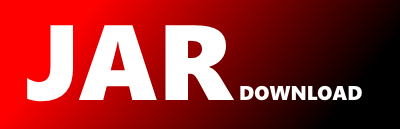
com.force.i18n.commons.util.collection.IntHashSet Maven / Gradle / Ivy
Show all versions of grammaticus Show documentation
/*
* Copyright (c) 2017, salesforce.com, inc.
* All rights reserved.
* Licensed under the BSD 3-Clause license.
* For full license text, see LICENSE.txt file in the repo root or https://opensource.org/licenses/BSD-3-Clause
*/
package com.force.i18n.commons.util.collection;
import java.io.Serializable;
import com.google.common.annotations.Beta;
/**
* This class implements the {@code IntSet} interface, backed by a hash table
* (actually a {@code IntHashMap} instance). It makes no guarantees as to the
* iteration order of the set; in particular, it does not guarantee that the
* order will remain constant over time.
*
* This class offers constant time performance for the basic operations
* ({@code add}, {@code remove}, {@code contains} and {@code size}),
* assuming the hash function disperses the elements properly among the
* buckets. Iterating over this set requires time proportional to the sum of
* the {@code IntHashSet} instance's size (the number of elements) plus the
* "capacity" of the backing {@code IntHashMap} instance (the number of
* buckets). Thus, it's very important not to set the intial capacity too
* high (or the load factor too low) if iteration performance is important.
*
* Note that this implementation is not synchronized. If multiple
* threads access a set concurrently, and at least one of the threads modifies
* the set, it must be synchronized externally.
*
* Beta class. Classes under com.force.i18n.commons package will be moved into a dedicated project.
*
* @author Based on Sun's java.util.HashSet (modified by koliver)
* @see IntSet
* @see IntHashMap
* @see IntMap
* Note: use {@link java.util.HashSet} or {@link java.util.EnumSet} instead.
*/
@Beta
public class IntHashSet extends AbstractIntSet implements Serializable {
private static final long serialVersionUID = 7500368467424833369L;
IntHashMap