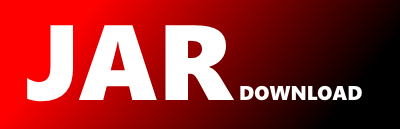
com.salesforce.jgrapht.util.FibonacciHeapNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of AptSpringProcessor Show documentation
Show all versions of AptSpringProcessor Show documentation
This project contains the apt processor that implements all the checks enumerated in @Verify. It is a self contained, and
shaded jar.
The newest version!
/*
* (C) Copyright 1999-2018, by Nathan Fiedler and Contributors.
*
* JGraphT : a free Java graph-theory library
*
* See the CONTRIBUTORS.md file distributed with this work for additional
* information regarding copyright ownership.
*
* This program and the accompanying materials are made available under the
* terms of the Eclipse Public License 2.0 which is available at
* http://www.eclipse.org/legal/epl-2.0, or the
* GNU Lesser General Public License v2.1 or later
* which is available at
* http://www.gnu.org/licenses/old-licenses/lgpl-2.1-standalone.html.
*
* SPDX-License-Identifier: EPL-2.0 OR LGPL-2.1-or-later
*/
package com.salesforce.jgrapht.util;
/**
* Implements a node of the Fibonacci heap. It holds the information necessary for maintaining the
* structure of the heap. It also holds the reference to the key value (which is used to determine
* the heap structure).
*
* @param node data type
*
* @author Nathan Fiedler
*/
public class FibonacciHeapNode
{
/**
* Node data.
*/
T data;
/**
* first child node
*/
FibonacciHeapNode child;
/**
* left sibling node
*/
FibonacciHeapNode left;
/**
* parent node
*/
FibonacciHeapNode parent;
/**
* right sibling node
*/
FibonacciHeapNode right;
/**
* true if this node has had a child removed since this node was added to its parent
*/
boolean mark;
/**
* key value for this node
*/
double key;
/**
* number of children of this node (does not count grandchildren)
*/
int degree;
/**
* Constructs a new node.
*
* @param data data for this node
*/
public FibonacciHeapNode(T data)
{
this.data = data;
}
/**
* Obtain the key for this node.
*
* @return the key
*/
public final double getKey()
{
return key;
}
/**
* Obtain the data for this node.
*
* @return the data
*/
public final T getData()
{
return data;
}
/**
* Return the string representation of this object.
*
* @return string representing this object
*/
@Override
public String toString()
{
return Double.toString(key);
}
// toString
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy