPath()) {
bitField0_ |= 0x00000008;
path_ = other.path_;
onChanged();
}
if (other.hasLocation()) {
bitField0_ |= 0x00000010;
location_ = other.location_;
onChanged();
}
if (other.hasMachine()) {
bitField0_ |= 0x00000020;
machine_ = other.machine_;
onChanged();
}
if (other.hasEnv()) {
bitField0_ |= 0x00000040;
env_ = other.env_;
onChanged();
}
if (other.hasIp()) {
bitField0_ |= 0x00000080;
ip_ = other.ip_;
onChanged();
}
if (other.hasLanguageVersion()) {
bitField0_ |= 0x00000100;
languageVersion_ = other.languageVersion_;
onChanged();
}
if (other.hasProgramVersion()) {
bitField0_ |= 0x00000200;
programVersion_ = other.programVersion_;
onChanged();
}
if (other.hasOs()) {
bitField0_ |= 0x00000400;
os_ = other.os_;
onChanged();
}
if (other.hasOsVersion()) {
bitField0_ |= 0x00000800;
osVersion_ = other.osVersion_;
onChanged();
}
if (other.hasProcessId()) {
bitField0_ |= 0x00001000;
processId_ = other.processId_;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.rhythm.pb.RequestProtos.IdentityPB parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.rhythm.pb.RequestProtos.IdentityPB) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional string user = 1;
private java.lang.Object user_ = "";
/**
* optional string user = 1;
*/
public boolean hasUser() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string user = 1;
*/
public java.lang.String getUser() {
java.lang.Object ref = user_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
user_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string user = 1;
*/
public com.google.protobuf.ByteString
getUserBytes() {
java.lang.Object ref = user_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
user_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string user = 1;
*/
public Builder setUser(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
user_ = value;
onChanged();
return this;
}
/**
* optional string user = 1;
*/
public Builder clearUser() {
bitField0_ = (bitField0_ & ~0x00000001);
user_ = getDefaultInstance().getUser();
onChanged();
return this;
}
/**
* optional string user = 1;
*/
public Builder setUserBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
user_ = value;
onChanged();
return this;
}
// optional string language = 2;
private java.lang.Object language_ = "";
/**
* optional string language = 2;
*/
public boolean hasLanguage() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string language = 2;
*/
public java.lang.String getLanguage() {
java.lang.Object ref = language_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
language_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string language = 2;
*/
public com.google.protobuf.ByteString
getLanguageBytes() {
java.lang.Object ref = language_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
language_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string language = 2;
*/
public Builder setLanguage(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
language_ = value;
onChanged();
return this;
}
/**
* optional string language = 2;
*/
public Builder clearLanguage() {
bitField0_ = (bitField0_ & ~0x00000002);
language_ = getDefaultInstance().getLanguage();
onChanged();
return this;
}
/**
* optional string language = 2;
*/
public Builder setLanguageBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
language_ = value;
onChanged();
return this;
}
// optional string program = 3;
private java.lang.Object program_ = "";
/**
* optional string program = 3;
*/
public boolean hasProgram() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string program = 3;
*/
public java.lang.String getProgram() {
java.lang.Object ref = program_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
program_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string program = 3;
*/
public com.google.protobuf.ByteString
getProgramBytes() {
java.lang.Object ref = program_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
program_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string program = 3;
*/
public Builder setProgram(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
program_ = value;
onChanged();
return this;
}
/**
* optional string program = 3;
*/
public Builder clearProgram() {
bitField0_ = (bitField0_ & ~0x00000004);
program_ = getDefaultInstance().getProgram();
onChanged();
return this;
}
/**
* optional string program = 3;
*/
public Builder setProgramBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
program_ = value;
onChanged();
return this;
}
// optional string path = 4;
private java.lang.Object path_ = "";
/**
* optional string path = 4;
*/
public boolean hasPath() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional string path = 4;
*/
public java.lang.String getPath() {
java.lang.Object ref = path_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
path_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string path = 4;
*/
public com.google.protobuf.ByteString
getPathBytes() {
java.lang.Object ref = path_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
path_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string path = 4;
*/
public Builder setPath(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
path_ = value;
onChanged();
return this;
}
/**
* optional string path = 4;
*/
public Builder clearPath() {
bitField0_ = (bitField0_ & ~0x00000008);
path_ = getDefaultInstance().getPath();
onChanged();
return this;
}
/**
* optional string path = 4;
*/
public Builder setPathBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
path_ = value;
onChanged();
return this;
}
// optional string location = 5;
private java.lang.Object location_ = "";
/**
* optional string location = 5;
*/
public boolean hasLocation() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string location = 5;
*/
public java.lang.String getLocation() {
java.lang.Object ref = location_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
location_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string location = 5;
*/
public com.google.protobuf.ByteString
getLocationBytes() {
java.lang.Object ref = location_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
location_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string location = 5;
*/
public Builder setLocation(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
location_ = value;
onChanged();
return this;
}
/**
* optional string location = 5;
*/
public Builder clearLocation() {
bitField0_ = (bitField0_ & ~0x00000010);
location_ = getDefaultInstance().getLocation();
onChanged();
return this;
}
/**
* optional string location = 5;
*/
public Builder setLocationBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
location_ = value;
onChanged();
return this;
}
// optional string machine = 6;
private java.lang.Object machine_ = "";
/**
* optional string machine = 6;
*/
public boolean hasMachine() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional string machine = 6;
*/
public java.lang.String getMachine() {
java.lang.Object ref = machine_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
machine_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string machine = 6;
*/
public com.google.protobuf.ByteString
getMachineBytes() {
java.lang.Object ref = machine_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
machine_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string machine = 6;
*/
public Builder setMachine(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
machine_ = value;
onChanged();
return this;
}
/**
* optional string machine = 6;
*/
public Builder clearMachine() {
bitField0_ = (bitField0_ & ~0x00000020);
machine_ = getDefaultInstance().getMachine();
onChanged();
return this;
}
/**
* optional string machine = 6;
*/
public Builder setMachineBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
machine_ = value;
onChanged();
return this;
}
// optional string env = 7;
private java.lang.Object env_ = "";
/**
* optional string env = 7;
*/
public boolean hasEnv() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional string env = 7;
*/
public java.lang.String getEnv() {
java.lang.Object ref = env_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
env_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string env = 7;
*/
public com.google.protobuf.ByteString
getEnvBytes() {
java.lang.Object ref = env_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
env_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string env = 7;
*/
public Builder setEnv(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
env_ = value;
onChanged();
return this;
}
/**
* optional string env = 7;
*/
public Builder clearEnv() {
bitField0_ = (bitField0_ & ~0x00000040);
env_ = getDefaultInstance().getEnv();
onChanged();
return this;
}
/**
* optional string env = 7;
*/
public Builder setEnvBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
env_ = value;
onChanged();
return this;
}
// optional string ip = 8;
private java.lang.Object ip_ = "";
/**
* optional string ip = 8;
*/
public boolean hasIp() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional string ip = 8;
*/
public java.lang.String getIp() {
java.lang.Object ref = ip_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
ip_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string ip = 8;
*/
public com.google.protobuf.ByteString
getIpBytes() {
java.lang.Object ref = ip_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
ip_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string ip = 8;
*/
public Builder setIp(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
ip_ = value;
onChanged();
return this;
}
/**
* optional string ip = 8;
*/
public Builder clearIp() {
bitField0_ = (bitField0_ & ~0x00000080);
ip_ = getDefaultInstance().getIp();
onChanged();
return this;
}
/**
* optional string ip = 8;
*/
public Builder setIpBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
ip_ = value;
onChanged();
return this;
}
// optional string languageVersion = 9;
private java.lang.Object languageVersion_ = "";
/**
* optional string languageVersion = 9;
*/
public boolean hasLanguageVersion() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional string languageVersion = 9;
*/
public java.lang.String getLanguageVersion() {
java.lang.Object ref = languageVersion_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
languageVersion_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string languageVersion = 9;
*/
public com.google.protobuf.ByteString
getLanguageVersionBytes() {
java.lang.Object ref = languageVersion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
languageVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string languageVersion = 9;
*/
public Builder setLanguageVersion(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
languageVersion_ = value;
onChanged();
return this;
}
/**
* optional string languageVersion = 9;
*/
public Builder clearLanguageVersion() {
bitField0_ = (bitField0_ & ~0x00000100);
languageVersion_ = getDefaultInstance().getLanguageVersion();
onChanged();
return this;
}
/**
* optional string languageVersion = 9;
*/
public Builder setLanguageVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000100;
languageVersion_ = value;
onChanged();
return this;
}
// optional string programVersion = 10;
private java.lang.Object programVersion_ = "";
/**
* optional string programVersion = 10;
*/
public boolean hasProgramVersion() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
* optional string programVersion = 10;
*/
public java.lang.String getProgramVersion() {
java.lang.Object ref = programVersion_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
programVersion_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string programVersion = 10;
*/
public com.google.protobuf.ByteString
getProgramVersionBytes() {
java.lang.Object ref = programVersion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
programVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string programVersion = 10;
*/
public Builder setProgramVersion(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000200;
programVersion_ = value;
onChanged();
return this;
}
/**
* optional string programVersion = 10;
*/
public Builder clearProgramVersion() {
bitField0_ = (bitField0_ & ~0x00000200);
programVersion_ = getDefaultInstance().getProgramVersion();
onChanged();
return this;
}
/**
* optional string programVersion = 10;
*/
public Builder setProgramVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000200;
programVersion_ = value;
onChanged();
return this;
}
// optional string os = 11;
private java.lang.Object os_ = "";
/**
* optional string os = 11;
*/
public boolean hasOs() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
* optional string os = 11;
*/
public java.lang.String getOs() {
java.lang.Object ref = os_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
os_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string os = 11;
*/
public com.google.protobuf.ByteString
getOsBytes() {
java.lang.Object ref = os_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
os_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string os = 11;
*/
public Builder setOs(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000400;
os_ = value;
onChanged();
return this;
}
/**
* optional string os = 11;
*/
public Builder clearOs() {
bitField0_ = (bitField0_ & ~0x00000400);
os_ = getDefaultInstance().getOs();
onChanged();
return this;
}
/**
* optional string os = 11;
*/
public Builder setOsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000400;
os_ = value;
onChanged();
return this;
}
// optional string osVersion = 12;
private java.lang.Object osVersion_ = "";
/**
* optional string osVersion = 12;
*/
public boolean hasOsVersion() {
return ((bitField0_ & 0x00000800) == 0x00000800);
}
/**
* optional string osVersion = 12;
*/
public java.lang.String getOsVersion() {
java.lang.Object ref = osVersion_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
osVersion_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string osVersion = 12;
*/
public com.google.protobuf.ByteString
getOsVersionBytes() {
java.lang.Object ref = osVersion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
osVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string osVersion = 12;
*/
public Builder setOsVersion(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
osVersion_ = value;
onChanged();
return this;
}
/**
* optional string osVersion = 12;
*/
public Builder clearOsVersion() {
bitField0_ = (bitField0_ & ~0x00000800);
osVersion_ = getDefaultInstance().getOsVersion();
onChanged();
return this;
}
/**
* optional string osVersion = 12;
*/
public Builder setOsVersionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
osVersion_ = value;
onChanged();
return this;
}
// optional string processId = 13;
private java.lang.Object processId_ = "";
/**
* optional string processId = 13;
*/
public boolean hasProcessId() {
return ((bitField0_ & 0x00001000) == 0x00001000);
}
/**
* optional string processId = 13;
*/
public java.lang.String getProcessId() {
java.lang.Object ref = processId_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
processId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string processId = 13;
*/
public com.google.protobuf.ByteString
getProcessIdBytes() {
java.lang.Object ref = processId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
processId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string processId = 13;
*/
public Builder setProcessId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00001000;
processId_ = value;
onChanged();
return this;
}
/**
* optional string processId = 13;
*/
public Builder clearProcessId() {
bitField0_ = (bitField0_ & ~0x00001000);
processId_ = getDefaultInstance().getProcessId();
onChanged();
return this;
}
/**
* optional string processId = 13;
*/
public Builder setProcessIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00001000;
processId_ = value;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:louie.IdentityPB)
}
static {
defaultInstance = new IdentityPB(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:louie.IdentityPB)
}
public interface SessionKeyOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional string key = 1;
/**
* optional string key = 1;
*/
boolean hasKey();
/**
* optional string key = 1;
*/
java.lang.String getKey();
/**
* optional string key = 1;
*/
com.google.protobuf.ByteString
getKeyBytes();
}
/**
* Protobuf type {@code louie.SessionKey}
*/
public static final class SessionKey extends
com.google.protobuf.GeneratedMessage
implements SessionKeyOrBuilder {
// Use SessionKey.newBuilder() to construct.
private SessionKey(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private SessionKey(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final SessionKey defaultInstance;
public static SessionKey getDefaultInstance() {
return defaultInstance;
}
public SessionKey getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SessionKey(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
key_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.rhythm.pb.RequestProtos.internal_static_louie_SessionKey_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.rhythm.pb.RequestProtos.internal_static_louie_SessionKey_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.rhythm.pb.RequestProtos.SessionKey.class, com.rhythm.pb.RequestProtos.SessionKey.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public SessionKey parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SessionKey(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional string key = 1;
public static final int KEY_FIELD_NUMBER = 1;
private java.lang.Object key_;
/**
* optional string key = 1;
*/
public boolean hasKey() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string key = 1;
*/
public java.lang.String getKey() {
java.lang.Object ref = key_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
key_ = s;
}
return s;
}
}
/**
* optional string key = 1;
*/
public com.google.protobuf.ByteString
getKeyBytes() {
java.lang.Object ref = key_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
key_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
key_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getKeyBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getKeyBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.rhythm.pb.RequestProtos.SessionKey)) {
return super.equals(obj);
}
com.rhythm.pb.RequestProtos.SessionKey other = (com.rhythm.pb.RequestProtos.SessionKey) obj;
boolean result = true;
result = result && (hasKey() == other.hasKey());
if (hasKey()) {
result = result && getKey()
.equals(other.getKey());
}
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasKey()) {
hash = (37 * hash) + KEY_FIELD_NUMBER;
hash = (53 * hash) + getKey().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.rhythm.pb.RequestProtos.SessionKey parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.rhythm.pb.RequestProtos.SessionKey parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.SessionKey parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.rhythm.pb.RequestProtos.SessionKey parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.SessionKey parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.rhythm.pb.RequestProtos.SessionKey parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.SessionKey parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.rhythm.pb.RequestProtos.SessionKey parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.SessionKey parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.rhythm.pb.RequestProtos.SessionKey parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.rhythm.pb.RequestProtos.SessionKey prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code louie.SessionKey}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.rhythm.pb.RequestProtos.SessionKeyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.rhythm.pb.RequestProtos.internal_static_louie_SessionKey_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.rhythm.pb.RequestProtos.internal_static_louie_SessionKey_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.rhythm.pb.RequestProtos.SessionKey.class, com.rhythm.pb.RequestProtos.SessionKey.Builder.class);
}
// Construct using com.rhythm.pb.RequestProtos.SessionKey.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
key_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.rhythm.pb.RequestProtos.internal_static_louie_SessionKey_descriptor;
}
public com.rhythm.pb.RequestProtos.SessionKey getDefaultInstanceForType() {
return com.rhythm.pb.RequestProtos.SessionKey.getDefaultInstance();
}
public com.rhythm.pb.RequestProtos.SessionKey build() {
com.rhythm.pb.RequestProtos.SessionKey result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.rhythm.pb.RequestProtos.SessionKey buildPartial() {
com.rhythm.pb.RequestProtos.SessionKey result = new com.rhythm.pb.RequestProtos.SessionKey(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.key_ = key_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.rhythm.pb.RequestProtos.SessionKey) {
return mergeFrom((com.rhythm.pb.RequestProtos.SessionKey)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.rhythm.pb.RequestProtos.SessionKey other) {
if (other == com.rhythm.pb.RequestProtos.SessionKey.getDefaultInstance()) return this;
if (other.hasKey()) {
bitField0_ |= 0x00000001;
key_ = other.key_;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.rhythm.pb.RequestProtos.SessionKey parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.rhythm.pb.RequestProtos.SessionKey) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional string key = 1;
private java.lang.Object key_ = "";
/**
* optional string key = 1;
*/
public boolean hasKey() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string key = 1;
*/
public java.lang.String getKey() {
java.lang.Object ref = key_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
key_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string key = 1;
*/
public com.google.protobuf.ByteString
getKeyBytes() {
java.lang.Object ref = key_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
key_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string key = 1;
*/
public Builder setKey(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
key_ = value;
onChanged();
return this;
}
/**
* optional string key = 1;
*/
public Builder clearKey() {
bitField0_ = (bitField0_ & ~0x00000001);
key_ = getDefaultInstance().getKey();
onChanged();
return this;
}
/**
* optional string key = 1;
*/
public Builder setKeyBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
key_ = value;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:louie.SessionKey)
}
static {
defaultInstance = new SessionKey(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:louie.SessionKey)
}
public interface SessionBPBOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional .louie.SessionKey key = 1;
/**
* optional .louie.SessionKey key = 1;
*/
boolean hasKey();
/**
* optional .louie.SessionKey key = 1;
*/
com.rhythm.pb.RequestProtos.SessionKey getKey();
/**
* optional .louie.SessionKey key = 1;
*/
com.rhythm.pb.RequestProtos.SessionKeyOrBuilder getKeyOrBuilder();
// optional .louie.IdentityPB identity = 2;
/**
* optional .louie.IdentityPB identity = 2;
*/
boolean hasIdentity();
/**
* optional .louie.IdentityPB identity = 2;
*/
com.rhythm.pb.RequestProtos.IdentityPB getIdentity();
/**
* optional .louie.IdentityPB identity = 2;
*/
com.rhythm.pb.RequestProtos.IdentityPBOrBuilder getIdentityOrBuilder();
// optional .louie.SessionStatsPB stats = 3;
/**
* optional .louie.SessionStatsPB stats = 3;
*/
boolean hasStats();
/**
* optional .louie.SessionStatsPB stats = 3;
*/
com.rhythm.pb.RequestProtos.SessionStatsPB getStats();
/**
* optional .louie.SessionStatsPB stats = 3;
*/
com.rhythm.pb.RequestProtos.SessionStatsPBOrBuilder getStatsOrBuilder();
}
/**
* Protobuf type {@code louie.SessionBPB}
*/
public static final class SessionBPB extends
com.google.protobuf.GeneratedMessage
implements SessionBPBOrBuilder {
// Use SessionBPB.newBuilder() to construct.
private SessionBPB(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private SessionBPB(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final SessionBPB defaultInstance;
public static SessionBPB getDefaultInstance() {
return defaultInstance;
}
public SessionBPB getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SessionBPB(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
com.rhythm.pb.RequestProtos.SessionKey.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
subBuilder = key_.toBuilder();
}
key_ = input.readMessage(com.rhythm.pb.RequestProtos.SessionKey.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(key_);
key_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 18: {
com.rhythm.pb.RequestProtos.IdentityPB.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = identity_.toBuilder();
}
identity_ = input.readMessage(com.rhythm.pb.RequestProtos.IdentityPB.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(identity_);
identity_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
case 26: {
com.rhythm.pb.RequestProtos.SessionStatsPB.Builder subBuilder = null;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
subBuilder = stats_.toBuilder();
}
stats_ = input.readMessage(com.rhythm.pb.RequestProtos.SessionStatsPB.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(stats_);
stats_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000004;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.rhythm.pb.RequestProtos.internal_static_louie_SessionBPB_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.rhythm.pb.RequestProtos.internal_static_louie_SessionBPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.rhythm.pb.RequestProtos.SessionBPB.class, com.rhythm.pb.RequestProtos.SessionBPB.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public SessionBPB parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SessionBPB(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional .louie.SessionKey key = 1;
public static final int KEY_FIELD_NUMBER = 1;
private com.rhythm.pb.RequestProtos.SessionKey key_;
/**
* optional .louie.SessionKey key = 1;
*/
public boolean hasKey() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .louie.SessionKey key = 1;
*/
public com.rhythm.pb.RequestProtos.SessionKey getKey() {
return key_;
}
/**
* optional .louie.SessionKey key = 1;
*/
public com.rhythm.pb.RequestProtos.SessionKeyOrBuilder getKeyOrBuilder() {
return key_;
}
// optional .louie.IdentityPB identity = 2;
public static final int IDENTITY_FIELD_NUMBER = 2;
private com.rhythm.pb.RequestProtos.IdentityPB identity_;
/**
* optional .louie.IdentityPB identity = 2;
*/
public boolean hasIdentity() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .louie.IdentityPB identity = 2;
*/
public com.rhythm.pb.RequestProtos.IdentityPB getIdentity() {
return identity_;
}
/**
* optional .louie.IdentityPB identity = 2;
*/
public com.rhythm.pb.RequestProtos.IdentityPBOrBuilder getIdentityOrBuilder() {
return identity_;
}
// optional .louie.SessionStatsPB stats = 3;
public static final int STATS_FIELD_NUMBER = 3;
private com.rhythm.pb.RequestProtos.SessionStatsPB stats_;
/**
* optional .louie.SessionStatsPB stats = 3;
*/
public boolean hasStats() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .louie.SessionStatsPB stats = 3;
*/
public com.rhythm.pb.RequestProtos.SessionStatsPB getStats() {
return stats_;
}
/**
* optional .louie.SessionStatsPB stats = 3;
*/
public com.rhythm.pb.RequestProtos.SessionStatsPBOrBuilder getStatsOrBuilder() {
return stats_;
}
private void initFields() {
key_ = com.rhythm.pb.RequestProtos.SessionKey.getDefaultInstance();
identity_ = com.rhythm.pb.RequestProtos.IdentityPB.getDefaultInstance();
stats_ = com.rhythm.pb.RequestProtos.SessionStatsPB.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeMessage(1, key_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(2, identity_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeMessage(3, stats_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, key_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, identity_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, stats_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.rhythm.pb.RequestProtos.SessionBPB)) {
return super.equals(obj);
}
com.rhythm.pb.RequestProtos.SessionBPB other = (com.rhythm.pb.RequestProtos.SessionBPB) obj;
boolean result = true;
result = result && (hasKey() == other.hasKey());
if (hasKey()) {
result = result && getKey()
.equals(other.getKey());
}
result = result && (hasIdentity() == other.hasIdentity());
if (hasIdentity()) {
result = result && getIdentity()
.equals(other.getIdentity());
}
result = result && (hasStats() == other.hasStats());
if (hasStats()) {
result = result && getStats()
.equals(other.getStats());
}
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasKey()) {
hash = (37 * hash) + KEY_FIELD_NUMBER;
hash = (53 * hash) + getKey().hashCode();
}
if (hasIdentity()) {
hash = (37 * hash) + IDENTITY_FIELD_NUMBER;
hash = (53 * hash) + getIdentity().hashCode();
}
if (hasStats()) {
hash = (37 * hash) + STATS_FIELD_NUMBER;
hash = (53 * hash) + getStats().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.rhythm.pb.RequestProtos.SessionBPB parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.rhythm.pb.RequestProtos.SessionBPB parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.SessionBPB parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.rhythm.pb.RequestProtos.SessionBPB parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.SessionBPB parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.rhythm.pb.RequestProtos.SessionBPB parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.SessionBPB parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.rhythm.pb.RequestProtos.SessionBPB parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.SessionBPB parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.rhythm.pb.RequestProtos.SessionBPB parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.rhythm.pb.RequestProtos.SessionBPB prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code louie.SessionBPB}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.rhythm.pb.RequestProtos.SessionBPBOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.rhythm.pb.RequestProtos.internal_static_louie_SessionBPB_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.rhythm.pb.RequestProtos.internal_static_louie_SessionBPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.rhythm.pb.RequestProtos.SessionBPB.class, com.rhythm.pb.RequestProtos.SessionBPB.Builder.class);
}
// Construct using com.rhythm.pb.RequestProtos.SessionBPB.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getKeyFieldBuilder();
getIdentityFieldBuilder();
getStatsFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
if (keyBuilder_ == null) {
key_ = com.rhythm.pb.RequestProtos.SessionKey.getDefaultInstance();
} else {
keyBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
if (identityBuilder_ == null) {
identity_ = com.rhythm.pb.RequestProtos.IdentityPB.getDefaultInstance();
} else {
identityBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
if (statsBuilder_ == null) {
stats_ = com.rhythm.pb.RequestProtos.SessionStatsPB.getDefaultInstance();
} else {
statsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.rhythm.pb.RequestProtos.internal_static_louie_SessionBPB_descriptor;
}
public com.rhythm.pb.RequestProtos.SessionBPB getDefaultInstanceForType() {
return com.rhythm.pb.RequestProtos.SessionBPB.getDefaultInstance();
}
public com.rhythm.pb.RequestProtos.SessionBPB build() {
com.rhythm.pb.RequestProtos.SessionBPB result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.rhythm.pb.RequestProtos.SessionBPB buildPartial() {
com.rhythm.pb.RequestProtos.SessionBPB result = new com.rhythm.pb.RequestProtos.SessionBPB(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
if (keyBuilder_ == null) {
result.key_ = key_;
} else {
result.key_ = keyBuilder_.build();
}
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
if (identityBuilder_ == null) {
result.identity_ = identity_;
} else {
result.identity_ = identityBuilder_.build();
}
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
if (statsBuilder_ == null) {
result.stats_ = stats_;
} else {
result.stats_ = statsBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.rhythm.pb.RequestProtos.SessionBPB) {
return mergeFrom((com.rhythm.pb.RequestProtos.SessionBPB)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.rhythm.pb.RequestProtos.SessionBPB other) {
if (other == com.rhythm.pb.RequestProtos.SessionBPB.getDefaultInstance()) return this;
if (other.hasKey()) {
mergeKey(other.getKey());
}
if (other.hasIdentity()) {
mergeIdentity(other.getIdentity());
}
if (other.hasStats()) {
mergeStats(other.getStats());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.rhythm.pb.RequestProtos.SessionBPB parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.rhythm.pb.RequestProtos.SessionBPB) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional .louie.SessionKey key = 1;
private com.rhythm.pb.RequestProtos.SessionKey key_ = com.rhythm.pb.RequestProtos.SessionKey.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.rhythm.pb.RequestProtos.SessionKey, com.rhythm.pb.RequestProtos.SessionKey.Builder, com.rhythm.pb.RequestProtos.SessionKeyOrBuilder> keyBuilder_;
/**
* optional .louie.SessionKey key = 1;
*/
public boolean hasKey() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional .louie.SessionKey key = 1;
*/
public com.rhythm.pb.RequestProtos.SessionKey getKey() {
if (keyBuilder_ == null) {
return key_;
} else {
return keyBuilder_.getMessage();
}
}
/**
* optional .louie.SessionKey key = 1;
*/
public Builder setKey(com.rhythm.pb.RequestProtos.SessionKey value) {
if (keyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
key_ = value;
onChanged();
} else {
keyBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .louie.SessionKey key = 1;
*/
public Builder setKey(
com.rhythm.pb.RequestProtos.SessionKey.Builder builderForValue) {
if (keyBuilder_ == null) {
key_ = builderForValue.build();
onChanged();
} else {
keyBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .louie.SessionKey key = 1;
*/
public Builder mergeKey(com.rhythm.pb.RequestProtos.SessionKey value) {
if (keyBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001) &&
key_ != com.rhythm.pb.RequestProtos.SessionKey.getDefaultInstance()) {
key_ =
com.rhythm.pb.RequestProtos.SessionKey.newBuilder(key_).mergeFrom(value).buildPartial();
} else {
key_ = value;
}
onChanged();
} else {
keyBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
* optional .louie.SessionKey key = 1;
*/
public Builder clearKey() {
if (keyBuilder_ == null) {
key_ = com.rhythm.pb.RequestProtos.SessionKey.getDefaultInstance();
onChanged();
} else {
keyBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
* optional .louie.SessionKey key = 1;
*/
public com.rhythm.pb.RequestProtos.SessionKey.Builder getKeyBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getKeyFieldBuilder().getBuilder();
}
/**
* optional .louie.SessionKey key = 1;
*/
public com.rhythm.pb.RequestProtos.SessionKeyOrBuilder getKeyOrBuilder() {
if (keyBuilder_ != null) {
return keyBuilder_.getMessageOrBuilder();
} else {
return key_;
}
}
/**
* optional .louie.SessionKey key = 1;
*/
private com.google.protobuf.SingleFieldBuilder<
com.rhythm.pb.RequestProtos.SessionKey, com.rhythm.pb.RequestProtos.SessionKey.Builder, com.rhythm.pb.RequestProtos.SessionKeyOrBuilder>
getKeyFieldBuilder() {
if (keyBuilder_ == null) {
keyBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.rhythm.pb.RequestProtos.SessionKey, com.rhythm.pb.RequestProtos.SessionKey.Builder, com.rhythm.pb.RequestProtos.SessionKeyOrBuilder>(
key_,
getParentForChildren(),
isClean());
key_ = null;
}
return keyBuilder_;
}
// optional .louie.IdentityPB identity = 2;
private com.rhythm.pb.RequestProtos.IdentityPB identity_ = com.rhythm.pb.RequestProtos.IdentityPB.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.rhythm.pb.RequestProtos.IdentityPB, com.rhythm.pb.RequestProtos.IdentityPB.Builder, com.rhythm.pb.RequestProtos.IdentityPBOrBuilder> identityBuilder_;
/**
* optional .louie.IdentityPB identity = 2;
*/
public boolean hasIdentity() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .louie.IdentityPB identity = 2;
*/
public com.rhythm.pb.RequestProtos.IdentityPB getIdentity() {
if (identityBuilder_ == null) {
return identity_;
} else {
return identityBuilder_.getMessage();
}
}
/**
* optional .louie.IdentityPB identity = 2;
*/
public Builder setIdentity(com.rhythm.pb.RequestProtos.IdentityPB value) {
if (identityBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
identity_ = value;
onChanged();
} else {
identityBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .louie.IdentityPB identity = 2;
*/
public Builder setIdentity(
com.rhythm.pb.RequestProtos.IdentityPB.Builder builderForValue) {
if (identityBuilder_ == null) {
identity_ = builderForValue.build();
onChanged();
} else {
identityBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .louie.IdentityPB identity = 2;
*/
public Builder mergeIdentity(com.rhythm.pb.RequestProtos.IdentityPB value) {
if (identityBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
identity_ != com.rhythm.pb.RequestProtos.IdentityPB.getDefaultInstance()) {
identity_ =
com.rhythm.pb.RequestProtos.IdentityPB.newBuilder(identity_).mergeFrom(value).buildPartial();
} else {
identity_ = value;
}
onChanged();
} else {
identityBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .louie.IdentityPB identity = 2;
*/
public Builder clearIdentity() {
if (identityBuilder_ == null) {
identity_ = com.rhythm.pb.RequestProtos.IdentityPB.getDefaultInstance();
onChanged();
} else {
identityBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* optional .louie.IdentityPB identity = 2;
*/
public com.rhythm.pb.RequestProtos.IdentityPB.Builder getIdentityBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getIdentityFieldBuilder().getBuilder();
}
/**
* optional .louie.IdentityPB identity = 2;
*/
public com.rhythm.pb.RequestProtos.IdentityPBOrBuilder getIdentityOrBuilder() {
if (identityBuilder_ != null) {
return identityBuilder_.getMessageOrBuilder();
} else {
return identity_;
}
}
/**
* optional .louie.IdentityPB identity = 2;
*/
private com.google.protobuf.SingleFieldBuilder<
com.rhythm.pb.RequestProtos.IdentityPB, com.rhythm.pb.RequestProtos.IdentityPB.Builder, com.rhythm.pb.RequestProtos.IdentityPBOrBuilder>
getIdentityFieldBuilder() {
if (identityBuilder_ == null) {
identityBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.rhythm.pb.RequestProtos.IdentityPB, com.rhythm.pb.RequestProtos.IdentityPB.Builder, com.rhythm.pb.RequestProtos.IdentityPBOrBuilder>(
identity_,
getParentForChildren(),
isClean());
identity_ = null;
}
return identityBuilder_;
}
// optional .louie.SessionStatsPB stats = 3;
private com.rhythm.pb.RequestProtos.SessionStatsPB stats_ = com.rhythm.pb.RequestProtos.SessionStatsPB.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.rhythm.pb.RequestProtos.SessionStatsPB, com.rhythm.pb.RequestProtos.SessionStatsPB.Builder, com.rhythm.pb.RequestProtos.SessionStatsPBOrBuilder> statsBuilder_;
/**
* optional .louie.SessionStatsPB stats = 3;
*/
public boolean hasStats() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional .louie.SessionStatsPB stats = 3;
*/
public com.rhythm.pb.RequestProtos.SessionStatsPB getStats() {
if (statsBuilder_ == null) {
return stats_;
} else {
return statsBuilder_.getMessage();
}
}
/**
* optional .louie.SessionStatsPB stats = 3;
*/
public Builder setStats(com.rhythm.pb.RequestProtos.SessionStatsPB value) {
if (statsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
stats_ = value;
onChanged();
} else {
statsBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .louie.SessionStatsPB stats = 3;
*/
public Builder setStats(
com.rhythm.pb.RequestProtos.SessionStatsPB.Builder builderForValue) {
if (statsBuilder_ == null) {
stats_ = builderForValue.build();
onChanged();
} else {
statsBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .louie.SessionStatsPB stats = 3;
*/
public Builder mergeStats(com.rhythm.pb.RequestProtos.SessionStatsPB value) {
if (statsBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004) &&
stats_ != com.rhythm.pb.RequestProtos.SessionStatsPB.getDefaultInstance()) {
stats_ =
com.rhythm.pb.RequestProtos.SessionStatsPB.newBuilder(stats_).mergeFrom(value).buildPartial();
} else {
stats_ = value;
}
onChanged();
} else {
statsBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
* optional .louie.SessionStatsPB stats = 3;
*/
public Builder clearStats() {
if (statsBuilder_ == null) {
stats_ = com.rhythm.pb.RequestProtos.SessionStatsPB.getDefaultInstance();
onChanged();
} else {
statsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
/**
* optional .louie.SessionStatsPB stats = 3;
*/
public com.rhythm.pb.RequestProtos.SessionStatsPB.Builder getStatsBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getStatsFieldBuilder().getBuilder();
}
/**
* optional .louie.SessionStatsPB stats = 3;
*/
public com.rhythm.pb.RequestProtos.SessionStatsPBOrBuilder getStatsOrBuilder() {
if (statsBuilder_ != null) {
return statsBuilder_.getMessageOrBuilder();
} else {
return stats_;
}
}
/**
* optional .louie.SessionStatsPB stats = 3;
*/
private com.google.protobuf.SingleFieldBuilder<
com.rhythm.pb.RequestProtos.SessionStatsPB, com.rhythm.pb.RequestProtos.SessionStatsPB.Builder, com.rhythm.pb.RequestProtos.SessionStatsPBOrBuilder>
getStatsFieldBuilder() {
if (statsBuilder_ == null) {
statsBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.rhythm.pb.RequestProtos.SessionStatsPB, com.rhythm.pb.RequestProtos.SessionStatsPB.Builder, com.rhythm.pb.RequestProtos.SessionStatsPBOrBuilder>(
stats_,
getParentForChildren(),
isClean());
stats_ = null;
}
return statsBuilder_;
}
// @@protoc_insertion_point(builder_scope:louie.SessionBPB)
}
static {
defaultInstance = new SessionBPB(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:louie.SessionBPB)
}
public interface SessionStatsPBOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional sint32 requestCount = 1;
/**
* optional sint32 requestCount = 1;
*/
boolean hasRequestCount();
/**
* optional sint32 requestCount = 1;
*/
int getRequestCount();
// optional uint64 createTime = 2;
/**
* optional uint64 createTime = 2;
*/
boolean hasCreateTime();
/**
* optional uint64 createTime = 2;
*/
long getCreateTime();
// optional uint64 lastRequestTime = 3;
/**
* optional uint64 lastRequestTime = 3;
*/
boolean hasLastRequestTime();
/**
* optional uint64 lastRequestTime = 3;
*/
long getLastRequestTime();
}
/**
* Protobuf type {@code louie.SessionStatsPB}
*/
public static final class SessionStatsPB extends
com.google.protobuf.GeneratedMessage
implements SessionStatsPBOrBuilder {
// Use SessionStatsPB.newBuilder() to construct.
private SessionStatsPB(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private SessionStatsPB(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final SessionStatsPB defaultInstance;
public static SessionStatsPB getDefaultInstance() {
return defaultInstance;
}
public SessionStatsPB getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SessionStatsPB(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
requestCount_ = input.readSInt32();
break;
}
case 16: {
bitField0_ |= 0x00000002;
createTime_ = input.readUInt64();
break;
}
case 24: {
bitField0_ |= 0x00000004;
lastRequestTime_ = input.readUInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.rhythm.pb.RequestProtos.internal_static_louie_SessionStatsPB_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.rhythm.pb.RequestProtos.internal_static_louie_SessionStatsPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.rhythm.pb.RequestProtos.SessionStatsPB.class, com.rhythm.pb.RequestProtos.SessionStatsPB.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public SessionStatsPB parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SessionStatsPB(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional sint32 requestCount = 1;
public static final int REQUESTCOUNT_FIELD_NUMBER = 1;
private int requestCount_;
/**
* optional sint32 requestCount = 1;
*/
public boolean hasRequestCount() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional sint32 requestCount = 1;
*/
public int getRequestCount() {
return requestCount_;
}
// optional uint64 createTime = 2;
public static final int CREATETIME_FIELD_NUMBER = 2;
private long createTime_;
/**
* optional uint64 createTime = 2;
*/
public boolean hasCreateTime() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional uint64 createTime = 2;
*/
public long getCreateTime() {
return createTime_;
}
// optional uint64 lastRequestTime = 3;
public static final int LASTREQUESTTIME_FIELD_NUMBER = 3;
private long lastRequestTime_;
/**
* optional uint64 lastRequestTime = 3;
*/
public boolean hasLastRequestTime() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional uint64 lastRequestTime = 3;
*/
public long getLastRequestTime() {
return lastRequestTime_;
}
private void initFields() {
requestCount_ = 0;
createTime_ = 0L;
lastRequestTime_ = 0L;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeSInt32(1, requestCount_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeUInt64(2, createTime_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeUInt64(3, lastRequestTime_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeSInt32Size(1, requestCount_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(2, createTime_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(3, lastRequestTime_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.rhythm.pb.RequestProtos.SessionStatsPB)) {
return super.equals(obj);
}
com.rhythm.pb.RequestProtos.SessionStatsPB other = (com.rhythm.pb.RequestProtos.SessionStatsPB) obj;
boolean result = true;
result = result && (hasRequestCount() == other.hasRequestCount());
if (hasRequestCount()) {
result = result && (getRequestCount()
== other.getRequestCount());
}
result = result && (hasCreateTime() == other.hasCreateTime());
if (hasCreateTime()) {
result = result && (getCreateTime()
== other.getCreateTime());
}
result = result && (hasLastRequestTime() == other.hasLastRequestTime());
if (hasLastRequestTime()) {
result = result && (getLastRequestTime()
== other.getLastRequestTime());
}
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasRequestCount()) {
hash = (37 * hash) + REQUESTCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getRequestCount();
}
if (hasCreateTime()) {
hash = (37 * hash) + CREATETIME_FIELD_NUMBER;
hash = (53 * hash) + hashLong(getCreateTime());
}
if (hasLastRequestTime()) {
hash = (37 * hash) + LASTREQUESTTIME_FIELD_NUMBER;
hash = (53 * hash) + hashLong(getLastRequestTime());
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.rhythm.pb.RequestProtos.SessionStatsPB parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.rhythm.pb.RequestProtos.SessionStatsPB parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.SessionStatsPB parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.rhythm.pb.RequestProtos.SessionStatsPB parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.SessionStatsPB parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.rhythm.pb.RequestProtos.SessionStatsPB parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.SessionStatsPB parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.rhythm.pb.RequestProtos.SessionStatsPB parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.SessionStatsPB parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.rhythm.pb.RequestProtos.SessionStatsPB parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.rhythm.pb.RequestProtos.SessionStatsPB prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code louie.SessionStatsPB}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.rhythm.pb.RequestProtos.SessionStatsPBOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.rhythm.pb.RequestProtos.internal_static_louie_SessionStatsPB_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.rhythm.pb.RequestProtos.internal_static_louie_SessionStatsPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.rhythm.pb.RequestProtos.SessionStatsPB.class, com.rhythm.pb.RequestProtos.SessionStatsPB.Builder.class);
}
// Construct using com.rhythm.pb.RequestProtos.SessionStatsPB.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
requestCount_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
createTime_ = 0L;
bitField0_ = (bitField0_ & ~0x00000002);
lastRequestTime_ = 0L;
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.rhythm.pb.RequestProtos.internal_static_louie_SessionStatsPB_descriptor;
}
public com.rhythm.pb.RequestProtos.SessionStatsPB getDefaultInstanceForType() {
return com.rhythm.pb.RequestProtos.SessionStatsPB.getDefaultInstance();
}
public com.rhythm.pb.RequestProtos.SessionStatsPB build() {
com.rhythm.pb.RequestProtos.SessionStatsPB result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.rhythm.pb.RequestProtos.SessionStatsPB buildPartial() {
com.rhythm.pb.RequestProtos.SessionStatsPB result = new com.rhythm.pb.RequestProtos.SessionStatsPB(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.requestCount_ = requestCount_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.createTime_ = createTime_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.lastRequestTime_ = lastRequestTime_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.rhythm.pb.RequestProtos.SessionStatsPB) {
return mergeFrom((com.rhythm.pb.RequestProtos.SessionStatsPB)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.rhythm.pb.RequestProtos.SessionStatsPB other) {
if (other == com.rhythm.pb.RequestProtos.SessionStatsPB.getDefaultInstance()) return this;
if (other.hasRequestCount()) {
setRequestCount(other.getRequestCount());
}
if (other.hasCreateTime()) {
setCreateTime(other.getCreateTime());
}
if (other.hasLastRequestTime()) {
setLastRequestTime(other.getLastRequestTime());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.rhythm.pb.RequestProtos.SessionStatsPB parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.rhythm.pb.RequestProtos.SessionStatsPB) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional sint32 requestCount = 1;
private int requestCount_ ;
/**
* optional sint32 requestCount = 1;
*/
public boolean hasRequestCount() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional sint32 requestCount = 1;
*/
public int getRequestCount() {
return requestCount_;
}
/**
* optional sint32 requestCount = 1;
*/
public Builder setRequestCount(int value) {
bitField0_ |= 0x00000001;
requestCount_ = value;
onChanged();
return this;
}
/**
* optional sint32 requestCount = 1;
*/
public Builder clearRequestCount() {
bitField0_ = (bitField0_ & ~0x00000001);
requestCount_ = 0;
onChanged();
return this;
}
// optional uint64 createTime = 2;
private long createTime_ ;
/**
* optional uint64 createTime = 2;
*/
public boolean hasCreateTime() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional uint64 createTime = 2;
*/
public long getCreateTime() {
return createTime_;
}
/**
* optional uint64 createTime = 2;
*/
public Builder setCreateTime(long value) {
bitField0_ |= 0x00000002;
createTime_ = value;
onChanged();
return this;
}
/**
* optional uint64 createTime = 2;
*/
public Builder clearCreateTime() {
bitField0_ = (bitField0_ & ~0x00000002);
createTime_ = 0L;
onChanged();
return this;
}
// optional uint64 lastRequestTime = 3;
private long lastRequestTime_ ;
/**
* optional uint64 lastRequestTime = 3;
*/
public boolean hasLastRequestTime() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional uint64 lastRequestTime = 3;
*/
public long getLastRequestTime() {
return lastRequestTime_;
}
/**
* optional uint64 lastRequestTime = 3;
*/
public Builder setLastRequestTime(long value) {
bitField0_ |= 0x00000004;
lastRequestTime_ = value;
onChanged();
return this;
}
/**
* optional uint64 lastRequestTime = 3;
*/
public Builder clearLastRequestTime() {
bitField0_ = (bitField0_ & ~0x00000004);
lastRequestTime_ = 0L;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:louie.SessionStatsPB)
}
static {
defaultInstance = new SessionStatsPB(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:louie.SessionStatsPB)
}
public interface RequestPBOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional uint32 id = 1;
/**
* optional uint32 id = 1;
*/
boolean hasId();
/**
* optional uint32 id = 1;
*/
int getId();
// optional string service = 2;
/**
* optional string service = 2;
*/
boolean hasService();
/**
* optional string service = 2;
*/
java.lang.String getService();
/**
* optional string service = 2;
*/
com.google.protobuf.ByteString
getServiceBytes();
// optional string method = 3;
/**
* optional string method = 3;
*/
boolean hasMethod();
/**
* optional string method = 3;
*/
java.lang.String getMethod();
/**
* optional string method = 3;
*/
com.google.protobuf.ByteString
getMethodBytes();
// repeated string type = 4;
/**
* repeated string type = 4;
*/
java.util.List
getTypeList();
/**
* repeated string type = 4;
*/
int getTypeCount();
/**
* repeated string type = 4;
*/
java.lang.String getType(int index);
/**
* repeated string type = 4;
*/
com.google.protobuf.ByteString
getTypeBytes(int index);
// optional uint32 param_count = 5 [deprecated = true];
/**
* optional uint32 param_count = 5 [deprecated = true];
*/
@java.lang.Deprecated boolean hasParamCount();
/**
* optional uint32 param_count = 5 [deprecated = true];
*/
@java.lang.Deprecated int getParamCount();
// repeated .louie.RoutePB route = 20;
/**
* repeated .louie.RoutePB route = 20;
*/
java.util.List
getRouteList();
/**
* repeated .louie.RoutePB route = 20;
*/
com.rhythm.pb.RequestProtos.RoutePB getRoute(int index);
/**
* repeated .louie.RoutePB route = 20;
*/
int getRouteCount();
/**
* repeated .louie.RoutePB route = 20;
*/
java.util.List extends com.rhythm.pb.RequestProtos.RoutePBOrBuilder>
getRouteOrBuilderList();
/**
* repeated .louie.RoutePB route = 20;
*/
com.rhythm.pb.RequestProtos.RoutePBOrBuilder getRouteOrBuilder(
int index);
// optional string routeUser = 21;
/**
* optional string routeUser = 21;
*/
boolean hasRouteUser();
/**
* optional string routeUser = 21;
*/
java.lang.String getRouteUser();
/**
* optional string routeUser = 21;
*/
com.google.protobuf.ByteString
getRouteUserBytes();
// optional sint64 threadId = 22;
/**
* optional sint64 threadId = 22;
*/
boolean hasThreadId();
/**
* optional sint64 threadId = 22;
*/
long getThreadId();
// optional sint64 start_time = 23;
/**
* optional sint64 start_time = 23;
*/
boolean hasStartTime();
/**
* optional sint64 start_time = 23;
*/
long getStartTime();
}
/**
* Protobuf type {@code louie.RequestPB}
*/
public static final class RequestPB extends
com.google.protobuf.GeneratedMessage
implements RequestPBOrBuilder {
// Use RequestPB.newBuilder() to construct.
private RequestPB(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private RequestPB(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final RequestPB defaultInstance;
public static RequestPB getDefaultInstance() {
return defaultInstance;
}
public RequestPB getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RequestPB(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
id_ = input.readUInt32();
break;
}
case 18: {
bitField0_ |= 0x00000002;
service_ = input.readBytes();
break;
}
case 26: {
bitField0_ |= 0x00000004;
method_ = input.readBytes();
break;
}
case 34: {
if (!((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
type_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000008;
}
type_.add(input.readBytes());
break;
}
case 40: {
bitField0_ |= 0x00000008;
paramCount_ = input.readUInt32();
break;
}
case 162: {
if (!((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
route_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000020;
}
route_.add(input.readMessage(com.rhythm.pb.RequestProtos.RoutePB.PARSER, extensionRegistry));
break;
}
case 170: {
bitField0_ |= 0x00000010;
routeUser_ = input.readBytes();
break;
}
case 176: {
bitField0_ |= 0x00000020;
threadId_ = input.readSInt64();
break;
}
case 184: {
bitField0_ |= 0x00000040;
startTime_ = input.readSInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
type_ = new com.google.protobuf.UnmodifiableLazyStringList(type_);
}
if (((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
route_ = java.util.Collections.unmodifiableList(route_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.rhythm.pb.RequestProtos.internal_static_louie_RequestPB_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.rhythm.pb.RequestProtos.internal_static_louie_RequestPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.rhythm.pb.RequestProtos.RequestPB.class, com.rhythm.pb.RequestProtos.RequestPB.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public RequestPB parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RequestPB(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional uint32 id = 1;
public static final int ID_FIELD_NUMBER = 1;
private int id_;
/**
* optional uint32 id = 1;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional uint32 id = 1;
*/
public int getId() {
return id_;
}
// optional string service = 2;
public static final int SERVICE_FIELD_NUMBER = 2;
private java.lang.Object service_;
/**
* optional string service = 2;
*/
public boolean hasService() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string service = 2;
*/
public java.lang.String getService() {
java.lang.Object ref = service_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
service_ = s;
}
return s;
}
}
/**
* optional string service = 2;
*/
public com.google.protobuf.ByteString
getServiceBytes() {
java.lang.Object ref = service_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
service_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string method = 3;
public static final int METHOD_FIELD_NUMBER = 3;
private java.lang.Object method_;
/**
* optional string method = 3;
*/
public boolean hasMethod() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string method = 3;
*/
public java.lang.String getMethod() {
java.lang.Object ref = method_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
method_ = s;
}
return s;
}
}
/**
* optional string method = 3;
*/
public com.google.protobuf.ByteString
getMethodBytes() {
java.lang.Object ref = method_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
method_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// repeated string type = 4;
public static final int TYPE_FIELD_NUMBER = 4;
private com.google.protobuf.LazyStringList type_;
/**
* repeated string type = 4;
*/
public java.util.List
getTypeList() {
return type_;
}
/**
* repeated string type = 4;
*/
public int getTypeCount() {
return type_.size();
}
/**
* repeated string type = 4;
*/
public java.lang.String getType(int index) {
return type_.get(index);
}
/**
* repeated string type = 4;
*/
public com.google.protobuf.ByteString
getTypeBytes(int index) {
return type_.getByteString(index);
}
// optional uint32 param_count = 5 [deprecated = true];
public static final int PARAM_COUNT_FIELD_NUMBER = 5;
private int paramCount_;
/**
* optional uint32 param_count = 5 [deprecated = true];
*/
@java.lang.Deprecated public boolean hasParamCount() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional uint32 param_count = 5 [deprecated = true];
*/
@java.lang.Deprecated public int getParamCount() {
return paramCount_;
}
// repeated .louie.RoutePB route = 20;
public static final int ROUTE_FIELD_NUMBER = 20;
private java.util.List route_;
/**
* repeated .louie.RoutePB route = 20;
*/
public java.util.List getRouteList() {
return route_;
}
/**
* repeated .louie.RoutePB route = 20;
*/
public java.util.List extends com.rhythm.pb.RequestProtos.RoutePBOrBuilder>
getRouteOrBuilderList() {
return route_;
}
/**
* repeated .louie.RoutePB route = 20;
*/
public int getRouteCount() {
return route_.size();
}
/**
* repeated .louie.RoutePB route = 20;
*/
public com.rhythm.pb.RequestProtos.RoutePB getRoute(int index) {
return route_.get(index);
}
/**
* repeated .louie.RoutePB route = 20;
*/
public com.rhythm.pb.RequestProtos.RoutePBOrBuilder getRouteOrBuilder(
int index) {
return route_.get(index);
}
// optional string routeUser = 21;
public static final int ROUTEUSER_FIELD_NUMBER = 21;
private java.lang.Object routeUser_;
/**
* optional string routeUser = 21;
*/
public boolean hasRouteUser() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional string routeUser = 21;
*/
public java.lang.String getRouteUser() {
java.lang.Object ref = routeUser_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
routeUser_ = s;
}
return s;
}
}
/**
* optional string routeUser = 21;
*/
public com.google.protobuf.ByteString
getRouteUserBytes() {
java.lang.Object ref = routeUser_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
routeUser_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional sint64 threadId = 22;
public static final int THREADID_FIELD_NUMBER = 22;
private long threadId_;
/**
* optional sint64 threadId = 22;
*/
public boolean hasThreadId() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional sint64 threadId = 22;
*/
public long getThreadId() {
return threadId_;
}
// optional sint64 start_time = 23;
public static final int START_TIME_FIELD_NUMBER = 23;
private long startTime_;
/**
* optional sint64 start_time = 23;
*/
public boolean hasStartTime() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional sint64 start_time = 23;
*/
public long getStartTime() {
return startTime_;
}
private void initFields() {
id_ = 0;
service_ = "";
method_ = "";
type_ = com.google.protobuf.LazyStringArrayList.EMPTY;
paramCount_ = 0;
route_ = java.util.Collections.emptyList();
routeUser_ = "";
threadId_ = 0L;
startTime_ = 0L;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeUInt32(1, id_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getServiceBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, getMethodBytes());
}
for (int i = 0; i < type_.size(); i++) {
output.writeBytes(4, type_.getByteString(i));
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeUInt32(5, paramCount_);
}
for (int i = 0; i < route_.size(); i++) {
output.writeMessage(20, route_.get(i));
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeBytes(21, getRouteUserBytes());
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeSInt64(22, threadId_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeSInt64(23, startTime_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, id_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getServiceBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, getMethodBytes());
}
{
int dataSize = 0;
for (int i = 0; i < type_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeBytesSizeNoTag(type_.getByteString(i));
}
size += dataSize;
size += 1 * getTypeList().size();
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(5, paramCount_);
}
for (int i = 0; i < route_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(20, route_.get(i));
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(21, getRouteUserBytes());
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(22, threadId_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(23, startTime_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.rhythm.pb.RequestProtos.RequestPB)) {
return super.equals(obj);
}
com.rhythm.pb.RequestProtos.RequestPB other = (com.rhythm.pb.RequestProtos.RequestPB) obj;
boolean result = true;
result = result && (hasId() == other.hasId());
if (hasId()) {
result = result && (getId()
== other.getId());
}
result = result && (hasService() == other.hasService());
if (hasService()) {
result = result && getService()
.equals(other.getService());
}
result = result && (hasMethod() == other.hasMethod());
if (hasMethod()) {
result = result && getMethod()
.equals(other.getMethod());
}
result = result && getTypeList()
.equals(other.getTypeList());
result = result && (hasParamCount() == other.hasParamCount());
if (hasParamCount()) {
result = result && (getParamCount()
== other.getParamCount());
}
result = result && getRouteList()
.equals(other.getRouteList());
result = result && (hasRouteUser() == other.hasRouteUser());
if (hasRouteUser()) {
result = result && getRouteUser()
.equals(other.getRouteUser());
}
result = result && (hasThreadId() == other.hasThreadId());
if (hasThreadId()) {
result = result && (getThreadId()
== other.getThreadId());
}
result = result && (hasStartTime() == other.hasStartTime());
if (hasStartTime()) {
result = result && (getStartTime()
== other.getStartTime());
}
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId();
}
if (hasService()) {
hash = (37 * hash) + SERVICE_FIELD_NUMBER;
hash = (53 * hash) + getService().hashCode();
}
if (hasMethod()) {
hash = (37 * hash) + METHOD_FIELD_NUMBER;
hash = (53 * hash) + getMethod().hashCode();
}
if (getTypeCount() > 0) {
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + getTypeList().hashCode();
}
if (hasParamCount()) {
hash = (37 * hash) + PARAM_COUNT_FIELD_NUMBER;
hash = (53 * hash) + getParamCount();
}
if (getRouteCount() > 0) {
hash = (37 * hash) + ROUTE_FIELD_NUMBER;
hash = (53 * hash) + getRouteList().hashCode();
}
if (hasRouteUser()) {
hash = (37 * hash) + ROUTEUSER_FIELD_NUMBER;
hash = (53 * hash) + getRouteUser().hashCode();
}
if (hasThreadId()) {
hash = (37 * hash) + THREADID_FIELD_NUMBER;
hash = (53 * hash) + hashLong(getThreadId());
}
if (hasStartTime()) {
hash = (37 * hash) + START_TIME_FIELD_NUMBER;
hash = (53 * hash) + hashLong(getStartTime());
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.rhythm.pb.RequestProtos.RequestPB parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.rhythm.pb.RequestProtos.RequestPB parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.RequestPB parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.rhythm.pb.RequestProtos.RequestPB parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.RequestPB parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.rhythm.pb.RequestProtos.RequestPB parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.RequestPB parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.rhythm.pb.RequestProtos.RequestPB parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.RequestPB parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.rhythm.pb.RequestProtos.RequestPB parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.rhythm.pb.RequestProtos.RequestPB prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code louie.RequestPB}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.rhythm.pb.RequestProtos.RequestPBOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.rhythm.pb.RequestProtos.internal_static_louie_RequestPB_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.rhythm.pb.RequestProtos.internal_static_louie_RequestPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.rhythm.pb.RequestProtos.RequestPB.class, com.rhythm.pb.RequestProtos.RequestPB.Builder.class);
}
// Construct using com.rhythm.pb.RequestProtos.RequestPB.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getRouteFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
id_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
service_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
method_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
type_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000008);
paramCount_ = 0;
bitField0_ = (bitField0_ & ~0x00000010);
if (routeBuilder_ == null) {
route_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
} else {
routeBuilder_.clear();
}
routeUser_ = "";
bitField0_ = (bitField0_ & ~0x00000040);
threadId_ = 0L;
bitField0_ = (bitField0_ & ~0x00000080);
startTime_ = 0L;
bitField0_ = (bitField0_ & ~0x00000100);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.rhythm.pb.RequestProtos.internal_static_louie_RequestPB_descriptor;
}
public com.rhythm.pb.RequestProtos.RequestPB getDefaultInstanceForType() {
return com.rhythm.pb.RequestProtos.RequestPB.getDefaultInstance();
}
public com.rhythm.pb.RequestProtos.RequestPB build() {
com.rhythm.pb.RequestProtos.RequestPB result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.rhythm.pb.RequestProtos.RequestPB buildPartial() {
com.rhythm.pb.RequestProtos.RequestPB result = new com.rhythm.pb.RequestProtos.RequestPB(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.id_ = id_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.service_ = service_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.method_ = method_;
if (((bitField0_ & 0x00000008) == 0x00000008)) {
type_ = new com.google.protobuf.UnmodifiableLazyStringList(
type_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.type_ = type_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000008;
}
result.paramCount_ = paramCount_;
if (routeBuilder_ == null) {
if (((bitField0_ & 0x00000020) == 0x00000020)) {
route_ = java.util.Collections.unmodifiableList(route_);
bitField0_ = (bitField0_ & ~0x00000020);
}
result.route_ = route_;
} else {
result.route_ = routeBuilder_.build();
}
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000010;
}
result.routeUser_ = routeUser_;
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000020;
}
result.threadId_ = threadId_;
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000040;
}
result.startTime_ = startTime_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.rhythm.pb.RequestProtos.RequestPB) {
return mergeFrom((com.rhythm.pb.RequestProtos.RequestPB)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.rhythm.pb.RequestProtos.RequestPB other) {
if (other == com.rhythm.pb.RequestProtos.RequestPB.getDefaultInstance()) return this;
if (other.hasId()) {
setId(other.getId());
}
if (other.hasService()) {
bitField0_ |= 0x00000002;
service_ = other.service_;
onChanged();
}
if (other.hasMethod()) {
bitField0_ |= 0x00000004;
method_ = other.method_;
onChanged();
}
if (!other.type_.isEmpty()) {
if (type_.isEmpty()) {
type_ = other.type_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureTypeIsMutable();
type_.addAll(other.type_);
}
onChanged();
}
if (other.hasParamCount()) {
setParamCount(other.getParamCount());
}
if (routeBuilder_ == null) {
if (!other.route_.isEmpty()) {
if (route_.isEmpty()) {
route_ = other.route_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensureRouteIsMutable();
route_.addAll(other.route_);
}
onChanged();
}
} else {
if (!other.route_.isEmpty()) {
if (routeBuilder_.isEmpty()) {
routeBuilder_.dispose();
routeBuilder_ = null;
route_ = other.route_;
bitField0_ = (bitField0_ & ~0x00000020);
routeBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getRouteFieldBuilder() : null;
} else {
routeBuilder_.addAllMessages(other.route_);
}
}
}
if (other.hasRouteUser()) {
bitField0_ |= 0x00000040;
routeUser_ = other.routeUser_;
onChanged();
}
if (other.hasThreadId()) {
setThreadId(other.getThreadId());
}
if (other.hasStartTime()) {
setStartTime(other.getStartTime());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.rhythm.pb.RequestProtos.RequestPB parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.rhythm.pb.RequestProtos.RequestPB) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional uint32 id = 1;
private int id_ ;
/**
* optional uint32 id = 1;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional uint32 id = 1;
*/
public int getId() {
return id_;
}
/**
* optional uint32 id = 1;
*/
public Builder setId(int value) {
bitField0_ |= 0x00000001;
id_ = value;
onChanged();
return this;
}
/**
* optional uint32 id = 1;
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000001);
id_ = 0;
onChanged();
return this;
}
// optional string service = 2;
private java.lang.Object service_ = "";
/**
* optional string service = 2;
*/
public boolean hasService() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string service = 2;
*/
public java.lang.String getService() {
java.lang.Object ref = service_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
service_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string service = 2;
*/
public com.google.protobuf.ByteString
getServiceBytes() {
java.lang.Object ref = service_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
service_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string service = 2;
*/
public Builder setService(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
service_ = value;
onChanged();
return this;
}
/**
* optional string service = 2;
*/
public Builder clearService() {
bitField0_ = (bitField0_ & ~0x00000002);
service_ = getDefaultInstance().getService();
onChanged();
return this;
}
/**
* optional string service = 2;
*/
public Builder setServiceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
service_ = value;
onChanged();
return this;
}
// optional string method = 3;
private java.lang.Object method_ = "";
/**
* optional string method = 3;
*/
public boolean hasMethod() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string method = 3;
