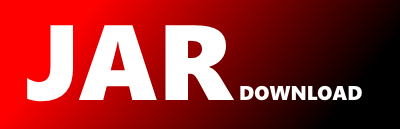
com.salesforce.einsteinbot.sdk.cache.InMemoryCache Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of einstein-bot-sdk-java Show documentation
Show all versions of einstein-bot-sdk-java Show documentation
Java SDK to interact with Einstein Bots Runtime.
This SDK is a wrapper around the Einstein Bots Runtime API that provides a few added features out of the box like Auth support, Session management
/*
* Copyright (c) 2022, salesforce.com, inc.
* All rights reserved.
* SPDX-License-Identifier: BSD-3-Clause
* For full license text, see the LICENSE file in the repo root or https://opensource.org/licenses/BSD-3-Clause
*/
package com.salesforce.einsteinbot.sdk.cache;
import com.google.common.cache.CacheBuilder;
import java.util.Optional;
import java.util.concurrent.TimeUnit;
/**
* InMemoryCache is an implementation of {@link Cache} which caches entries in memory. It is
* intended for testing and is not meant to be used in a distributed or production environment.
*/
public class InMemoryCache implements Cache {
private final com.google.common.cache.Cache cache;
public InMemoryCache(long ttlSeconds) {
cache = CacheBuilder.newBuilder().expireAfterAccess(ttlSeconds, TimeUnit.SECONDS).build();
}
@Override
public Optional get(String key) {
String val = cache.getIfPresent(key);
return Optional.ofNullable(val);
}
@Override
public void set(String key, String val) {
cache.put(key, val);
}
/**
* This method does not respect the ttlSeconds parameter.
*/
@Override
public void set(String key, String val, long ttlSeconds) {
cache.put(key, val);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy