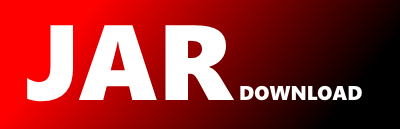
com.salesforce.einsteinbot.sdk.model.RequestEnvelope Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of einstein-bot-sdk-java Show documentation
Show all versions of einstein-bot-sdk-java Show documentation
Java SDK to interact with Einstein Bots Runtime.
This SDK is a wrapper around the Einstein Bots Runtime API that provides a few added features out of the box like Auth support, Session management
/*
* Einstein Bots API (BETA)
* No description provided (generated by Openapi Generator https://github.com/openapitools/openapi-generator)
*
* The version of the OpenAPI document: v4
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.salesforce.einsteinbot.sdk.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import com.salesforce.einsteinbot.sdk.model.ChoiceMessage;
import com.salesforce.einsteinbot.sdk.model.EndSessionMessage;
import com.salesforce.einsteinbot.sdk.model.ForceConfig;
import com.salesforce.einsteinbot.sdk.model.InitMessage;
import com.salesforce.einsteinbot.sdk.model.RedirectMessage;
import com.salesforce.einsteinbot.sdk.model.ResponseOptions;
import com.salesforce.einsteinbot.sdk.model.TextMessage;
import com.salesforce.einsteinbot.sdk.model.TransferFailedRequestMessage;
import com.salesforce.einsteinbot.sdk.model.TransferSucceededRequestMessage;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.util.ArrayList;
import java.util.List;
import org.openapitools.jackson.nullable.JsonNullable;
import com.fasterxml.jackson.annotation.JsonIgnore;
import org.openapitools.jackson.nullable.JsonNullable;
import java.util.NoSuchElementException;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
/**
* RequestEnvelope
*/
@JsonPropertyOrder({
RequestEnvelope.JSON_PROPERTY_SESSION_ID,
RequestEnvelope.JSON_PROPERTY_EXTERNAL_SESSION_KEY,
RequestEnvelope.JSON_PROPERTY_BOT_ID,
RequestEnvelope.JSON_PROPERTY_MESSAGES,
RequestEnvelope.JSON_PROPERTY_FORCE_CONFIG,
RequestEnvelope.JSON_PROPERTY_RESPONSE_OPTIONS
})
@JsonTypeName("RequestEnvelope")
@javax.annotation.Generated(value = "com.salesforce.einsteinbot.openapi.codegen.EinsteinBotCodeGenerator", date = "2022-03-24T00:55:11.933462Z[Etc/UTC]")
public class RequestEnvelope {
public static final String JSON_PROPERTY_SESSION_ID = "sessionId";
private JsonNullable sessionId = JsonNullable.undefined();
public static final String JSON_PROPERTY_EXTERNAL_SESSION_KEY = "externalSessionKey";
private String externalSessionKey;
public static final String JSON_PROPERTY_BOT_ID = "botId";
private String botId;
public static final String JSON_PROPERTY_MESSAGES = "messages";
private List messages = new ArrayList<>();
public static final String JSON_PROPERTY_FORCE_CONFIG = "forceConfig";
private ForceConfig forceConfig;
public static final String JSON_PROPERTY_RESPONSE_OPTIONS = "responseOptions";
private ResponseOptions responseOptions;
public RequestEnvelope() {
}
public RequestEnvelope sessionId(String sessionId) {
this.sessionId = JsonNullable.of(sessionId);
return this;
}
/**
* Chatbot Runtime Session ID
* @return sessionId
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "57904eb6-5352-4c5e-adf6-5f100572cf5d", value = "Chatbot Runtime Session ID")
@JsonIgnore
public String getSessionId() {
return sessionId.orElse(null);
}
@JsonProperty(JSON_PROPERTY_SESSION_ID)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public JsonNullable getSessionId_JsonNullable() {
return sessionId;
}
@JsonProperty(JSON_PROPERTY_SESSION_ID)
public void setSessionId_JsonNullable(JsonNullable sessionId) {
this.sessionId = sessionId;
}
public void setSessionId(String sessionId) {
this.sessionId = JsonNullable.of(sessionId);
}
public RequestEnvelope externalSessionKey(String externalSessionKey) {
this.externalSessionKey = externalSessionKey;
return this;
}
/**
* Channel specific unique key for the conversation
* @return externalSessionKey
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "57904eb6-5352-4c5e-adf6-5f100572cf5d", value = "Channel specific unique key for the conversation")
@JsonProperty(JSON_PROPERTY_EXTERNAL_SESSION_KEY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getExternalSessionKey() {
return externalSessionKey;
}
@JsonProperty(JSON_PROPERTY_EXTERNAL_SESSION_KEY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setExternalSessionKey(String externalSessionKey) {
this.externalSessionKey = externalSessionKey;
}
public RequestEnvelope botId(String botId) {
this.botId = botId;
return this;
}
/**
* Chatbot ID
* @return botId
**/
@javax.annotation.Nonnull
@ApiModelProperty(example = "0XxRM0000004Cyw0AE", required = true, value = "Chatbot ID")
@JsonProperty(JSON_PROPERTY_BOT_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getBotId() {
return botId;
}
@JsonProperty(JSON_PROPERTY_BOT_ID)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setBotId(String botId) {
this.botId = botId;
}
public RequestEnvelope messages(List messages) {
this.messages = messages;
return this;
}
public RequestEnvelope addMessagesItem(AnyRequestMessage messagesItem) {
this.messages.add(messagesItem);
return this;
}
/**
* Input messages
* @return messages
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "Input messages")
@JsonProperty(JSON_PROPERTY_MESSAGES)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getMessages() {
return messages;
}
@JsonProperty(JSON_PROPERTY_MESSAGES)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setMessages(List messages) {
this.messages = messages;
}
public RequestEnvelope forceConfig(ForceConfig forceConfig) {
this.forceConfig = forceConfig;
return this;
}
/**
* Get forceConfig
* @return forceConfig
**/
@javax.annotation.Nonnull
@ApiModelProperty(required = true, value = "")
@JsonProperty(JSON_PROPERTY_FORCE_CONFIG)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public ForceConfig getForceConfig() {
return forceConfig;
}
@JsonProperty(JSON_PROPERTY_FORCE_CONFIG)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setForceConfig(ForceConfig forceConfig) {
this.forceConfig = forceConfig;
}
public RequestEnvelope responseOptions(ResponseOptions responseOptions) {
this.responseOptions = responseOptions;
return this;
}
/**
* Get responseOptions
* @return responseOptions
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
@JsonProperty(JSON_PROPERTY_RESPONSE_OPTIONS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ResponseOptions getResponseOptions() {
return responseOptions;
}
@JsonProperty(JSON_PROPERTY_RESPONSE_OPTIONS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setResponseOptions(ResponseOptions responseOptions) {
this.responseOptions = responseOptions;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
RequestEnvelope requestEnvelope = (RequestEnvelope) o;
return equalsNullable(this.sessionId, requestEnvelope.sessionId) &&
Objects.equals(this.externalSessionKey, requestEnvelope.externalSessionKey) &&
Objects.equals(this.botId, requestEnvelope.botId) &&
Objects.equals(this.messages, requestEnvelope.messages) &&
Objects.equals(this.forceConfig, requestEnvelope.forceConfig) &&
Objects.equals(this.responseOptions, requestEnvelope.responseOptions);
}
private static boolean equalsNullable(JsonNullable a, JsonNullable b) {
return a == b || (a != null && b != null && a.isPresent() && b.isPresent() && Objects.deepEquals(a.get(), b.get()));
}
@Override
public int hashCode() {
return Objects.hash(hashCodeNullable(sessionId), externalSessionKey, botId, messages, forceConfig, responseOptions);
}
private static int hashCodeNullable(JsonNullable a) {
if (a == null) {
return 1;
}
return a.isPresent() ? Arrays.deepHashCode(new Object[]{a.get()}) : 31;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class RequestEnvelope {\n");
sb.append(" sessionId: ").append(toIndentedString(sessionId)).append("\n");
sb.append(" externalSessionKey: ").append(toIndentedString(externalSessionKey)).append("\n");
sb.append(" botId: ").append(toIndentedString(botId)).append("\n");
sb.append(" messages: ").append(toIndentedString(messages)).append("\n");
sb.append(" forceConfig: ").append(toIndentedString(forceConfig)).append("\n");
sb.append(" responseOptions: ").append(toIndentedString(responseOptions)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy