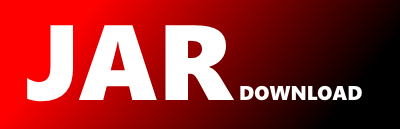
com.sailthru.client.params.Email Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sailthru-java-client Show documentation
Show all versions of sailthru-java-client Show documentation
Java client library for Sailthru API
The newest version!
package com.sailthru.client.params;
import com.google.gson.reflect.TypeToken;
import com.sailthru.client.ApiAction;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Map;
/**
*
* @author Prajwal Tuladhar
*/
public class Email extends AbstractApiParams implements ApiParams {
protected String email;
protected Integer verified;
protected String optout;
protected Map lists;
protected Map templates;
protected String send;
protected Map send_vars;
protected Map vars;
public Email() {
this.vars = new HashMap();
this.send_vars = new HashMap();
this.lists = new HashMap();
this.templates = new HashMap();
}
public Email setEmail(String email) {
this.email = email;
return this;
}
public Email setVerified(Integer verified) {
this.verified = verified;
return this;
}
public Email setOptout(String optout) {
this.optout = optout;
return this;
}
public Email setLists(java.util.List lists) {
this.lists.clear();
for (String list : lists) {
this.lists.put(list, 1);
}
return this;
}
public Email setLists(Map lists) {
this.lists.clear();
for (Map.Entry entry : lists.entrySet()) {
String list = entry.getKey();
int value = entry.getValue() != 0 ? 1 : 0;
this.lists.put(list, value);
}
return this;
}
public Email setTemplates(java.util.List templates) {
for (String template : templates) {
this.templates.put(template, 1);
}
return this;
}
public Email setTemplates(Map templates) {
this.templates = templates;
return this;
}
public Email setSend(String template) {
this.send = template;
return this;
}
public Email setSendVars(Map sendVars) {
this.send_vars = sendVars;
return this;
}
@SuppressWarnings("unchecked")
public Email setVars(Map vars) {
this.vars = vars;
return this;
}
public Email setTextOnly() {
this.vars.put("text_only", 1);
return this;
}
public Type getType() {
Type type = new TypeToken() {}.getType();
return type;
}
@Override
public ApiAction getApiCall() {
return ApiAction.email;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy