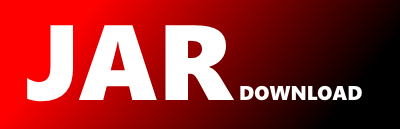
com.rustknife.jknife.cache.CacheKit Maven / Gradle / Ivy
The newest version!
/**
* Copyright (c) 2015-2017, Chill Zhuang 庄骞 ([email protected]).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.rustknife.jknife.cache;
import org.springframework.util.CollectionUtils;
import java.util.List;
/**
* 缓存工具类
*/
public class CacheKit {
public static void put(String cacheName, String key, Object value) {
if(cacheName.startsWith(AppCacheManager.getRedisPrefix()))
CacheRedisKit.set(cacheName, key, value);
else
CacheEhCacheKit.put(cacheName, key, value);
}
public static T get(String cacheName, String key) {
if(cacheName.startsWith(AppCacheManager.getRedisPrefix()))
return CacheRedisKit.get(cacheName, key);
else
return CacheEhCacheKit.get(cacheName, key);
}
@SuppressWarnings("rawtypes")
public static List getKeys(String cacheName) {
if(cacheName.startsWith(AppCacheManager.getRedisPrefix()))
return CollectionUtils.arrayToList(CacheRedisKit.keys(cacheName, null));
else
return CacheEhCacheKit.getKeys(cacheName);
}
public static void remove(String cacheName, Object key) {
if(cacheName.startsWith(AppCacheManager.getRedisPrefix()))
CacheRedisKit.del(cacheName, key.toString());
else
CacheEhCacheKit.remove(cacheName, key);
}
public static void removeAll(String cacheName) {
if(cacheName.startsWith(AppCacheManager.getRedisPrefix()))
CacheRedisKit.delByRowKeys(CacheRedisKit.keysRaw(cacheName, null));
else
CacheEhCacheKit.removeAll(cacheName);
}
public static void removeLike(String cacheName, String keyLike){
if(cacheName.startsWith(AppCacheManager.getRedisPrefix())){
CacheRedisKit.delByRowKeys(CacheRedisKit.keysRaw(cacheName, "*" + keyLike + "*"));
}
else{
@SuppressWarnings("unchecked") List keys = CacheEhCacheKit.getKeys(cacheName);
for(String key : keys) {
if(key.contains(keyLike))
CacheEhCacheKit.remove(cacheName, key);
}
}
}
public static void removeStartLike(String cacheName, String keyStartLike){
if(cacheName.startsWith(AppCacheManager.getRedisPrefix())){
CacheRedisKit.delByRowKeys(CacheRedisKit.keysRaw(cacheName, keyStartLike + "*"));
}
else{
@SuppressWarnings("unchecked") List keys = CacheEhCacheKit.getKeys(cacheName);
for(String key : keys) {
if(key.startsWith(keyStartLike))
CacheEhCacheKit.remove(cacheName, key);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy