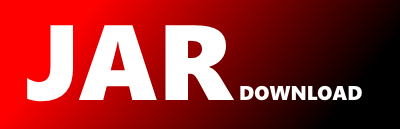
com.robrua.orianna.api.dto.ChampionMasteryAPI Maven / Gradle / Ivy
The newest version!
package com.robrua.orianna.api.dto;
import java.lang.reflect.Type;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.google.gson.reflect.TypeToken;
import com.robrua.orianna.type.core.common.PlatformID;
import com.robrua.orianna.type.dto.championmastery.ChampionMastery;
public abstract class ChampionMasteryAPI {
/**
* @param summonerID
* the summoner to get champion mastery for
* @return the summoner's mastery for all their champions
* @see
* Riot API Specification
*/
public static List getChampionMastery(final long summonerID) {
final PlatformID platform = PlatformID.fromRegion(BaseRiotAPI.region);
final String request = "/championmastery/location/" + platform + "/player/" + summonerID + "/champions";
final Type type = new TypeToken>() {}.getType();
return BaseRiotAPI.GSON.fromJson(BaseRiotAPI.getRoot(request, null, false), type);
}
/**
* @param summonerID
* the ID of the summoner to get mastery for
* @param championID
* the ID of the champion to get mastery for
* @return the champion's mastery score
* @see
* Riot API Specification
*/
public static ChampionMastery getChampionMastery(final long summonerID, final long championID) {
final PlatformID platform = PlatformID.fromRegion(BaseRiotAPI.region);
final String request = "/championmastery/location/" + platform + "/player/" + summonerID + "/champion/" + championID;
return BaseRiotAPI.GSON.fromJson(BaseRiotAPI.getRoot(request, null, false), ChampionMastery.class);
}
/**
* @param summonerID
* the summoner to get masteries for
* @param count
* the number of top champions to get
* @return the *count* highest champion masteries for the summoner
* @see
* Riot API Specification
*/
public static List getTopChampionMastery(final long summonerID, final int count) {
if(count <= 0) {
throw new IllegalArgumentException("Count must be positive!");
}
final PlatformID platform = PlatformID.fromRegion(BaseRiotAPI.region);
final Map params = new HashMap<>();
params.put("count", Integer.toString(count));
final String request = "/championmastery/location/" + platform + "/player/" + summonerID + "/topchampions";
final Type type = new TypeToken>() {}.getType();
return BaseRiotAPI.GSON.fromJson(BaseRiotAPI.getRoot(request, params, false), type);
}
/**
* @param summonerID
* the summoner to get total mastery level for
* @return the summoner's total mastery level
* @see
* Riot API Specification
*/
public static int getTotalMasteryLevel(final long summonerID) {
final PlatformID platform = PlatformID.fromRegion(BaseRiotAPI.region);
final String request = "/championmastery/location/" + platform + "/player/" + summonerID + "/score";
return Integer.parseInt(BaseRiotAPI.getRoot(request, null, false));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy