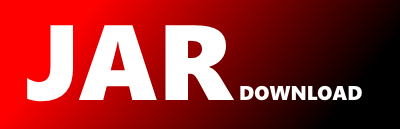
com.rometools.rome.feed.module.DCModuleImpl Maven / Gradle / Ivy
Show all versions of rome Show documentation
/*
* Copyright 2004 Sun Microsystems, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package com.rometools.rome.feed.module;
import java.util.Collections;
import java.util.Date;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.rometools.rome.feed.CopyFrom;
import com.rometools.rome.feed.impl.CloneableBean;
import com.rometools.rome.feed.impl.CopyFromHelper;
import com.rometools.rome.feed.impl.EqualsBean;
import com.rometools.rome.feed.impl.ToStringBean;
import com.rometools.utils.Lists;
/**
* Dublin Core ModuleImpl, default implementation.
*
*
* @see Dublin Core module.
*/
public class DCModuleImpl extends ModuleImpl implements DCModule {
private static final long serialVersionUID = 1L;
private List title;
private List creator;
private List subject;
private List description;
private List publisher;
private List contributors;
private List date;
private List type;
private List format;
private List identifier;
private List source;
private List language;
private List relation;
private List coverage;
private List rights;
/**
* Properties to be ignored when cloning.
*/
private static final Set IGNORE_PROPERTIES = new HashSet();
/**
* Unmodifiable Set containing the convenience properties of this class.
*
* Convenience properties are mapped to Modules, for cloning the convenience properties can be
* ignored as the will be copied as part of the module cloning.
*/
public static final Set CONVENIENCE_PROPERTIES = Collections.unmodifiableSet(IGNORE_PROPERTIES);
static {
IGNORE_PROPERTIES.add("title");
IGNORE_PROPERTIES.add("creator");
IGNORE_PROPERTIES.add("subject");
IGNORE_PROPERTIES.add("description");
IGNORE_PROPERTIES.add("publisher");
IGNORE_PROPERTIES.add("contributor");
IGNORE_PROPERTIES.add("date");
IGNORE_PROPERTIES.add("type");
IGNORE_PROPERTIES.add("format");
IGNORE_PROPERTIES.add("identifier");
IGNORE_PROPERTIES.add("source");
IGNORE_PROPERTIES.add("language");
IGNORE_PROPERTIES.add("relation");
IGNORE_PROPERTIES.add("coverage");
IGNORE_PROPERTIES.add("rights");
}
public DCModuleImpl() {
super(DCModule.class, URI);
}
/**
* Returns the DublinCore module titles.
*
*
* @return a list of Strings representing the DublinCore module title, an empty list if none.
*
*/
@Override
public List getTitles() {
return title = Lists.createWhenNull(title);
}
/**
* Sets the DublinCore module titles.
*
*
* @param titles the list of String representing the DublinCore module titles to set, an empty
* list or null if none.
*
*/
@Override
public void setTitles(final List titles) {
title = titles;
}
/**
* Gets the DublinCore module title. Convenience method that can be used to obtain the first
* item, null if none.
*
*
* @return the first DublinCore module title, null if none.
*/
@Override
public String getTitle() {
return Lists.firstEntry(title);
}
/**
* Sets the DublinCore module title. Convenience method that can be used when there is only one
* title to set.
*
*
* @param title the DublinCore module title to set, null if none.
*
*/
@Override
public void setTitle(final String title) {
this.title = Lists.create(title);
}
/**
* Returns the DublinCore module creator.
*
*
* @return a list of Strings representing the DublinCore module creator, an empty list if none.
*
*/
@Override
public List getCreators() {
return creator = Lists.createWhenNull(creator);
}
/**
* Sets the DublinCore module creators.
*
*
* @param creators the list of String representing the DublinCore module creators to set, an
* empty list or null if none.
*
*/
@Override
public void setCreators(final List creators) {
creator = creators;
}
/**
* Gets the DublinCore module title. Convenience method that can be used to obtain the first
* item, null if none.
*
*
* @return the first DublinCore module title, null if none.
*/
@Override
public String getCreator() {
return Lists.firstEntry(creator);
}
/**
* Sets the DublinCore module creator. Convenience method that can be used when there is only
* one creator to set.
*
*
* @param creator the DublinCore module creator to set, null if none.
*
*/
@Override
public void setCreator(final String creator) {
this.creator = Lists.create(creator);
}
/**
* Returns the DublinCore module subjects.
*
*
* @return a list of DCSubject elements with the DublinCore module subjects, an empty list if
* none.
*
*/
@Override
public List getSubjects() {
return subject = Lists.createWhenNull(subject);
}
/**
* Sets the DublinCore module subjects.
*
*
* @param subjects the list of DCSubject elements with the DublinCore module subjects to set, an
* empty list or null if none.
*
*/
@Override
public void setSubjects(final List subjects) {
subject = subjects;
}
/**
* Gets the DublinCore module subject. Convenience method that can be used to obtain the first
* item, null if none.
*
*
* @return the first DublinCore module subject, null if none.
*/
@Override
public DCSubject getSubject() {
return Lists.firstEntry(subject);
}
/**
* Sets the DCSubject element. Convenience method that can be used when there is only one
* subject to set.
*
*
* @param subject the DublinCore module subject to set, null if none.
*
*/
@Override
public void setSubject(final DCSubject subject) {
this.subject = Lists.create(subject);
}
/**
* Returns the DublinCore module description.
*
*
* @return a list of Strings representing the DublinCore module description, an empty list if
* none.
*
*/
@Override
public List getDescriptions() {
return description = Lists.createWhenNull(description);
}
/**
* Sets the DublinCore module descriptions.
*
*
* @param descriptions the list of String representing the DublinCore module descriptions to
* set, an empty list or null if none.
*
*/
@Override
public void setDescriptions(final List descriptions) {
description = descriptions;
}
/**
* Gets the DublinCore module description. Convenience method that can be used to obtain the
* first item, null if none.
*
*
* @return the first DublinCore module description, null if none.
*/
@Override
public String getDescription() {
return Lists.firstEntry(description);
}
/**
* Sets the DublinCore module description. Convenience method that can be used when there is
* only one description to set.
*
*
* @param description the DublinCore module description to set, null if none.
*
*/
@Override
public void setDescription(final String description) {
this.description = Lists.create(description);
}
/**
* Returns the DublinCore module publisher.
*
*
* @return a list of Strings representing the DublinCore module publisher, an empty list if
* none.
*
*/
@Override
public List getPublishers() {
return publisher = Lists.createWhenNull(publisher);
}
/**
* Sets the DublinCore module publishers.
*
*
* @param publishers the list of String representing the DublinCore module publishers to set, an
* empty list or null if none.
*
*/
@Override
public void setPublishers(final List publishers) {
publisher = publishers;
}
/**
* Gets the DublinCore module title. Convenience method that can be used to obtain the first
* item, null if none.
*
*
* @return the first DublinCore module title, null if none.
*/
@Override
public String getPublisher() {
return Lists.firstEntry(publisher);
}
/**
* Sets the DublinCore module publisher. Convenience method that can be used when there is only
* one publisher to set.
*
*
* @param publisher the DublinCore module publisher to set, null if none.
*
*/
@Override
public void setPublisher(final String publisher) {
this.publisher = Lists.create(publisher);
}
/**
* Returns the DublinCore module contributor.
*
*
* @return a list of Strings representing the DublinCore module contributor, an empty list if
* none.
*
*/
@Override
public List getContributors() {
return contributors = Lists.createWhenNull(contributors);
}
/**
* Sets the DublinCore module contributors.
*
*
* @param contributors the list of String representing the DublinCore module contributors to
* set, an empty list or null if none.
*
*/
@Override
public void setContributors(final List contributors) {
this.contributors = contributors;
}
/**
* Gets the DublinCore module contributor. Convenience method that can be used to obtain the
* first item, null if none.
*
*
* @return the first DublinCore module contributor, null if none.
*/
@Override
public String getContributor() {
return Lists.firstEntry(contributors);
}
/**
* Sets the DublinCore module contributor. Convenience method that can be used when there is
* only one contributor to set.
*
*
* @param contributor the DublinCore module contributor to set, null if none.
*
*/
@Override
public void setContributor(final String contributor) {
contributors = Lists.create(contributor);
}
/**
* Returns the DublinCore module date.
*
*
* @return a list of Strings representing the DublinCore module date, an empty list if none.
*
*/
@Override
public List getDates() {
return date = Lists.createWhenNull(date);
}
/**
* Sets the DublinCore module dates.
*
*
* @param dates the list of Date representing the DublinCore module dates to set, an empty list
* or null if none.
*
*/
@Override
public void setDates(final List dates) {
date = dates;
}
/**
* Gets the DublinCore module date. Convenience method that can be used to obtain the first
* item, null if none.
*
*
* @return the first DublinCore module date, null if none.
*/
@Override
public Date getDate() {
return Lists.firstEntry(date);
}
/**
* Sets the DublinCore module date. Convenience method that can be used when there is only one
* date to set.
*
*
* @param date the DublinCore module date to set, null if none.
*
*/
@Override
public void setDate(final Date date) {
this.date = Lists.create(date);
}
/**
* Returns the DublinCore module type.
*
*
* @return a list of Strings representing the DublinCore module type, an empty list if none.
*
*/
@Override
public List getTypes() {
return type = Lists.createWhenNull(type);
}
/**
* Sets the DublinCore module types.
*
*
* @param types the list of String representing the DublinCore module types to set, an empty
* list or null if none.
*
*/
@Override
public void setTypes(final List types) {
type = types;
}
/**
* Gets the DublinCore module type. Convenience method that can be used to obtain the first
* item, null if none.
*
*
* @return the first DublinCore module type, null if none.
*/
@Override
public String getType() {
return Lists.firstEntry(type);
}
/**
* Sets the DublinCore module type. Convenience method that can be used when there is only one
* type to set.
*
*
* @param type the DublinCore module type to set, null if none.
*
*/
@Override
public void setType(final String type) {
this.type = Lists.create(type);
}
/**
* Returns the DublinCore module format.
*
*
* @return a list of Strings representing the DublinCore module format, an empty list if none.
*
*/
@Override
public List getFormats() {
return format = Lists.createWhenNull(format);
}
/**
* Sets the DublinCore module formats.
*
*
* @param formats the list of String representing the DublinCore module formats to set, an empty
* list or null if none.
*
*/
@Override
public void setFormats(final List formats) {
format = formats;
}
/**
* Gets the DublinCore module format. Convenience method that can be used to obtain the first
* item, null if none.
*
*
* @return the first DublinCore module format, null if none.
*/
@Override
public String getFormat() {
return Lists.firstEntry(format);
}
/**
* Sets the DublinCore module format. Convenience method that can be used when there is only one
* format to set.
*
*
* @param format the DublinCore module format to set, null if none.
*
*/
@Override
public void setFormat(final String format) {
this.format = Lists.create(format);
}
/**
* Returns the DublinCore module identifier.
*
*
* @return a list of Strings representing the DublinCore module identifier, an empty list if
* none.
*
*/
@Override
public List getIdentifiers() {
return identifier = Lists.createWhenNull(identifier);
}
/**
* Sets the DublinCore module identifiers.
*
*
* @param identifiers the list of String representing the DublinCore module identifiers to set,
* an empty list or null if none.
*
*/
@Override
public void setIdentifiers(final List identifiers) {
identifier = identifiers;
}
/**
* Gets the DublinCore module identifier. Convenience method that can be used to obtain the
* first item, null if none.
*
*
* @return the first DublinCore module identifier, null if none.
*/
@Override
public String getIdentifier() {
return Lists.firstEntry(identifier);
}
/**
* Sets the DublinCore module identifier. Convenience method that can be used when there is only
* one identifier to set.
*
*
* @param identifier the DublinCore module identifier to set, null if none.
*
*/
@Override
public void setIdentifier(final String identifier) {
this.identifier = Lists.create(identifier);
}
/**
* Returns the DublinCore module source.
*
*
* @return a list of Strings representing the DublinCore module source, an empty list if none.
*
*/
@Override
public List getSources() {
return source = Lists.createWhenNull(source);
}
/**
* Sets the DublinCore module sources.
*
*
* @param sources the list of String representing the DublinCore module sources to set, an empty
* list or null if none.
*
*/
@Override
public void setSources(final List sources) {
source = sources;
}
/**
* Gets the DublinCore module source. Convenience method that can be used to obtain the first
* item, null if none.
*
*
* @return the first DublinCore module source, null if none.
*/
@Override
public String getSource() {
return Lists.firstEntry(source);
}
/**
* Sets the DublinCore module source. Convenience method that can be used when there is only one
* source to set.
*
*
* @param source the DublinCore module source to set, null if none.
*
*/
@Override
public void setSource(final String source) {
this.source = Lists.create(source);
}
/**
* Returns the DublinCore module language.
*
*
* @return a list of Strings representing the DublinCore module language, an empty list if none.
*
*/
@Override
public List getLanguages() {
return language = Lists.createWhenNull(language);
}
/**
* Sets the DublinCore module languages.
*
*
* @param languages the list of String representing the DublinCore module languages to set, an
* empty list or null if none.
*
*/
@Override
public void setLanguages(final List languages) {
language = languages;
}
/**
* Gets the DublinCore module language. Convenience method that can be used to obtain the first
* item, null if none.
*
*
* @return the first DublinCore module langauge, null if none.
*/
@Override
public String getLanguage() {
return Lists.firstEntry(language);
}
/**
* Sets the DublinCore module language. Convenience method that can be used when there is only
* one language to set.
*
*
* @param language the DublinCore module language to set, null if none.
*
*/
@Override
public void setLanguage(final String language) {
this.language = Lists.create(language);
}
/**
* Returns the DublinCore module relation.
*
*
* @return a list of Strings representing the DublinCore module relation, an empty list if none.
*
*/
@Override
public List getRelations() {
return relation = Lists.createWhenNull(relation);
}
/**
* Sets the DublinCore module relations.
*
*
* @param relations the list of String representing the DublinCore module relations to set, an
* empty list or null if none.
*
*/
@Override
public void setRelations(final List relations) {
relation = relations;
}
/**
* Gets the DublinCore module relation. Convenience method that can be used to obtain the first
* item, null if none.
*
*
* @return the first DublinCore module relation, null if none.
*/
@Override
public String getRelation() {
return Lists.firstEntry(relation);
}
/**
* Sets the DublinCore module relation. Convenience method that can be used when there is only
* one relation to set.
*
*
* @param relation the DublinCore module relation to set, null if none.
*
*/
@Override
public void setRelation(final String relation) {
this.relation = Lists.create(relation);
}
/**
* Returns the DublinCore module coverage.
*
*
* @return a list of Strings representing the DublinCore module coverage, an empty list if none.
*
*/
@Override
public List getCoverages() {
return coverage = Lists.createWhenNull(coverage);
}
/**
* Sets the DublinCore module coverages.
*
*
* @param coverages the list of String representing the DublinCore module coverages to set, an
* empty list or null if none.
*
*/
@Override
public void setCoverages(final List coverages) {
coverage = coverages;
}
/**
* Gets the DublinCore module coverage. Convenience method that can be used to obtain the first
* item, null if none.
*
*
* @return the first DublinCore module coverage, null if none.
*/
@Override
public String getCoverage() {
return Lists.firstEntry(coverage);
}
/**
* Sets the DublinCore module coverage. Convenience method that can be used when there is only
* one coverage to set.
*
*
* @param coverage the DublinCore module coverage to set, null if none.
*
*/
@Override
public void setCoverage(final String coverage) {
this.coverage = Lists.create(coverage);
}
/**
* Returns the DublinCore module rights.
*
*
* @return a list of Strings representing the DublinCore module rights, an empty list if none.
*
*/
@Override
public List getRightsList() {
return rights = Lists.createWhenNull(rights);
}
/**
* Sets the DublinCore module rights.
*
*
* @param rights the list of String representing the DublinCore module rights to set, an empty
* list or null if none.
*
*/
@Override
public void setRightsList(final List rights) {
this.rights = rights;
}
/**
* Gets the DublinCore module rights. Convenience method that can be used to obtain the first
* item, null if none.
*
*
* @return the first DublinCore module rights, null if none.
*/
@Override
public String getRights() {
return Lists.firstEntry(rights);
}
/**
* Sets the DublinCore module rights. Convenience method that can be used when there is only one
* rights to set.
*
*
* @param rights the DublinCore module rights to set, null if none.
*
*/
@Override
public void setRights(final String rights) {
this.rights = Lists.create(rights);
}
/**
* Creates a deep 'bean' clone of the object.
*
*
* @return a clone of the object.
* @throws CloneNotSupportedException thrown if an element of the object cannot be cloned.
*
*/
@Override
public final Object clone() throws CloneNotSupportedException {
return CloneableBean.beanClone(this, CONVENIENCE_PROPERTIES);
}
/**
* Indicates whether some other object is "equal to" this one as defined by the Object equals()
* method.
*
*
* @param other he reference object with which to compare.
* @return true if 'this' object is equal to the 'other' object.
*
*/
@Override
public final boolean equals(final Object other) {
return EqualsBean.beanEquals(DCModule.class, this, other);
}
/**
* Returns a hashcode value for the object.
*
* It follows the contract defined by the Object hashCode() method.
*
*
* @return the hashcode of the bean object.
*
*/
@Override
public final int hashCode() {
return EqualsBean.beanHashCode(this);
}
/**
* Returns the String representation for the object.
*
*
* @return String representation for the object.
*
*/
@Override
public final String toString() {
return ToStringBean.toString(DCModule.class, this);
}
@Override
public final Class getInterface() {
return DCModule.class;
}
@Override
public final void copyFrom(final CopyFrom obj) {
COPY_FROM_HELPER.copy(this, obj);
}
private static final CopyFromHelper COPY_FROM_HELPER;
static {
final Map> basePropInterfaceMap = new HashMap>();
basePropInterfaceMap.put("titles", String.class);
basePropInterfaceMap.put("creators", String.class);
basePropInterfaceMap.put("subjects", DCSubject.class);
basePropInterfaceMap.put("descriptions", String.class);
basePropInterfaceMap.put("publishers", String.class);
basePropInterfaceMap.put("contributors", String.class);
basePropInterfaceMap.put("dates", Date.class);
basePropInterfaceMap.put("types", String.class);
basePropInterfaceMap.put("formats", String.class);
basePropInterfaceMap.put("identifiers", String.class);
basePropInterfaceMap.put("sources", String.class);
basePropInterfaceMap.put("languages", String.class);
basePropInterfaceMap.put("relations", String.class);
basePropInterfaceMap.put("coverages", String.class);
basePropInterfaceMap.put("rightsList", String.class);
final Map, Class>> basePropClassImplMap = new HashMap, Class>>();
basePropClassImplMap.put(DCSubject.class, DCSubjectImpl.class);
COPY_FROM_HELPER = new CopyFromHelper(DCModule.class, basePropInterfaceMap, basePropClassImplMap);
}
}