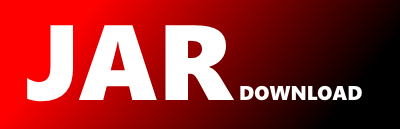
com.rometools.rome.feed.module.DCModule Maven / Gradle / Ivy
Show all versions of rome Show documentation
/*
* Copyright 2004 Sun Microsystems, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package com.rometools.rome.feed.module;
import java.util.Date;
import java.util.List;
/**
* Dublin Core Module.
*
*
* @see Dublin Core module.
*/
public interface DCModule extends Module {
/**
* URI of the Dublin Core Module (http://purl.org/dc/elements/1.1/).
*
*/
String URI = "http://purl.org/dc/elements/1.1/";
/**
* Returns the DublinCore module titles.
*
*
* @return a list of Strings representing the DublinCore module title, an empty list if none.
*
*/
List getTitles();
/**
* Sets the DublinCore module titles.
*
*
* @param titles the list of String representing the DublinCore module titles to set, an empty
* list or null if none.
*
*/
void setTitles(List titles);
/**
* Gets the DublinCore module title. Convenience method that can be used to obtain the first
* item, null if none.
*
*
* @return the first DublinCore module title, null if none.
*/
String getTitle();
/**
* Sets the DublinCore module title. Convenience method that can be used when there is only one
* title to set.
*
*
* @param title the DublinCore module title to set, null if none.
*
*/
void setTitle(String title);
/**
* Returns the DublinCore module creator.
*
*
* @return a list of Strings representing the DublinCore module creator, an empty list if none.
*
*/
List getCreators();
/**
* Sets the DublinCore module creators.
*
*
* @param creators the list of String representing the DublinCore module creators to set, an
* empty list or null if none.
*
*/
void setCreators(List creators);
/**
* Gets the DublinCore module creator. Convenience method that can be used to obtain the first
* item, null if none.
*
*
* @return the first DublinCore module creator, null if none.
*/
String getCreator();
/**
* Sets the DublinCore module creator. Convenience method that can be used when there is only
* one creator to set.
*
*
* @param creator the DublinCore module creator to set, null if none.
*
*/
void setCreator(String creator);
/**
* Returns the DublinCore module subjects.
*
*
* @return a list of DCSubject elements with the DublinCore module subjects, an empty list if
* none.
*
*/
List getSubjects();
/**
* Sets the DublinCore module subjects.
*
*
* @param subjects the list of DCSubject elements with the DublinCore module subjects to set, an
* empty list or null if none.
*
*/
void setSubjects(List subjects);
/**
* Gets the DublinCore module subject. Convenience method that can be used to obtain the first
* item, null if none.
*
*
* @return the first DublinCore module subject, null if none.
*/
DCSubject getSubject();
/**
* Sets the DCSubject element. Convenience method that can be used when there is only one
* subject to set.
*
*
* @param subject the DublinCore module subject to set, null if none.
*
*/
void setSubject(DCSubject subject);
/**
* Returns the DublinCore module description.
*
*
* @return a list of Strings representing the DublinCore module description, an empty list if
* none.
*
*/
List getDescriptions();
/**
* Sets the DublinCore module descriptions.
*
*
* @param descriptions the list of String representing the DublinCore module descriptions to
* set, an empty list or null if none.
*
*/
void setDescriptions(List descriptions);
/**
* Gets the DublinCore module description. Convenience method that can be used to obtain the
* first item, null if none.
*
*
* @return the first DublinCore module description, null if none.
*/
String getDescription();
/**
* Sets the DublinCore module description. Convenience method that can be used when there is
* only one description to set.
*
*
* @param description the DublinCore module description to set, null if none.
*
*/
void setDescription(String description);
/**
* Returns the DublinCore module publisher.
*
*
* @return a list of Strings representing the DublinCore module publisher, an empty list if
* none.
*
*/
List getPublishers();
/**
* Sets the DublinCore module publishers.
*
*
* @param publishers the list of String representing the DublinCore module publishers to set, an
* empty list or null if none.
*
*/
void setPublishers(List publishers);
/**
* Gets the DublinCore module publisher. Convenience method that can be used to obtain the first
* item, null if none.
*
*
* @return the first DublinCore module publisher, null if none.
*/
String getPublisher();
/**
* Sets the DublinCore module publisher. Convenience method that can be used when there is only
* one publisher to set.
*
*
* @param publisher the DublinCore module publisher to set, null if none.
*
*/
void setPublisher(String publisher);
/**
* Returns the DublinCore module contributor.
*
*
* @return a list of Strings representing the DublinCore module contributor, an empty list if
* none.
*
*/
List getContributors();
/**
* Sets the DublinCore module contributors.
*
*
* @param contributors the list of String representing the DublinCore module contributors to
* set, an empty list or null if none.
*
*/
void setContributors(List contributors);
/**
* Gets the DublinCore module contributor. Convenience method that can be used to obtain the
* first item, null if none.
*
*
* @return the first DublinCore module contributor, null if none.
*/
String getContributor();
/**
* Sets the DublinCore module contributor. Convenience method that can be used when there is
* only one contributor to set.
*
*
* @param contributor the DublinCore module contributor to set, null if none.
*
*/
void setContributor(String contributor);
/**
* Returns the DublinCore module date.
*
*
* @return a list of Strings representing the DublinCore module date, an empty list if none.
*
*/
List getDates();
/**
* Sets the DublinCore module dates.
*
*
* @param dates the list of Date representing the DublinCore module dates to set, an empty list
* or null if none.
*
*/
void setDates(List dates);
/**
* Gets the DublinCore module date. Convenience method that can be used to obtain the first
* item, null if none.
*
*
* @return the first DublinCore module date, null if none.
*/
Date getDate();
/**
* Sets the DublinCore module date. Convenience method that can be used when there is only one
* date to set.
*
*
* @param date the DublinCore module date to set, null if none.
*
*/
void setDate(Date date);
/**
* Returns the DublinCore module type.
*
*
* @return a list of Strings representing the DublinCore module type, an empty list if none.
*
*/
List getTypes();
/**
* Sets the DublinCore module types.
*
*
* @param types the list of String representing the DublinCore module types to set, an empty
* list or null if none.
*
*/
void setTypes(List types);
/**
* Gets the DublinCore module type. Convenience method that can be used to obtain the first
* item, null if none.
*
*
* @return the first DublinCore module type, null if none.
*/
String getType();
/**
* Sets the DublinCore module type. Convenience method that can be used when there is only one
* type to set.
*
*
* @param type the DublinCore module type to set, null if none.
*
*/
void setType(String type);
/**
* Returns the DublinCore module format.
*
*
* @return a list of Strings representing the DublinCore module format, an empty list if none.
*
*/
List getFormats();
/**
* Sets the DublinCore module formats.
*
*
* @param formats the list of String representing the DublinCore module formats to set, an empty
* list or null if none.
*
*/
void setFormats(List formats);
/**
* Gets the DublinCore module format. Convenience method that can be used to obtain the first
* item, null if none.
*
*
* @return the first DublinCore module format, null if none.
*/
String getFormat();
/**
* Sets the DublinCore module format. Convenience method that can be used when there is only one
* format to set.
*
*
* @param format the DublinCore module format to set, null if none.
*
*/
void setFormat(String format);
/**
* Returns the DublinCore module identifier.
*
*
* @return a list of Strings representing the DublinCore module identifier, an empty list if
* none.
*
*/
List getIdentifiers();
/**
* Sets the DublinCore module identifiers.
*
*
* @param identifiers the list of String representing the DublinCore module identifiers to set,
* an empty list or null if none.
*
*/
void setIdentifiers(List identifiers);
/**
* Gets the DublinCore module identifier. Convenience method that can be used to obtain the
* first item, null if none.
*
*
* @return the first DublinCore module identifier, null if none.
*/
String getIdentifier();
/**
* Sets the DublinCore module identifier. Convenience method that can be used when there is only
* one identifier to set.
*
*
* @param identifier the DublinCore module identifier to set, null if none.
*
*/
void setIdentifier(String identifier);
/**
* Returns the DublinCore module source.
*
*
* @return a list of Strings representing the DublinCore module source, an empty list if none.
*
*/
List getSources();
/**
* Sets the DublinCore module sources.
*
*
* @param sources the list of String representing the DublinCore module sources to set, an empty
* list or null if none.
*
*/
void setSources(List sources);
/**
* Gets the DublinCore module subject. Convenience method that can be used to obtain the first
* item, null if none.
*
*
* @return the first DublinCore module creator, null if none.
*/
String getSource();
/**
* Sets the DublinCore module source. Convenience method that can be used when there is only one
* source to set.
*
*
* @param source the DublinCore module source to set, null if none.
*
*/
void setSource(String source);
/**
* Returns the DublinCore module language.
*
*
* @return a list of Strings representing the DublinCore module language, an empty list if none.
*
*/
List getLanguages();
/**
* Sets the DublinCore module languages.
*
*
* @param languages the list of String representing the DublinCore module languages to set, an
* empty list or null if none.
*
*/
void setLanguages(List languages);
/**
* Gets the DublinCore module language. Convenience method that can be used to obtain the first
* item, null if none.
*
*
* @return the first DublinCore module language, null if none.
*/
String getLanguage();
/**
* Sets the DublinCore module language. Convenience method that can be used when there is only
* one language to set.
*
*
* @param language the DublinCore module language to set, null if none.
*
*/
void setLanguage(String language);
/**
* Returns the DublinCore module relation.
*
*
* @return a list of Strings representing the DublinCore module relation, an empty list if none.
*
*/
List getRelations();
/**
* Sets the DublinCore module relations.
*
*
* @param relations the list of String representing the DublinCore module relations to set, an
* empty list or null if none.
*
*/
void setRelations(List relations);
/**
* Gets the DublinCore module relation. Convenience method that can be used to obtain the first
* item, null if none.
*
*
* @return the first DublinCore module relation, null if none.
*/
String getRelation();
/**
* Sets the DublinCore module relation. Convenience method that can be used when there is only
* one relation to set.
*
*
* @param relation the DublinCore module relation to set, null if none.
*
*/
void setRelation(String relation);
/**
* Returns the DublinCore module coverage.
*
*
* @return a list of Strings representing the DublinCore module coverage, an empty list if none.
*
*/
List getCoverages();
/**
* Sets the DublinCore module coverages.
*
*
* @param coverages the list of String representing the DublinCore module coverages to set, an
* empty list or null if none.
*
*/
void setCoverages(List coverages);
/**
* Gets the DublinCore module coverage. Convenience method that can be used to obtain the first
* item, null if none.
*
*
* @return the first DublinCore module coverage, null if none.
*/
String getCoverage();
/**
* Sets the DublinCore module coverage. Convenience method that can be used when there is only
* one coverage to set.
*
*
* @param coverage the DublinCore module coverage to set, null if none.
*
*/
void setCoverage(String coverage);
/**
* Returns the DublinCore module rights.
*
*
* @return a list of Strings representing the DublinCore module rights, an empty list if none.
*
*/
List getRightsList();
/**
* Sets the DublinCore module rightss.
*
*
* @param rights the list of String representing the DublinCore module rights to set, an empty
* list or null if none.
*
*/
void setRightsList(List rights);
/**
* Gets the DublinCore module right. Convenience method that can be used to obtain the first
* item, null if none.
*
*
* @return the first DublinCore module right, null if none.
*/
String getRights();
/**
* Sets the DublinCore module rights. Convenience method that can be used when there is only one
* rights to set.
*
*
* @param rights the DublinCore module rights to set, null if none.
*
*/
void setRights(String rights);
}