*/
public java.lang.String getMethod() {
java.lang.Object ref = method_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
method_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string method = 3;
*/
public com.google.protobuf.ByteString
getMethodBytes() {
java.lang.Object ref = method_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
method_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string method = 3;
*/
public Builder setMethod(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
method_ = value;
onChanged();
return this;
}
/**
* optional string method = 3;
*/
public Builder clearMethod() {
bitField0_ = (bitField0_ & ~0x00000004);
method_ = getDefaultInstance().getMethod();
onChanged();
return this;
}
/**
* optional string method = 3;
*/
public Builder setMethodBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
method_ = value;
onChanged();
return this;
}
// repeated string type = 4;
private com.google.protobuf.LazyStringList type_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureTypeIsMutable() {
if (!((bitField0_ & 0x00000008) == 0x00000008)) {
type_ = new com.google.protobuf.LazyStringArrayList(type_);
bitField0_ |= 0x00000008;
}
}
/**
* repeated string type = 4;
*/
public java.util.List
getTypeList() {
return java.util.Collections.unmodifiableList(type_);
}
/**
* repeated string type = 4;
*/
public int getTypeCount() {
return type_.size();
}
/**
* repeated string type = 4;
*/
public java.lang.String getType(int index) {
return type_.get(index);
}
/**
* repeated string type = 4;
*/
public com.google.protobuf.ByteString
getTypeBytes(int index) {
return type_.getByteString(index);
}
/**
* repeated string type = 4;
*/
public Builder setType(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureTypeIsMutable();
type_.set(index, value);
onChanged();
return this;
}
/**
* repeated string type = 4;
*/
public Builder addType(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureTypeIsMutable();
type_.add(value);
onChanged();
return this;
}
/**
* repeated string type = 4;
*/
public Builder addAllType(
java.lang.Iterable values) {
ensureTypeIsMutable();
super.addAll(values, type_);
onChanged();
return this;
}
/**
* repeated string type = 4;
*/
public Builder clearType() {
type_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
/**
* repeated string type = 4;
*/
public Builder addTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
ensureTypeIsMutable();
type_.add(value);
onChanged();
return this;
}
// optional uint32 param_count = 5 [deprecated = true];
private int paramCount_ ;
/**
* optional uint32 param_count = 5 [deprecated = true];
*/
@java.lang.Deprecated public boolean hasParamCount() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional uint32 param_count = 5 [deprecated = true];
*/
@java.lang.Deprecated public int getParamCount() {
return paramCount_;
}
/**
* optional uint32 param_count = 5 [deprecated = true];
*/
@java.lang.Deprecated public Builder setParamCount(int value) {
bitField0_ |= 0x00000010;
paramCount_ = value;
onChanged();
return this;
}
/**
* optional uint32 param_count = 5 [deprecated = true];
*/
@java.lang.Deprecated public Builder clearParamCount() {
bitField0_ = (bitField0_ & ~0x00000010);
paramCount_ = 0;
onChanged();
return this;
}
// repeated .louie.RoutePB route = 20;
private java.util.List route_ =
java.util.Collections.emptyList();
private void ensureRouteIsMutable() {
if (!((bitField0_ & 0x00000020) == 0x00000020)) {
route_ = new java.util.ArrayList(route_);
bitField0_ |= 0x00000020;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.rhythm.pb.RequestProtos.RoutePB, com.rhythm.pb.RequestProtos.RoutePB.Builder, com.rhythm.pb.RequestProtos.RoutePBOrBuilder> routeBuilder_;
/**
* repeated .louie.RoutePB route = 20;
*/
public java.util.List getRouteList() {
if (routeBuilder_ == null) {
return java.util.Collections.unmodifiableList(route_);
} else {
return routeBuilder_.getMessageList();
}
}
/**
* repeated .louie.RoutePB route = 20;
*/
public int getRouteCount() {
if (routeBuilder_ == null) {
return route_.size();
} else {
return routeBuilder_.getCount();
}
}
/**
* repeated .louie.RoutePB route = 20;
*/
public com.rhythm.pb.RequestProtos.RoutePB getRoute(int index) {
if (routeBuilder_ == null) {
return route_.get(index);
} else {
return routeBuilder_.getMessage(index);
}
}
/**
* repeated .louie.RoutePB route = 20;
*/
public Builder setRoute(
int index, com.rhythm.pb.RequestProtos.RoutePB value) {
if (routeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRouteIsMutable();
route_.set(index, value);
onChanged();
} else {
routeBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .louie.RoutePB route = 20;
*/
public Builder setRoute(
int index, com.rhythm.pb.RequestProtos.RoutePB.Builder builderForValue) {
if (routeBuilder_ == null) {
ensureRouteIsMutable();
route_.set(index, builderForValue.build());
onChanged();
} else {
routeBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .louie.RoutePB route = 20;
*/
public Builder addRoute(com.rhythm.pb.RequestProtos.RoutePB value) {
if (routeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRouteIsMutable();
route_.add(value);
onChanged();
} else {
routeBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .louie.RoutePB route = 20;
*/
public Builder addRoute(
int index, com.rhythm.pb.RequestProtos.RoutePB value) {
if (routeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRouteIsMutable();
route_.add(index, value);
onChanged();
} else {
routeBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .louie.RoutePB route = 20;
*/
public Builder addRoute(
com.rhythm.pb.RequestProtos.RoutePB.Builder builderForValue) {
if (routeBuilder_ == null) {
ensureRouteIsMutable();
route_.add(builderForValue.build());
onChanged();
} else {
routeBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .louie.RoutePB route = 20;
*/
public Builder addRoute(
int index, com.rhythm.pb.RequestProtos.RoutePB.Builder builderForValue) {
if (routeBuilder_ == null) {
ensureRouteIsMutable();
route_.add(index, builderForValue.build());
onChanged();
} else {
routeBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .louie.RoutePB route = 20;
*/
public Builder addAllRoute(
java.lang.Iterable extends com.rhythm.pb.RequestProtos.RoutePB> values) {
if (routeBuilder_ == null) {
ensureRouteIsMutable();
super.addAll(values, route_);
onChanged();
} else {
routeBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .louie.RoutePB route = 20;
*/
public Builder clearRoute() {
if (routeBuilder_ == null) {
route_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
} else {
routeBuilder_.clear();
}
return this;
}
/**
* repeated .louie.RoutePB route = 20;
*/
public Builder removeRoute(int index) {
if (routeBuilder_ == null) {
ensureRouteIsMutable();
route_.remove(index);
onChanged();
} else {
routeBuilder_.remove(index);
}
return this;
}
/**
* repeated .louie.RoutePB route = 20;
*/
public com.rhythm.pb.RequestProtos.RoutePB.Builder getRouteBuilder(
int index) {
return getRouteFieldBuilder().getBuilder(index);
}
/**
* repeated .louie.RoutePB route = 20;
*/
public com.rhythm.pb.RequestProtos.RoutePBOrBuilder getRouteOrBuilder(
int index) {
if (routeBuilder_ == null) {
return route_.get(index); } else {
return routeBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .louie.RoutePB route = 20;
*/
public java.util.List extends com.rhythm.pb.RequestProtos.RoutePBOrBuilder>
getRouteOrBuilderList() {
if (routeBuilder_ != null) {
return routeBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(route_);
}
}
/**
* repeated .louie.RoutePB route = 20;
*/
public com.rhythm.pb.RequestProtos.RoutePB.Builder addRouteBuilder() {
return getRouteFieldBuilder().addBuilder(
com.rhythm.pb.RequestProtos.RoutePB.getDefaultInstance());
}
/**
* repeated .louie.RoutePB route = 20;
*/
public com.rhythm.pb.RequestProtos.RoutePB.Builder addRouteBuilder(
int index) {
return getRouteFieldBuilder().addBuilder(
index, com.rhythm.pb.RequestProtos.RoutePB.getDefaultInstance());
}
/**
* repeated .louie.RoutePB route = 20;
*/
public java.util.List
getRouteBuilderList() {
return getRouteFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.rhythm.pb.RequestProtos.RoutePB, com.rhythm.pb.RequestProtos.RoutePB.Builder, com.rhythm.pb.RequestProtos.RoutePBOrBuilder>
getRouteFieldBuilder() {
if (routeBuilder_ == null) {
routeBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.rhythm.pb.RequestProtos.RoutePB, com.rhythm.pb.RequestProtos.RoutePB.Builder, com.rhythm.pb.RequestProtos.RoutePBOrBuilder>(
route_,
((bitField0_ & 0x00000020) == 0x00000020),
getParentForChildren(),
isClean());
route_ = null;
}
return routeBuilder_;
}
// optional string routeUser = 21;
private java.lang.Object routeUser_ = "";
/**
* optional string routeUser = 21;
*/
public boolean hasRouteUser() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional string routeUser = 21;
*/
public java.lang.String getRouteUser() {
java.lang.Object ref = routeUser_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
routeUser_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string routeUser = 21;
*/
public com.google.protobuf.ByteString
getRouteUserBytes() {
java.lang.Object ref = routeUser_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
routeUser_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string routeUser = 21;
*/
public Builder setRouteUser(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
routeUser_ = value;
onChanged();
return this;
}
/**
* optional string routeUser = 21;
*/
public Builder clearRouteUser() {
bitField0_ = (bitField0_ & ~0x00000040);
routeUser_ = getDefaultInstance().getRouteUser();
onChanged();
return this;
}
/**
* optional string routeUser = 21;
*/
public Builder setRouteUserBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
routeUser_ = value;
onChanged();
return this;
}
// optional sint64 threadId = 22;
private long threadId_ ;
/**
* optional sint64 threadId = 22;
*/
public boolean hasThreadId() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional sint64 threadId = 22;
*/
public long getThreadId() {
return threadId_;
}
/**
* optional sint64 threadId = 22;
*/
public Builder setThreadId(long value) {
bitField0_ |= 0x00000080;
threadId_ = value;
onChanged();
return this;
}
/**
* optional sint64 threadId = 22;
*/
public Builder clearThreadId() {
bitField0_ = (bitField0_ & ~0x00000080);
threadId_ = 0L;
onChanged();
return this;
}
// optional sint64 start_time = 23;
private long startTime_ ;
/**
* optional sint64 start_time = 23;
*/
public boolean hasStartTime() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional sint64 start_time = 23;
*/
public long getStartTime() {
return startTime_;
}
/**
* optional sint64 start_time = 23;
*/
public Builder setStartTime(long value) {
bitField0_ |= 0x00000100;
startTime_ = value;
onChanged();
return this;
}
/**
* optional sint64 start_time = 23;
*/
public Builder clearStartTime() {
bitField0_ = (bitField0_ & ~0x00000100);
startTime_ = 0L;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:louie.RequestPB)
}
static {
defaultInstance = new RequestPB(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:louie.RequestPB)
}
public interface ResponseHeaderPBOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional uint32 count = 1;
/**
* optional uint32 count = 1;
*/
boolean hasCount();
/**
* optional uint32 count = 1;
*/
int getCount();
// optional .louie.SessionKey key = 2;
/**
* optional .louie.SessionKey key = 2;
*/
boolean hasKey();
/**
* optional .louie.SessionKey key = 2;
*/
com.rhythm.pb.RequestProtos.SessionKey getKey();
/**
* optional .louie.SessionKey key = 2;
*/
com.rhythm.pb.RequestProtos.SessionKeyOrBuilder getKeyOrBuilder();
// repeated .louie.InfoPB info = 3;
/**
* repeated .louie.InfoPB info = 3;
*/
java.util.List
getInfoList();
/**
* repeated .louie.InfoPB info = 3;
*/
com.rhythm.pb.RequestProtos.InfoPB getInfo(int index);
/**
* repeated .louie.InfoPB info = 3;
*/
int getInfoCount();
/**
* repeated .louie.InfoPB info = 3;
*/
java.util.List extends com.rhythm.pb.RequestProtos.InfoPBOrBuilder>
getInfoOrBuilderList();
/**
* repeated .louie.InfoPB info = 3;
*/
com.rhythm.pb.RequestProtos.InfoPBOrBuilder getInfoOrBuilder(
int index);
}
/**
* Protobuf type {@code louie.ResponseHeaderPB}
*/
public static final class ResponseHeaderPB extends
com.google.protobuf.GeneratedMessage
implements ResponseHeaderPBOrBuilder {
// Use ResponseHeaderPB.newBuilder() to construct.
private ResponseHeaderPB(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private ResponseHeaderPB(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final ResponseHeaderPB defaultInstance;
public static ResponseHeaderPB getDefaultInstance() {
return defaultInstance;
}
public ResponseHeaderPB getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ResponseHeaderPB(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
count_ = input.readUInt32();
break;
}
case 18: {
com.rhythm.pb.RequestProtos.SessionKey.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
subBuilder = key_.toBuilder();
}
key_ = input.readMessage(com.rhythm.pb.RequestProtos.SessionKey.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(key_);
key_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
info_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
info_.add(input.readMessage(com.rhythm.pb.RequestProtos.InfoPB.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
info_ = java.util.Collections.unmodifiableList(info_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.rhythm.pb.RequestProtos.internal_static_louie_ResponseHeaderPB_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.rhythm.pb.RequestProtos.internal_static_louie_ResponseHeaderPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.rhythm.pb.RequestProtos.ResponseHeaderPB.class, com.rhythm.pb.RequestProtos.ResponseHeaderPB.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public ResponseHeaderPB parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ResponseHeaderPB(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional uint32 count = 1;
public static final int COUNT_FIELD_NUMBER = 1;
private int count_;
/**
* optional uint32 count = 1;
*/
public boolean hasCount() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional uint32 count = 1;
*/
public int getCount() {
return count_;
}
// optional .louie.SessionKey key = 2;
public static final int KEY_FIELD_NUMBER = 2;
private com.rhythm.pb.RequestProtos.SessionKey key_;
/**
* optional .louie.SessionKey key = 2;
*/
public boolean hasKey() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .louie.SessionKey key = 2;
*/
public com.rhythm.pb.RequestProtos.SessionKey getKey() {
return key_;
}
/**
* optional .louie.SessionKey key = 2;
*/
public com.rhythm.pb.RequestProtos.SessionKeyOrBuilder getKeyOrBuilder() {
return key_;
}
// repeated .louie.InfoPB info = 3;
public static final int INFO_FIELD_NUMBER = 3;
private java.util.List info_;
/**
* repeated .louie.InfoPB info = 3;
*/
public java.util.List getInfoList() {
return info_;
}
/**
* repeated .louie.InfoPB info = 3;
*/
public java.util.List extends com.rhythm.pb.RequestProtos.InfoPBOrBuilder>
getInfoOrBuilderList() {
return info_;
}
/**
* repeated .louie.InfoPB info = 3;
*/
public int getInfoCount() {
return info_.size();
}
/**
* repeated .louie.InfoPB info = 3;
*/
public com.rhythm.pb.RequestProtos.InfoPB getInfo(int index) {
return info_.get(index);
}
/**
* repeated .louie.InfoPB info = 3;
*/
public com.rhythm.pb.RequestProtos.InfoPBOrBuilder getInfoOrBuilder(
int index) {
return info_.get(index);
}
private void initFields() {
count_ = 0;
key_ = com.rhythm.pb.RequestProtos.SessionKey.getDefaultInstance();
info_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeUInt32(1, count_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeMessage(2, key_);
}
for (int i = 0; i < info_.size(); i++) {
output.writeMessage(3, info_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, count_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, key_);
}
for (int i = 0; i < info_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, info_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.rhythm.pb.RequestProtos.ResponseHeaderPB)) {
return super.equals(obj);
}
com.rhythm.pb.RequestProtos.ResponseHeaderPB other = (com.rhythm.pb.RequestProtos.ResponseHeaderPB) obj;
boolean result = true;
result = result && (hasCount() == other.hasCount());
if (hasCount()) {
result = result && (getCount()
== other.getCount());
}
result = result && (hasKey() == other.hasKey());
if (hasKey()) {
result = result && getKey()
.equals(other.getKey());
}
result = result && getInfoList()
.equals(other.getInfoList());
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasCount()) {
hash = (37 * hash) + COUNT_FIELD_NUMBER;
hash = (53 * hash) + getCount();
}
if (hasKey()) {
hash = (37 * hash) + KEY_FIELD_NUMBER;
hash = (53 * hash) + getKey().hashCode();
}
if (getInfoCount() > 0) {
hash = (37 * hash) + INFO_FIELD_NUMBER;
hash = (53 * hash) + getInfoList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.rhythm.pb.RequestProtos.ResponseHeaderPB parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.rhythm.pb.RequestProtos.ResponseHeaderPB parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.ResponseHeaderPB parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.rhythm.pb.RequestProtos.ResponseHeaderPB parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.ResponseHeaderPB parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.rhythm.pb.RequestProtos.ResponseHeaderPB parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.ResponseHeaderPB parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.rhythm.pb.RequestProtos.ResponseHeaderPB parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.ResponseHeaderPB parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.rhythm.pb.RequestProtos.ResponseHeaderPB parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.rhythm.pb.RequestProtos.ResponseHeaderPB prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code louie.ResponseHeaderPB}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.rhythm.pb.RequestProtos.ResponseHeaderPBOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.rhythm.pb.RequestProtos.internal_static_louie_ResponseHeaderPB_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.rhythm.pb.RequestProtos.internal_static_louie_ResponseHeaderPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.rhythm.pb.RequestProtos.ResponseHeaderPB.class, com.rhythm.pb.RequestProtos.ResponseHeaderPB.Builder.class);
}
// Construct using com.rhythm.pb.RequestProtos.ResponseHeaderPB.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getKeyFieldBuilder();
getInfoFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
count_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
if (keyBuilder_ == null) {
key_ = com.rhythm.pb.RequestProtos.SessionKey.getDefaultInstance();
} else {
keyBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
if (infoBuilder_ == null) {
info_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
infoBuilder_.clear();
}
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.rhythm.pb.RequestProtos.internal_static_louie_ResponseHeaderPB_descriptor;
}
public com.rhythm.pb.RequestProtos.ResponseHeaderPB getDefaultInstanceForType() {
return com.rhythm.pb.RequestProtos.ResponseHeaderPB.getDefaultInstance();
}
public com.rhythm.pb.RequestProtos.ResponseHeaderPB build() {
com.rhythm.pb.RequestProtos.ResponseHeaderPB result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.rhythm.pb.RequestProtos.ResponseHeaderPB buildPartial() {
com.rhythm.pb.RequestProtos.ResponseHeaderPB result = new com.rhythm.pb.RequestProtos.ResponseHeaderPB(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.count_ = count_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
if (keyBuilder_ == null) {
result.key_ = key_;
} else {
result.key_ = keyBuilder_.build();
}
if (infoBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004)) {
info_ = java.util.Collections.unmodifiableList(info_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.info_ = info_;
} else {
result.info_ = infoBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.rhythm.pb.RequestProtos.ResponseHeaderPB) {
return mergeFrom((com.rhythm.pb.RequestProtos.ResponseHeaderPB)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.rhythm.pb.RequestProtos.ResponseHeaderPB other) {
if (other == com.rhythm.pb.RequestProtos.ResponseHeaderPB.getDefaultInstance()) return this;
if (other.hasCount()) {
setCount(other.getCount());
}
if (other.hasKey()) {
mergeKey(other.getKey());
}
if (infoBuilder_ == null) {
if (!other.info_.isEmpty()) {
if (info_.isEmpty()) {
info_ = other.info_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureInfoIsMutable();
info_.addAll(other.info_);
}
onChanged();
}
} else {
if (!other.info_.isEmpty()) {
if (infoBuilder_.isEmpty()) {
infoBuilder_.dispose();
infoBuilder_ = null;
info_ = other.info_;
bitField0_ = (bitField0_ & ~0x00000004);
infoBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getInfoFieldBuilder() : null;
} else {
infoBuilder_.addAllMessages(other.info_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.rhythm.pb.RequestProtos.ResponseHeaderPB parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.rhythm.pb.RequestProtos.ResponseHeaderPB) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional uint32 count = 1;
private int count_ ;
/**
* optional uint32 count = 1;
*/
public boolean hasCount() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional uint32 count = 1;
*/
public int getCount() {
return count_;
}
/**
* optional uint32 count = 1;
*/
public Builder setCount(int value) {
bitField0_ |= 0x00000001;
count_ = value;
onChanged();
return this;
}
/**
* optional uint32 count = 1;
*/
public Builder clearCount() {
bitField0_ = (bitField0_ & ~0x00000001);
count_ = 0;
onChanged();
return this;
}
// optional .louie.SessionKey key = 2;
private com.rhythm.pb.RequestProtos.SessionKey key_ = com.rhythm.pb.RequestProtos.SessionKey.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.rhythm.pb.RequestProtos.SessionKey, com.rhythm.pb.RequestProtos.SessionKey.Builder, com.rhythm.pb.RequestProtos.SessionKeyOrBuilder> keyBuilder_;
/**
* optional .louie.SessionKey key = 2;
*/
public boolean hasKey() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .louie.SessionKey key = 2;
*/
public com.rhythm.pb.RequestProtos.SessionKey getKey() {
if (keyBuilder_ == null) {
return key_;
} else {
return keyBuilder_.getMessage();
}
}
/**
* optional .louie.SessionKey key = 2;
*/
public Builder setKey(com.rhythm.pb.RequestProtos.SessionKey value) {
if (keyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
key_ = value;
onChanged();
} else {
keyBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .louie.SessionKey key = 2;
*/
public Builder setKey(
com.rhythm.pb.RequestProtos.SessionKey.Builder builderForValue) {
if (keyBuilder_ == null) {
key_ = builderForValue.build();
onChanged();
} else {
keyBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .louie.SessionKey key = 2;
*/
public Builder mergeKey(com.rhythm.pb.RequestProtos.SessionKey value) {
if (keyBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002) &&
key_ != com.rhythm.pb.RequestProtos.SessionKey.getDefaultInstance()) {
key_ =
com.rhythm.pb.RequestProtos.SessionKey.newBuilder(key_).mergeFrom(value).buildPartial();
} else {
key_ = value;
}
onChanged();
} else {
keyBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
* optional .louie.SessionKey key = 2;
*/
public Builder clearKey() {
if (keyBuilder_ == null) {
key_ = com.rhythm.pb.RequestProtos.SessionKey.getDefaultInstance();
onChanged();
} else {
keyBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
* optional .louie.SessionKey key = 2;
*/
public com.rhythm.pb.RequestProtos.SessionKey.Builder getKeyBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getKeyFieldBuilder().getBuilder();
}
/**
* optional .louie.SessionKey key = 2;
*/
public com.rhythm.pb.RequestProtos.SessionKeyOrBuilder getKeyOrBuilder() {
if (keyBuilder_ != null) {
return keyBuilder_.getMessageOrBuilder();
} else {
return key_;
}
}
/**
* optional .louie.SessionKey key = 2;
*/
private com.google.protobuf.SingleFieldBuilder<
com.rhythm.pb.RequestProtos.SessionKey, com.rhythm.pb.RequestProtos.SessionKey.Builder, com.rhythm.pb.RequestProtos.SessionKeyOrBuilder>
getKeyFieldBuilder() {
if (keyBuilder_ == null) {
keyBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.rhythm.pb.RequestProtos.SessionKey, com.rhythm.pb.RequestProtos.SessionKey.Builder, com.rhythm.pb.RequestProtos.SessionKeyOrBuilder>(
key_,
getParentForChildren(),
isClean());
key_ = null;
}
return keyBuilder_;
}
// repeated .louie.InfoPB info = 3;
private java.util.List info_ =
java.util.Collections.emptyList();
private void ensureInfoIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
info_ = new java.util.ArrayList(info_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.rhythm.pb.RequestProtos.InfoPB, com.rhythm.pb.RequestProtos.InfoPB.Builder, com.rhythm.pb.RequestProtos.InfoPBOrBuilder> infoBuilder_;
/**
* repeated .louie.InfoPB info = 3;
*/
public java.util.List getInfoList() {
if (infoBuilder_ == null) {
return java.util.Collections.unmodifiableList(info_);
} else {
return infoBuilder_.getMessageList();
}
}
/**
* repeated .louie.InfoPB info = 3;
*/
public int getInfoCount() {
if (infoBuilder_ == null) {
return info_.size();
} else {
return infoBuilder_.getCount();
}
}
/**
* repeated .louie.InfoPB info = 3;
*/
public com.rhythm.pb.RequestProtos.InfoPB getInfo(int index) {
if (infoBuilder_ == null) {
return info_.get(index);
} else {
return infoBuilder_.getMessage(index);
}
}
/**
* repeated .louie.InfoPB info = 3;
*/
public Builder setInfo(
int index, com.rhythm.pb.RequestProtos.InfoPB value) {
if (infoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureInfoIsMutable();
info_.set(index, value);
onChanged();
} else {
infoBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .louie.InfoPB info = 3;
*/
public Builder setInfo(
int index, com.rhythm.pb.RequestProtos.InfoPB.Builder builderForValue) {
if (infoBuilder_ == null) {
ensureInfoIsMutable();
info_.set(index, builderForValue.build());
onChanged();
} else {
infoBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .louie.InfoPB info = 3;
*/
public Builder addInfo(com.rhythm.pb.RequestProtos.InfoPB value) {
if (infoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureInfoIsMutable();
info_.add(value);
onChanged();
} else {
infoBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .louie.InfoPB info = 3;
*/
public Builder addInfo(
int index, com.rhythm.pb.RequestProtos.InfoPB value) {
if (infoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureInfoIsMutable();
info_.add(index, value);
onChanged();
} else {
infoBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .louie.InfoPB info = 3;
*/
public Builder addInfo(
com.rhythm.pb.RequestProtos.InfoPB.Builder builderForValue) {
if (infoBuilder_ == null) {
ensureInfoIsMutable();
info_.add(builderForValue.build());
onChanged();
} else {
infoBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .louie.InfoPB info = 3;
*/
public Builder addInfo(
int index, com.rhythm.pb.RequestProtos.InfoPB.Builder builderForValue) {
if (infoBuilder_ == null) {
ensureInfoIsMutable();
info_.add(index, builderForValue.build());
onChanged();
} else {
infoBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .louie.InfoPB info = 3;
*/
public Builder addAllInfo(
java.lang.Iterable extends com.rhythm.pb.RequestProtos.InfoPB> values) {
if (infoBuilder_ == null) {
ensureInfoIsMutable();
super.addAll(values, info_);
onChanged();
} else {
infoBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .louie.InfoPB info = 3;
*/
public Builder clearInfo() {
if (infoBuilder_ == null) {
info_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
infoBuilder_.clear();
}
return this;
}
/**
* repeated .louie.InfoPB info = 3;
*/
public Builder removeInfo(int index) {
if (infoBuilder_ == null) {
ensureInfoIsMutable();
info_.remove(index);
onChanged();
} else {
infoBuilder_.remove(index);
}
return this;
}
/**
* repeated .louie.InfoPB info = 3;
*/
public com.rhythm.pb.RequestProtos.InfoPB.Builder getInfoBuilder(
int index) {
return getInfoFieldBuilder().getBuilder(index);
}
/**
* repeated .louie.InfoPB info = 3;
*/
public com.rhythm.pb.RequestProtos.InfoPBOrBuilder getInfoOrBuilder(
int index) {
if (infoBuilder_ == null) {
return info_.get(index); } else {
return infoBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .louie.InfoPB info = 3;
*/
public java.util.List extends com.rhythm.pb.RequestProtos.InfoPBOrBuilder>
getInfoOrBuilderList() {
if (infoBuilder_ != null) {
return infoBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(info_);
}
}
/**
* repeated .louie.InfoPB info = 3;
*/
public com.rhythm.pb.RequestProtos.InfoPB.Builder addInfoBuilder() {
return getInfoFieldBuilder().addBuilder(
com.rhythm.pb.RequestProtos.InfoPB.getDefaultInstance());
}
/**
* repeated .louie.InfoPB info = 3;
*/
public com.rhythm.pb.RequestProtos.InfoPB.Builder addInfoBuilder(
int index) {
return getInfoFieldBuilder().addBuilder(
index, com.rhythm.pb.RequestProtos.InfoPB.getDefaultInstance());
}
/**
* repeated .louie.InfoPB info = 3;
*/
public java.util.List
getInfoBuilderList() {
return getInfoFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.rhythm.pb.RequestProtos.InfoPB, com.rhythm.pb.RequestProtos.InfoPB.Builder, com.rhythm.pb.RequestProtos.InfoPBOrBuilder>
getInfoFieldBuilder() {
if (infoBuilder_ == null) {
infoBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.rhythm.pb.RequestProtos.InfoPB, com.rhythm.pb.RequestProtos.InfoPB.Builder, com.rhythm.pb.RequestProtos.InfoPBOrBuilder>(
info_,
((bitField0_ & 0x00000004) == 0x00000004),
getParentForChildren(),
isClean());
info_ = null;
}
return infoBuilder_;
}
// @@protoc_insertion_point(builder_scope:louie.ResponseHeaderPB)
}
static {
defaultInstance = new ResponseHeaderPB(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:louie.ResponseHeaderPB)
}
public interface ResponsePBOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional uint32 id = 1;
/**
* optional uint32 id = 1;
*/
boolean hasId();
/**
* optional uint32 id = 1;
*/
int getId();
// optional uint32 count = 2;
/**
* optional uint32 count = 2;
*/
boolean hasCount();
/**
* optional uint32 count = 2;
*/
int getCount();
// optional string type = 3;
/**
* optional string type = 3;
*/
boolean hasType();
/**
* optional string type = 3;
*/
java.lang.String getType();
/**
* optional string type = 3;
*/
com.google.protobuf.ByteString
getTypeBytes();
// optional .louie.ErrorPB error = 4;
/**
* optional .louie.ErrorPB error = 4;
*/
boolean hasError();
/**
* optional .louie.ErrorPB error = 4;
*/
com.rhythm.pb.RequestProtos.ErrorPB getError();
/**
* optional .louie.ErrorPB error = 4;
*/
com.rhythm.pb.RequestProtos.ErrorPBOrBuilder getErrorOrBuilder();
// repeated .louie.RoutePathPB route = 20;
/**
* repeated .louie.RoutePathPB route = 20;
*/
java.util.List
getRouteList();
/**
* repeated .louie.RoutePathPB route = 20;
*/
com.rhythm.pb.RequestProtos.RoutePathPB getRoute(int index);
/**
* repeated .louie.RoutePathPB route = 20;
*/
int getRouteCount();
/**
* repeated .louie.RoutePathPB route = 20;
*/
java.util.List extends com.rhythm.pb.RequestProtos.RoutePathPBOrBuilder>
getRouteOrBuilderList();
/**
* repeated .louie.RoutePathPB route = 20;
*/
com.rhythm.pb.RequestProtos.RoutePathPBOrBuilder getRouteOrBuilder(
int index);
}
/**
* Protobuf type {@code louie.ResponsePB}
*/
public static final class ResponsePB extends
com.google.protobuf.GeneratedMessage
implements ResponsePBOrBuilder {
// Use ResponsePB.newBuilder() to construct.
private ResponsePB(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private ResponsePB(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final ResponsePB defaultInstance;
public static ResponsePB getDefaultInstance() {
return defaultInstance;
}
public ResponsePB getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ResponsePB(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
id_ = input.readUInt32();
break;
}
case 16: {
bitField0_ |= 0x00000002;
count_ = input.readUInt32();
break;
}
case 26: {
bitField0_ |= 0x00000004;
type_ = input.readBytes();
break;
}
case 34: {
com.rhythm.pb.RequestProtos.ErrorPB.Builder subBuilder = null;
if (((bitField0_ & 0x00000008) == 0x00000008)) {
subBuilder = error_.toBuilder();
}
error_ = input.readMessage(com.rhythm.pb.RequestProtos.ErrorPB.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(error_);
error_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000008;
break;
}
case 162: {
if (!((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
route_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000010;
}
route_.add(input.readMessage(com.rhythm.pb.RequestProtos.RoutePathPB.PARSER, extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
route_ = java.util.Collections.unmodifiableList(route_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.rhythm.pb.RequestProtos.internal_static_louie_ResponsePB_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.rhythm.pb.RequestProtos.internal_static_louie_ResponsePB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.rhythm.pb.RequestProtos.ResponsePB.class, com.rhythm.pb.RequestProtos.ResponsePB.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public ResponsePB parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ResponsePB(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional uint32 id = 1;
public static final int ID_FIELD_NUMBER = 1;
private int id_;
/**
* optional uint32 id = 1;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional uint32 id = 1;
*/
public int getId() {
return id_;
}
// optional uint32 count = 2;
public static final int COUNT_FIELD_NUMBER = 2;
private int count_;
/**
* optional uint32 count = 2;
*/
public boolean hasCount() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional uint32 count = 2;
*/
public int getCount() {
return count_;
}
// optional string type = 3;
public static final int TYPE_FIELD_NUMBER = 3;
private java.lang.Object type_;
/**
* optional string type = 3;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string type = 3;
*/
public java.lang.String getType() {
java.lang.Object ref = type_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
type_ = s;
}
return s;
}
}
/**
* optional string type = 3;
*/
public com.google.protobuf.ByteString
getTypeBytes() {
java.lang.Object ref = type_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
type_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional .louie.ErrorPB error = 4;
public static final int ERROR_FIELD_NUMBER = 4;
private com.rhythm.pb.RequestProtos.ErrorPB error_;
/**
* optional .louie.ErrorPB error = 4;
*/
public boolean hasError() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .louie.ErrorPB error = 4;
*/
public com.rhythm.pb.RequestProtos.ErrorPB getError() {
return error_;
}
/**
* optional .louie.ErrorPB error = 4;
*/
public com.rhythm.pb.RequestProtos.ErrorPBOrBuilder getErrorOrBuilder() {
return error_;
}
// repeated .louie.RoutePathPB route = 20;
public static final int ROUTE_FIELD_NUMBER = 20;
private java.util.List route_;
/**
* repeated .louie.RoutePathPB route = 20;
*/
public java.util.List getRouteList() {
return route_;
}
/**
* repeated .louie.RoutePathPB route = 20;
*/
public java.util.List extends com.rhythm.pb.RequestProtos.RoutePathPBOrBuilder>
getRouteOrBuilderList() {
return route_;
}
/**
* repeated .louie.RoutePathPB route = 20;
*/
public int getRouteCount() {
return route_.size();
}
/**
* repeated .louie.RoutePathPB route = 20;
*/
public com.rhythm.pb.RequestProtos.RoutePathPB getRoute(int index) {
return route_.get(index);
}
/**
* repeated .louie.RoutePathPB route = 20;
*/
public com.rhythm.pb.RequestProtos.RoutePathPBOrBuilder getRouteOrBuilder(
int index) {
return route_.get(index);
}
private void initFields() {
id_ = 0;
count_ = 0;
type_ = "";
error_ = com.rhythm.pb.RequestProtos.ErrorPB.getDefaultInstance();
route_ = java.util.Collections.emptyList();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeUInt32(1, id_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeUInt32(2, count_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, getTypeBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeMessage(4, error_);
}
for (int i = 0; i < route_.size(); i++) {
output.writeMessage(20, route_.get(i));
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, id_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(2, count_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, getTypeBytes());
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, error_);
}
for (int i = 0; i < route_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(20, route_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.rhythm.pb.RequestProtos.ResponsePB)) {
return super.equals(obj);
}
com.rhythm.pb.RequestProtos.ResponsePB other = (com.rhythm.pb.RequestProtos.ResponsePB) obj;
boolean result = true;
result = result && (hasId() == other.hasId());
if (hasId()) {
result = result && (getId()
== other.getId());
}
result = result && (hasCount() == other.hasCount());
if (hasCount()) {
result = result && (getCount()
== other.getCount());
}
result = result && (hasType() == other.hasType());
if (hasType()) {
result = result && getType()
.equals(other.getType());
}
result = result && (hasError() == other.hasError());
if (hasError()) {
result = result && getError()
.equals(other.getError());
}
result = result && getRouteList()
.equals(other.getRouteList());
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId();
}
if (hasCount()) {
hash = (37 * hash) + COUNT_FIELD_NUMBER;
hash = (53 * hash) + getCount();
}
if (hasType()) {
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + getType().hashCode();
}
if (hasError()) {
hash = (37 * hash) + ERROR_FIELD_NUMBER;
hash = (53 * hash) + getError().hashCode();
}
if (getRouteCount() > 0) {
hash = (37 * hash) + ROUTE_FIELD_NUMBER;
hash = (53 * hash) + getRouteList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.rhythm.pb.RequestProtos.ResponsePB parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.rhythm.pb.RequestProtos.ResponsePB parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.ResponsePB parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.rhythm.pb.RequestProtos.ResponsePB parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.ResponsePB parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.rhythm.pb.RequestProtos.ResponsePB parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.ResponsePB parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.rhythm.pb.RequestProtos.ResponsePB parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.ResponsePB parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.rhythm.pb.RequestProtos.ResponsePB parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.rhythm.pb.RequestProtos.ResponsePB prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code louie.ResponsePB}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.rhythm.pb.RequestProtos.ResponsePBOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.rhythm.pb.RequestProtos.internal_static_louie_ResponsePB_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.rhythm.pb.RequestProtos.internal_static_louie_ResponsePB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.rhythm.pb.RequestProtos.ResponsePB.class, com.rhythm.pb.RequestProtos.ResponsePB.Builder.class);
}
// Construct using com.rhythm.pb.RequestProtos.ResponsePB.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getErrorFieldBuilder();
getRouteFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
id_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
count_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
type_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
if (errorBuilder_ == null) {
error_ = com.rhythm.pb.RequestProtos.ErrorPB.getDefaultInstance();
} else {
errorBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
if (routeBuilder_ == null) {
route_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
} else {
routeBuilder_.clear();
}
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.rhythm.pb.RequestProtos.internal_static_louie_ResponsePB_descriptor;
}
public com.rhythm.pb.RequestProtos.ResponsePB getDefaultInstanceForType() {
return com.rhythm.pb.RequestProtos.ResponsePB.getDefaultInstance();
}
public com.rhythm.pb.RequestProtos.ResponsePB build() {
com.rhythm.pb.RequestProtos.ResponsePB result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.rhythm.pb.RequestProtos.ResponsePB buildPartial() {
com.rhythm.pb.RequestProtos.ResponsePB result = new com.rhythm.pb.RequestProtos.ResponsePB(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.id_ = id_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.count_ = count_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.type_ = type_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
if (errorBuilder_ == null) {
result.error_ = error_;
} else {
result.error_ = errorBuilder_.build();
}
if (routeBuilder_ == null) {
if (((bitField0_ & 0x00000010) == 0x00000010)) {
route_ = java.util.Collections.unmodifiableList(route_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.route_ = route_;
} else {
result.route_ = routeBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.rhythm.pb.RequestProtos.ResponsePB) {
return mergeFrom((com.rhythm.pb.RequestProtos.ResponsePB)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.rhythm.pb.RequestProtos.ResponsePB other) {
if (other == com.rhythm.pb.RequestProtos.ResponsePB.getDefaultInstance()) return this;
if (other.hasId()) {
setId(other.getId());
}
if (other.hasCount()) {
setCount(other.getCount());
}
if (other.hasType()) {
bitField0_ |= 0x00000004;
type_ = other.type_;
onChanged();
}
if (other.hasError()) {
mergeError(other.getError());
}
if (routeBuilder_ == null) {
if (!other.route_.isEmpty()) {
if (route_.isEmpty()) {
route_ = other.route_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureRouteIsMutable();
route_.addAll(other.route_);
}
onChanged();
}
} else {
if (!other.route_.isEmpty()) {
if (routeBuilder_.isEmpty()) {
routeBuilder_.dispose();
routeBuilder_ = null;
route_ = other.route_;
bitField0_ = (bitField0_ & ~0x00000010);
routeBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getRouteFieldBuilder() : null;
} else {
routeBuilder_.addAllMessages(other.route_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.rhythm.pb.RequestProtos.ResponsePB parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.rhythm.pb.RequestProtos.ResponsePB) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional uint32 id = 1;
private int id_ ;
/**
* optional uint32 id = 1;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional uint32 id = 1;
*/
public int getId() {
return id_;
}
/**
* optional uint32 id = 1;
*/
public Builder setId(int value) {
bitField0_ |= 0x00000001;
id_ = value;
onChanged();
return this;
}
/**
* optional uint32 id = 1;
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000001);
id_ = 0;
onChanged();
return this;
}
// optional uint32 count = 2;
private int count_ ;
/**
* optional uint32 count = 2;
*/
public boolean hasCount() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional uint32 count = 2;
*/
public int getCount() {
return count_;
}
/**
* optional uint32 count = 2;
*/
public Builder setCount(int value) {
bitField0_ |= 0x00000002;
count_ = value;
onChanged();
return this;
}
/**
* optional uint32 count = 2;
*/
public Builder clearCount() {
bitField0_ = (bitField0_ & ~0x00000002);
count_ = 0;
onChanged();
return this;
}
// optional string type = 3;
private java.lang.Object type_ = "";
/**
* optional string type = 3;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string type = 3;
*/
public java.lang.String getType() {
java.lang.Object ref = type_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
type_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string type = 3;
*/
public com.google.protobuf.ByteString
getTypeBytes() {
java.lang.Object ref = type_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
type_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string type = 3;
*/
public Builder setType(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
type_ = value;
onChanged();
return this;
}
/**
* optional string type = 3;
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000004);
type_ = getDefaultInstance().getType();
onChanged();
return this;
}
/**
* optional string type = 3;
*/
public Builder setTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
type_ = value;
onChanged();
return this;
}
// optional .louie.ErrorPB error = 4;
private com.rhythm.pb.RequestProtos.ErrorPB error_ = com.rhythm.pb.RequestProtos.ErrorPB.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
com.rhythm.pb.RequestProtos.ErrorPB, com.rhythm.pb.RequestProtos.ErrorPB.Builder, com.rhythm.pb.RequestProtos.ErrorPBOrBuilder> errorBuilder_;
/**
* optional .louie.ErrorPB error = 4;
*/
public boolean hasError() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional .louie.ErrorPB error = 4;
*/
public com.rhythm.pb.RequestProtos.ErrorPB getError() {
if (errorBuilder_ == null) {
return error_;
} else {
return errorBuilder_.getMessage();
}
}
/**
* optional .louie.ErrorPB error = 4;
*/
public Builder setError(com.rhythm.pb.RequestProtos.ErrorPB value) {
if (errorBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
error_ = value;
onChanged();
} else {
errorBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .louie.ErrorPB error = 4;
*/
public Builder setError(
com.rhythm.pb.RequestProtos.ErrorPB.Builder builderForValue) {
if (errorBuilder_ == null) {
error_ = builderForValue.build();
onChanged();
} else {
errorBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .louie.ErrorPB error = 4;
*/
public Builder mergeError(com.rhythm.pb.RequestProtos.ErrorPB value) {
if (errorBuilder_ == null) {
if (((bitField0_ & 0x00000008) == 0x00000008) &&
error_ != com.rhythm.pb.RequestProtos.ErrorPB.getDefaultInstance()) {
error_ =
com.rhythm.pb.RequestProtos.ErrorPB.newBuilder(error_).mergeFrom(value).buildPartial();
} else {
error_ = value;
}
onChanged();
} else {
errorBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000008;
return this;
}
/**
* optional .louie.ErrorPB error = 4;
*/
public Builder clearError() {
if (errorBuilder_ == null) {
error_ = com.rhythm.pb.RequestProtos.ErrorPB.getDefaultInstance();
onChanged();
} else {
errorBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
return this;
}
/**
* optional .louie.ErrorPB error = 4;
*/
public com.rhythm.pb.RequestProtos.ErrorPB.Builder getErrorBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getErrorFieldBuilder().getBuilder();
}
/**
* optional .louie.ErrorPB error = 4;
*/
public com.rhythm.pb.RequestProtos.ErrorPBOrBuilder getErrorOrBuilder() {
if (errorBuilder_ != null) {
return errorBuilder_.getMessageOrBuilder();
} else {
return error_;
}
}
/**
* optional .louie.ErrorPB error = 4;
*/
private com.google.protobuf.SingleFieldBuilder<
com.rhythm.pb.RequestProtos.ErrorPB, com.rhythm.pb.RequestProtos.ErrorPB.Builder, com.rhythm.pb.RequestProtos.ErrorPBOrBuilder>
getErrorFieldBuilder() {
if (errorBuilder_ == null) {
errorBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.rhythm.pb.RequestProtos.ErrorPB, com.rhythm.pb.RequestProtos.ErrorPB.Builder, com.rhythm.pb.RequestProtos.ErrorPBOrBuilder>(
error_,
getParentForChildren(),
isClean());
error_ = null;
}
return errorBuilder_;
}
// repeated .louie.RoutePathPB route = 20;
private java.util.List route_ =
java.util.Collections.emptyList();
private void ensureRouteIsMutable() {
if (!((bitField0_ & 0x00000010) == 0x00000010)) {
route_ = new java.util.ArrayList(route_);
bitField0_ |= 0x00000010;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.rhythm.pb.RequestProtos.RoutePathPB, com.rhythm.pb.RequestProtos.RoutePathPB.Builder, com.rhythm.pb.RequestProtos.RoutePathPBOrBuilder> routeBuilder_;
/**
* repeated .louie.RoutePathPB route = 20;
*/
public java.util.List getRouteList() {
if (routeBuilder_ == null) {
return java.util.Collections.unmodifiableList(route_);
} else {
return routeBuilder_.getMessageList();
}
}
/**
* repeated .louie.RoutePathPB route = 20;
*/
public int getRouteCount() {
if (routeBuilder_ == null) {
return route_.size();
} else {
return routeBuilder_.getCount();
}
}
/**
* repeated .louie.RoutePathPB route = 20;
*/
public com.rhythm.pb.RequestProtos.RoutePathPB getRoute(int index) {
if (routeBuilder_ == null) {
return route_.get(index);
} else {
return routeBuilder_.getMessage(index);
}
}
/**
* repeated .louie.RoutePathPB route = 20;
*/
public Builder setRoute(
int index, com.rhythm.pb.RequestProtos.RoutePathPB value) {
if (routeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRouteIsMutable();
route_.set(index, value);
onChanged();
} else {
routeBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .louie.RoutePathPB route = 20;
*/
public Builder setRoute(
int index, com.rhythm.pb.RequestProtos.RoutePathPB.Builder builderForValue) {
if (routeBuilder_ == null) {
ensureRouteIsMutable();
route_.set(index, builderForValue.build());
onChanged();
} else {
routeBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .louie.RoutePathPB route = 20;
*/
public Builder addRoute(com.rhythm.pb.RequestProtos.RoutePathPB value) {
if (routeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRouteIsMutable();
route_.add(value);
onChanged();
} else {
routeBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .louie.RoutePathPB route = 20;
*/
public Builder addRoute(
int index, com.rhythm.pb.RequestProtos.RoutePathPB value) {
if (routeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRouteIsMutable();
route_.add(index, value);
onChanged();
} else {
routeBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .louie.RoutePathPB route = 20;
*/
public Builder addRoute(
com.rhythm.pb.RequestProtos.RoutePathPB.Builder builderForValue) {
if (routeBuilder_ == null) {
ensureRouteIsMutable();
route_.add(builderForValue.build());
onChanged();
} else {
routeBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .louie.RoutePathPB route = 20;
*/
public Builder addRoute(
int index, com.rhythm.pb.RequestProtos.RoutePathPB.Builder builderForValue) {
if (routeBuilder_ == null) {
ensureRouteIsMutable();
route_.add(index, builderForValue.build());
onChanged();
} else {
routeBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .louie.RoutePathPB route = 20;
*/
public Builder addAllRoute(
java.lang.Iterable extends com.rhythm.pb.RequestProtos.RoutePathPB> values) {
if (routeBuilder_ == null) {
ensureRouteIsMutable();
super.addAll(values, route_);
onChanged();
} else {
routeBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .louie.RoutePathPB route = 20;
*/
public Builder clearRoute() {
if (routeBuilder_ == null) {
route_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
} else {
routeBuilder_.clear();
}
return this;
}
/**
* repeated .louie.RoutePathPB route = 20;
*/
public Builder removeRoute(int index) {
if (routeBuilder_ == null) {
ensureRouteIsMutable();
route_.remove(index);
onChanged();
} else {
routeBuilder_.remove(index);
}
return this;
}
/**
* repeated .louie.RoutePathPB route = 20;
*/
public com.rhythm.pb.RequestProtos.RoutePathPB.Builder getRouteBuilder(
int index) {
return getRouteFieldBuilder().getBuilder(index);
}
/**
* repeated .louie.RoutePathPB route = 20;
*/
public com.rhythm.pb.RequestProtos.RoutePathPBOrBuilder getRouteOrBuilder(
int index) {
if (routeBuilder_ == null) {
return route_.get(index); } else {
return routeBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .louie.RoutePathPB route = 20;
*/
public java.util.List extends com.rhythm.pb.RequestProtos.RoutePathPBOrBuilder>
getRouteOrBuilderList() {
if (routeBuilder_ != null) {
return routeBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(route_);
}
}
/**
* repeated .louie.RoutePathPB route = 20;
*/
public com.rhythm.pb.RequestProtos.RoutePathPB.Builder addRouteBuilder() {
return getRouteFieldBuilder().addBuilder(
com.rhythm.pb.RequestProtos.RoutePathPB.getDefaultInstance());
}
/**
* repeated .louie.RoutePathPB route = 20;
*/
public com.rhythm.pb.RequestProtos.RoutePathPB.Builder addRouteBuilder(
int index) {
return getRouteFieldBuilder().addBuilder(
index, com.rhythm.pb.RequestProtos.RoutePathPB.getDefaultInstance());
}
/**
* repeated .louie.RoutePathPB route = 20;
*/
public java.util.List
getRouteBuilderList() {
return getRouteFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.rhythm.pb.RequestProtos.RoutePathPB, com.rhythm.pb.RequestProtos.RoutePathPB.Builder, com.rhythm.pb.RequestProtos.RoutePathPBOrBuilder>
getRouteFieldBuilder() {
if (routeBuilder_ == null) {
routeBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.rhythm.pb.RequestProtos.RoutePathPB, com.rhythm.pb.RequestProtos.RoutePathPB.Builder, com.rhythm.pb.RequestProtos.RoutePathPBOrBuilder>(
route_,
((bitField0_ & 0x00000010) == 0x00000010),
getParentForChildren(),
isClean());
route_ = null;
}
return routeBuilder_;
}
// @@protoc_insertion_point(builder_scope:louie.ResponsePB)
}
static {
defaultInstance = new ResponsePB(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:louie.ResponsePB)
}
public interface ErrorPBOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional uint32 code = 1;
/**
* optional uint32 code = 1;
*/
boolean hasCode();
/**
* optional uint32 code = 1;
*/
int getCode();
// optional string type = 2;
/**
* optional string type = 2;
*/
boolean hasType();
/**
* optional string type = 2;
*/
java.lang.String getType();
/**
* optional string type = 2;
*/
com.google.protobuf.ByteString
getTypeBytes();
// optional string description = 3;
/**
* optional string description = 3;
*/
boolean hasDescription();
/**
* optional string description = 3;
*/
java.lang.String getDescription();
/**
* optional string description = 3;
*/
com.google.protobuf.ByteString
getDescriptionBytes();
}
/**
* Protobuf type {@code louie.ErrorPB}
*/
public static final class ErrorPB extends
com.google.protobuf.GeneratedMessage
implements ErrorPBOrBuilder {
// Use ErrorPB.newBuilder() to construct.
private ErrorPB(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private ErrorPB(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final ErrorPB defaultInstance;
public static ErrorPB getDefaultInstance() {
return defaultInstance;
}
public ErrorPB getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ErrorPB(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
code_ = input.readUInt32();
break;
}
case 18: {
bitField0_ |= 0x00000002;
type_ = input.readBytes();
break;
}
case 26: {
bitField0_ |= 0x00000004;
description_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.rhythm.pb.RequestProtos.internal_static_louie_ErrorPB_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.rhythm.pb.RequestProtos.internal_static_louie_ErrorPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.rhythm.pb.RequestProtos.ErrorPB.class, com.rhythm.pb.RequestProtos.ErrorPB.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public ErrorPB parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ErrorPB(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional uint32 code = 1;
public static final int CODE_FIELD_NUMBER = 1;
private int code_;
/**
* optional uint32 code = 1;
*/
public boolean hasCode() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional uint32 code = 1;
*/
public int getCode() {
return code_;
}
// optional string type = 2;
public static final int TYPE_FIELD_NUMBER = 2;
private java.lang.Object type_;
/**
* optional string type = 2;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string type = 2;
*/
public java.lang.String getType() {
java.lang.Object ref = type_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
type_ = s;
}
return s;
}
}
/**
* optional string type = 2;
*/
public com.google.protobuf.ByteString
getTypeBytes() {
java.lang.Object ref = type_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
type_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string description = 3;
public static final int DESCRIPTION_FIELD_NUMBER = 3;
private java.lang.Object description_;
/**
* optional string description = 3;
*/
public boolean hasDescription() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string description = 3;
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
description_ = s;
}
return s;
}
}
/**
* optional string description = 3;
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
code_ = 0;
type_ = "";
description_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeUInt32(1, code_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getTypeBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, getDescriptionBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, code_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getTypeBytes());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, getDescriptionBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.rhythm.pb.RequestProtos.ErrorPB)) {
return super.equals(obj);
}
com.rhythm.pb.RequestProtos.ErrorPB other = (com.rhythm.pb.RequestProtos.ErrorPB) obj;
boolean result = true;
result = result && (hasCode() == other.hasCode());
if (hasCode()) {
result = result && (getCode()
== other.getCode());
}
result = result && (hasType() == other.hasType());
if (hasType()) {
result = result && getType()
.equals(other.getType());
}
result = result && (hasDescription() == other.hasDescription());
if (hasDescription()) {
result = result && getDescription()
.equals(other.getDescription());
}
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasCode()) {
hash = (37 * hash) + CODE_FIELD_NUMBER;
hash = (53 * hash) + getCode();
}
if (hasType()) {
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + getType().hashCode();
}
if (hasDescription()) {
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.rhythm.pb.RequestProtos.ErrorPB parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.rhythm.pb.RequestProtos.ErrorPB parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.ErrorPB parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.rhythm.pb.RequestProtos.ErrorPB parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.ErrorPB parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.rhythm.pb.RequestProtos.ErrorPB parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.ErrorPB parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.rhythm.pb.RequestProtos.ErrorPB parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.ErrorPB parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.rhythm.pb.RequestProtos.ErrorPB parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.rhythm.pb.RequestProtos.ErrorPB prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code louie.ErrorPB}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.rhythm.pb.RequestProtos.ErrorPBOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.rhythm.pb.RequestProtos.internal_static_louie_ErrorPB_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.rhythm.pb.RequestProtos.internal_static_louie_ErrorPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.rhythm.pb.RequestProtos.ErrorPB.class, com.rhythm.pb.RequestProtos.ErrorPB.Builder.class);
}
// Construct using com.rhythm.pb.RequestProtos.ErrorPB.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
code_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
type_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
description_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.rhythm.pb.RequestProtos.internal_static_louie_ErrorPB_descriptor;
}
public com.rhythm.pb.RequestProtos.ErrorPB getDefaultInstanceForType() {
return com.rhythm.pb.RequestProtos.ErrorPB.getDefaultInstance();
}
public com.rhythm.pb.RequestProtos.ErrorPB build() {
com.rhythm.pb.RequestProtos.ErrorPB result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.rhythm.pb.RequestProtos.ErrorPB buildPartial() {
com.rhythm.pb.RequestProtos.ErrorPB result = new com.rhythm.pb.RequestProtos.ErrorPB(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.code_ = code_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.type_ = type_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.description_ = description_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.rhythm.pb.RequestProtos.ErrorPB) {
return mergeFrom((com.rhythm.pb.RequestProtos.ErrorPB)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.rhythm.pb.RequestProtos.ErrorPB other) {
if (other == com.rhythm.pb.RequestProtos.ErrorPB.getDefaultInstance()) return this;
if (other.hasCode()) {
setCode(other.getCode());
}
if (other.hasType()) {
bitField0_ |= 0x00000002;
type_ = other.type_;
onChanged();
}
if (other.hasDescription()) {
bitField0_ |= 0x00000004;
description_ = other.description_;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.rhythm.pb.RequestProtos.ErrorPB parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.rhythm.pb.RequestProtos.ErrorPB) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional uint32 code = 1;
private int code_ ;
/**
* optional uint32 code = 1;
*/
public boolean hasCode() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional uint32 code = 1;
*/
public int getCode() {
return code_;
}
/**
* optional uint32 code = 1;
*/
public Builder setCode(int value) {
bitField0_ |= 0x00000001;
code_ = value;
onChanged();
return this;
}
/**
* optional uint32 code = 1;
*/
public Builder clearCode() {
bitField0_ = (bitField0_ & ~0x00000001);
code_ = 0;
onChanged();
return this;
}
// optional string type = 2;
private java.lang.Object type_ = "";
/**
* optional string type = 2;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string type = 2;
*/
public java.lang.String getType() {
java.lang.Object ref = type_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
type_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string type = 2;
*/
public com.google.protobuf.ByteString
getTypeBytes() {
java.lang.Object ref = type_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
type_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string type = 2;
*/
public Builder setType(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
type_ = value;
onChanged();
return this;
}
/**
* optional string type = 2;
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000002);
type_ = getDefaultInstance().getType();
onChanged();
return this;
}
/**
* optional string type = 2;
*/
public Builder setTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
type_ = value;
onChanged();
return this;
}
// optional string description = 3;
private java.lang.Object description_ = "";
/**
* optional string description = 3;
*/
public boolean hasDescription() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string description = 3;
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
description_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string description = 3;
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string description = 3;
*/
public Builder setDescription(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
description_ = value;
onChanged();
return this;
}
/**
* optional string description = 3;
*/
public Builder clearDescription() {
bitField0_ = (bitField0_ & ~0x00000004);
description_ = getDefaultInstance().getDescription();
onChanged();
return this;
}
/**
* optional string description = 3;
*/
public Builder setDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
description_ = value;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:louie.ErrorPB)
}
static {
defaultInstance = new ErrorPB(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:louie.ErrorPB)
}
public interface InfoPBOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional string key = 1;
/**
* optional string key = 1;
*/
boolean hasKey();
/**
* optional string key = 1;
*/
java.lang.String getKey();
/**
* optional string key = 1;
*/
com.google.protobuf.ByteString
getKeyBytes();
// optional string value = 2;
/**
* optional string value = 2;
*/
boolean hasValue();
/**
* optional string value = 2;
*/
java.lang.String getValue();
/**
* optional string value = 2;
*/
com.google.protobuf.ByteString
getValueBytes();
}
/**
* Protobuf type {@code louie.InfoPB}
*/
public static final class InfoPB extends
com.google.protobuf.GeneratedMessage
implements InfoPBOrBuilder {
// Use InfoPB.newBuilder() to construct.
private InfoPB(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private InfoPB(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final InfoPB defaultInstance;
public static InfoPB getDefaultInstance() {
return defaultInstance;
}
public InfoPB getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private InfoPB(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
key_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
value_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.rhythm.pb.RequestProtos.internal_static_louie_InfoPB_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.rhythm.pb.RequestProtos.internal_static_louie_InfoPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.rhythm.pb.RequestProtos.InfoPB.class, com.rhythm.pb.RequestProtos.InfoPB.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public InfoPB parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new InfoPB(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional string key = 1;
public static final int KEY_FIELD_NUMBER = 1;
private java.lang.Object key_;
/**
* optional string key = 1;
*/
public boolean hasKey() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string key = 1;
*/
public java.lang.String getKey() {
java.lang.Object ref = key_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
key_ = s;
}
return s;
}
}
/**
* optional string key = 1;
*/
public com.google.protobuf.ByteString
getKeyBytes() {
java.lang.Object ref = key_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
key_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string value = 2;
public static final int VALUE_FIELD_NUMBER = 2;
private java.lang.Object value_;
/**
* optional string value = 2;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string value = 2;
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
value_ = s;
}
return s;
}
}
/**
* optional string value = 2;
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
key_ = "";
value_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getKeyBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getValueBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getKeyBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getValueBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.rhythm.pb.RequestProtos.InfoPB)) {
return super.equals(obj);
}
com.rhythm.pb.RequestProtos.InfoPB other = (com.rhythm.pb.RequestProtos.InfoPB) obj;
boolean result = true;
result = result && (hasKey() == other.hasKey());
if (hasKey()) {
result = result && getKey()
.equals(other.getKey());
}
result = result && (hasValue() == other.hasValue());
if (hasValue()) {
result = result && getValue()
.equals(other.getValue());
}
result = result &&
getUnknownFields().equals(other.getUnknownFields());
return result;
}
private int memoizedHashCode = 0;
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptorForType().hashCode();
if (hasKey()) {
hash = (37 * hash) + KEY_FIELD_NUMBER;
hash = (53 * hash) + getKey().hashCode();
}
if (hasValue()) {
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.rhythm.pb.RequestProtos.InfoPB parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.rhythm.pb.RequestProtos.InfoPB parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.InfoPB parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.rhythm.pb.RequestProtos.InfoPB parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.InfoPB parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.rhythm.pb.RequestProtos.InfoPB parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.InfoPB parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static com.rhythm.pb.RequestProtos.InfoPB parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static com.rhythm.pb.RequestProtos.InfoPB parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static com.rhythm.pb.RequestProtos.InfoPB parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(com.rhythm.pb.RequestProtos.InfoPB prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code louie.InfoPB}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements com.rhythm.pb.RequestProtos.InfoPBOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.rhythm.pb.RequestProtos.internal_static_louie_InfoPB_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.rhythm.pb.RequestProtos.internal_static_louie_InfoPB_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.rhythm.pb.RequestProtos.InfoPB.class, com.rhythm.pb.RequestProtos.InfoPB.Builder.class);
}
// Construct using com.rhythm.pb.RequestProtos.InfoPB.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
key_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
value_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.rhythm.pb.RequestProtos.internal_static_louie_InfoPB_descriptor;
}
public com.rhythm.pb.RequestProtos.InfoPB getDefaultInstanceForType() {
return com.rhythm.pb.RequestProtos.InfoPB.getDefaultInstance();
}
public com.rhythm.pb.RequestProtos.InfoPB build() {
com.rhythm.pb.RequestProtos.InfoPB result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.rhythm.pb.RequestProtos.InfoPB buildPartial() {
com.rhythm.pb.RequestProtos.InfoPB result = new com.rhythm.pb.RequestProtos.InfoPB(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.key_ = key_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.value_ = value_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.rhythm.pb.RequestProtos.InfoPB) {
return mergeFrom((com.rhythm.pb.RequestProtos.InfoPB)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.rhythm.pb.RequestProtos.InfoPB other) {
if (other == com.rhythm.pb.RequestProtos.InfoPB.getDefaultInstance()) return this;
if (other.hasKey()) {
bitField0_ |= 0x00000001;
key_ = other.key_;
onChanged();
}
if (other.hasValue()) {
bitField0_ |= 0x00000002;
value_ = other.value_;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.rhythm.pb.RequestProtos.InfoPB parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.rhythm.pb.RequestProtos.InfoPB) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional string key = 1;
private java.lang.Object key_ = "";
/**
* optional string key = 1;
*/
public boolean hasKey() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string key = 1;
*/
public java.lang.String getKey() {
java.lang.Object ref = key_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
key_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string key = 1;
*/
public com.google.protobuf.ByteString
getKeyBytes() {
java.lang.Object ref = key_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
key_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string key = 1;
*/
public Builder setKey(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
key_ = value;
onChanged();
return this;
}
/**
* optional string key = 1;
*/
public Builder clearKey() {
bitField0_ = (bitField0_ & ~0x00000001);
key_ = getDefaultInstance().getKey();
onChanged();
return this;
}
/**
* optional string key = 1;
*/
public Builder setKeyBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
key_ = value;
onChanged();
return this;
}
// optional string value = 2;
private java.lang.Object value_ = "";
/**
* optional string value = 2;
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string value = 2;
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
value_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string value = 2;
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string value = 2;
*/
public Builder setValue(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
value_ = value;
onChanged();
return this;
}
/**
* optional string value = 2;
*/
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000002);
value_ = getDefaultInstance().getValue();
onChanged();
return this;
}
/**
* optional string value = 2;
*/
public Builder setValueBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
value_ = value;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:louie.InfoPB)
}
static {
defaultInstance = new InfoPB(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:louie.InfoPB)
}
private static com.google.protobuf.Descriptors.Descriptor
internal_static_louie_RequestHeaderPB_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_louie_RequestHeaderPB_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_louie_RoutePB_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_louie_RoutePB_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_louie_RoutePathPB_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_louie_RoutePathPB_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_louie_IdentityPB_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_louie_IdentityPB_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_louie_SessionKey_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_louie_SessionKey_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_louie_SessionBPB_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_louie_SessionBPB_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_louie_SessionStatsPB_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_louie_SessionStatsPB_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_louie_RequestPB_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_louie_RequestPB_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_louie_ResponseHeaderPB_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_louie_ResponseHeaderPB_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_louie_ResponsePB_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_louie_ResponsePB_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_louie_ErrorPB_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_louie_ErrorPB_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_louie_InfoPB_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_louie_InfoPB_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\023louie/request.proto\022\005louie\"\300\001\n\017Request" +
"HeaderPB\022\020\n\004user\030\001 \001(\tB\002\030\001\022\r\n\005agent\030\002 \001(" +
"\t\022\r\n\005count\030\003 \001(\r\022\036\n\003key\030\n \001(\0132\021.louie.Se" +
"ssionKey\022#\n\010identity\030\013 \001(\0132\021.louie.Ident" +
"ityPB\022!\n\005route\030\024 \003(\0132\016.louie.RoutePBB\002\030\001" +
"\022\025\n\trouteUser\030\036 \001(\tB\002\030\001\"L\n\007RoutePB\022\016\n\006ho" +
"stIp\030\001 \001(\t\022\017\n\007gateway\030\002 \001(\t\022\017\n\007service\030\003" +
" \001(\t\022\017\n\007variant\030\004 \001(\t\"N\n\013RoutePathPB\022\035\n\005" +
"route\030\001 \001(\0132\016.louie.RoutePB\022 \n\004path\030\002 \003(" +
"\0132\022.louie.RoutePathPB\"\352\001\n\nIdentityPB\022\014\n\004",
"user\030\001 \001(\t\022\020\n\010language\030\002 \001(\t\022\017\n\007program\030" +
"\003 \001(\t\022\014\n\004path\030\004 \001(\t\022\020\n\010location\030\005 \001(\t\022\017\n" +
"\007machine\030\006 \001(\t\022\013\n\003env\030\007 \001(\t\022\n\n\002ip\030\010 \001(\t\022" +
"\027\n\017languageVersion\030\t \001(\t\022\026\n\016programVersi" +
"on\030\n \001(\t\022\n\n\002os\030\013 \001(\t\022\021\n\tosVersion\030\014 \001(\t\022" +
"\021\n\tprocessId\030\r \001(\t\"\031\n\nSessionKey\022\013\n\003key\030" +
"\001 \001(\t\"w\n\nSessionBPB\022\036\n\003key\030\001 \001(\0132\021.louie" +
".SessionKey\022#\n\010identity\030\002 \001(\0132\021.louie.Id" +
"entityPB\022$\n\005stats\030\003 \001(\0132\025.louie.SessionS" +
"tatsPB\"S\n\016SessionStatsPB\022\024\n\014requestCount",
"\030\001 \001(\021\022\022\n\ncreateTime\030\002 \001(\004\022\027\n\017lastReques" +
"tTime\030\003 \001(\004\"\267\001\n\tRequestPB\022\n\n\002id\030\001 \001(\r\022\017\n" +
"\007service\030\002 \001(\t\022\016\n\006method\030\003 \001(\t\022\014\n\004type\030\004" +
" \003(\t\022\027\n\013param_count\030\005 \001(\rB\002\030\001\022\035\n\005route\030\024" +
" \003(\0132\016.louie.RoutePB\022\021\n\trouteUser\030\025 \001(\t\022" +
"\020\n\010threadId\030\026 \001(\022\022\022\n\nstart_time\030\027 \001(\022\"^\n" +
"\020ResponseHeaderPB\022\r\n\005count\030\001 \001(\r\022\036\n\003key\030" +
"\002 \001(\0132\021.louie.SessionKey\022\033\n\004info\030\003 \003(\0132\r" +
".louie.InfoPB\"w\n\nResponsePB\022\n\n\002id\030\001 \001(\r\022" +
"\r\n\005count\030\002 \001(\r\022\014\n\004type\030\003 \001(\t\022\035\n\005error\030\004 ",
"\001(\0132\016.louie.ErrorPB\022!\n\005route\030\024 \003(\0132\022.lou" +
"ie.RoutePathPB\":\n\007ErrorPB\022\014\n\004code\030\001 \001(\r\022" +
"\014\n\004type\030\002 \001(\t\022\023\n\013description\030\003 \001(\t\"$\n\006In" +
"foPB\022\013\n\003key\030\001 \001(\t\022\r\n\005value\030\002 \001(\tB!\n\rcom." +
"rhythm.pbB\rRequestProtos\240\001\001"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
internal_static_louie_RequestHeaderPB_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_louie_RequestHeaderPB_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_louie_RequestHeaderPB_descriptor,
new java.lang.String[] { "User", "Agent", "Count", "Key", "Identity", "Route", "RouteUser", });
internal_static_louie_RoutePB_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_louie_RoutePB_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_louie_RoutePB_descriptor,
new java.lang.String[] { "HostIp", "Gateway", "Service", "Variant", });
internal_static_louie_RoutePathPB_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_louie_RoutePathPB_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_louie_RoutePathPB_descriptor,
new java.lang.String[] { "Route", "Path", });
internal_static_louie_IdentityPB_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_louie_IdentityPB_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_louie_IdentityPB_descriptor,
new java.lang.String[] { "User", "Language", "Program", "Path", "Location", "Machine", "Env", "Ip", "LanguageVersion", "ProgramVersion", "Os", "OsVersion", "ProcessId", });
internal_static_louie_SessionKey_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_louie_SessionKey_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_louie_SessionKey_descriptor,
new java.lang.String[] { "Key", });
internal_static_louie_SessionBPB_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_louie_SessionBPB_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_louie_SessionBPB_descriptor,
new java.lang.String[] { "Key", "Identity", "Stats", });
internal_static_louie_SessionStatsPB_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_louie_SessionStatsPB_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_louie_SessionStatsPB_descriptor,
new java.lang.String[] { "RequestCount", "CreateTime", "LastRequestTime", });
internal_static_louie_RequestPB_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_louie_RequestPB_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_louie_RequestPB_descriptor,
new java.lang.String[] { "Id", "Service", "Method", "Type", "ParamCount", "Route", "RouteUser", "ThreadId", "StartTime", });
internal_static_louie_ResponseHeaderPB_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_louie_ResponseHeaderPB_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_louie_ResponseHeaderPB_descriptor,
new java.lang.String[] { "Count", "Key", "Info", });
internal_static_louie_ResponsePB_descriptor =
getDescriptor().getMessageTypes().get(9);
internal_static_louie_ResponsePB_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_louie_ResponsePB_descriptor,
new java.lang.String[] { "Id", "Count", "Type", "Error", "Route", });
internal_static_louie_ErrorPB_descriptor =
getDescriptor().getMessageTypes().get(10);
internal_static_louie_ErrorPB_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_louie_ErrorPB_descriptor,
new java.lang.String[] { "Code", "Type", "Description", });
internal_static_louie_InfoPB_descriptor =
getDescriptor().getMessageTypes().get(11);
internal_static_louie_InfoPB_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_louie_InfoPB_descriptor,
new java.lang.String[] { "Key", "Value", });
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
}, assigner);
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy