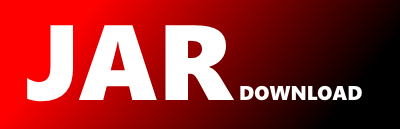
com.rometools.propono.atom.server.SecuritySupport Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rome-propono Show documentation
Show all versions of rome-propono Show documentation
The ROME Propono subproject is a Java class library that
supports publishing protocols, specifically the Atom Publishing Protocol
and the legacy MetaWeblog API. Propono includes an Atom client library,
Atom server framework and a Blog client that supports both Atom protocol
and the MetaWeblog API.
/*
* Copyright 2007 Sun Microsystems, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.rometools.propono.atom.server;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.InputStream;
import java.security.AccessController;
import java.security.PrivilegedAction;
import java.security.PrivilegedActionException;
import java.security.PrivilegedExceptionAction;
/**
* This class is duplicated for each subpackage, it is package private and therefore is not exposed
* as part of the public API.
*/
class SecuritySupport {
ClassLoader getContextClassLoader() {
final PrivilegedAction action = new PrivilegedAction() {
@Override
public ClassLoader run() {
ClassLoader cl = null;
try {
cl = Thread.currentThread().getContextClassLoader();
} catch (final SecurityException ex) {
}
return cl;
}
};
return AccessController.doPrivileged(action);
}
String getSystemProperty(final String propName) {
final PrivilegedAction action = new PrivilegedAction() {
@Override
public String run() {
return System.getProperty(propName);
}
};
return AccessController.doPrivileged(action);
}
FileInputStream getFileInputStream(final File file) throws FileNotFoundException {
try {
final PrivilegedExceptionAction action = new PrivilegedExceptionAction() {
@Override
public FileInputStream run() throws FileNotFoundException {
return new FileInputStream(file);
}
};
return AccessController.doPrivileged(action);
} catch (final PrivilegedActionException e) {
throw (FileNotFoundException) e.getException();
}
}
InputStream getResourceAsStream(final ClassLoader cl, final String name) {
final PrivilegedAction action = new PrivilegedAction() {
@Override
public InputStream run() {
InputStream ris;
if (cl == null) {
ris = ClassLoader.getSystemResourceAsStream(name);
} else {
ris = cl.getResourceAsStream(name);
}
return ris;
}
};
return AccessController.doPrivileged(action);
}
boolean doesFileExist(final File f) {
final PrivilegedAction action = new PrivilegedAction() {
@Override
public Boolean run() {
return f.exists();
}
};
return AccessController.doPrivileged(action).booleanValue();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy