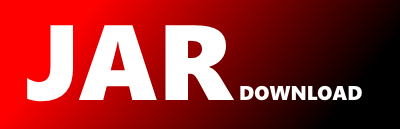
com.artemis.systems.event.EventProcessingSystem2 Maven / Gradle / Ivy
package com.artemis.systems.event;
import com.artemis.Entity;
import com.artemis.Filter;
import com.artemis.systems.EntitySystem;
import com.badlogic.gdx.utils.Array;
/**
* System for processing two events. Reduces boiler plate for event
* processing systems.
*
* @author apotapov
*
* @param First event that this system processes.
* @param Second event that this system processes.
*/
public abstract class EventProcessingSystem2 extends EntitySystem {
Array events;
Class eventType;
Array events2;
Class eventType2;
/**
* Constructs an event system
* @param filter Filter for this system.
* @param eventType Class of the first event this system will process.
* @param eventType2 Class of the second event this system will process.
*/
public EventProcessingSystem2(Filter filter, Class eventType, Class eventType2) {
super(filter);
this.eventType = eventType;
this.events = new Array();
this.eventType2 = eventType2;
this.events2 = new Array();
}
/**
* For each event and entity processes them as a pair.
*/
@Override
protected final void processEntities(Array entities) {
world.getEvents(this, eventType, events);
for (T event : events) {
for (Entity e : entities) {
processEvent(e, event);
}
}
world.getEvents(this, eventType2, events2);
for (U event : events2) {
for (Entity e : entities) {
processEvent2(e, event);
}
}
}
/**
* Processes the event with respect to the specified entity.
*
* @param e Entity to process
* @param event Event to process
*/
protected abstract void processEvent(Entity e, T event);
/**
* Processes the second event with respect to the specified entity.
*
* @param e Entity to process
* @param event Event to process
*/
protected abstract void processEvent2(Entity e, U event);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy