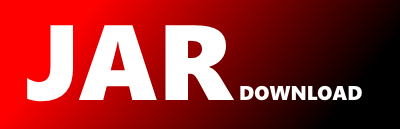
com.safecharge.request.AddUPOAPMRequest Maven / Gradle / Ivy
Show all versions of safecharge-sdk-java Show documentation
package com.safecharge.request;
import java.util.HashMap;
import java.util.Map;
import javax.validation.ConstraintViolationException;
import javax.validation.constraints.NotNull;
import javax.validation.constraints.Size;
import com.safecharge.model.UserDetails;
import com.safecharge.model.UserDetailsCashier;
import com.safecharge.request.builder.SafechargeBuilder;
import com.safecharge.util.AddressUtils;
import com.safecharge.util.Constants;
import com.safecharge.util.ValidChecksum;
import com.safecharge.util.ValidationUtils;
/**
* Copyright (C) 2007-2017 SafeCharge International Group Limited.
*
* Request to add APM User Payment Option to a User.
*
* The goal of this request is to add an APM UPO account for specific users according to their User Token ID.
* Once an APM UPO is added to a consumer’s UPO list, the APM is displayed in the user’s available payment options on the payment page.
*
* @author Nikola Dichev
* @see AddUPOCreditCardByTempTokenRequest
* @see AddUPOCreditCardRequest
* @see com.safecharge.response.AddUPOAPMResponse
* @since 3/21/2017
*/
@ValidChecksum(orderMappingName = Constants.ChecksumOrderMapping.ADD_CASHIER_APM)
public class AddUPOAPMRequest extends SafechargeRequest {
/**
* The unique name of the payment method in Checkout Page system (for example apmgw_Neteller). For a list of possible values, see APM Unique SafeCharge Checkout Names.
*/
@NotNull(message = "paymentMethodName parameter is mandatory!")
private String paymentMethodName;
/**
* A list of name-value pairs that contain the parameters of the user payment option.
*/
@NotNull(message = "apmData parameter is mandatory!")
@Size(min = 1, message = "apmData must have at least one entry")
private Map apmData;
/**
* This parameter is a unique identifier for each customer generated by you.
*/
@NotNull(message = "userTokenId parameter is mandatory!")
private String userTokenId;
/**
* Billing address related to a user payment option. Since order can contain only one payment option billing address is part of the order parameters.
*/
private UserDetailsCashier billingAddress;
public static Builder builder() {
return new Builder();
}
public String getPaymentMethodName() {
return paymentMethodName;
}
public void setPaymentMethodName(String paymentMethodName) {
this.paymentMethodName = paymentMethodName;
}
public Map getApmData() {
return apmData;
}
public void setApmData(Map apmData) {
this.apmData = apmData;
}
public String getUserTokenId() {
return userTokenId;
}
public void setUserTokenId(String userTokenId) {
this.userTokenId = userTokenId;
}
public UserDetailsCashier getBillingAddress() {
return billingAddress;
}
public void setBillingAddress(UserDetailsCashier billingAddress) {
this.billingAddress = billingAddress;
}
@Override
public String toString() {
final StringBuilder sb = new StringBuilder("AddUPOAPM{");
sb.append("billingAddress=")
.append(billingAddress);
sb.append(", paymentMethodName='")
.append(paymentMethodName)
.append('\'');
sb.append(", apmData=")
.append(apmData);
sb.append(", userTokenId='")
.append(userTokenId)
.append('\'');
sb.append(", ")
.append(super.toString());
sb.append('}');
return sb.toString();
}
public static class Builder extends SafechargeBuilder {
UserDetailsCashier billingAddress;
private String paymentMethodName;
private Map apmData;
@NotNull
private String userTokenId;
/**
* Adds the payment method name to the request.
*
* @param paymentMethodName The unique name of the payment method in Checkout Page system.
* @return this object
* @see APM account identifiers
*/
public Builder addPaymentMethodName(String paymentMethodName) {
this.paymentMethodName = paymentMethodName;
return this;
}
/**
* Adds user token id to the request.
*
* @param userTokenId The user token as {@link String}
* @return this object
*/
public Builder addUserTokenId(String userTokenId) {
this.userTokenId = userTokenId;
return this;
}
/**
* Adds map from APM specific data to the request. E.g. username -> user1111, password -> p4ssw0rd
*
* @param apmData map of APM specific key-value data
* @return this object
*/
public Builder addApmData(Map apmData) {
this.apmData = apmData;
return this;
}
/**
* Adds one key-value pair of APM specific data to the request.
*
* @param key {@link String} key. E.g. "username"
* @param value {@link String} value to assign to the {@code key}. E.g. "user1111"
* @return this object
*/
public Builder addApmDataEntry(String key, String value) {
if (apmData == null) {
apmData = new HashMap<>();
}
apmData.put(key, value);
return this;
}
/**
* Adds billing address data to the request.
*
* @param billingAddress {@link UserDetails} object to get the billing details from
* @return this object
*/
public Builder addBillingAddress(UserDetailsCashier billingAddress) {
this.billingAddress = billingAddress;
return this;
}
/**
* Adds billing address data to the request.
*
* @param firstName The first name of the recipient
* @param lastName The last name of the recipient
* @param address The address of the recipient
* @param phone The phone number of the recipient
* @param zip The postal code of the recipient
* @param city The city of the recipient
* @param countryCode The country of the recipient(two-letter ISO country code)
* @param state The state of the recipient(two-letter ISO state code)
* @param email The email of the recipient
* @param birthdate The date of birth of the recipient
* @param locale The date of birth of the recipient
* @return this object
*/
public Builder addBillingAddress(String address, String city, String countryCode, String email, String firstName,
String lastName, String phone, String state, String zip, String birthdate,
String county, String locale) {
UserDetailsCashier billingAddress = AddressUtils.createUserDetailsCashierFromParams(address, city, countryCode, email, firstName,
lastName, phone, state, zip, birthdate, county, locale);
return addBillingAddress(billingAddress);
}
/**
* Builds the request.
*
* @return {@link SafechargeRequest} object build from the params set by this builder
* @throws ConstraintViolationException if the validation of the params fails
*/
@Override
public SafechargeBaseRequest build() throws ConstraintViolationException {
AddUPOAPMRequest addUPOAPM = new AddUPOAPMRequest();
addUPOAPM.setPaymentMethodName(paymentMethodName);
addUPOAPM.setUserTokenId(userTokenId);
addUPOAPM.setApmData(apmData);
addUPOAPM.setBillingAddress(billingAddress);
return ValidationUtils.validate(super.build(addUPOAPM));
}
}
}