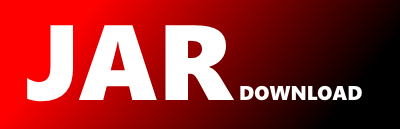
com.sailthru.client.http.SailthruHttpClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sailthru-java-client Show documentation
Show all versions of sailthru-java-client Show documentation
Java client library for Sailthru API
The newest version!
package com.sailthru.client.http;
import com.sailthru.client.AbstractSailthruClient.HttpRequestMethod;
import com.sailthru.client.SailthruClient;
import org.apache.http.NameValuePair;
import org.apache.http.client.ResponseHandler;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpDelete;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.client.methods.HttpUriRequest;
import org.apache.http.client.utils.URLEncodedUtils;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.mime.HttpMultipartMode;
import org.apache.http.entity.mime.MultipartEntityBuilder;
import org.apache.http.impl.client.DefaultHttpClient;
import org.apache.http.impl.conn.tsccm.ThreadSafeClientConnManager;
import org.apache.http.message.BasicNameValuePair;
import org.apache.http.params.HttpParams;
import java.io.ByteArrayInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.InputStream;
import java.io.UnsupportedEncodingException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
public class SailthruHttpClient extends DefaultHttpClient {
public SailthruHttpClient(ThreadSafeClientConnManager connManager,
HttpParams params) {
super(connManager, params);
}
private HttpUriRequest buildRequest(String urlString, HttpRequestMethod method, Map queryParams) throws UnsupportedEncodingException {
List nameValuePairs = new ArrayList();
for (Entry entry : queryParams.entrySet()) {
nameValuePairs.add(new BasicNameValuePair(entry.getKey(), entry.getValue()));
}
switch(method) {
case GET:
return new HttpGet(urlString + "?" + extractQueryString(nameValuePairs));
case POST:
HttpPost httpPost = new HttpPost(urlString);
httpPost.setEntity(new UrlEncodedFormEntity(nameValuePairs, SailthruClient.DEFAULT_ENCODING));
return httpPost;
case DELETE:
return new HttpDelete(urlString + "?" + extractQueryString(nameValuePairs));
}
return null;
}
private HttpUriRequest buildRequest(String urlString, HttpRequestMethod method, Map queryParams, Map files)
throws FileNotFoundException {
Map fileObj = new HashMap(files);
return buildRequest2(urlString, method, queryParams, fileObj);
}
private HttpUriRequest buildRequest2(String urlString, HttpRequestMethod method, Map queryParams, Map files)
throws FileNotFoundException {
List nameValuePairs = new ArrayList();
for( Entry entry : queryParams.entrySet() ) {
nameValuePairs.add(new BasicNameValuePair(entry.getKey(), entry.getValue()));
}
switch(method) {
case GET:
return new HttpGet(urlString + "?" + extractQueryString(nameValuePairs));
case POST:
HttpPost httpPost = new HttpPost(urlString);
MultipartEntityBuilder builder = MultipartEntityBuilder.create();
builder.setMode(HttpMultipartMode.BROWSER_COMPATIBLE);
for (Entry entry : queryParams.entrySet()) {
builder.addTextBody(entry.getKey(), entry.getValue());
}
for (Entry fileObjectEntry : files.entrySet()) {
File file;
InputStream inputStream;
String fileObjKey = fileObjectEntry.getKey();
Object fileObjValue = fileObjectEntry.getValue();
String filename;
// Object should either be File or InputStream
if (fileObjValue instanceof File) {
file = (File) fileObjValue;
filename = file.getName();
inputStream = new FileInputStream(file);
} else if (fileObjValue instanceof InputStream) {
filename = "import_job_data_" + fileObjKey + ".csv";
inputStream = (InputStream) fileObjValue;
} else {
throw new IllegalArgumentException("File param value should be of File or an InputStream type");
}
builder.addBinaryBody(fileObjKey, inputStream, ContentType.APPLICATION_OCTET_STREAM, filename);
}
httpPost.setEntity(builder.build());
return httpPost;
case DELETE:
return new HttpDelete(urlString + "?" + extractQueryString(nameValuePairs));
}
return null;
}
public Object executeHttpRequest(String urlString, HttpRequestMethod method, Map params, ResponseHandler
© 2015 - 2025 Weber Informatics LLC | Privacy Policy