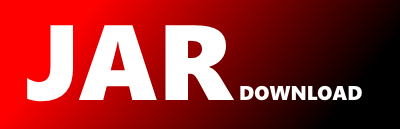
com.salesforce.hyperdb.grpc.HyperServiceGrpc Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jdbc Show documentation
Show all versions of jdbc Show documentation
Salesforce Data Cloud JDBC Driver
package com.salesforce.hyperdb.grpc;
import static io.grpc.MethodDescriptor.generateFullMethodName;
/**
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.68.1)",
comments = "Source: hyper_service.proto")
@io.grpc.stub.annotations.GrpcGenerated
public final class HyperServiceGrpc {
private HyperServiceGrpc() {}
public static final java.lang.String SERVICE_NAME = "salesforce.hyperdb.grpc.v1.HyperService";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor getExecuteQueryMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ExecuteQuery",
requestType = com.salesforce.hyperdb.grpc.QueryParam.class,
responseType = com.salesforce.hyperdb.grpc.ExecuteQueryResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
public static io.grpc.MethodDescriptor getExecuteQueryMethod() {
io.grpc.MethodDescriptor getExecuteQueryMethod;
if ((getExecuteQueryMethod = HyperServiceGrpc.getExecuteQueryMethod) == null) {
synchronized (HyperServiceGrpc.class) {
if ((getExecuteQueryMethod = HyperServiceGrpc.getExecuteQueryMethod) == null) {
HyperServiceGrpc.getExecuteQueryMethod = getExecuteQueryMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ExecuteQuery"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.salesforce.hyperdb.grpc.QueryParam.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.salesforce.hyperdb.grpc.ExecuteQueryResponse.getDefaultInstance()))
.setSchemaDescriptor(new HyperServiceMethodDescriptorSupplier("ExecuteQuery"))
.build();
}
}
}
return getExecuteQueryMethod;
}
private static volatile io.grpc.MethodDescriptor getGetQueryResultMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetQueryResult",
requestType = com.salesforce.hyperdb.grpc.QueryResultParam.class,
responseType = com.salesforce.hyperdb.grpc.QueryResult.class,
methodType = io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
public static io.grpc.MethodDescriptor getGetQueryResultMethod() {
io.grpc.MethodDescriptor getGetQueryResultMethod;
if ((getGetQueryResultMethod = HyperServiceGrpc.getGetQueryResultMethod) == null) {
synchronized (HyperServiceGrpc.class) {
if ((getGetQueryResultMethod = HyperServiceGrpc.getGetQueryResultMethod) == null) {
HyperServiceGrpc.getGetQueryResultMethod = getGetQueryResultMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetQueryResult"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.salesforce.hyperdb.grpc.QueryResultParam.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.salesforce.hyperdb.grpc.QueryResult.getDefaultInstance()))
.setSchemaDescriptor(new HyperServiceMethodDescriptorSupplier("GetQueryResult"))
.build();
}
}
}
return getGetQueryResultMethod;
}
private static volatile io.grpc.MethodDescriptor getGetQueryInfoMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "GetQueryInfo",
requestType = com.salesforce.hyperdb.grpc.QueryInfoParam.class,
responseType = com.salesforce.hyperdb.grpc.QueryInfo.class,
methodType = io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
public static io.grpc.MethodDescriptor getGetQueryInfoMethod() {
io.grpc.MethodDescriptor getGetQueryInfoMethod;
if ((getGetQueryInfoMethod = HyperServiceGrpc.getGetQueryInfoMethod) == null) {
synchronized (HyperServiceGrpc.class) {
if ((getGetQueryInfoMethod = HyperServiceGrpc.getGetQueryInfoMethod) == null) {
HyperServiceGrpc.getGetQueryInfoMethod = getGetQueryInfoMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "GetQueryInfo"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.salesforce.hyperdb.grpc.QueryInfoParam.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.salesforce.hyperdb.grpc.QueryInfo.getDefaultInstance()))
.setSchemaDescriptor(new HyperServiceMethodDescriptorSupplier("GetQueryInfo"))
.build();
}
}
}
return getGetQueryInfoMethod;
}
private static volatile io.grpc.MethodDescriptor getCancelQueryMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "CancelQuery",
requestType = com.salesforce.hyperdb.grpc.CancelQueryParam.class,
responseType = com.google.protobuf.Empty.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getCancelQueryMethod() {
io.grpc.MethodDescriptor getCancelQueryMethod;
if ((getCancelQueryMethod = HyperServiceGrpc.getCancelQueryMethod) == null) {
synchronized (HyperServiceGrpc.class) {
if ((getCancelQueryMethod = HyperServiceGrpc.getCancelQueryMethod) == null) {
HyperServiceGrpc.getCancelQueryMethod = getCancelQueryMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "CancelQuery"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.salesforce.hyperdb.grpc.CancelQueryParam.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.google.protobuf.Empty.getDefaultInstance()))
.setSchemaDescriptor(new HyperServiceMethodDescriptorSupplier("CancelQuery"))
.build();
}
}
}
return getCancelQueryMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static HyperServiceStub newStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public HyperServiceStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new HyperServiceStub(channel, callOptions);
}
};
return HyperServiceStub.newStub(factory, channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static HyperServiceBlockingStub newBlockingStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public HyperServiceBlockingStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new HyperServiceBlockingStub(channel, callOptions);
}
};
return HyperServiceBlockingStub.newStub(factory, channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static HyperServiceFutureStub newFutureStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public HyperServiceFutureStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new HyperServiceFutureStub(channel, callOptions);
}
};
return HyperServiceFutureStub.newStub(factory, channel);
}
/**
*/
public interface AsyncService {
/**
*/
default void executeQuery(com.salesforce.hyperdb.grpc.QueryParam request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getExecuteQueryMethod(), responseObserver);
}
/**
*/
default void getQueryResult(com.salesforce.hyperdb.grpc.QueryResultParam request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetQueryResultMethod(), responseObserver);
}
/**
*/
default void getQueryInfo(com.salesforce.hyperdb.grpc.QueryInfoParam request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetQueryInfoMethod(), responseObserver);
}
/**
*/
default void cancelQuery(com.salesforce.hyperdb.grpc.CancelQueryParam request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getCancelQueryMethod(), responseObserver);
}
}
/**
* Base class for the server implementation of the service HyperService.
*/
public static abstract class HyperServiceImplBase
implements io.grpc.BindableService, AsyncService {
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return HyperServiceGrpc.bindService(this);
}
}
/**
* A stub to allow clients to do asynchronous rpc calls to service HyperService.
*/
public static final class HyperServiceStub
extends io.grpc.stub.AbstractAsyncStub {
private HyperServiceStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected HyperServiceStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new HyperServiceStub(channel, callOptions);
}
/**
*/
public void executeQuery(com.salesforce.hyperdb.grpc.QueryParam request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncServerStreamingCall(
getChannel().newCall(getExecuteQueryMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void getQueryResult(com.salesforce.hyperdb.grpc.QueryResultParam request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncServerStreamingCall(
getChannel().newCall(getGetQueryResultMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void getQueryInfo(com.salesforce.hyperdb.grpc.QueryInfoParam request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncServerStreamingCall(
getChannel().newCall(getGetQueryInfoMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void cancelQuery(com.salesforce.hyperdb.grpc.CancelQueryParam request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getCancelQueryMethod(), getCallOptions()), request, responseObserver);
}
}
/**
* A stub to allow clients to do synchronous rpc calls to service HyperService.
*/
public static final class HyperServiceBlockingStub
extends io.grpc.stub.AbstractBlockingStub {
private HyperServiceBlockingStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected HyperServiceBlockingStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new HyperServiceBlockingStub(channel, callOptions);
}
/**
*/
public java.util.Iterator executeQuery(
com.salesforce.hyperdb.grpc.QueryParam request) {
return io.grpc.stub.ClientCalls.blockingServerStreamingCall(
getChannel(), getExecuteQueryMethod(), getCallOptions(), request);
}
/**
*/
public java.util.Iterator getQueryResult(
com.salesforce.hyperdb.grpc.QueryResultParam request) {
return io.grpc.stub.ClientCalls.blockingServerStreamingCall(
getChannel(), getGetQueryResultMethod(), getCallOptions(), request);
}
/**
*/
public java.util.Iterator getQueryInfo(
com.salesforce.hyperdb.grpc.QueryInfoParam request) {
return io.grpc.stub.ClientCalls.blockingServerStreamingCall(
getChannel(), getGetQueryInfoMethod(), getCallOptions(), request);
}
/**
*/
public com.google.protobuf.Empty cancelQuery(com.salesforce.hyperdb.grpc.CancelQueryParam request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getCancelQueryMethod(), getCallOptions(), request);
}
}
/**
* A stub to allow clients to do ListenableFuture-style rpc calls to service HyperService.
*/
public static final class HyperServiceFutureStub
extends io.grpc.stub.AbstractFutureStub {
private HyperServiceFutureStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected HyperServiceFutureStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new HyperServiceFutureStub(channel, callOptions);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture cancelQuery(
com.salesforce.hyperdb.grpc.CancelQueryParam request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getCancelQueryMethod(), getCallOptions()), request);
}
}
private static final int METHODID_EXECUTE_QUERY = 0;
private static final int METHODID_GET_QUERY_RESULT = 1;
private static final int METHODID_GET_QUERY_INFO = 2;
private static final int METHODID_CANCEL_QUERY = 3;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final AsyncService serviceImpl;
private final int methodId;
MethodHandlers(AsyncService serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_EXECUTE_QUERY:
serviceImpl.executeQuery((com.salesforce.hyperdb.grpc.QueryParam) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_QUERY_RESULT:
serviceImpl.getQueryResult((com.salesforce.hyperdb.grpc.QueryResultParam) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_QUERY_INFO:
serviceImpl.getQueryInfo((com.salesforce.hyperdb.grpc.QueryInfoParam) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_CANCEL_QUERY:
serviceImpl.cancelQuery((com.salesforce.hyperdb.grpc.CancelQueryParam) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new AssertionError();
}
}
}
public static final io.grpc.ServerServiceDefinition bindService(AsyncService service) {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getExecuteQueryMethod(),
io.grpc.stub.ServerCalls.asyncServerStreamingCall(
new MethodHandlers<
com.salesforce.hyperdb.grpc.QueryParam,
com.salesforce.hyperdb.grpc.ExecuteQueryResponse>(
service, METHODID_EXECUTE_QUERY)))
.addMethod(
getGetQueryResultMethod(),
io.grpc.stub.ServerCalls.asyncServerStreamingCall(
new MethodHandlers<
com.salesforce.hyperdb.grpc.QueryResultParam,
com.salesforce.hyperdb.grpc.QueryResult>(
service, METHODID_GET_QUERY_RESULT)))
.addMethod(
getGetQueryInfoMethod(),
io.grpc.stub.ServerCalls.asyncServerStreamingCall(
new MethodHandlers<
com.salesforce.hyperdb.grpc.QueryInfoParam,
com.salesforce.hyperdb.grpc.QueryInfo>(
service, METHODID_GET_QUERY_INFO)))
.addMethod(
getCancelQueryMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.salesforce.hyperdb.grpc.CancelQueryParam,
com.google.protobuf.Empty>(
service, METHODID_CANCEL_QUERY)))
.build();
}
private static abstract class HyperServiceBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
HyperServiceBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return com.salesforce.hyperdb.grpc.HyperDatabaseServiceProto.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("HyperService");
}
}
private static final class HyperServiceFileDescriptorSupplier
extends HyperServiceBaseDescriptorSupplier {
HyperServiceFileDescriptorSupplier() {}
}
private static final class HyperServiceMethodDescriptorSupplier
extends HyperServiceBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final java.lang.String methodName;
HyperServiceMethodDescriptorSupplier(java.lang.String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (HyperServiceGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new HyperServiceFileDescriptorSupplier())
.addMethod(getExecuteQueryMethod())
.addMethod(getGetQueryResultMethod())
.addMethod(getGetQueryInfoMethod())
.addMethod(getCancelQueryMethod())
.build();
}
}
}
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy