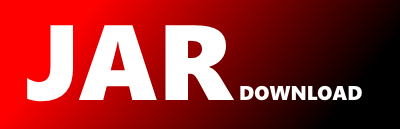
com.artemis.systems.event.BasicEventDeliverySystem Maven / Gradle / Ivy
package com.artemis.systems.event;
import com.artemis.systems.EntitySystem;
import com.badlogic.gdx.utils.Array;
import com.badlogic.gdx.utils.ObjectMap;
import com.badlogic.gdx.utils.ObjectMap.Entry;
/**
* Basic implementation of event system. All the events that are
* sent in gets put into a buffer. During the processing cycle, the
* buffered events get transfered to the currentEvents list where
* they can be consumed when interested parties call getEvents.
* CurrentEvents gets cleared during every processing cycle.
* If there are systems that need events to last longer than a single
* processing cycle, use a different EventSystem such as DedicatedQueueEventSystem.
*
* @author apotapov
*
*/
public class BasicEventDeliverySystem implements EventDeliverySystem {
/**
* Event buffer by type of event, that all new events get loaded into.
*/
protected ObjectMap, Array> buffer;
/**
* After each process cycle the events from the buffer get transferred
* to currentEvents to be consumed by the systems that call getEvents().
*/
protected ObjectMap, Array> currentEvents;
/**
* Used to generate unique event identifiers.
*/
protected int currentEventId;
/**
* Default constructor.
*/
public BasicEventDeliverySystem() {
this.buffer = new ObjectMap, Array>();
this.currentEvents = new ObjectMap, Array>();
}
/**
* Adds the specified event from the sender to the queue to be processed
* EntitySystems that care.
*/
@Override
public void postEvent(EntitySystem sender, SystemEvent event) {
synchronized (buffer) {
//update event with tracking information
event.eventId = currentEventId++;
event.sender = sender;
// get the appropriate buffer queue for the event
Class extends SystemEvent> type = event.getClass();
Array bufferQueue;
if (buffer.containsKey(type)) {
bufferQueue = buffer.get(type);
} else {
bufferQueue = new Array();
buffer.put(type, bufferQueue);
}
// add to the queue
bufferQueue.add(event);
}
}
/**
* Retrieves events of specific type and adds them to the events Set.
*/
@SuppressWarnings("unchecked")
@Override
public void getEvents(EntitySystem pollingSystem, Class type, Array events) {
if (currentEvents.containsKey(type)) {
Array currentEventsByType = currentEvents.get(type);
for (int i = 0; i < currentEventsByType.size; i++) {
SystemEvent event = currentEventsByType.get(i);
if (!event.handled) {
events.add((T)event);
}
}
}
}
@Override
public void initialize() {
}
/**
* When system is processed, transfer all events from the
* buffer to currentEvents list.
*/
@Override
public void update() {
synchronized (buffer) {
// clear out all the existing events
for (Array queue : currentEvents.values()) {
SystemEvent.free(queue);
queue.clear();
}
// transfer from buffer to current events
transferEvents(buffer, currentEvents);
// clear out the buffer
clearBuffer();
}
}
/**
* Helper method to clears the buffer.
*/
protected void clearBuffer() {
for (Array events : buffer.values()) {
events.clear();
}
}
/**
* Helper method to transer events from one object map to another.
* @param from Map to transfer from.
* @param to Map to transfer to.
*/
protected static void transferEvents(ObjectMap, Array> from,
ObjectMap, Array> to) {
for (Entry, Array> entry : from.entries()) {
// ensure that the "to" map has the required event queues
Array queue;
if (to.containsKey(entry.key)) {
queue = to.get(entry.key);
} else {
queue = new Array();
to.put(entry.key, queue);
}
queue.addAll(entry.value);
}
}
@Override
public void dispose() {
for (Array events : buffer.values()) {
SystemEvent.free(events);
events.clear();
}
buffer.clear();
for (Array events : currentEvents.values()) {
SystemEvent.free(events);
events.clear();
}
currentEvents.clear();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy