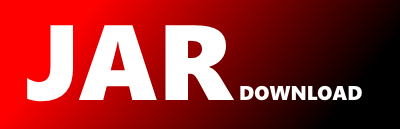
com.artemis.managers.PlayerManager Maven / Gradle / Ivy
package com.artemis.managers;
import com.artemis.Entity;
import com.badlogic.gdx.utils.Array;
import com.badlogic.gdx.utils.ObjectMap;
import com.badlogic.gdx.utils.Pool;
/**
* You may sometimes want to specify to which player an entity belongs to.
*
* An entity can only belong to a single player at a time.
*
* @author Arni Arent
*
*/
public class PlayerManager extends Manager {
private static final Array EMPTY_ENTITY_ARRAY = new Array();
protected ObjectMap playerByEntity;
protected ObjectMap> entitiesByPlayer;
protected Pool> entityArrayPool;
public PlayerManager() {
playerByEntity = new ObjectMap();
entitiesByPlayer = new ObjectMap>();
entityArrayPool = new Pool>() {
@Override
protected Array newObject() {
return new Array();
}
};
}
/**
* Adds entity to the specified player.
*
* @param e Entity that belongs to the player.
* @param player The owner of the entity.
*/
public void setPlayer(Entity e, String player) {
removeFromPlayer(e);
playerByEntity.put(e, player);
Array entities = entitiesByPlayer.get(player);
if(entities == null) {
entities = entityArrayPool.obtain();
entitiesByPlayer.put(player, entities);
}
entities.add(e);
}
/**
* Returns all entities the belongs to the player.
*
* WARNING: the array should not be modified.
*
* @param player Player to get the entities for.
* @return An array of entities belonging to the player.
*/
public Array getEntitiesOfPlayer(String player) {
Array entities = entitiesByPlayer.get(player);
if(entities == null) {
entities = EMPTY_ENTITY_ARRAY;
}
return entities;
}
/**
* Removes the specified entity from the player it belongs to.
*
* @param e Entity to disown.
*/
public void removeFromPlayer(Entity e) {
String player = playerByEntity.remove(e);
if(player != null) {
Array entities = entitiesByPlayer.get(player);
if(entities != null) {
entities.removeValue(e, true);
if (entities.size == 0) {
entityArrayPool.free(entitiesByPlayer.remove(player));
}
}
}
}
/**
* Returns the player an entity belongs to.
*
* @param e Entity to check.
* @return A player that the entity belongs to, or null if none.
*/
public String getPlayer(Entity e) {
return playerByEntity.get(e);
}
@Override
public void deleted(Entity e) {
removeFromPlayer(e);
}
@Override
public void dispose() {
playerByEntity.clear();
entitiesByPlayer.clear();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy