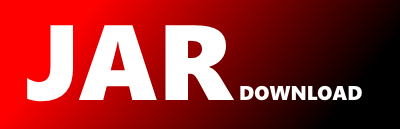
com.salesforce.jgrapht.graph.DirectedMaskSubgraph Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of AptSpringProcessor Show documentation
Show all versions of AptSpringProcessor Show documentation
This project contains the apt processor that implements all the checks enumerated in @Verify. It is a self contained, and
shaded jar.
/*
* (C) Copyright 2007-2017, by France Telecom and Contributors.
*
* JGraphT : a free Java graph-theory library
*
* This program and the accompanying materials are dual-licensed under
* either
*
* (a) the terms of the GNU Lesser General Public License version 2.1
* as published by the Free Software Foundation, or (at your option) any
* later version.
*
* or (per the licensee's choosing)
*
* (b) the terms of the Eclipse Public License v1.0 as published by
* the Eclipse Foundation.
*/
package com.salesforce.jgrapht.graph;
import java.util.*;
import java.util.function.*;
import com.salesforce.jgrapht.*;
/**
* A directed graph that is a {@link MaskSubgraph} of another graph.
*
* @param the graph vertex type
* @param the graph edge type
*
* @author Guillaume Boulmier
* @since July 5, 2007
*/
public class DirectedMaskSubgraph
extends MaskSubgraph
implements DirectedGraph
{
/**
* Create a new directed {@link MaskSubgraph} of another graph.
*
* @param base the base graph
* @param mask vertices and edges to exclude in the subgraph. If a vertex/edge is masked, it is
* as if it is not in the subgraph.
* @deprecated in favor of using lambdas
*/
@Deprecated
public DirectedMaskSubgraph(DirectedGraph base, MaskFunctor mask)
{
super(base, mask);
}
/**
* Create a new directed {@link MaskSubgraph} of another graph.
*
* @param base the base graph
* @param vertexMask vertices to exclude in the subgraph. If a vertex is masked, it is as if it
* is not in the subgraph. Edges incident to the masked vertex are also masked.
* @param edgeMask edges to exclude in the subgraph. If an edge is masked, it is as if it is not
* in the subgraph.
*/
public DirectedMaskSubgraph(
DirectedGraph base, Predicate vertexMask, Predicate edgeMask)
{
super(base, vertexMask, edgeMask);
}
/**
* {@inheritDoc}
*/
@Override
public int inDegreeOf(V vertex)
{
return incomingEdgesOf(vertex).size();
}
/**
* {@inheritDoc}
*/
@Override
public Set incomingEdgesOf(V vertex)
{
assertVertexExist(vertex);
return new MaskEdgeSet<>(
base, ((DirectedGraph) base).incomingEdgesOf(vertex), vertexMask, edgeMask);
}
/**
* {@inheritDoc}
*/
@Override
public int outDegreeOf(V vertex)
{
return outgoingEdgesOf(vertex).size();
}
/**
* {@inheritDoc}
*/
@Override
public Set outgoingEdgesOf(V vertex)
{
assertVertexExist(vertex);
return new MaskEdgeSet<>(
base, ((DirectedGraph) base).outgoingEdgesOf(vertex), vertexMask, edgeMask);
}
}
// End DirectedMaskSubgraph.java
© 2015 - 2025 Weber Informatics LLC | Privacy Policy