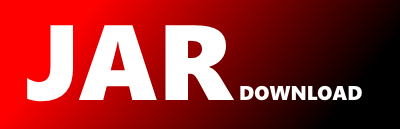
com.riskalyze.alerts.drivers.opsgenie.OpsGenieAlertsService.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of alerts-kotlin Show documentation
Show all versions of alerts-kotlin Show documentation
This alerts module is used to standardize alerting for JVM-based application. It uses a modular driver system to allow easily swapping out alerting services.
The newest version!
package com.riskalyze.alerts.drivers.opsgenie
import com.google.gson.FieldNamingPolicy
import com.riskalyze.alerts.AlertsService
import com.riskalyze.alerts.drivers.opsgenie.dtos.OpsGenieAlertResponseDTO
import com.riskalyze.alerts.enumerations.Priority
import com.riskalyze.alerts.http.HttpClient
import com.riskalyze.alerts.models.Alert
import com.riskalyze.alerts.models.AlertResponse
import com.riskalyze.alerts.models.Credentials
import java.util.concurrent.CompletableFuture
import java.util.concurrent.TimeUnit.SECONDS
internal class OpsGenieAlertsService(
private val client: HttpClient,
private val adapter: OpsGenieAdapter,
credentials: Credentials
): AlertsService {
init {
client.baseUrl = "https://api.opsgenie.com"
client.setConnectTimeout(20, SECONDS)
for ((name, value) in credentials.getAuthHeaders()) {
client.setHeader(name, value)
}
client.setNamingPolicy(FieldNamingPolicy.IDENTITY)
}
override fun send(alert: Alert): CompletableFuture {
val payload = adapter.convertAlertToDTO(alert)
val request = client.post("/v2/alerts", payload, OpsGenieAlertResponseDTO::class)
return request.thenApplyAsync {
adapter.convertResponse(it)
}
}
override fun close(alias: String): CompletableFuture {
val request = client.post("/v2/alerts/$alias/close?identifierType=alias", OpsGenieAlertResponseDTO::class)
return request.thenApplyAsync {
adapter.convertResponse(it)
}
}
override fun report(exception: Exception, tags: List): CompletableFuture {
val className = exception::class.simpleName ?: "Exception"
val allTags = tags.union(listOf("exception")).toList()
return buildAndSend(className, Priority.HIGH, exception.message, null, allTags)
}
override fun info(message: String, description: String?, tags: List): CompletableFuture {
return buildAndSend(message, Priority.INFO, description, null, tags)
}
override fun low(message: String, description: String?, tags: List): CompletableFuture {
return buildAndSend(message, Priority.LOW, description, null, tags)
}
override fun moderate(message: String, description: String?, tags: List): CompletableFuture {
return buildAndSend(message, Priority.MODERATE, description, null, tags)
}
override fun high(message: String, description: String?, tags: List): CompletableFuture {
return buildAndSend(message, Priority.HIGH, description, null, tags)
}
override fun critical(message: String, description: String?, tags: List): CompletableFuture {
return buildAndSend(message, Priority.CRITICAL, description, null, tags)
}
private fun buildAndSend(
message: String,
priority: Priority,
description: String?,
alias: String?,
tags: List
): CompletableFuture {
val alert = Alert(message, priority, description, alias, tags)
return send(alert)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy