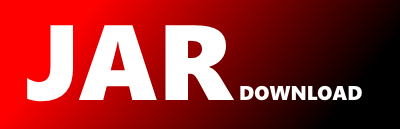
com.sailthru.client.AbstractSailthruClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sailthru-java-client Show documentation
Show all versions of sailthru-java-client Show documentation
Java client library for Sailthru API
The newest version!
package com.sailthru.client;
import com.google.common.annotations.VisibleForTesting;
import com.google.gson.Gson;
import com.google.gson.reflect.TypeToken;
import com.sailthru.client.handler.JsonHandler;
import com.sailthru.client.handler.SailthruResponseHandler;
import com.sailthru.client.handler.response.JsonResponse;
import com.sailthru.client.http.SailthruHandler;
import com.sailthru.client.http.SailthruHttpClient;
import com.sailthru.client.params.ApiFileParams;
import com.sailthru.client.params.ApiParams;
import java.io.IOException;
import java.lang.reflect.Type;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import org.apache.http.HttpVersion;
import org.apache.http.conn.scheme.PlainSocketFactory;
import org.apache.http.conn.scheme.Scheme;
import org.apache.http.conn.scheme.SchemeRegistry;
import org.apache.http.conn.ssl.SSLSocketFactory;
import org.apache.http.impl.conn.tsccm.ThreadSafeClientConnManager;
import org.apache.http.params.BasicHttpParams;
import org.apache.http.params.HttpConnectionParams;
import org.apache.http.params.HttpParams;
import org.apache.http.params.HttpProtocolParams;
/**
* Abstract class exposing generic API calls for Sailthru API as per http://docs.sailthru.com/api
* @author Prajwal Tuladhar
*/
public abstract class AbstractSailthruClient {
private static final Gson GSON = SailthruUtil.createGson();
public static final String DEFAULT_API_URL = "https://api.sailthru.com";
public static final int DEFAULT_HTTP_PORT = 80;
public static final int DEFAULT_HTTPS_PORT = 443;
public static final String DEFAULT_USER_AGENT = "Sailthru Java Client";
public static final String DEFAULT_ENCODING = "UTF-8";
//HTTP methods supported by Sailthru API
public static enum HttpRequestMethod {
GET,
POST,
DELETE
}
protected String apiKey;
protected String apiSecret;
protected String apiUrl;
protected SailthruHttpClient httpClient;
private SailthruHandler handler;
private Map customHeaders = null;
private final SailthruHttpClientConfiguration sailthruHttpClientConfiguration;
private final Map lastRateLimitInfoMap = new HashMap();
/**
* Main constructor class for setting up the client
* @param apiKey
* @param apiSecret
* @param apiUrl
*/
public AbstractSailthruClient(String apiKey, String apiSecret, String apiUrl) {
this(apiKey, apiSecret, apiUrl, new DefaultSailthruHttpClientConfiguration());
}
/**
*
* @param apiKey
* @param apiSecret
* @param apiUrl
* @param sailthruHttpClientConfiguration
*/
public AbstractSailthruClient(String apiKey, String apiSecret, String apiUrl, SailthruHttpClientConfiguration sailthruHttpClientConfiguration) {
this.apiKey = apiKey;
this.apiSecret = apiSecret;
this.apiUrl = apiUrl;
handler = new SailthruHandler(new JsonHandler());
this.sailthruHttpClientConfiguration = sailthruHttpClientConfiguration;
httpClient = create();
}
/**
* Create SailthruHttpClient
*/
private SailthruHttpClient create() {
HttpParams params = new BasicHttpParams();
HttpProtocolParams.setVersion(params, HttpVersion.HTTP_1_1);
HttpProtocolParams.setContentCharset(params, DEFAULT_ENCODING);
HttpProtocolParams.setUserAgent(params, DEFAULT_USER_AGENT);
HttpProtocolParams.setUseExpectContinue(params, true);
HttpConnectionParams.setConnectionTimeout(params, sailthruHttpClientConfiguration.getConnectionTimeout());
HttpConnectionParams.setSoTimeout(params, sailthruHttpClientConfiguration.getSoTimeout());
HttpConnectionParams.setSoReuseaddr(params, sailthruHttpClientConfiguration.getSoReuseaddr());
HttpConnectionParams.setTcpNoDelay(params, sailthruHttpClientConfiguration.getTcpNoDelay());
SchemeRegistry schemeRegistry = new SchemeRegistry();
schemeRegistry.register(getScheme());
ThreadSafeClientConnManager connManager = new ThreadSafeClientConnManager(schemeRegistry);
connManager.setMaxTotal(sailthruHttpClientConfiguration.getMaxTotalConnections());
connManager.setDefaultMaxPerRoute(sailthruHttpClientConfiguration.getDefaultMaxConnectionsPerRoute());
return new SailthruHttpClient(connManager, params);
}
/**
* Getter for SailthruHttpClient
*/
public SailthruHttpClient getSailthruHttpClient() {
return httpClient;
}
/**
* Get Scheme Object
*/
protected Scheme getScheme() {
String scheme;
try {
URI uri = new URI(this.apiUrl);
scheme = uri.getScheme();
} catch (URISyntaxException e) {
scheme = "http";
}
if (scheme.equals("https")) {
return new Scheme(scheme, DEFAULT_HTTPS_PORT, SSLSocketFactory.getSocketFactory());
} else {
return new Scheme(scheme, DEFAULT_HTTP_PORT, PlainSocketFactory.getSocketFactory());
}
}
/**
* Make Http request to Sailthru API for given resource with given method and data
* @param action
* @param method
* @param data parameter data
* @return Object
* @throws IOException
*/
protected Object httpRequest(ApiAction action, HttpRequestMethod method, Map data) throws IOException {
String url = this.apiUrl + "/" + action.toString().toLowerCase();
Type type = new TypeToken
© 2015 - 2025 Weber Informatics LLC | Privacy Policy