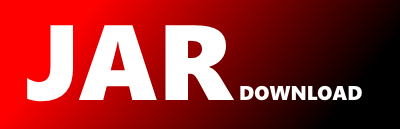
com.salesforce.jgrapht.graph.AsUnmodifiableGraph Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of AptSpringProcessor Show documentation
Show all versions of AptSpringProcessor Show documentation
This project contains the apt processor that implements all the checks enumerated in @Verify. It is a self contained, and
shaded jar.
The newest version!
/*
* (C) Copyright 2003-2018, by Barak Naveh and Contributors.
*
* JGraphT : a free Java graph-theory library
*
* See the CONTRIBUTORS.md file distributed with this work for additional
* information regarding copyright ownership.
*
* This program and the accompanying materials are made available under the
* terms of the Eclipse Public License 2.0 which is available at
* http://www.eclipse.org/legal/epl-2.0, or the
* GNU Lesser General Public License v2.1 or later
* which is available at
* http://www.gnu.org/licenses/old-licenses/lgpl-2.1-standalone.html.
*
* SPDX-License-Identifier: EPL-2.0 OR LGPL-2.1-or-later
*/
package com.salesforce.jgrapht.graph;
import com.salesforce.jgrapht.*;
import java.io.*;
import java.util.*;
/**
* An unmodifiable view of the backing graph specified in the constructor. This graph allows modules
* to provide users with "read-only" access to internal graphs. Query operations on this graph "read
* through" to the backing graph, and attempts to modify this graph result in an
* UnsupportedOperationException
.
*
*
* This graph does not pass the hashCode and equals operations through to the backing graph,
* but relies on Object's equals and hashCode methods. This graph will be
* serializable if the backing graph is serializable.
*
*
* @param the graph vertex type
* @param the graph edge type
*
* @author Barak Naveh
*/
public class AsUnmodifiableGraph
extends
GraphDelegator
implements
Serializable
{
private static final long serialVersionUID = -8186686968362705760L;
private static final String UNMODIFIABLE = "this graph is unmodifiable";
/**
* Creates a new unmodifiable graph based on the specified backing graph.
*
* @param g the backing graph on which an unmodifiable graph is to be created.
*/
public AsUnmodifiableGraph(Graph g)
{
super(g);
}
/**
* @see Graph#addEdge(Object, Object)
*/
@Override
public E addEdge(V sourceVertex, V targetVertex)
{
throw new UnsupportedOperationException(UNMODIFIABLE);
}
/**
* @see Graph#addEdge(Object, Object, Object)
*/
@Override
public boolean addEdge(V sourceVertex, V targetVertex, E e)
{
throw new UnsupportedOperationException(UNMODIFIABLE);
}
/**
* @see Graph#addVertex(Object)
*/
@Override
public boolean addVertex(V v)
{
throw new UnsupportedOperationException(UNMODIFIABLE);
}
/**
* @see Graph#removeAllEdges(Collection)
*/
@Override
public boolean removeAllEdges(Collection extends E> edges)
{
throw new UnsupportedOperationException(UNMODIFIABLE);
}
/**
* @see Graph#removeAllEdges(Object, Object)
*/
@Override
public Set removeAllEdges(V sourceVertex, V targetVertex)
{
throw new UnsupportedOperationException(UNMODIFIABLE);
}
/**
* @see Graph#removeAllVertices(Collection)
*/
@Override
public boolean removeAllVertices(Collection extends V> vertices)
{
throw new UnsupportedOperationException(UNMODIFIABLE);
}
/**
* @see Graph#removeEdge(Object)
*/
@Override
public boolean removeEdge(E e)
{
throw new UnsupportedOperationException(UNMODIFIABLE);
}
/**
* @see Graph#removeEdge(Object, Object)
*/
@Override
public E removeEdge(V sourceVertex, V targetVertex)
{
throw new UnsupportedOperationException(UNMODIFIABLE);
}
/**
* @see Graph#removeVertex(Object)
*/
@Override
public boolean removeVertex(V v)
{
throw new UnsupportedOperationException(UNMODIFIABLE);
}
/**
* {@inheritDoc}
*/
@Override
public GraphType getType()
{
return super.getType().asUnmodifiable();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy