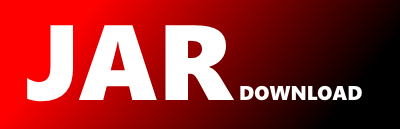
com.salesforce.jgrapht.graph.BaseIntrusiveEdgesSpecifics Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of AptSpringProcessor Show documentation
Show all versions of AptSpringProcessor Show documentation
This project contains the apt processor that implements all the checks enumerated in @Verify. It is a self contained, and
shaded jar.
The newest version!
/*
* (C) Copyright 2003-2018, by Barak Naveh and Contributors.
*
* JGraphT : a free Java graph-theory library
*
* See the CONTRIBUTORS.md file distributed with this work for additional
* information regarding copyright ownership.
*
* This program and the accompanying materials are made available under the
* terms of the Eclipse Public License 2.0 which is available at
* http://www.eclipse.org/legal/epl-2.0, or the
* GNU Lesser General Public License v2.1 or later
* which is available at
* http://www.gnu.org/licenses/old-licenses/lgpl-2.1-standalone.html.
*
* SPDX-License-Identifier: EPL-2.0 OR LGPL-2.1-or-later
*/
package com.salesforce.jgrapht.graph;
import com.salesforce.jgrapht.*;
import com.salesforce.jgrapht.util.*;
import java.io.*;
import java.util.*;
/**
* A base implementation for the intrusive edges specifics.
*
* @author Barak Naveh
* @author Dimitrios Michail
*
* @param the graph vertex type
* @param the graph edge type
* @param the intrusive edge type
*/
public abstract class BaseIntrusiveEdgesSpecifics
implements
Serializable
{
private static final long serialVersionUID = -7498268216742485L;
protected Map edgeMap;
protected transient Set unmodifiableEdgeSet = null;
/**
* Constructor
*
* @param edgeMap the map to use for storage
*/
public BaseIntrusiveEdgesSpecifics(Map edgeMap)
{
this.edgeMap = Objects.requireNonNull(edgeMap);
}
/**
* Check if an edge exists
*
* @param e the edge
* @return true if the edge exists, false otherwise
*/
public boolean containsEdge(E e)
{
return edgeMap.containsKey(e);
}
/**
* Get the edge set.
*
* @return an unmodifiable edge set
*/
public Set getEdgeSet()
{
if (unmodifiableEdgeSet == null) {
unmodifiableEdgeSet = Collections.unmodifiableSet(edgeMap.keySet());
}
return unmodifiableEdgeSet;
}
/**
* Remove an edge.
*
* @param e the edge
*/
public void remove(E e)
{
edgeMap.remove(e);
}
/**
* Get the source of an edge.
*
* @param e the edge
* @return the source vertex of an edge
*/
public V getEdgeSource(E e)
{
IntrusiveEdge ie = getIntrusiveEdge(e);
if (ie == null) {
throw new IllegalArgumentException("no such edge in graph: " + e.toString());
}
return TypeUtil.uncheckedCast(ie.source);
}
/**
* Get the target of an edge.
*
* @param e the edge
* @return the target vertex of an edge
*/
public V getEdgeTarget(E e)
{
IntrusiveEdge ie = getIntrusiveEdge(e);
if (ie == null) {
throw new IllegalArgumentException("no such edge in graph: " + e.toString());
}
return TypeUtil.uncheckedCast(ie.target);
}
/**
* Get the weight of an edge.
*
* @param e the edge
* @return the weight of an edge
*/
public double getEdgeWeight(E e)
{
return Graph.DEFAULT_EDGE_WEIGHT;
}
/**
* Set the weight of an edge
*
* @param e the edge
* @param weight the new weight
*/
public void setEdgeWeight(E e, double weight)
{
throw new UnsupportedOperationException();
}
/**
* Add a new edge
*
* @param e the edge
* @param sourceVertex the source vertex of the edge
* @param targetVertex the target vertex of the edge
* @return true if the edge was added, false if the edge was already present
*/
public abstract boolean add(E e, V sourceVertex, V targetVertex);
/**
* Get the intrusive edge of an edge.
*
* @param e the edge
* @return the intrusive edge
*/
protected abstract IE getIntrusiveEdge(E e);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy