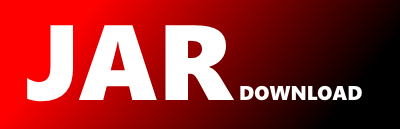
com.sailthru.client.params.query.Query Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sailthru-java-client Show documentation
Show all versions of sailthru-java-client Show documentation
Java client library for Sailthru API
package com.sailthru.client.params.query;
import java.util.ArrayList;
import com.google.gson.Gson;
import com.sailthru.client.SailthruUtil;
import com.sailthru.client.handler.JsonHandler;
import java.util.HashMap;
import java.util.Map;
/**
* Map query builder parameters for API calls
* @author Prajwal Tuladhar
*/
public class Query {
protected String source_list;
protected QueryMode query_mode;
protected java.util.List criteria;
protected java.util.List value;
protected java.util.List engagement;
protected java.util.List geo_frequency;
protected java.util.List threshold;
protected java.util.List timerange;
protected java.util.List field;
public Query() {
this.criteria = new ArrayList();
this.value = new ArrayList();
this.engagement = new ArrayList();
this.geo_frequency = new ArrayList();
this.threshold = new ArrayList();
this.timerange = new ArrayList();
this.field = new ArrayList();
}
public Query setSourceList(String list) {
this.source_list = list;
return this;
}
public Query addCriteria(Criteria criteria) {
this.criteria.add(criteria.toString());
return this;
}
public Query addValue(String value) {
this.value.add(value);
return this;
}
public Query addEngagement(int level) {
this.engagement.add(level);
return this;
}
public Query addGeoFrequency(int geoFrequency) {
this.geo_frequency.add(geoFrequency);
return this;
}
public Query addThreshold(int threshold) {
this.threshold.add(threshold);
return this;
}
public Query addTimeRange(TimeRange timeRange) {
this.timerange.add(timeRange.toString());
return this;
}
public Map toHashMap() {
Gson gson = SailthruUtil.createGson();
String json = gson.toJson(this);
JsonHandler handler = new JsonHandler();
return (Map)handler.parseResponse(json);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy