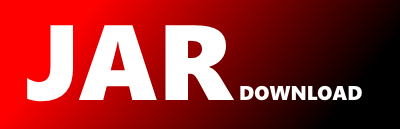
jais.AISSentence Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jais Show documentation
Show all versions of jais Show documentation
Java NMEA AIS decoding library
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy