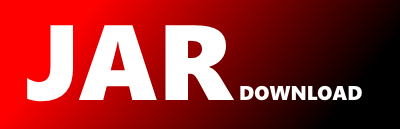
com.salesforce.jgrapht.alg.DijkstraShortestPath Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of AptSpringProcessor Show documentation
Show all versions of AptSpringProcessor Show documentation
This project contains the apt processor that implements all the checks enumerated in @Verify. It is a self contained, and
shaded jar.
/*
* (C) Copyright 2003-2017, by John V Sichi and Contributors.
*
* JGraphT : a free Java graph-theory library
*
* This program and the accompanying materials are dual-licensed under
* either
*
* (a) the terms of the GNU Lesser General Public License version 2.1
* as published by the Free Software Foundation, or (at your option) any
* later version.
*
* or (per the licensee's choosing)
*
* (b) the terms of the Eclipse Public License v1.0 as published by
* the Eclipse Foundation.
*/
package com.salesforce.jgrapht.alg;
import java.util.*;
import com.salesforce.jgrapht.*;
import com.salesforce.jgrapht.graph.*;
import com.salesforce.jgrapht.traverse.*;
/**
* An implementation of Dijkstra's
* shortest path algorithm using ClosestFirstIterator
.
*
* @param the graph vertex type
* @param the graph edge type
*
* @author John V. Sichi
* @since Sep 2, 2003
* @deprecated in favor of {@link com.salesforce.jgrapht.alg.shortestpath.DijkstraShortestPath}
*/
@Deprecated
public final class DijkstraShortestPath
{
private GraphPath path;
/**
* Creates and executes a new DijkstraShortestPath algorithm instance. An instance is only good
* for a single search; after construction, it can be accessed to retrieve information about the
* path found.
*
* @param graph the graph to be searched
* @param startVertex the vertex at which the path should start
* @param endVertex the vertex at which the path should end
*/
public DijkstraShortestPath(Graph graph, V startVertex, V endVertex)
{
this(graph, startVertex, endVertex, Double.POSITIVE_INFINITY);
}
/**
* Creates and executes a new DijkstraShortestPath algorithm instance. An instance is only good
* for a single search; after construction, it can be accessed to retrieve information about the
* path found.
*
* @param graph the graph to be searched
* @param startVertex the vertex at which the path should start
* @param endVertex the vertex at which the path should end
* @param radius limit on weighted path length, or Double.POSITIVE_INFINITY for unbounded search
*/
public DijkstraShortestPath(Graph graph, V startVertex, V endVertex, double radius)
{
if (!graph.containsVertex(endVertex)) {
throw new IllegalArgumentException("graph must contain the end vertex");
}
ClosestFirstIterator iter = new ClosestFirstIterator<>(graph, startVertex, radius);
while (iter.hasNext()) {
V vertex = iter.next();
if (vertex.equals(endVertex)) {
createEdgeList(graph, iter, startVertex, endVertex);
return;
}
}
path = null;
}
/**
* Return the edges making up the path found.
*
* @return List of Edges, or null if no path exists
*/
public List getPathEdgeList()
{
if (path == null) {
return null;
} else {
return path.getEdgeList();
}
}
/**
* Return the path found.
*
* @return path representation, or null if no path exists
*/
public GraphPath getPath()
{
return path;
}
/**
* Return the weighted length of the path found.
*
* @return path length, or Double.POSITIVE_INFINITY if no path exists
*/
public double getPathLength()
{
if (path == null) {
return Double.POSITIVE_INFINITY;
} else {
return path.getWeight();
}
}
/**
* Convenience method to find the shortest path via a single static method call. If you need a
* more advanced search (e.g. limited by radius, or computation of the path length), use the
* constructor instead.
*
* @param graph the graph to be searched
* @param startVertex the vertex at which the path should start
* @param endVertex the vertex at which the path should end
*
* @param the graph vertex type
* @param the graph edge type
*
* @return List of Edges, or null if no path exists
*/
public static List findPathBetween(Graph graph, V startVertex, V endVertex)
{
DijkstraShortestPath alg = new DijkstraShortestPath<>(graph, startVertex, endVertex);
return alg.getPathEdgeList();
}
private void createEdgeList(
Graph graph, ClosestFirstIterator iter, V startVertex, V endVertex)
{
List edgeList = new ArrayList<>();
List vertexList = new ArrayList<>();
vertexList.add(endVertex);
V v = endVertex;
while (true) {
E edge = iter.getSpanningTreeEdge(v);
if (edge == null) {
break;
}
edgeList.add(edge);
v = Graphs.getOppositeVertex(graph, edge, v);
vertexList.add(v);
}
Collections.reverse(edgeList);
Collections.reverse(vertexList);
double pathLength = iter.getShortestPathLength(endVertex);
path = new GraphWalk<>(graph, startVertex, endVertex, vertexList, edgeList, pathLength);
}
}
// End DijkstraShortestPath.java
© 2015 - 2025 Weber Informatics LLC | Privacy Policy