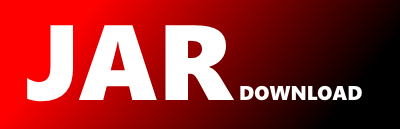
com.rometools.rome.feed.synd.SyndEnclosureImpl Maven / Gradle / Ivy
Show all versions of rome Show documentation
package com.rometools.rome.feed.synd;
import java.io.Serializable;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import com.rometools.rome.feed.CopyFrom;
import com.rometools.rome.feed.impl.CopyFromHelper;
import com.rometools.rome.feed.impl.ObjectBean;
/**
* @author Alejandro Abdelnur
*/
public class SyndEnclosureImpl implements Serializable, SyndEnclosure {
private static final long serialVersionUID = 1L;
private static final CopyFromHelper COPY_FROM_HELPER;
private final ObjectBean objBean;
private String url;
private String type;
private long length;
static {
final Map> basePropInterfaceMap = new HashMap>();
basePropInterfaceMap.put("url", String.class);
basePropInterfaceMap.put("type", String.class);
basePropInterfaceMap.put("length", Long.TYPE);
final Map, Class>> basePropClassImplMap = Collections., Class>> emptyMap();
COPY_FROM_HELPER = new CopyFromHelper(SyndEnclosure.class, basePropInterfaceMap, basePropClassImplMap);
}
/**
* Default constructor. All properties are set to null.
*
*
*/
public SyndEnclosureImpl() {
objBean = new ObjectBean(SyndEnclosure.class, this);
}
/**
* Creates a deep 'bean' clone of the object.
*
*
* @return a clone of the object.
* @throws CloneNotSupportedException thrown if an element of the object cannot be cloned.
*
*/
@Override
public Object clone() throws CloneNotSupportedException {
return objBean.clone();
}
/**
* Indicates whether some other object is "equal to" this one as defined by the Object equals()
* method.
*
*
* @param other he reference object with which to compare.
* @return true if 'this' object is equal to the 'other' object.
*
*/
@Override
public boolean equals(final Object other) {
return objBean.equals(other);
}
/**
* Returns a hashcode value for the object.
*
* It follows the contract defined by the Object hashCode() method.
*
*
* @return the hashcode of the bean object.
*
*/
@Override
public int hashCode() {
return objBean.hashCode();
}
/**
* Returns the String representation for the object.
*
*
* @return String representation for the object.
*
*/
@Override
public String toString() {
return objBean.toString();
}
/**
* Returns the enclosure URL.
*
*
* @return the enclosure URL, null if none.
*/
@Override
public String getUrl() {
return url;
}
/**
* Sets the enclosure URL.
*
*
* @param url the enclosure URL to set, null if none.
*/
@Override
public void setUrl(final String url) {
this.url = url;
}
/**
* Returns the enclosure length.
*
*
* @return the enclosure length, null if none.
*/
@Override
public long getLength() {
return length;
}
/**
* Sets the enclosure length.
*
*
* @param length the enclosure length to set, null if none.
*/
@Override
public void setLength(final long length) {
this.length = length;
}
/**
* Returns the enclosure type.
*
*
* @return the enclosure type, null if none.
*/
@Override
public String getType() {
return type;
}
/**
* Sets the enclosure type.
*
*
* @param type the enclosure type to set, null if none.
*/
@Override
public void setType(final String type) {
this.type = type;
}
@Override
public Class getInterface() {
return SyndEnclosure.class;
}
@Override
public void copyFrom(final CopyFrom obj) {
COPY_FROM_HELPER.copy(this, obj);
}
}